Advanced Sorting Algorithms: Beyond Quick Sort and Merge Sort - Hiike
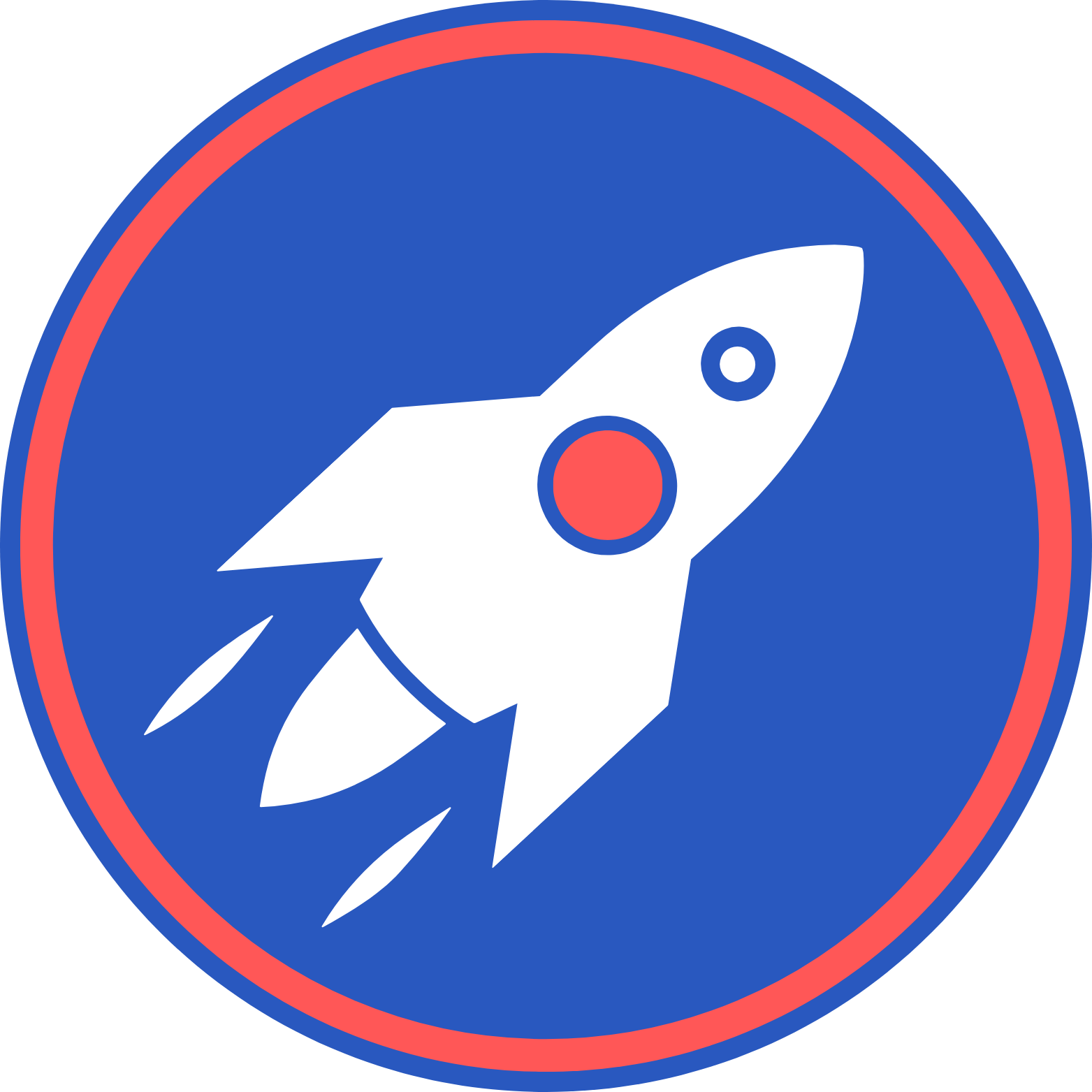
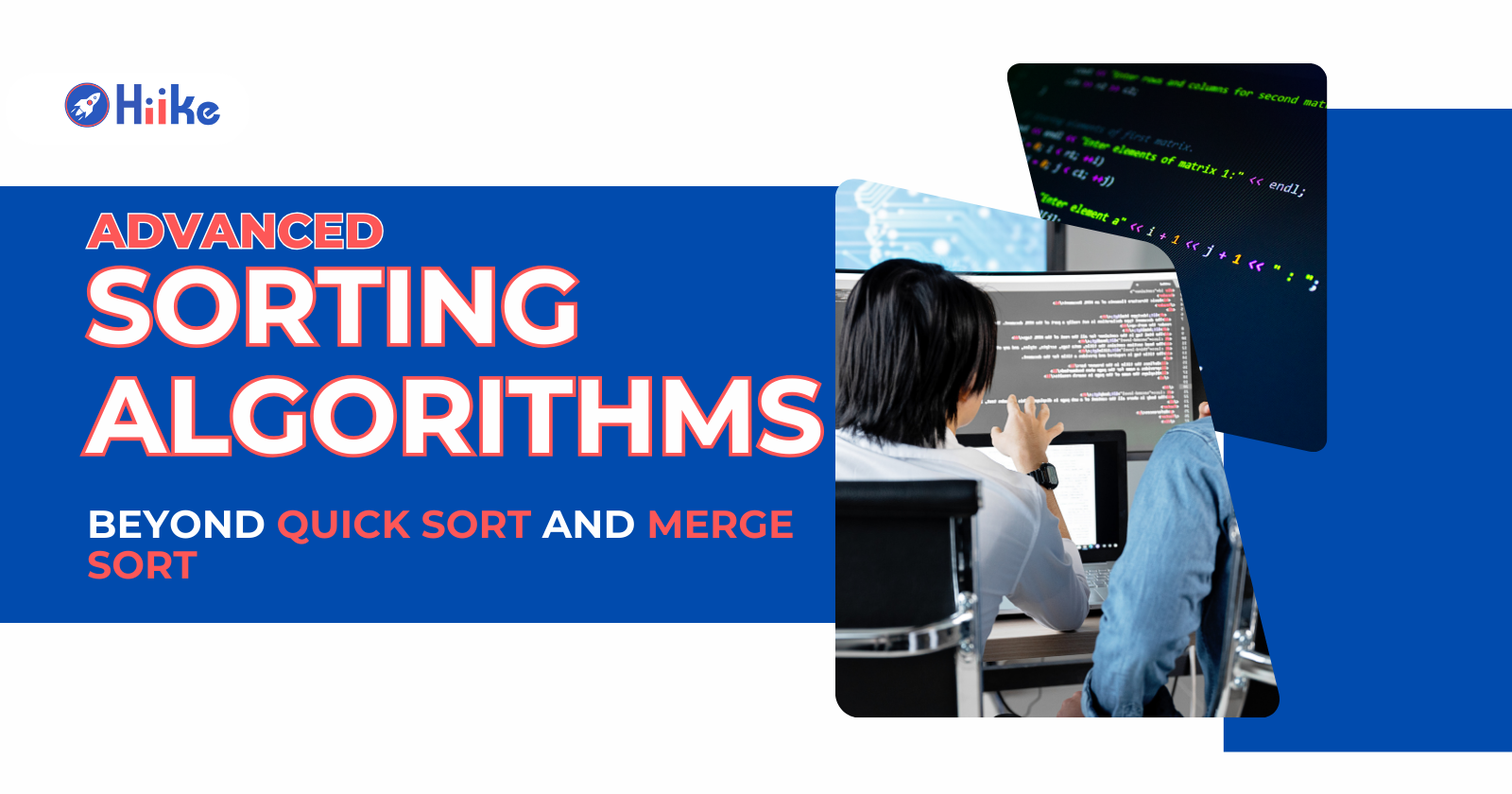
Sorting algorithms are fundamental in computer science, playing a crucial role in organizing data for efficient processing. While Quick Sort and Merge Sort are widely known and used, several advanced sorting algorithms offer unique advantages in specific scenarios. This blog post will explore these advanced algorithms, provide practical examples and exercises, compare their performance and efficiency, and discuss their applications and use cases.
Overview of Advanced Sorting Algorithms
Advanced sorting algorithms are designed to handle complex data sorting requirements more efficiently. These algorithms include:
Heap Sort
Radix Sort
Tim Sort
Counting Sort
Bucket Sort
Each algorithm has its strengths and weaknesses, making them suitable for different types of data and applications.
Detailed Explanation of Each Algorithm
Heap Sort
Heap Sort is a comparison-based sorting technique based on the binary heap data structure. It is particularly efficient for large datasets and provides a reliable worst-case performance.
Steps:
Build a max heap from the input data.
Remove the largest element from the heap and move it to the end of the array.
Heapify the remaining elements.
Repeat until all elements are sorted.
Radix Sort
Radix Sort is a non-comparison sorting algorithm that sorts integers by processing individual digits. It is particularly efficient for large lists of integers.
Steps:
Sort the elements based on the least significant digit.
Move to the next significant digit and sort again.
Repeat the process for all digits.
Tim Sort
Tim Sort is a hybrid sorting algorithm derived from Merge Sort and Insertion Sort. It is optimized for real-world data and used as the default sorting algorithm in Python and Java.
Steps:
Divide the array into small chunks (runs) and sort each run using Insertion Sort.
Merge the sorted runs using a modified Merge Sort.
Counting Sort
Counting Sort is a non-comparison-based algorithm that sorts elements by counting the occurrences of each unique element. It is effective for sorting integers within a specific range.
Steps:
Count the number of occurrences of each element.
Calculate the position of each element in the sorted array.
Place the elements in the correct positions.
Bucket Sort
Bucket Sort distributes elements into several buckets and then sorts each bucket individually. It is particularly useful for sorting floating-point numbers uniformly distributed over a range.
Steps:
Divide the range of input data into equal-sized buckets.
Distribute the elements into these buckets.
Sort each bucket individually using a suitable sorting algorithm.
Concatenate the sorted buckets.
Practical Examples and Exercises
Exercise 1: Implementing Heap Sort
Write a function to implement Heap Sort and test it with an array of random integers.
def heapify(arr, n, i):
largest = i
left = 2 * i + 1
right = 2 * i + 2
if left < n and arr[i] < arr[left]:
largest = left
if right < n and arr[largest] < arr[right]:
largest = right
if largest != i:
arr[i], arr[largest] = arr[largest], arr[i]
heapify(arr, n, largest)
def heap_sort(arr):
n = len(arr)
for i in range(n//2 - 1, -1, -1):
heapify(arr, n, i)
for i in range(n-1, 0, -1):
arr[i], arr[0] = arr[0], arr[i]
heapify(arr, i, 0)
arr = [4, 10, 3, 5, 1]
heap_sort(arr)
print("Sorted array is:", arr)
Exercise 2: Implementing Radix Sort
Write a function to implement Radix Sort and test it with an array of random integers.
def counting_sort(arr, exp):
n = len(arr)
output = [0] * n
count = [0] * 10
for i in range(n):
index = arr[i] // exp
count[index % 10] += 1
for i in range(1, 10):
count[i] += count[i - 1]
i = n - 1
while i >= 0:
index = arr[i] // exp
output[count[index % 10] - 1] = arr[i]
count[index % 10] -= 1
i -= 1
for i in range(n):
arr[i] = output[i]
def radix_sort(arr):
max_val = max(arr)
exp = 1
while max_val // exp > 0:
counting_sort(arr, exp)
exp *= 10
arr = [170, 45, 75, 90, 802, 24, 2, 66]
radix_sort(arr)
print("Sorted array is:", arr)
Comparison of Performance and Efficiency
Heap Sort: O(n log n) in all cases. It uses O(1) extra space and is not stable.
Radix Sort: O(nk), where k is the number of digits. It uses O(n + k) space and is stable.
Tim Sort: O(n log n) on average. It uses O(n) extra space and is stable.
Counting Sort: O(n + k), where k is the range of input. It uses O(k) extra space and is stable.
Bucket Sort: O(n + k), where k is the number of buckets. It uses O(n + k) space and can be stable or unstable depending on the underlying sorting algorithm used within the buckets.
**
Applications and Use Cases**
Heap Sort: Suitable for large datasets where memory is limited.
Radix Sort: Ideal for sorting integers or strings with fixed-length parts.
Tim Sort: Used in real-world applications like Python's sort() and Java's Arrays.sort().
Counting Sort: Effective for sorting small integers within a known range.
Bucket Sort: Useful for sorting floating-point numbers or uniformly distributed data.
Conclusion
Advanced sorting algorithms offer various advantages over traditional methods, particularly in specific scenarios where they can significantly enhance performance and efficiency. Understanding these algorithms allows developers to select the best tool for their particular needs.
At Hiike, we specialize in equipping developers with comprehensive knowledge and practical skills in Data Structures, Algorithms, and System Design. Our Top 30 Program provides advanced training, real-world scenarios, and expert mentorship to help you excel in your career. Join Hiike today and take the next step towards mastering advanced sorting algorithms and beyond.
For more information, visit our website and explore our courses designed to elevate your skills and open doors to exciting career opportunities in the tech industry.
Subscribe to my newsletter
Read articles from Hiike directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
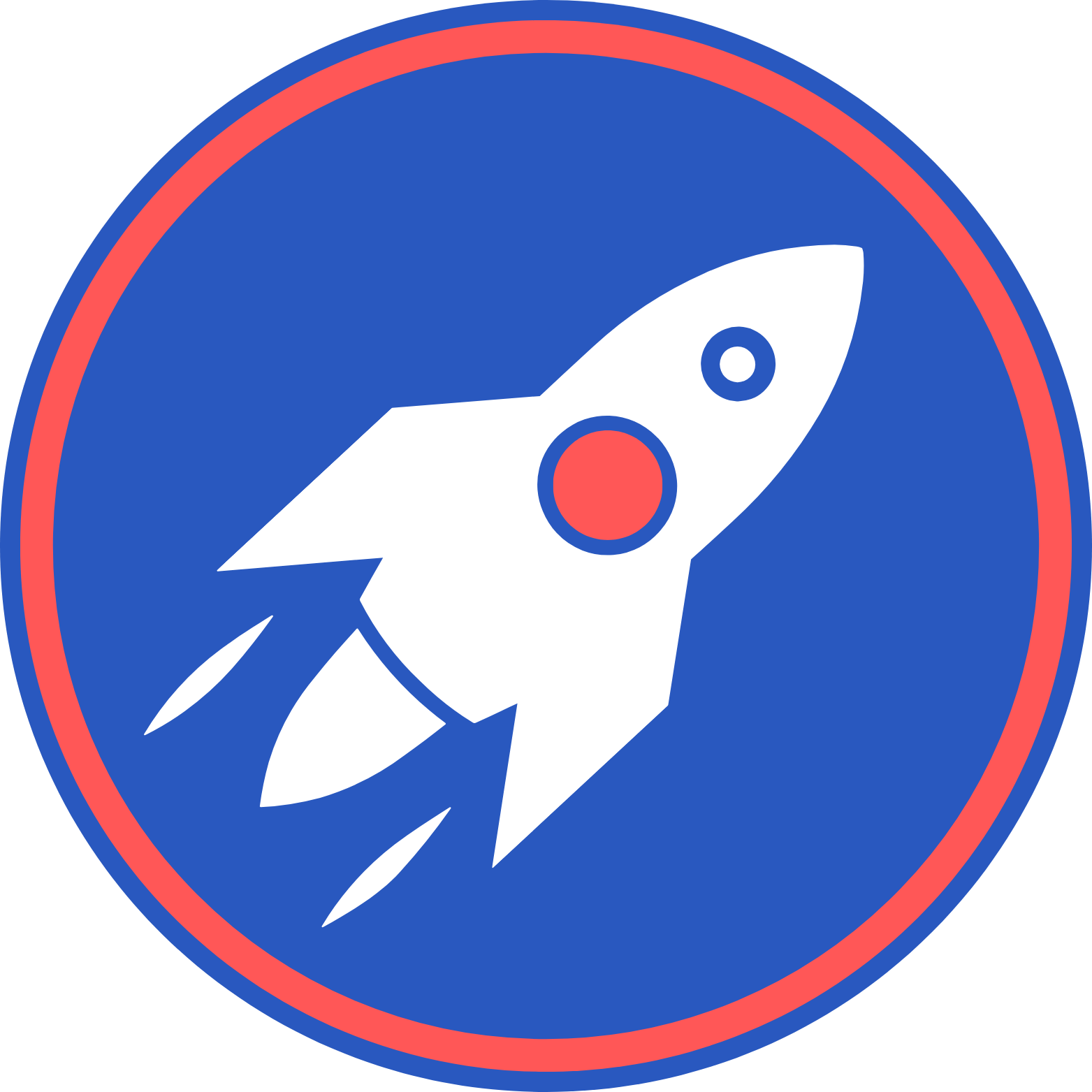
Hiike
Hiike
๐๐๐ข๐ข๐ค๐ - Crafting Your Tech Odyssey! ๐ Join Hiike for immersive learning, expert mentorship, and accelerated career growth. Our IITian-led courses and vibrant community ensure you excel in Data Structures, Algorithms, and System Design. Transform your tech career with Hiike today!