How to Create an Animated YOU Loader with HTML and CSS
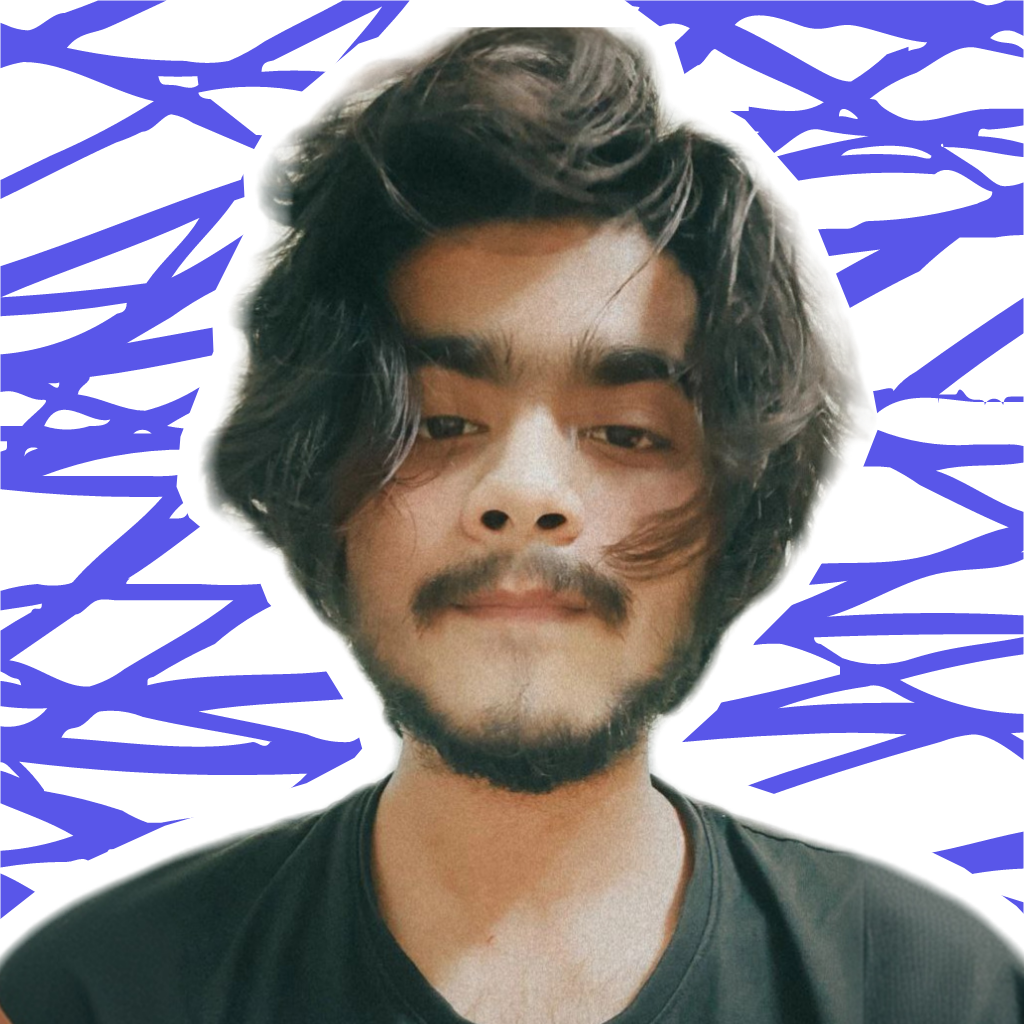
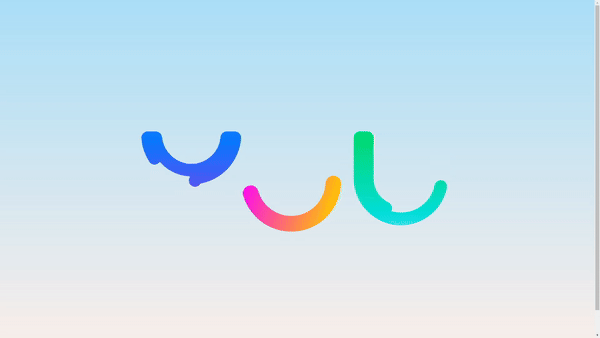
Introduction
Creating an animated loader can add a touch of elegance to your website, enhancing user experience while content loads. In this guide, we'll walk you through creating an engaging animated loader using HTML and CSS. This project is part of the Day 45 challenge of the #100DaysOfCode initiative.
Understanding the Project
Day 45 of #100DaysOfCode Challenge
This animated loader is a creative project aimed at improving your skills in HTML, CSS, and SVG animations. It's a part of the #100DaysOfCode challenge, which encourages developers to code daily for 100 days.
Setting Up the HTML Structure
To start, let's set up a basic HTML structure.
HTML Boilerplate
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>Animated Loader</title>
</head>
<body>
<!-- Loader container -->
<div class="loader">
<!-- SVG and loader elements will go here -->
</div>
</body>
</html>
Loader Container
The div
with the class loader
will hold our SVG elements.
Adding SVG for Gradient Definitions
Next, we'll define the gradients for the loader using SVG.
Creating the SVG Element
<svg height="0" width="0" viewBox="0 0 64 64" class="absolute">
<!-- Gradient definitions will go here -->
</svg>
Defining the Gradients
We'll define three linear gradients for our loader:
<defs>
<linearGradient id="b" gradientUnits="userSpaceOnUse" y2="2" x2="0" y1="62" x1="0">
<stop stop-color="#973BED"></stop>
<stop stop-color="#007CFF" offset="1"></stop>
</linearGradient>
<linearGradient id="c" gradientUnits="userSpaceOnUse" y2="0" x2="0" y1="64" x1="0">
<stop stop-color="#FFC800"></stop>
<stop stop-color="#F0F" offset="1"></stop>
<animateTransform
attributeName="gradientTransform"
type="rotate"
values="0 32 32;-270 32 32;-270 32 32;-540 32 32;-540 32 32;-810 32 32;-810 32 32;-1080 32 32;-1080 32 32"
dur="8s"
repeatCount="indefinite"
keySplines=".42,0,.58,1;.42,0,.58,1;.42,0,.58,1;.42,0,.58,1;.42,0,.58,1;.42,0,.58,1;.42,0,.58,1;.42,0,.58,1"
keyTimes="0;0.125;0.25;0.375;0.5;0.625;0.75;0.875;1">
</animateTransform>
</linearGradient>
<linearGradient id="d" gradientUnits="userSpaceOnUse" y2="2" x2="0" y1="62" x1="0">
<stop stop-color="#00E0ED"></stop>
<stop stop-color="#00DA72" offset="1"></stop>
</linearGradient>
</defs>
SVG Elements for Loader Parts
We'll create SVG elements for different parts of the loader.
First Part of the Loader
<svg xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 64 64" height="64" width="64" class="inline-block">
<path stroke-linejoin="round" stroke-linecap="round" stroke-width="8" stroke="url(#b)"
d="M 54.722656,3.9726563 A 2.0002,2.0002 0 0 0 54.941406,4 h 5.007813 C 58.955121,17.046124 49.099667,27.677057 36.121094,29.580078 a 2.0002,2.0002 0 0 0 -1.708985,1.978516 V 60 H 29.587891 V 31.558594 A 2.0002,2.0002 0 0 0 27.878906,29.580078 C 14.900333,27.677057 5.0448787,17.046124 4.0507812,4 H 9.28125 c 1.231666,11.63657 10.984383,20.554048 22.6875,20.734375 a 2.0002,2.0002 0 0 0 0.02344,0 c 11.806958,0.04283 21.70649,-9.003371 22.730469,-20.7617187 z"
class="dash" id="y" pathLength="360"></path>
</svg>
Rotating Part of the Loader
<svg xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 64 64" height="64" width="64" class="inline-block">
<path stroke-linejoin="round" stroke-linecap="round" stroke-width="10" stroke="url(#c)"
d="M 32 32 m 0 -27 a 27 27 0 1 1 0 54 a 27 27 0 1 1 0 -54" class="spin" id="o" pathLength="360"></path>
</svg>
Second Part of the Loader
<svg xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 64 64" height="64" width="64" class="inline-block">
<path stroke-linejoin="round" stroke-linecap="round" stroke-width="8" stroke="url(#d)"
d="M 4,4 h 4.6230469 v 25.919922 c -0.00276,11.916203 9.8364941,21.550422 21.7500001,21.296875 11.616666,-0.240651 21.014356,-9.63894 21.253906,-21.25586 a 2.0002,2.0002 0 0 0 0,-0.04102 V 4 H 56.25 v 25.919922 c 0,14.33873 -11.581192,25.919922 -25.919922,25.919922 a 2.0002,2.0002 0 0 0 -0.0293,0 C 15.812309,56.052941 3.998433,44.409961 4,29.919922 Z"
class="dash" id="u" pathLength="360"></path>
</svg>
Styling with CSS
Let's style our loader with CSS.
Body Styling
body {
display: flex;
align-items: center;
justify-content: center;
min-height: 100vh;
background-image: linear-gradient(to top, #fff1eb 0%, #ace0f9 100%);
}
Loader Container Styling
.loader {
display: flex;
margin: 0.25em 0;
}
.absolute {
position: absolute;
}
.inline-block {
display: inline-block;
}
.w
-2 {
width: 0.5em;
}
Animating the Loader
Dash Array Animation
.dash {
animation: dashArray 2s ease-in-out infinite, dashOffset 2s linear infinite;
}
Spin Dash Array Animation
.spin {
animation: spinDashArray 2s ease-in-out infinite, spin 8s ease-in-out infinite, dashOffset 2s linear infinite;
transform-origin: center;
}
Keyframe Animations
Dash Array Animation
@keyframes dashArray {
0% {
stroke-dasharray: 0 1 359 0;
}
50% {
stroke-dasharray: 0 359 1 0;
}
100% {
stroke-dasharray: 359 1 0 0;
}
}
Spin Dash Array Animation
@keyframes spinDashArray {
0% {
stroke-dasharray: 270 90;
}
50% {
stroke-dasharray: 0 360;
}
100% {
stroke-dasharray: 270 90;
}
}
Dash Offset Animation
@keyframes dashOffset {
0% {
stroke-dashoffset: 365;
}
100% {
stroke-dashoffset: 5;
}
}
Spin Animation
@keyframes spin {
0% {
rotate: 0deg;
}
12.5%, 25% {
rotate: 270deg;
}
37.5%, 50% {
rotate: 540deg;
}
62.5%, 75% {
rotate: 810deg;
}
87.5%, 100% {
rotate: 1080deg;
}
}
Combining HTML and CSS
By integrating the SVG and CSS, we create a visually appealing and functional loader. Place all the SVG elements and CSS in the respective body
and style.css
sections.
Testing and Debugging
Ensure that the loader works across different devices and browsers. Test for responsiveness and compatibility.
Conclusion
Creating an animated loader with HTML and CSS enhances user experience and demonstrates your ability to create engaging web elements. This project, part of the #100DaysOfCode challenge, showcases your skills and creativity.
FAQs
What is the purpose of an animated loader? An animated loader keeps users engaged while the content of your website loads, improving the overall user experience.
Why use SVG for animations? SVGs provide scalable and high-quality graphics, making them ideal for web animations.
Can I customize the colors of the loader? Yes, you can customize the colors by modifying the gradient definitions in the SVG.
Is this loader responsive? Yes, the loader is designed to be responsive and works well across different screen sizes.
How can I integrate this loader into my existing project? Simply include the HTML and CSS code in your project files, ensuring the paths to the CSS and SVG elements are correct.
Subscribe to my newsletter
Read articles from Aarzoo Islam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
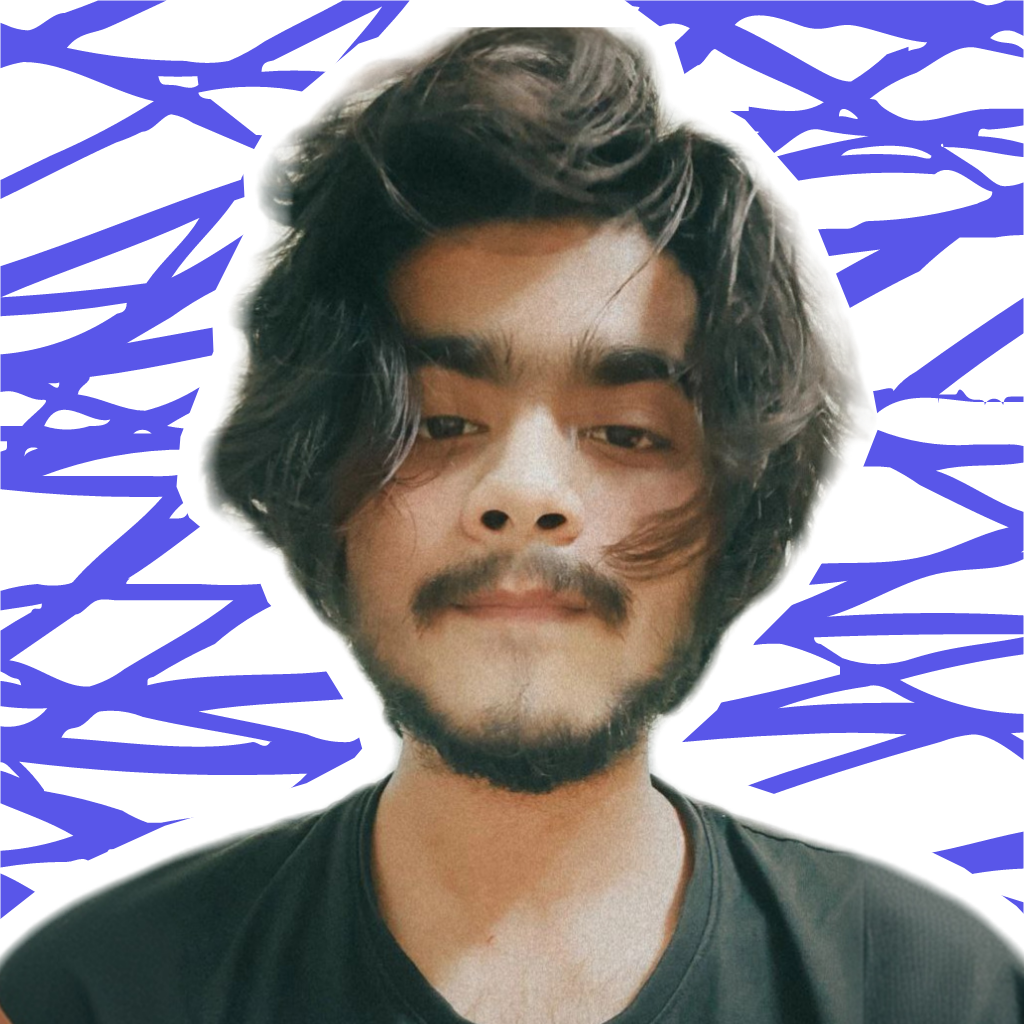
Aarzoo Islam
Aarzoo Islam
Founder of AroNus ๐ | Full-stack web developer ๐จ๐ปโ๐ป | Sharing web development tips and showcasing projects ๐ | Transforming ideas into digital realities ๐