Internationalization in Next.js: Building Multilingual Apps
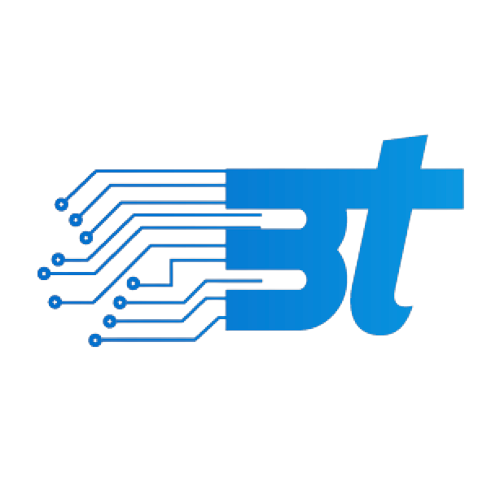
Table of contents
- Why Internationalization Matters
- Setting Up Internationalization in Next.js
- Step 1: Configure Next.js for Internationalization
- Step 2: Create Locale-Specific Content
- Step 3: Install and Set Up a Translation Library
- Step 4: Use Translations in Your Components
- Step 5: Implement Locale Switching
- Step 6: SEO Considerations
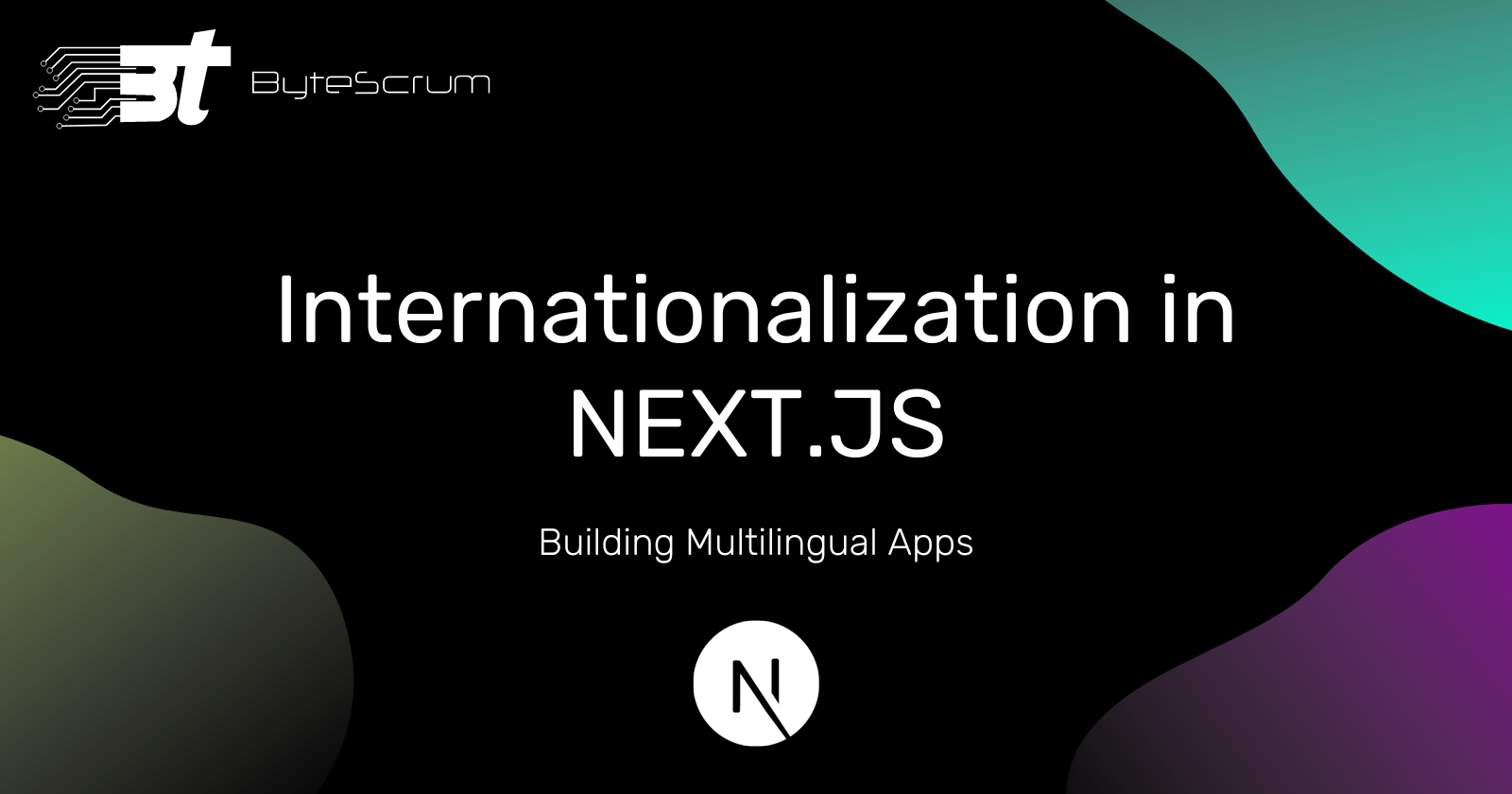
In today's globalized world, offering your application in multiple languages can significantly broaden your audience. Next.js provides robust support for internationalization (i18n), enabling you to create multilingual apps seamlessly. This blog will guide you through the process of adding internationalization to your Next.js application.
Why Internationalization Matters
Internationalization allows your application to be easily adapted to various languages and regions without requiring significant changes to the codebase. It enhances user experience, increases accessibility, and can potentially boost your app's reach and engagement.
Setting Up Internationalization in Next.js
Next.js has built-in support for internationalized routing, making it straightforward to manage multiple languages. Let's walk through the steps to set up internationalization in a Next.js app.
Step 1: Configure Next.js for Internationalization
First, you need to update your next.config.js
file to specify the locales your application will support.
// next.config.js
module.exports = {
i18n: {
locales: ['en', 'fr', 'es'],
defaultLocale: 'en',
},
};
locales
: An array of supported locales.defaultLocale
: The default locale for the application.
Step 2: Create Locale-Specific Content
Create a folder structure for storing your locale-specific content. A common approach is to use JSON files to store translations.
/locales
/en
common.json
/fr
common.json
/es
common.json
Example common.json
for English:
{
"greeting": "Hello",
"welcome": "Welcome to our application"
}
Example common.json
for French:
{
"greeting": "Bonjour",
"welcome": "Bienvenue dans notre application"
}
Step 3: Install and Set Up a Translation Library
You need a translation library to load and manage your translations. next-translate
is a popular choice for Next.js applications.
Install next-translate
:
npm install next-translate
Create a i18n.js
configuration file in the root of your project:
// i18n.js
module.exports = {
locales: ['en', 'fr', 'es'],
defaultLocale: 'en',
pages: {
'*': ['common'],
},
};
Step 4: Use Translations in Your Components
To use translations in your components, you can use the useTranslation
hook provided by next-translate
.
Example component:
// components/Greeting.js
import useTranslation from 'next-translate/useTranslation';
const Greeting = () => {
const { t } = useTranslation('common');
return <h1>{t('greeting')}</h1>;
};
export default Greeting;
Use the Greeting
component in your pages:
// pages/index.js
import Greeting from '../components/Greeting';
const Home = () => {
return (
<div>
<Greeting />
</div>
);
};
export default Home;
Step 5: Implement Locale Switching
To allow users to switch between languages, you can create a simple language switcher component.
Example LanguageSwitcher
component:
// components/LanguageSwitcher.js
import { useRouter } from 'next/router';
const LanguageSwitcher = () => {
const router = useRouter();
const { locale, locales } = router;
const handleChangeLanguage = (e) => {
const newLocale = e.target.value;
const path = router.asPath;
router.push(path, path, { locale: newLocale });
};
return (
<select value={locale} onChange={handleChangeLanguage}>
{locales.map((loc) => (
<option key={loc} value={loc}>
{loc}
</option>
))}
</select>
);
};
export default LanguageSwitcher;
Use the LanguageSwitcher
component in your pages:
// pages/index.js
import Greeting from '../components/Greeting';
import LanguageSwitcher from '../components/LanguageSwitcher';
const Home = () => {
return (
<div>
<LanguageSwitcher />
<Greeting />
</div>
);
};
export default Home;
Step 6: SEO Considerations
To improve SEO for your multilingual site, ensure each language version of your pages is correctly indexed by search engines. Use the next/head
component to set the hreflang
attributes.
Example:
import Head from 'next/head';
import { useRouter } from 'next/router';
const Home = () => {
const { locales, asPath } = useRouter();
const siteUrl = 'https://www.yourwebsite.com';
return (
<>
<Head>
{locales.map((locale) => (
<link
key={locale}
rel="alternate"
hrefLang={locale}
href={`${siteUrl}/${locale}${asPath}`}
/>
))}
</Head>
<div>
<LanguageSwitcher />
<Greeting />
</div>
</>
);
};
export default Home;
Conclusion
next-translate
, you can easily manage multiple languages and optimize your app for global users. Follow these steps to set up internationalization in your Next.js app, and enjoy the benefits of a multilingual application.Explore the official Next.js internationalization documentation for more details and advanced configurations.
Happy coding!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
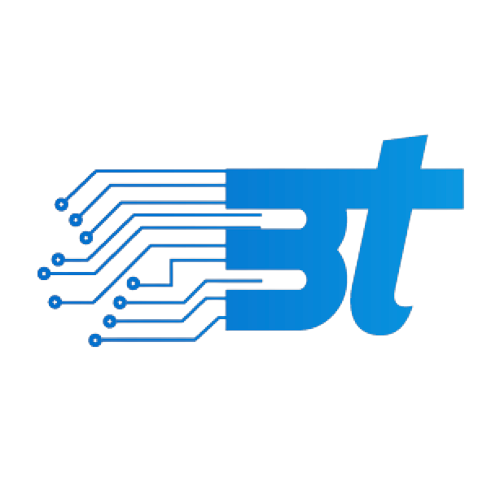
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.