Basic Of JavaScript:
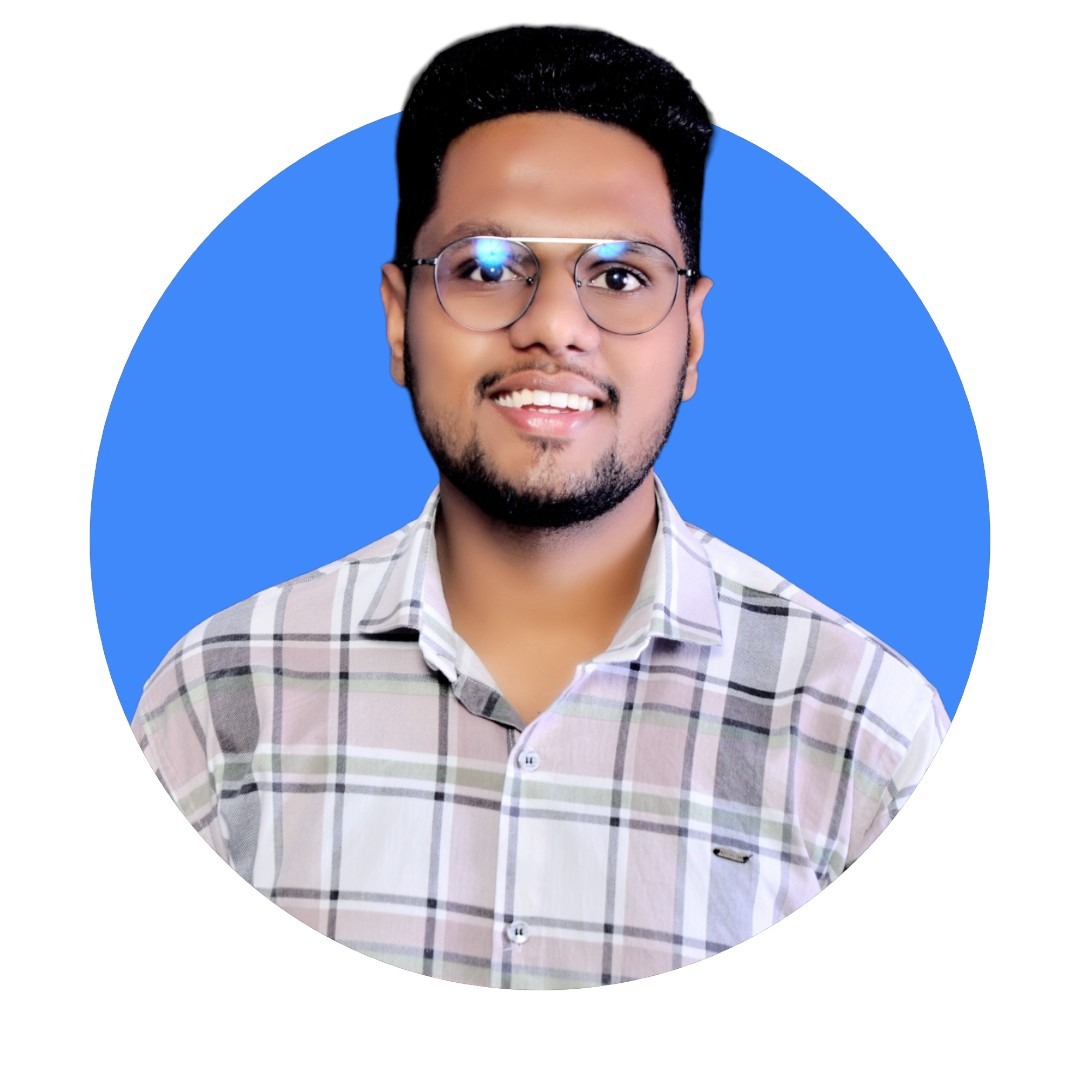
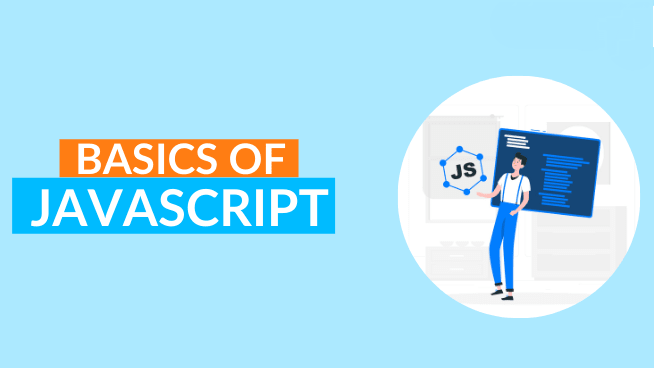
JavaScript Comment :
The JavaScript comments are meaningful way to deliver message.
It is used to add information about the code, warnings or suggestions so that end user can easily interpret the code.
The JavaScript comment is ignored by the JavaScript engine i.e. embedded in the browser.
Advantages of JavaScript comments :
There are mainly two advantages of JavaScript comments.
To make code easy to understand It can be used to elaborate the code so that end user can easily understand the code.
To avoid the unnecessary code It can also be used to avoid the code being executed. Sometimes, we add the code to perform some action. But after sometime, there may be need to disable the code. In such case, it is better to use comments.
JavaScript Variable :
A JavaScript variable is simply a name of storage location.
There are two types of variables in JavaScript : local variable and global variable.
There are some rules while declaring a JavaScript variable :
Name must start with a letter (a to z or A to Z), underscore( _ ), or dollar( $ ) sign.
After first letter we can use digits (0 to 9), for example value1.
JavaScript variables are case sensitive, for example x and X are different variables.
Correct JavaScript variables :
var x = 10;
var _value="sonoo";
Incorrect JavaScript variables :
var 123=30;
var *aa=320;
Example of JavaScript variable :
Let’s see a simple example of JavaScript variable.
<html>
<body>
<script>
var x = 10;
var y = 20;
var z=x+y;
document.write(z);
</script>
</body>
</html>
Output :
JavaScript local variable :
A JavaScript local variable is declared inside block or function.
It is accessible within the function or block only.
Example:
<script>
function abc(){
var x=10;//local variable
}
</script>
Or
<script>
If(10<13){
var y=20;//JavaScript local variable
}
</script>
JavaScript global variable :
A JavaScript global variable is accessible from any function.
A variable i.e. declared outside the function or declared with window object is known as global variable.
For example:
<html>
<body>
<script>
var data=200;//gloabal variable
function a(){
document.writeln(data);
}
function b(){
document.writeln(data);
}
a();//calling JavaScript function
b();
</script>
</body>
</html>
Output:
Javascript Data Types :
JavaScript provides different data types to hold different types of values.
There are two types of data types in JavaScript.
Primitive data type
Non-primitive data type
JavaScript is a dynamic type language, means you don't need to specify type of the variable because it is dynamically used by JavaScript engine.
You need to use var here to specify the data type.
It can hold any type of values such as numbers, strings etc.
For Example:
var a=40;//holding number
var b="Rahul";//holding string
JavaScript primitive data types :
There are five types of primitive data types in JavaScript. They are as follows:
Data Type | Description |
String | represents sequence of characters e.g. "hello" |
Number | represents numeric values e.g. 100 |
Boolean | represents boolean value either false or true |
Undefined | represents undefined value |
Null | represents null i.e. no value at all |
JavaScript non-primitive data types :
The non-primitive data types are as follows:
Data Type | Description |
Object | represents instance through which we can access members |
Array | represents group of similar values |
RegExp | represents regular expression |
JavaScript Operators :
JavaScript operators are symbols that are used to perform operations on operands.
For example:
- var sum=10+20;
Here, + is the arithmetic operator and = is the assignment operator.
There are following types of operators in JavaScript.
Arithmetic Operators
Comparison (Relational) Operators
Bitwise Operators
Logical Operators
Assignment Operators
Special Operators
JavaScript Arithmetic Operators :
Arithmetic operators are used to perform arithmetic operations on the operands. The following operators are known as JavaScript arithmetic operators.
Operator | Description | Example |
+ | Addition | 10+20 = 30 |
- | Subtraction | 20-10 = 10 |
* | Multiplication | 10*20 = 200 |
/ | Division | 20/10 = 2 |
% | Modulus (Remainder) | 20%10 = 0 |
++ | Increment | var a=10; a++; Now a = 11 |
-- | Decrement | var a=10; a--; Now a = 9 |
JavaScript Comparison Operators :
The JavaScript comparison operator compares the two operands. The comparison operators are as follows:
Operator | Description | Example |
\== | Is equal to | 10==20 = false |
\=== | Identical (equal and of same type) | 10==20 = false |
!= | Not equal to | 10!=20 = true |
!== | Not Identical | 20!==20 = false |
\> | Greater than | 20>10 = true |
\>= | Greater than or equal to | 20>=10 = true |
< | Less than | 20<10 = false |
<= | Less than or equal to | 20<=10 = false |
JavaScript Bitwise Operators :
The bitwise operators perform bitwise operations on operands. The bitwise operators are as follows:
Operator | Description | Example | ||
& | Bitwise AND | (10==20 & 20==33) = false | ||
Bitwise OR | (10==20 | 20==33) = false | ||
^ | Bitwise XOR | (10==20 ^ 20==33) = false | ||
~ | Bitwise NOT | (~10) = -10 | ||
<< | Bitwise Left Shift | (10<<2) = 40 | ||
\>> | Bitwise Right Shift | (10>>2) = 2 | ||
\>>> | Bitwise Right Shift with Zero | (10>>>2) = 2 |
JavaScript Logical Operators :
The following operators are known as JavaScript logical operators.
Operator | Description | Example | ||||
&& | Logical AND | (10==20 && 20==33) = false | ||||
Logical OR | (10==20 | 20==33) = false | ||||
! | Logical Not | !(10==20) = true |
JavaScript Assignment Operators :
The following operators are known as JavaScript assignment operators.
Operator | Description | Example |
\= | Assign | 10+10 = 20 |
+= | Add and assign | var a=10; a+=20; Now a = 30 |
-= | Subtract and assign | var a=20; a-=10; Now a = 10 |
*= | Multiply and assign | var a=10; a*=20; Now a = 200 |
/= | Divide and assign | var a=10; a/=2; Now a = 5 |
%= | Modulus and assign | var a=10; a%=2; Now a = 0 |
JavaScript Special Operators :
The following operators are known as JavaScript special operators.
Operator | Description |
(?:) | Conditional Operator returns value based on the condition. It is like if-else. |
, | Comma Operator allows multiple expressions to be evaluated as single statement. |
delete | Delete Operator deletes a property from the object. |
in | In Operator checks if object has the given property |
instanceof | checks if the object is an instance of given type |
new | creates an instance (object) |
typeof | checks the type of object. |
void | it discards the expression's return value. |
yield | checks what is returned in a generator by the generator's iterator. |
What Next ?
Javascript Conditional and looping statements.
Subscribe to my newsletter
Read articles from Aditya Gadhave directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
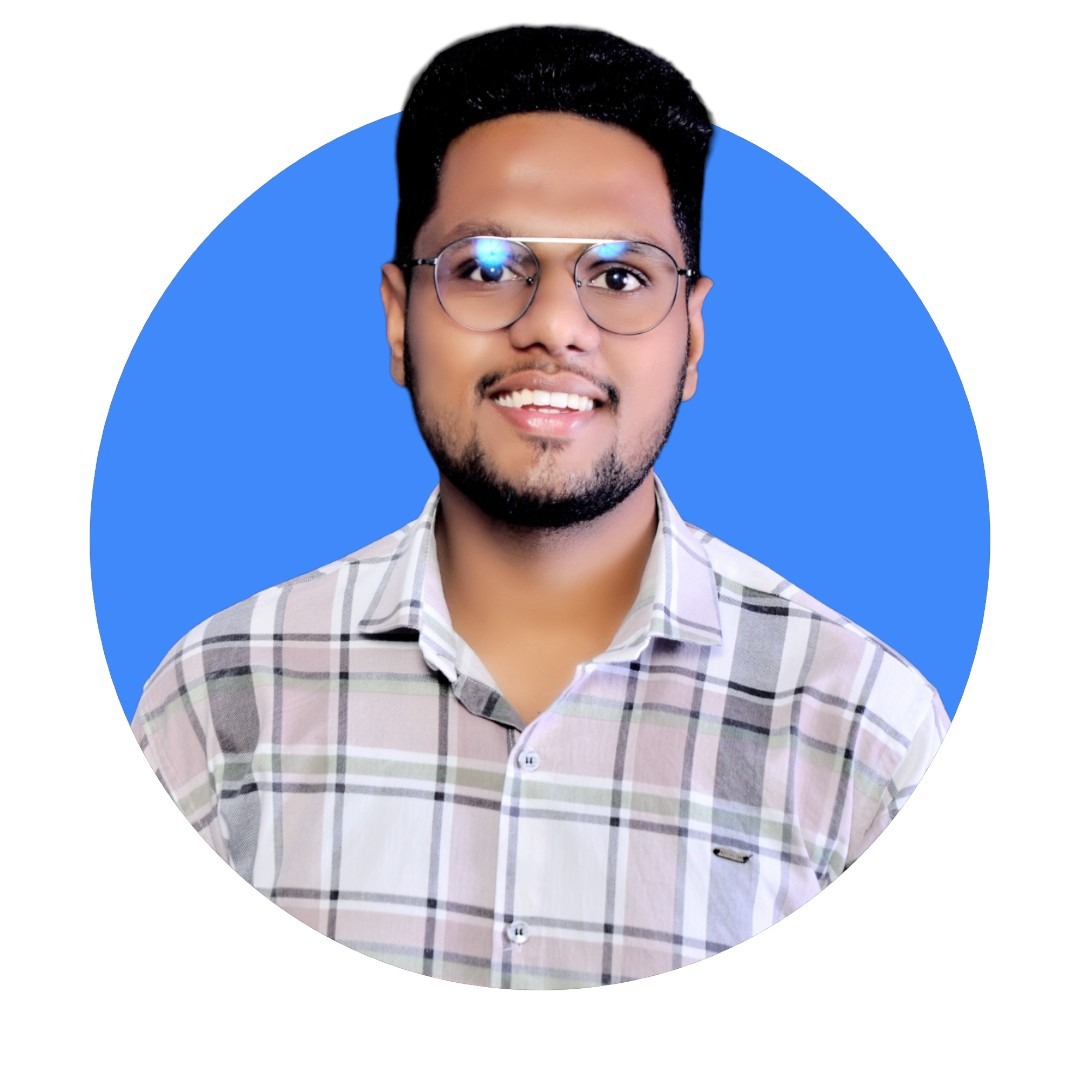
Aditya Gadhave
Aditya Gadhave
👋 Hello! I'm Aditya Gadhave, an enthusiastic Computer Engineering Undergraduate Student. My passion for technology has led me on an exciting journey where I'm honing my skills and making meaningful contributions.