Python’s Modules: Creating your Own Module

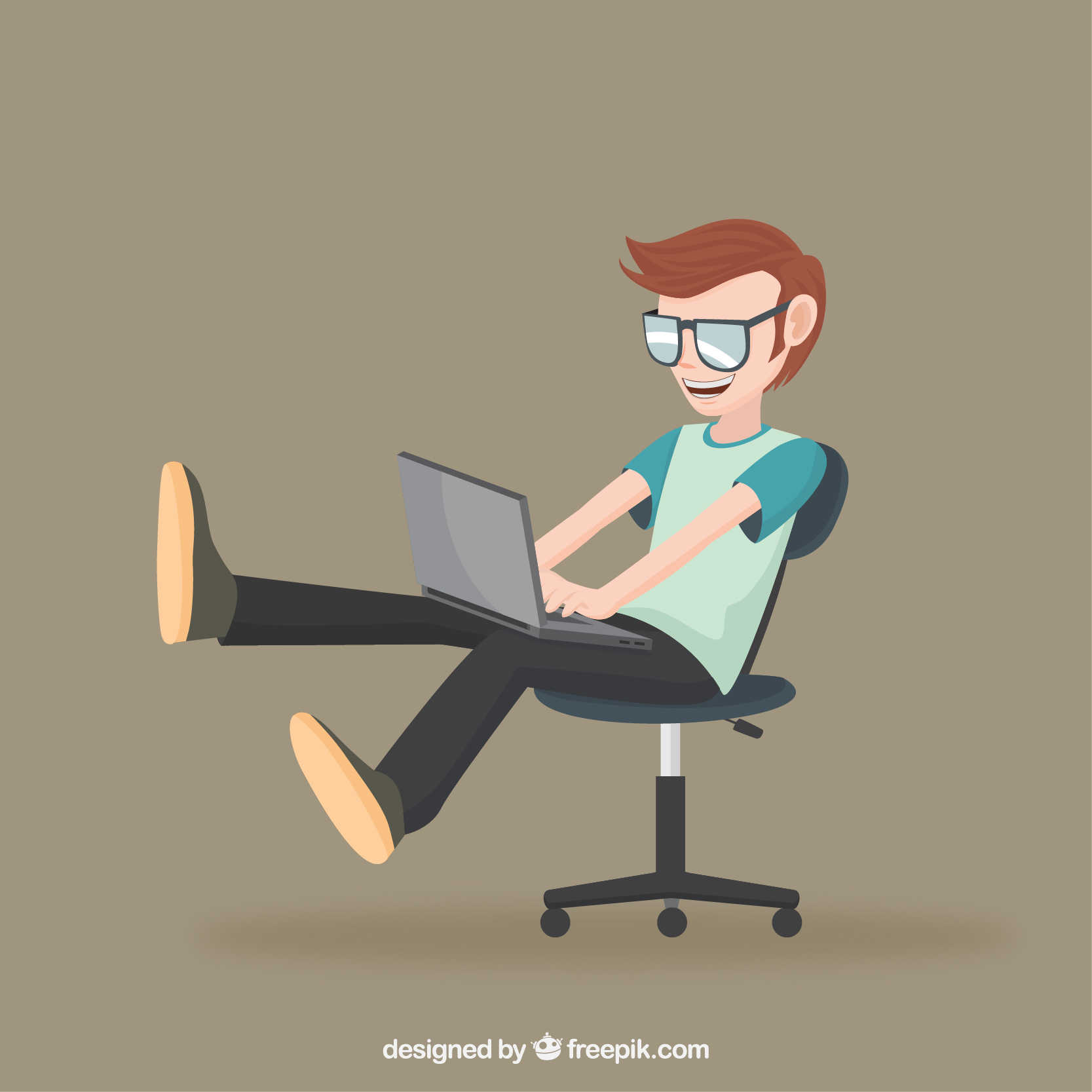
We left off on my last post talking some stuff about creating your own module. That’s worth putting our built-in modules series on hold so you could learn this important feat in preparation for the many modules you may soon write. It’s quite easy really. To create your own module, just create a new python file with variables and functions in it, doing some particular thing, then you import that in another file and use. This is a good summary of the process, but there may be specifications you should probably pay attention to. For instance, let’s work with the code below. It’s our very own math module and while it is definitely not as robust as the built in module, it does a few things for us. You could check out my previous post here if you’re a bit lost on what I’m talking about.
def addNumbers(number1, number2):
result = number1 + number2
return result
def subtractNumbers(number1, number2):
result = number1 - number2
return result
def multiplyNumbers(number1, number2):
result = number1 * number2
return result
A seen above, we have created a simple python file I aptly named ‘mathStuff.’ with only three functions: addNumbers(), subtractNumbers() and multiplyNumbers(). (ps. Always give your functions descriptive names. I had a deplorable habit of never doing that, and many sleepless nights going through code I wrote and not understanding a thing of it has left me repentant).
These three functions add, subtract and multiply numbers respectively. In the same folder, that contains ‘mathStuff’, we create a new file called ‘useMathStuff’ and place the following code.
from mathStuff import addNumbers, subtractNumbers, multiplyNumbers
firstNumber = 36
secondNumber = 12
theSum = addNumbers(firstNumber, secondNumber)
print(theSum)
#You can go ahead and try the other methods
You can see that we have created a module of our own and used it in a different file. That’s awesome but what happens if we want to share this awesome ‘better than the built-in version’ math module with a friend some distance away? Unfortunately, he or she wouldn’t be able to use it automatically as he or she would use the math module since the file is in your present directory, which is in your own computer.
While I can’t help with that (there are ways to share your code with the rest of the world of course but we won't look into them here) or promise your module would make it to the Python standard library, there is a way to make it such that anyone who uses your computer would be able to access and use your module without having to manually look for your file and place it in their own working directory. Below is a step by step guide.
- Figure out where Python checks for modules and packages by running the following snippet. You would find a list of paths.
import sys
print(sys.path)
- Move your module into the Python site packages directory. It may look like this…
'C:\\Python311\\Lib\\site-packages'
And walla! Just like that you can access your awesome module irrespective of what directory you’re in.
In the next post, we would continue our series on built-in modules.
Subscribe to my newsletter
Read articles from Amanda Ene Adoyi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Amanda Ene Adoyi
Amanda Ene Adoyi
I am a Software Developer from Nigeria. I love learning about new technologies and talking about coding. I talk about coding so much that I genuinely think I could explode if I don't . I have been freelance writing for years and now I do the two things I love the most: writing and talking about tech.