IOS Platform Actual Implementation
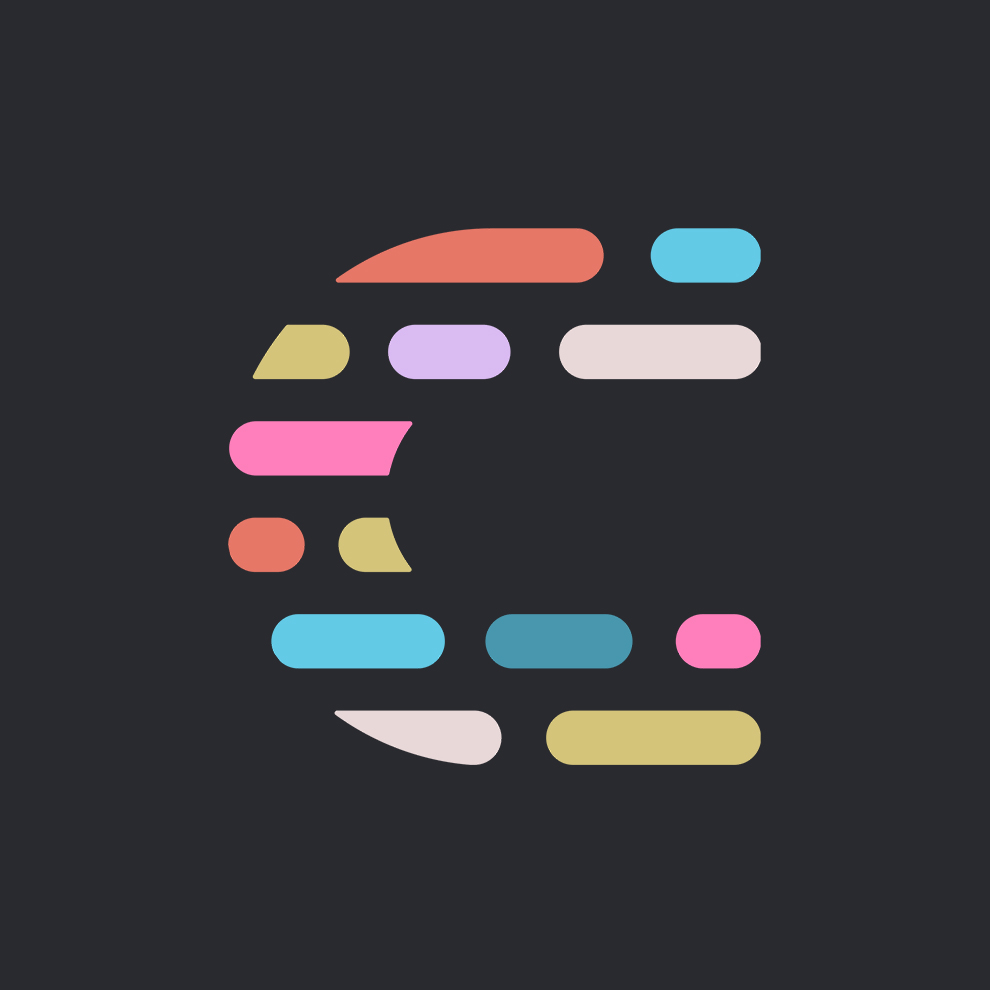
In today’s mobile development landscape, Kotlin Multiplatform (KMP) is a powerful tool that allows developers to write shared code for both Android and iOS platforms. This approach significantly reduces code duplication, ensures consistency, and speeds up the development process. This blog explores the actual implementation of KMP for iOS, focusing on practical steps, best practices, and insights from real-world applications.
Understanding Kotlin Multiplatform Project Structure
A Kotlin Multiplatform project typically consists of three main components:
Shared Module:
CommonMain: Contains platform-independent business logic and shared code.
CommonTest: Houses tests for the shared code.
Platform-Specific Modules:
AndroidMain: Android-specific code, leveraging Kotlin/JVM.
iOSMain: iOS-specific code, utilizing Kotlin/Native.
Each module is meticulously structured to facilitate seamless integration and maintainability across platforms.
Steps to Implement KMP in an iOS Application
1. Set Up Your Development Environment
To get started, ensure you have the following tools installed:
Android Studio: For Android development.
Xcode: For iOS development.
Kotlin Plugin: Integrated into Android Studio for KMP support.
2. Create a New Multiplatform Project
Use the Kotlin Multiplatform project wizard in Android Studio to create a new project. This sets up the necessary modules and dependencies.
3. Configure Shared Module
Define your shared code in the commonMain
source set. Use the expect/actual mechanism to handle platform-specific implementations.
// commonMain
expect fun getPlatformName(): String
// androidMain
actual fun getPlatformName(): String {
return "Android"
}
// iosMain
actual fun getPlatformName(): String {
return "iOS"
}
4. Add Dependencies
Manage dependencies using Gradle. Declare common dependencies in the shared module and platform-specific dependencies in their respective modules.
// shared build.gradle.kts
kotlin {
sourceSets {
val commonMain by getting {
dependencies {
implementation("org.jetbrains.kotlinx:kotlinx-coroutines-core:1.5.0")
}
}
}
}
5. Writing Shared Business Logic
Leverage Kotlin's powerful features to write shared business logic. This could include data models, network requests, and utility functions.
// commonMain
class Greeting {
fun greet(): String {
return "Hello, ${getPlatformName()}"
}
}
6. Platform-Specific Implementations
Implement platform-specific code in androidMain
and iosMain
. Use actual declarations to fulfill expect declarations from the shared module.
// iOS specific implementation in Swift
actual fun getPlatformName(): String {
return UIDevice.current.systemName
}
7. Testing
Ensure your shared code works correctly by writing tests in the commonTest
source set. Use platform-specific tests for any native code.
// commonTest
class GreetingTest {
@Test
fun testGreeting() {
assertEquals("Hello, iOS", Greeting().greet())
}
}
8. Running the Application
Configure your run configurations for both Android and iOS. For Android, use the standard Gradle tasks. For iOS, use Xcode to build and run the application on a simulator or a real device.
Advantages of Using KMP
Code Reusability: Write once, use everywhere.
Consistency: Shared business logic ensures uniform behavior across platforms.
Reduced Development Time: Faster implementation with fewer lines of code.
Maintainability: Easier to maintain and update shared logic.
Real-World Insights and Best Practices
Start Small: Begin with a small, non-critical part of your app to understand the intricacies of KMP.
Gradual Integration: Gradually move more business logic to the shared module as you gain confidence.
Leverage Community Libraries: Use existing KMP libraries to avoid reinventing the wheel.
Regular Testing: Ensure consistent behavior across platforms by running regular tests.
Conclusion
Developing iOS using Kotlin Multiplatform is a strategic move that offers numerous benefits. By following the steps outlined above, you can leverage shared code to build efficient, consistent, and maintainable applications for both platforms. As the KMP ecosystem continues to evolve, staying updated with the latest practices and tools will help you maximize its potential.
Subscribe to my newsletter
Read articles from Codefy Labs directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
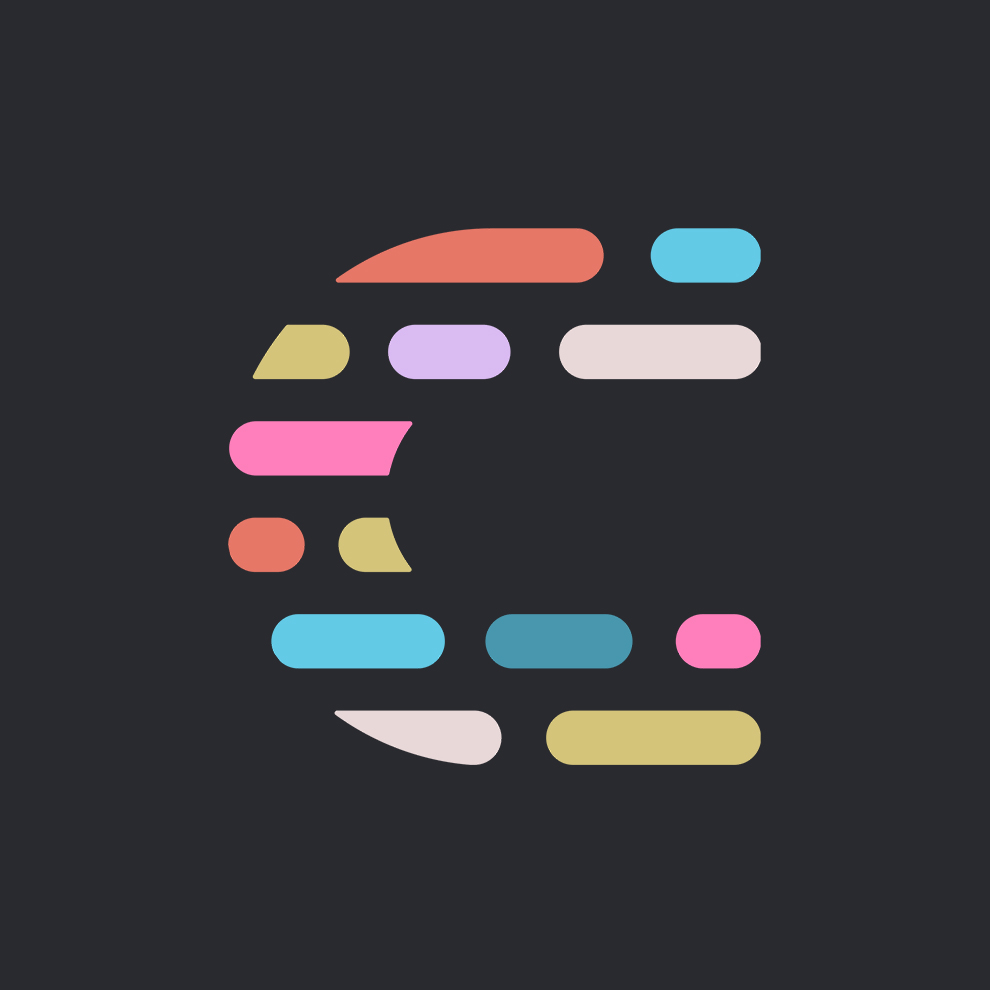
Codefy Labs
Codefy Labs
We build products that scale