Understanding Wrappers in Java
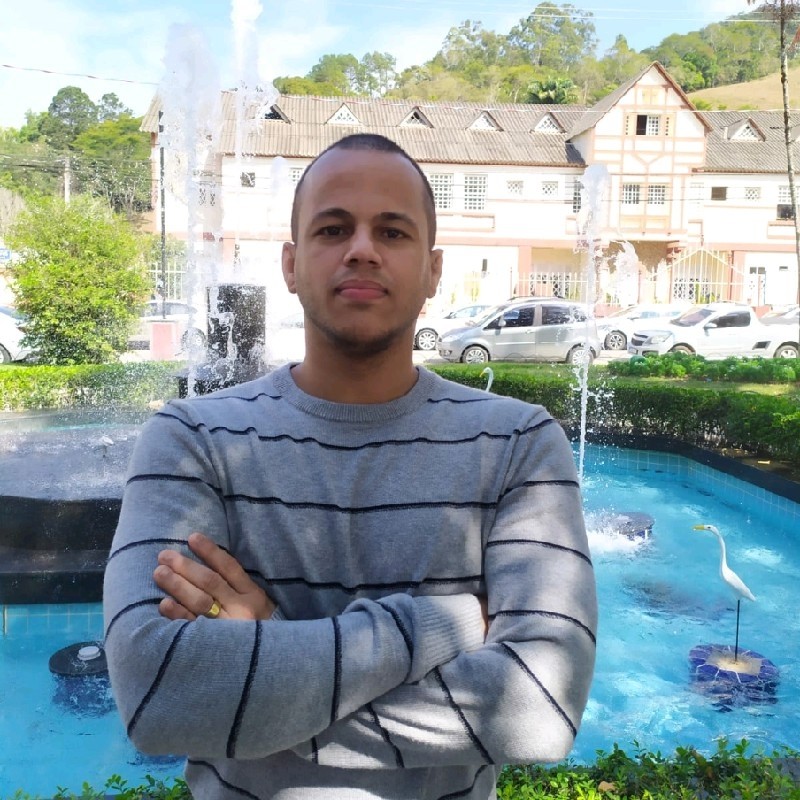
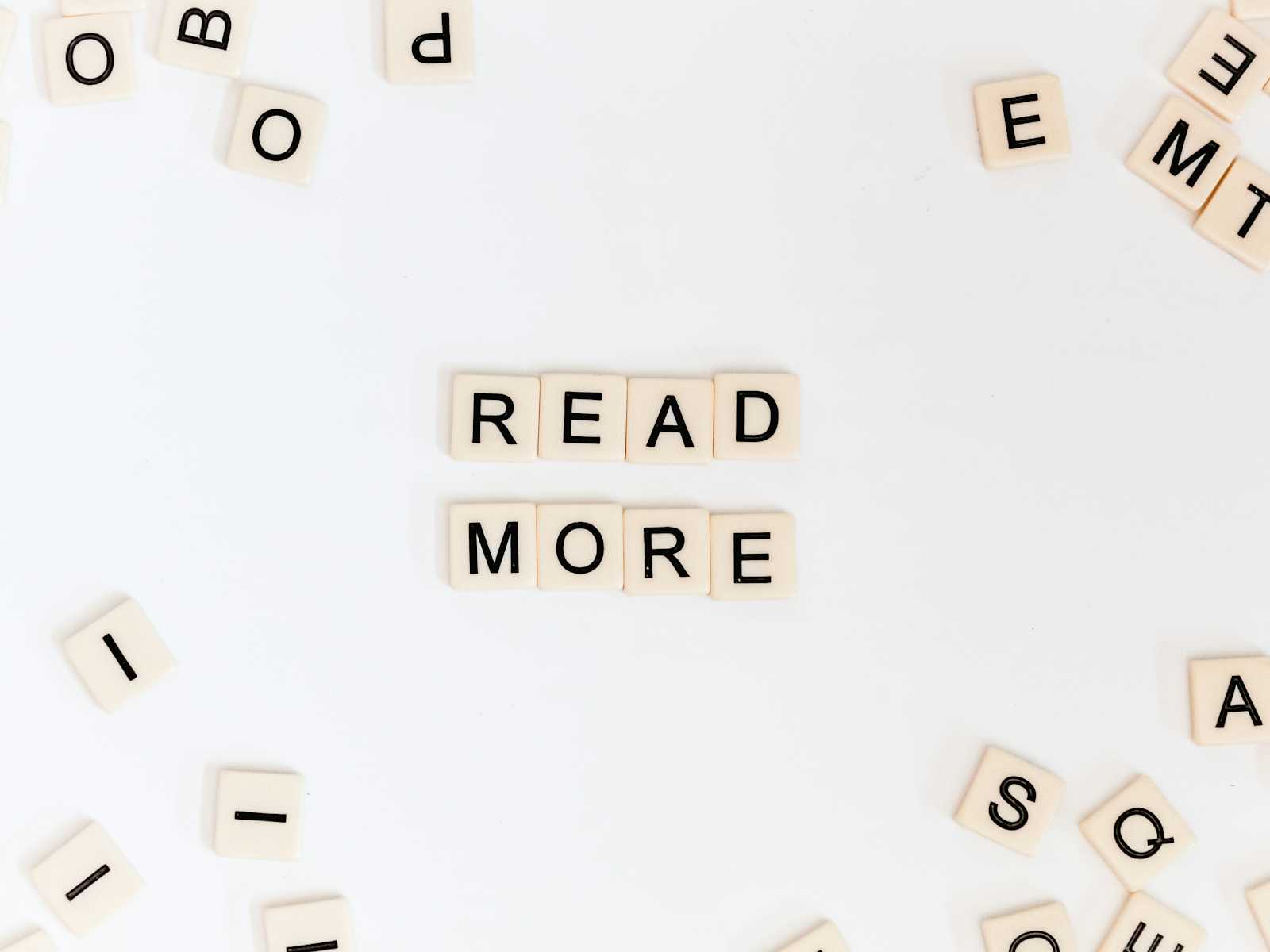
Introduction
Wrappers in Java are a crucial yet often overlooked feature. They provide a way to use primitive data types as objects, which is essential for various Java functionalities like collections and generics. This article aims to provide an in-depth understanding of wrappers, their necessity, usage, and applications with practical examples.
What Are Wrappers?
Wrappers are classes in Java that encapsulate a primitive data type into an object. Java provides a wrapper class for each of the eight primitive data types:
byte
->Byte
short
->Short
int
->Integer
long
->Long
float
->Float
double
->Double
char
->Character
boolean
->Boolean
Why Are Wrappers Necessary?
Object-Oriented Nature: Java is an object-oriented language, and certain features like collections and generics require objects. Wrappers convert primitives into objects to work with these features.
Utility Methods: Wrapper classes provide useful utility methods for converting between types, parsing strings, and performing operations.
Immutable Objects: Wrappers are immutable, meaning once created, their value cannot be changed. This makes them thread-safe.
When Are Wrappers Used?
Wrappers are used in several situations:
Collections Framework: Collections (like
ArrayList
,HashSet
) cannot store primitives directly.Generics: Java Generics work only with objects, not primitives.
Reflection: Reflection API requires objects.
Serialization: Only objects can be serialized.
Auto-boxing and Unboxing
Auto-boxing is the automatic conversion that the Java compiler makes between the primitive types and their corresponding object wrapper classes. For example, converting an int
to an Integer
, a double
to a Double
, and so on.
Example:
List<Integer> list = new ArrayList<>();
list.add(10); // Autoboxing converts int to Integer
int num = list.get(0); // Unboxing converts Integer to int
Explanation:
list.add(10)
converts the primitiveint
10 to anInteger
object automatically.list.get(0)
retrieves theInteger
object and automatically converts it back to a primitiveint
.
Comparison with Wrappers
When comparing wrapper objects, it's important to use .equals()
instead of ==
. The ==
operator compares references, while .equals()
compares values.
Example:
Integer a = 128;
Integer b = 128;
System.out.println(a == b); // false, compares references
System.out.println(a.equals(b)); // true, compares values
Simple Examples
Example 1: Converting Primitive to Wrapper and Vice Versa
public class WrapperExample {
public static void main(String[] args) {
// Primitive to Wrapper
int primitiveInt = 5;
Integer wrapperInt = Integer.valueOf(primitiveInt);
System.out.println("Wrapper Integer: " + wrapperInt);
// Wrapper to Primitive
int unwrappedInt = wrapperInt.intValue();
System.out.println("Primitive Integer: " + unwrappedInt);
}
}
Integer.valueOf(primitiveInt)
converts primitive int to Integer object.wrapperInt.intValue()
converts Integer object back to primitive int.
Example 2: Using Wrappers in Collections
import java.util.ArrayList;
import java.util.List;
public class CollectionExample {
public static void main(String[] args) {
List<Integer> intList = new ArrayList<>();
intList.add(10); // Autoboxing converts int to Integer
intList.add(20);
System.out.println("List: " + intList);
int sum = 0;
for (Integer num : intList) {
sum += num; // Unboxing converts Integer to int
}
System.out.println("Sum: " + sum);
}
}
List<Integer>
can store Integer objects, not int primitives.Autoboxing and unboxing automatically convert between primitives and wrappers.
Example 3: Parsing Strings to Primitives
public class ParsingExample {
public static void main(String[] args) {
String numberStr = "100";
int number = Integer.parseInt(numberStr); // Convert string to int
System.out.println("Parsed Integer: " + number);
String booleanStr = "true";
boolean bool = Boolean.parseBoolean(booleanStr); // Convert string to boolean
System.out.println("Parsed Boolean: " + bool);
}
}
Integer.parseInt(numberStr)
converts string to int.Boolean.parseBoolean(booleanStr)
converts string to boolean.
Complex Examples
Example 1: Using Wrappers with Generics
import java.util.ArrayList;
import java.util.List;
public class GenericsExample<T> {
private List<T> items = new ArrayList<>();
public void addItem(T item) {
items.add(item);
}
public T getItem(int index) {
return items.get(index);
}
public static void main(String[] args) {
GenericsExample<Integer> intList = new GenericsExample<>();
intList.addItem(10);
intList.addItem(20);
System.out.println("First Item: " + intList.getItem(0));
GenericsExample<Double> doubleList = new GenericsExample<>();
doubleList.addItem(15.5);
doubleList.addItem(25.5);
System.out.println("First Item: " + doubleList.getItem(0));
}
}
GenericsExample<T>
uses a generic type T, which can be any wrapper type.addItem(T item)
adds an item to the list.getItem(int index)
retrieves an item from the list.
Example 2: Using Wrappers in Serialization
import java.io.*;
public class SerializationExample implements Serializable {
private static final long serialVersionUID = 1L;
private Integer id;
private String name;
public SerializationExample(Integer id, String name) {
this.id = id;
this.name = name;
}
public static void main(String[] args) {
SerializationExample example = new SerializationExample(1, "John Doe");
String filename = "example.ser";
// Serialize the object
try (ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream(filename))) {
out.writeObject(example);
System.out.println("Object serialized successfully");
} catch (IOException e) {
e.printStackTrace();
}
// Deserialize the object
try (ObjectInputStream in = new ObjectInputStream(new FileInputStream(filename))) {
SerializationExample deserializedExample = (SerializationExample) in.readObject();
System.out.println("Object deserialized successfully: " + deserializedExample.name);
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
Implements
Serializable
to allow the class to be serialized.Uses
ObjectOutputStream
to write the object to a file.Uses
ObjectInputStream
to read the object from the file.
Example 3: Using Wrappers with Reflection
import java.lang.reflect.Method;
public class ReflectionExample {
public static void main(String[] args) {
try {
Class<?> cls = Class.forName("java.lang.Integer");
Method method = cls.getMethod("parseInt", String.class);
int value = (int) method.invoke(null, "123");
System.out.println("Parsed value using reflection: " + value);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Uses
Class.forName("java.lang.Integer")
to get theInteger
class.Retrieves the
parseInt
method usinggetMethod("parseInt", String.class)
.Invokes the method with the string "123" and prints the parsed value.
Real-world Example: Using Wrappers in a Financial Application
Consider a financial application that processes transactions. Wrappers can be used to handle null values and provide type safety.
Without Wrappers:
public class Transaction {
private double amount;
public Transaction(double amount) {
this.amount = amount;
}
public double getAmount() {
return amount;
}
}
public class FinancialApp {
public static void main(String[] args) {
Transaction[] transactions = {
new Transaction(100.0),
new Transaction(200.0),
null,
new Transaction(300.0)
};
double total = 0;
for (Transaction t : transactions) {
if (t != null) {
total += t.getAmount();
}
}
System.out.println("Total: " + total);
}
}
With Wrappers:
import java.util.Optional;
public class Transaction {
private Double amount;
public Transaction(Double amount) {
this.amount = amount;
}
public Optional<Double> getAmount() {
return Optional.ofNullable(amount);
}
}
public class FinancialApp {
public static void main(String[] args) {
Transaction[] transactions = {
new Transaction(100.0),
new Transaction(200.0),
new Transaction(null),
new Transaction(300.0)
};
double total = 0;
for (Transaction t : transactions) {
total += t.getAmount().orElse(0.0);
}
System.out.println("Total: " + total);
}
}
Uses
Optional
to handle potential null values in a type-safe manner.getAmount().orElse(0.0)
provides a default value of 0.0 if the amount is null.
Conclusion
Wrappers in Java provide a way to use primitive data types as objects, which is essential for working with collections, generics, and other Java functionalities. They offer utility methods, immutability, and object-oriented features that enhance the flexibility and robustness of Java applications. By understanding and utilizing wrappers, developers can write cleaner, safer, and more efficient code.
Subscribe to my newsletter
Read articles from André Felipe Costa Bento directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
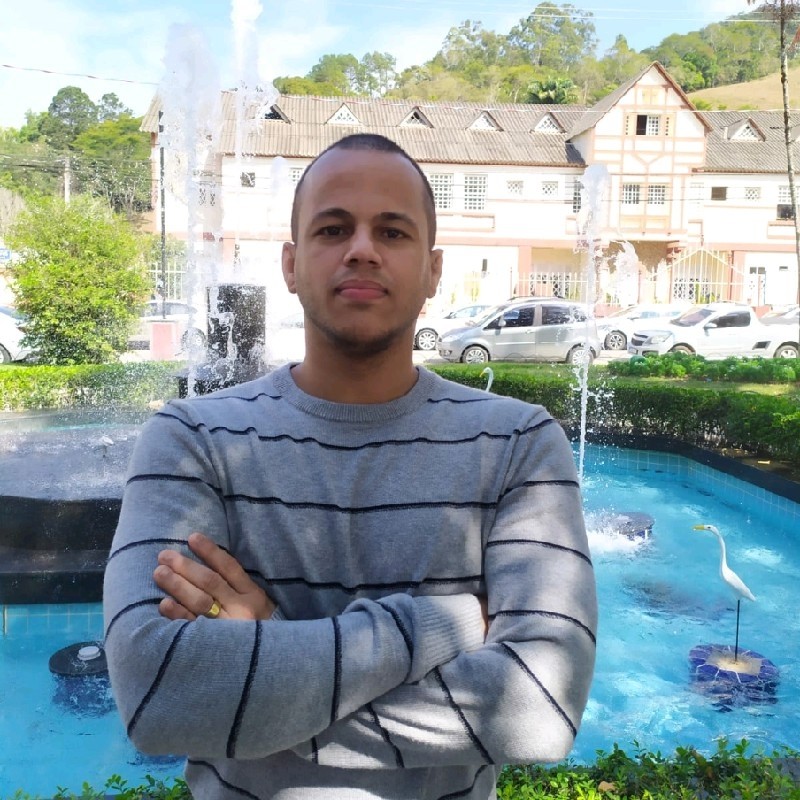
André Felipe Costa Bento
André Felipe Costa Bento
Fullstack Software Engineer.