A Comprehensive Guide to API Key Authentication
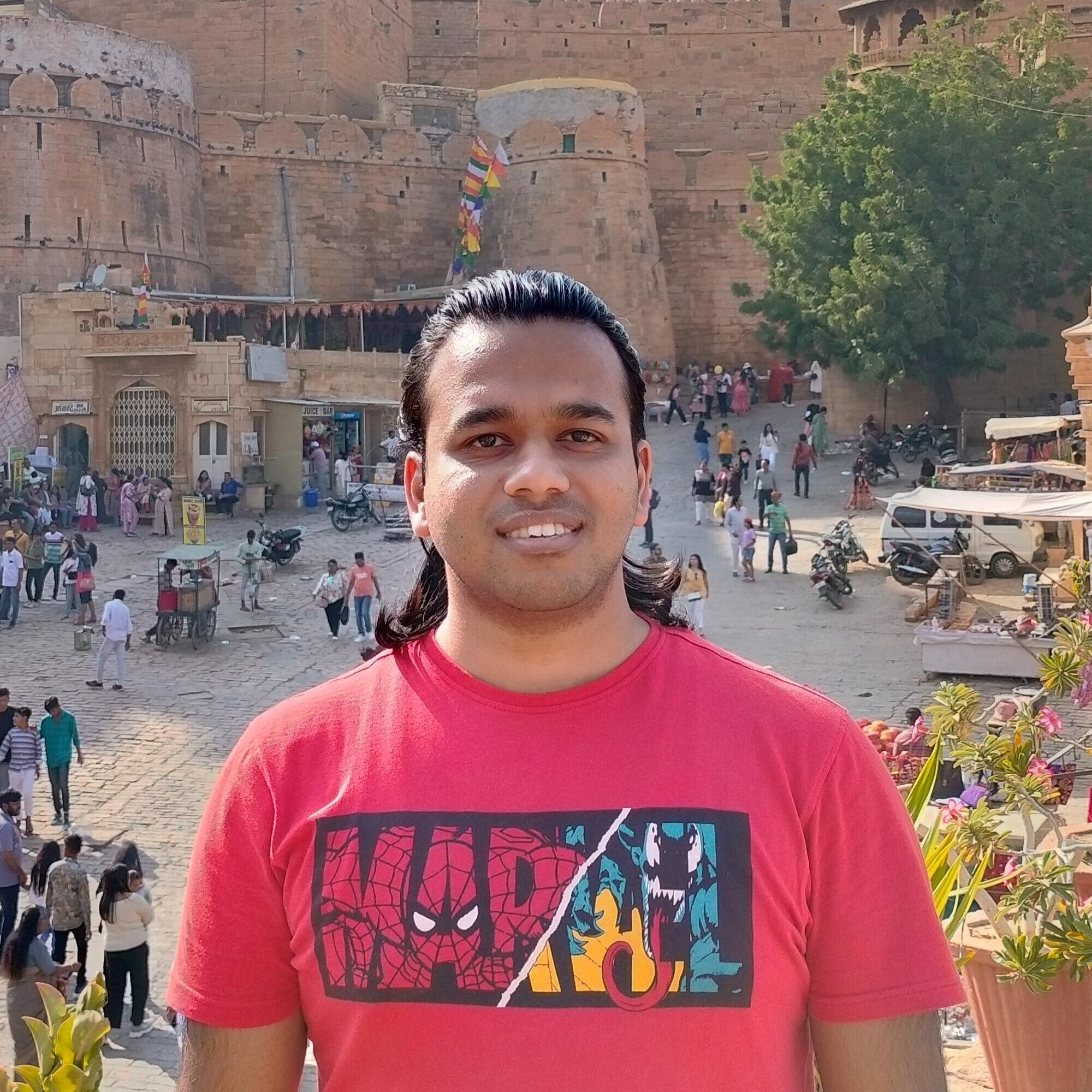
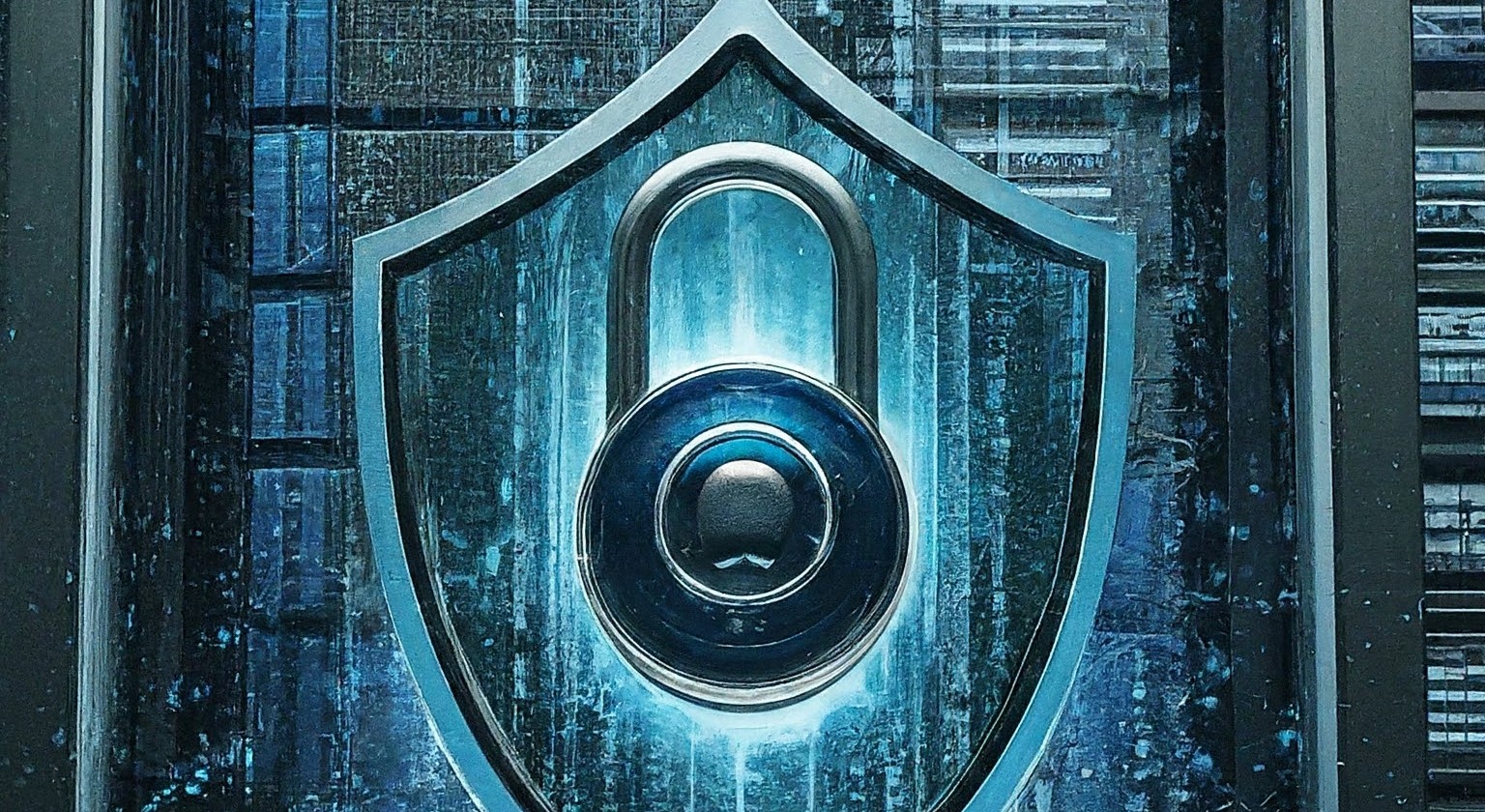
API keys are a fundamental building block in the world of web APIs, acting as a gateway between applications and the data or functionality they seek. Understanding API key authentication is crucial for both API providers and consumers. This article delves into the intricacies of this method, exploring its advantages, limitations, best practices, and implementation considerations.
Understanding API Key Authorisation
An API key is a unique, secret string of characters that identifies an application or project seeking access to an API. When a request is sent to the API, the API key is included, typically in a header or URL parameter. The API server then validates the key against its internal records. If valid, access is granted; otherwise, the request is rejected.
API key authorisation is a simple yet effective authentication method commonly used in micro-service architectures. It involves clients including a unique API key in their requests, typically as a header field. The server then validates the provided key against a predefined list of authorised keys. This approach offers several advantages:
Simplicity: API keys are easier to manage compared to complex username/password-based authentication.
Scalability: API keys can be easily revoked or granted access, making them suitable for dynamic environments.
Statelessness: API keys don’t require maintaining session state on the server, simplifying server-side implementation.
The Advantages of API Keys
API key authentication offers several advantages that contribute to its widespread adoption:
Simplicity: API keys are straightforward to implement for both providers and consumers. The authentication process is lightweight, requiring minimal code on both ends. This ease of use makes it ideal for public APIs or scenarios where user logins are unnecessary.
Ease of Integration: Embedding an API key into requests is a relatively simple task. Popular programming languages and frameworks often provide libraries that handle this process seamlessly.
Scalability: API keys can efficiently manage a large number of concurrent requests, making them suitable for APIs with high traffic volumes.
Rate Limiting and Usage Tracking: API keys can be used to enforce rate limits, restricting the number of requests an application can make within a specific timeframe. Additionally, they can be used for usage tracking, allowing providers to monitor API consumption by different applications.
Granular Control: While not as robust as other methods, some providers offer granular control with API keys. This might involve restricting access to specific API endpoints or associating quotas with individual keys.
Uncovering the Security Concerns
While convenient, API key authentication comes with inherent security limitations:
Exposure Risk: API keys are often transmitted within the request itself, making them vulnerable to interception if the communication channel is not secure (e.g., not using HTTPS).
Accidental Leaks: API keys can be accidentally leaked in code repositories, logs, or browser developer tools, compromising their secrecy.
Shared Access: An API key typically grants access to an entire application or project. If compromised, it can be misused to access all authorised functionalities.
Limited User Management: API keys cannot identify individual users within an application. This makes it difficult to track user activity or enforce user-specific permissions.
Fortifying the Gates: Best Practices for Secure API Key Usage
To mitigate the security risks associated with API keys, several best practices should be followed:
HTTPS is Mandatory: Always enforce HTTPS for all API communication. This encrypts data transmission, protecting API keys from eavesdropping.
Keep it Secret, Keep it Safe: Treat API keys with the same level of care as passwords. Avoid storing them in plain text, version control systems, or publicly accessible locations. Consider secure storage mechanisms like environment variables or dedicated secret management solutions.
Rotate Regularly: Rotate API keys periodically to minimise the damage caused by a potential compromise. This reduces the window of opportunity for attackers to exploit a leaked key.
Granular Control When Possible: If the API provider allows, implement granular access controls with API keys. Restrict access to specific functionalities or set quotas to limit potential misuse.
Monitor and Analyse: Continuously monitor API usage patterns to identify suspicious activity. Implement logging and alerting mechanisms to detect potential breaches early on.
Consider Alternatives for Sensitive Data: For APIs exposing highly sensitive data, consider stronger authentication mechanisms like OAuth or OpenID Connect, which offer additional security features.
Putting Theory into Practice: Implementing API Key Authentication
The specific implementation of API key authentication varies depending on the chosen API and programming language. Here’s a general outline:
Obtain an API Key: Register with the API provider and obtain an API key. This key will be used to authenticate your application’s requests.
Include the Key in Requests: When making requests to the API, include the API key in the appropriate location. This could be in a header field (e.g.,
Authorization: Bearer YOUR_API_KEY
) or a URL parameter. Refer to the API documentation for specific instructions.Handle Responses: The API server will respond with a success or failure message depending on the validity of the API key. Parse the response to determine if your request was authorised.
Advanced Considerations for API Key Authentication
While the core implementation is straightforward, API key authentication offers additional considerations for advanced use cases:
API Key Validation Mechanisms: API keys can be validated in different ways. Some providers use a simple string comparison, while others might employ cryptographic techniques like hashing for added security. Understanding the validation method can help with troubleshooting potential authentication failures.
API Key Lifecycle Management: Establish a process for managing the lifecycle of API keys. This includes procedures for creating, rotating, revoking, and auditing API key usage. Consider automation tools to streamline these tasks, especially for large deployments.
Client-Side vs. Server-Side Storage: Decide where to store the API key within your application. Storing it on the client-side (e.g., browser JavaScript) can be convenient but carries security risks. Server-side storage offers better protection but requires additional code to include the key in requests. Evaluate the trade-offs based on your specific security requirements.
Combining with Other Techniques: API key authentication can be combined with other security measures for a layered defence approach. This might involve IP address restrictions, rate limiting based on usage patterns, or two-factor authentication for sensitive operations.
The Future of API Keys: Evolving Landscape and Potential Alternatives
API keys remain a popular choice for API authentication, but the landscape is constantly evolving. Here are some trends to consider:
OpenID Connect (OIDC): OIDC is gaining traction as an alternative to API keys. It leverages existing user authentication mechanisms, offering stronger security and finer-grained access control.
API Gateway Integration: API gateways are becoming increasingly popular for managing APIs. These gateways can handle API key authentication and integrate with other security mechanisms for a more comprehensive approach.
Zero Trust Security: The concept of zero trust security is gaining momentum. In this model, no user or application is inherently trusted, and access is granted based on continuous verification. API key authentication can be incorporated into a zero-trust architecture with additional security checks.
While API keys are likely to remain relevant for some time, staying informed about emerging trends and alternative approaches is crucial for securing your APIs in the ever-evolving technological landscape.
Shielding Your Endpoints: API Key Authorisation in GoFr
In the world of micro-services, robust security is paramount. GoFr, with its focus on micro-service development, provides built-in functionalities for various security aspects. One such crucial feature is API key authorisation, allowing you to control access to your APIs and ensure only authorised clients can interact with them.
Lets delves into API key authorisation in GoFr, explaining its implementation, benefits, and best practices for securing your micro-services.
Implementing API Key Authorisation in GoFr
GoFr offers two approaches for implementing API key authorisation:
- Framework Default Validation: This is the most straightforward way. GoFr provides a function
EnableAPIKeyAuth
that takes a list of valid API keys as arguments. Any request with a matching key in the specified headerx-api-key
will be considered authorised.
Here’s an example:
package main
import (
"github.com/gofr-dev/gofr"
)
func main() {
app := gofr.New()
app.EnableAPIKeyAuth("your_valid_api_key")
app.GET("/protected", func(c *gofr.Context) (interface{}, error) {
return "access granted", nil
})
app.Run()
}
In this example, only requests with the API key “your_valid_api_key” in the “x-api-key” header will be able to access the “/protected” endpoint.
- Custom Validation Function: While convenient, the default approach has limitations. You might want to perform additional validation on the key or retrieve information about the key from a database. GoFr enables this through the
EnableAPIKeyAuthWithFunc
function. You provide a custom function that takes the provided API key as input and returns true if it's valid.
Here’s an example:
package main
import (
"gofr.dev/pkg/gofr"
"slices"
)
func apiKeyValidator(apiKey string) bool {
// Implement your logic to validate the key against a database or other source
validKeys := []string{"your_valid_api_key", "your_special_key"}
return slices.Contains(validKeys, apiKey)
}
func main() {
app := gofr.New()
app.EnableAPIKeyAuthWithFunc(apiKeyValidator)
app.GET("/protected", func(c *gofr.Context) (interface{}, error) {
return "access granted", nil
})
app.Run()
}
This allows for more granular control over API key validation based on your specific requirements.
Best Practices for API Key Authorisation
While API key authorisation offers advantages, it’s crucial to implement it securely:
Rotate Keys Regularly: Don’t use the same API key for extended periods. Regularly rotate keys to minimise the risk of unauthorised access in case a key is compromised.
Limit Scope: Grant API keys access only to the necessary resources and functionalities. This minimises the damage if a key is compromised.
Secure Storage: Store API keys securely, preferably in a dedicated secrets management solution, and avoid hardcoding them in your code.
HTTPS: Always use HTTPS for communication when using API keys. This ensures data is encrypted during transmission, protecting it from eavesdropping.
Conclusion
API key authorisation is a valuable tool for securing access to your GoFr micro-services. By leveraging GoFr’s built-in functionalities and following best practices, you can ensure robust authentication and protect your valuable resources. Remember, security is an ongoing process. Stay vigilant and adapt your approach based on evolving threats and security landscapes.
Happy Coding 🚀
Thank you for reading until the end. Before you go:
Please consider liking and following! 👏
You can also go through my other article on Monitoring GoLang APIs
Subscribe to my newsletter
Read articles from Srijan Rastogi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
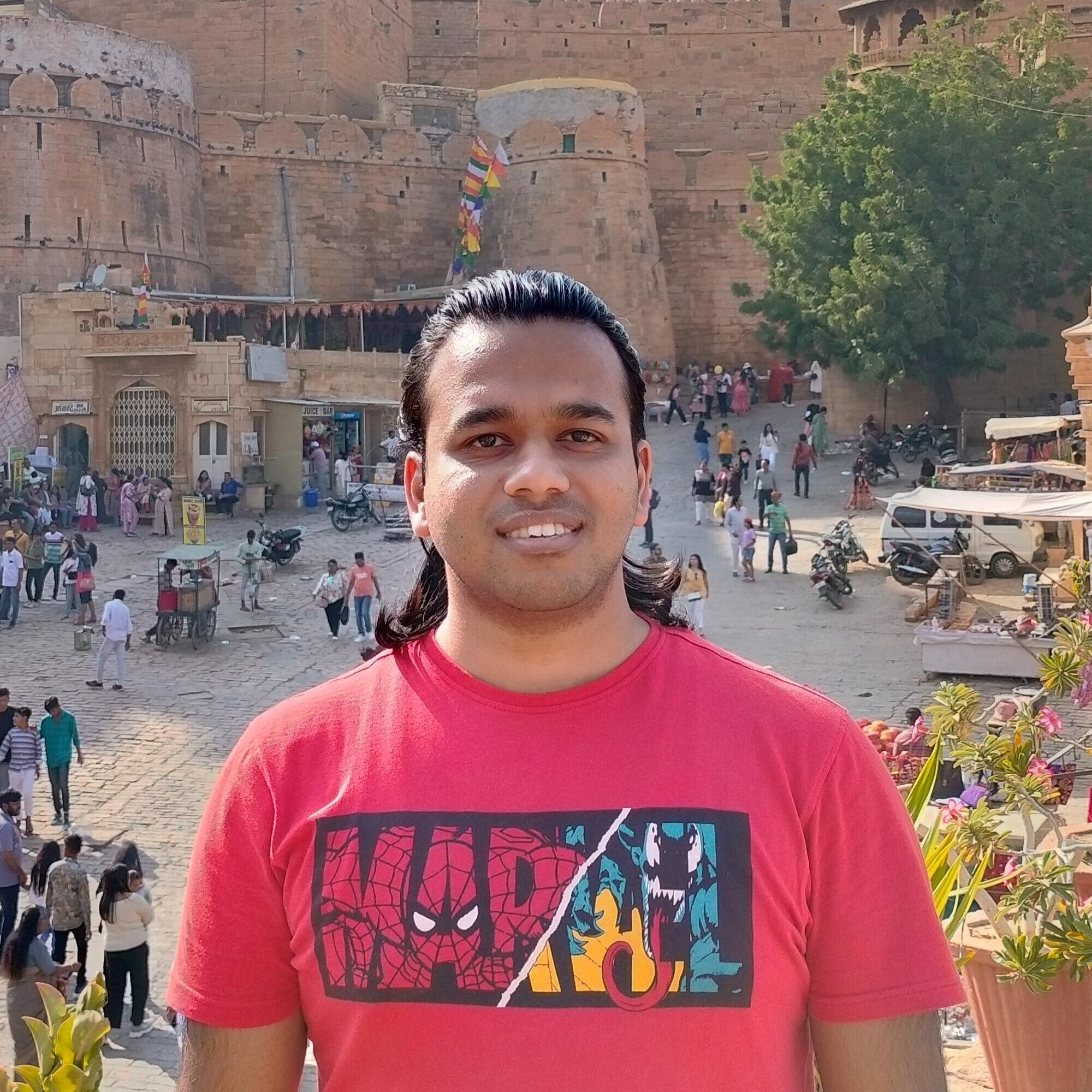
Srijan Rastogi
Srijan Rastogi
GoLang apprentice by day, sensei by night. Learning and building cool stuff, one commit at a time.