Understanding Service Workers in JavaScript: Enhancing Web Performance and Offline Capabilities
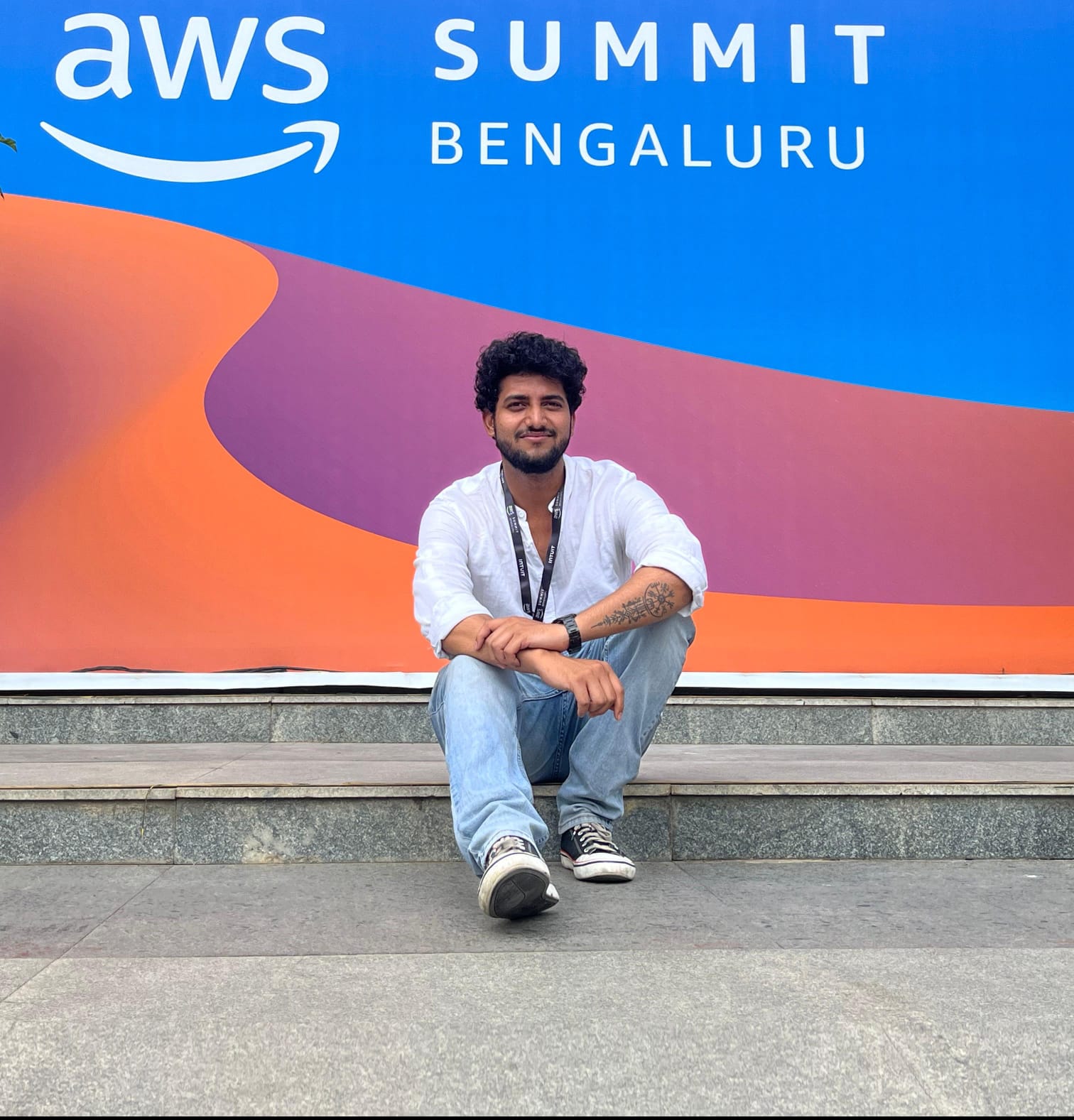
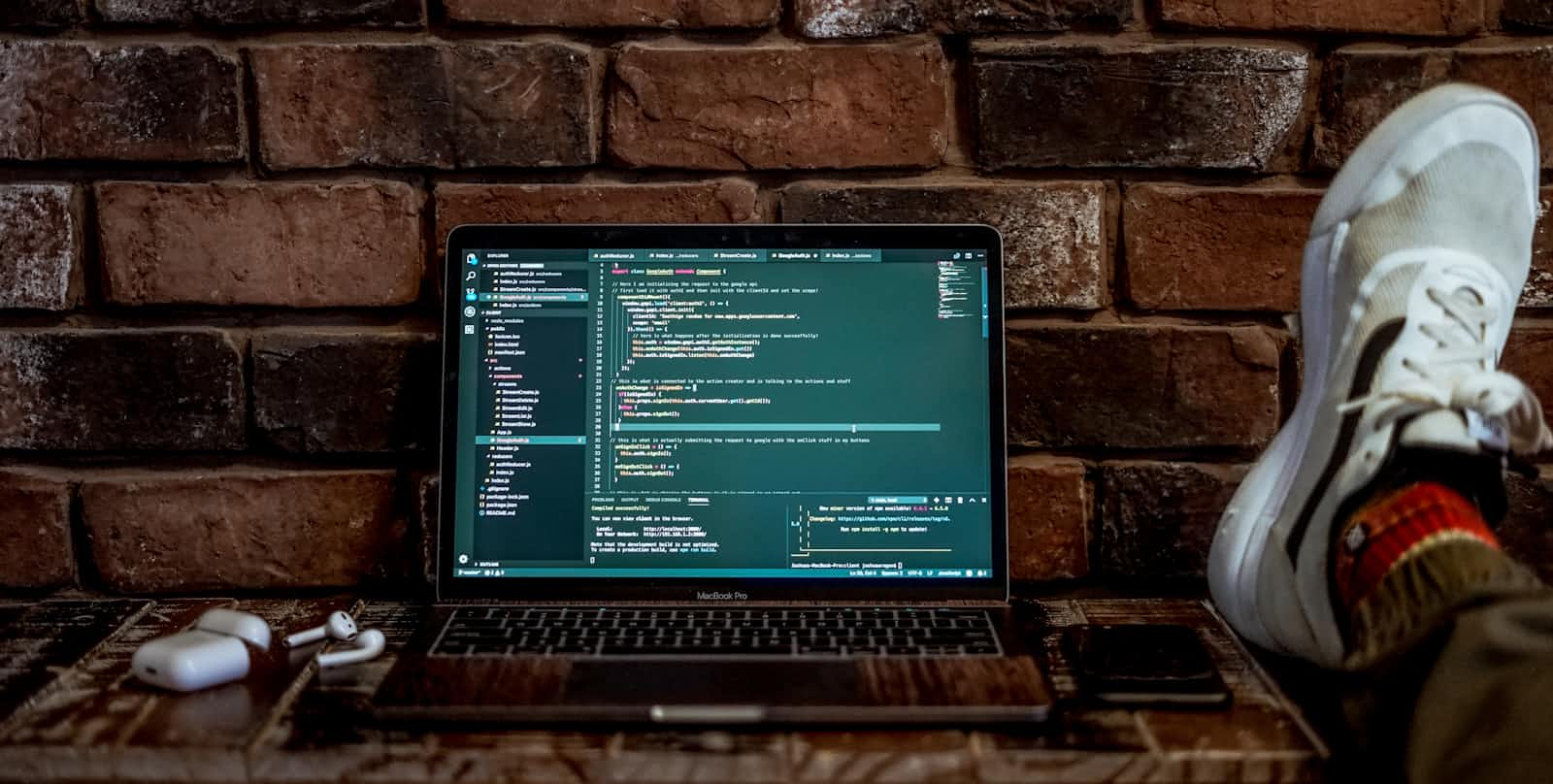
Service workers have revolutionised web development by enabling features like offline support, push notifications, and background syncing. This blog aims to demystify service workers in JavaScript, covering their functionality, implementation, and benefits.
Introduction to Service Workers
Service workers are scripts that run in the background, separate from the main browser thread. They act as a network proxy, intercepting network requests and caching or fetching resources as needed. This capability allows for improved performance and offline functionality.
What are Service Workers?
A service worker is a programmable network proxy that gives developers control over how network requests are handled. It sits between the web application and the network, enabling advanced caching strategies and offline capabilities.
Key Features:
Offline Support: Ensures the application works even without an internet connection.
Push Notifications: Allows sending notifications to users even when the app is not open.
Background Sync: Synchronises data in the background when the user is offline.
How Service Workers Work
Service workers follow a well-defined lifecycle with distinct phases: installation, activation, and fetch events. Let's explore each phase in detail.
Installation Phase:
During the installation phase, the service worker is downloaded and installed. This is an ideal time to cache necessary assets for offline use.
self.addEventListener('install', event => {
event.waitUntil(
caches.open('v1').then(cache => {
return cache.addAll([
'/',
'/styles/main.css',
'/scripts/main.js'
]);
})
);
});
Activation Phase:
Once installed, the service worker moves to the activation phase. Here, it cleans up old caches if needed and prepares to control the web pages.
self.addEventListener('activate', event => {
var cacheWhitelist = ['v1'];
event.waitUntil(
caches.keys().then(keyList => {
return Promise.all(keyList.map(key => {
if (cacheWhitelist.indexOf(key) === -1) {
return caches.delete(key);
}
}));
})
);
});
Fetch Event:
The fetch event is triggered for every network request. The service worker can intercept these requests, serving cached responses or fetching resources from the network.
self.addEventListener('fetch', event => {
event.respondWith(
caches.match(event.request).then(response => {
return response || fetch(event.request);
})
);
});
Implementing Service Workers
Implementing service workers involves several steps, from registering the service worker to defining caching strategies. Here's a step-by-step guide:
Step 1: Registering the Service Worker
To start using a service worker, register it in your main JavaScript file.
if ('serviceWorker' in navigator) {
window.addEventListener('load', () => {
navigator.serviceWorker.register('/service-worker.js').then(registration => {
console.log('Service Worker registered with scope:', registration.scope);
}).catch(error => {
console.log('Service Worker registration failed:', error);
});
});
}
Step 2: Defining the Service Worker
Create a file named service-worker.js
and define the service worker lifecycle events and caching strategies.
Step 3: Caching Strategies
Caching strategies determine how the service worker handles network requests and cached resources. Common strategies include:
Cache First: Serve cached resources if available, falling back to the network if not.
Network First: Try fetching from the network first, falling back to the cache if offline.
Cache Only: Serve only cached resources.
Network Only: Always fetch from the network.
self.addEventListener('fetch', event => {
event.respondWith(
caches.match(event.request).then(response => {
// If the resource is in the cache, return it
if (response) {
return response;
}
// If the resource is not in the cache, fetch it from the network
return fetch(event.request).then(networkResponse => {
// Cache the newly fetched resource
return caches.open('v1').then(cache => {
cache.put(event.request, networkResponse.clone());
return networkResponse;
});
});
})
);
});
other strategies can be implemented in the same way, above example is for cache first strategy.
Benefits of Using Service Workers
Improved Performance: Service workers can cache assets, reducing load times and enhancing user experience. By serving cached content, they reduce the need for repeated network requests.
Offline Capabilities: One of the significant advantages is the ability to provide offline functionality. Users can continue interacting with the app even without an internet connection.
Push Notifications: Service workers enable push notifications, allowing apps to engage users with timely updates and information, even when the app isn't actively open.
Background Sync: Background sync lets the app synchronise data in the background when the user is offline, ensuring that data is updated as soon as the connection is restored.
Common Use Cases for Service Workers
Progressive Web Apps (PWAs): Service workers are a core technology in PWAs, providing offline support, push notifications, and background sync.
Performance Optimization: Websites with heavy resources like images and scripts benefit from caching strategies to enhance load times and reduce bandwidth usage.
Reliable User Experience: Applications that need to provide a reliable experience despite network conditions use service workers to ensure functionality.
Conclusion
Service workers in JavaScript are a powerful tool for enhancing web performance and providing offline capabilities. By understanding their lifecycle, implementation, and best practices, developers can create robust, efficient web applications that deliver a seamless user experience.
Subscribe to my newsletter
Read articles from Brijesh Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
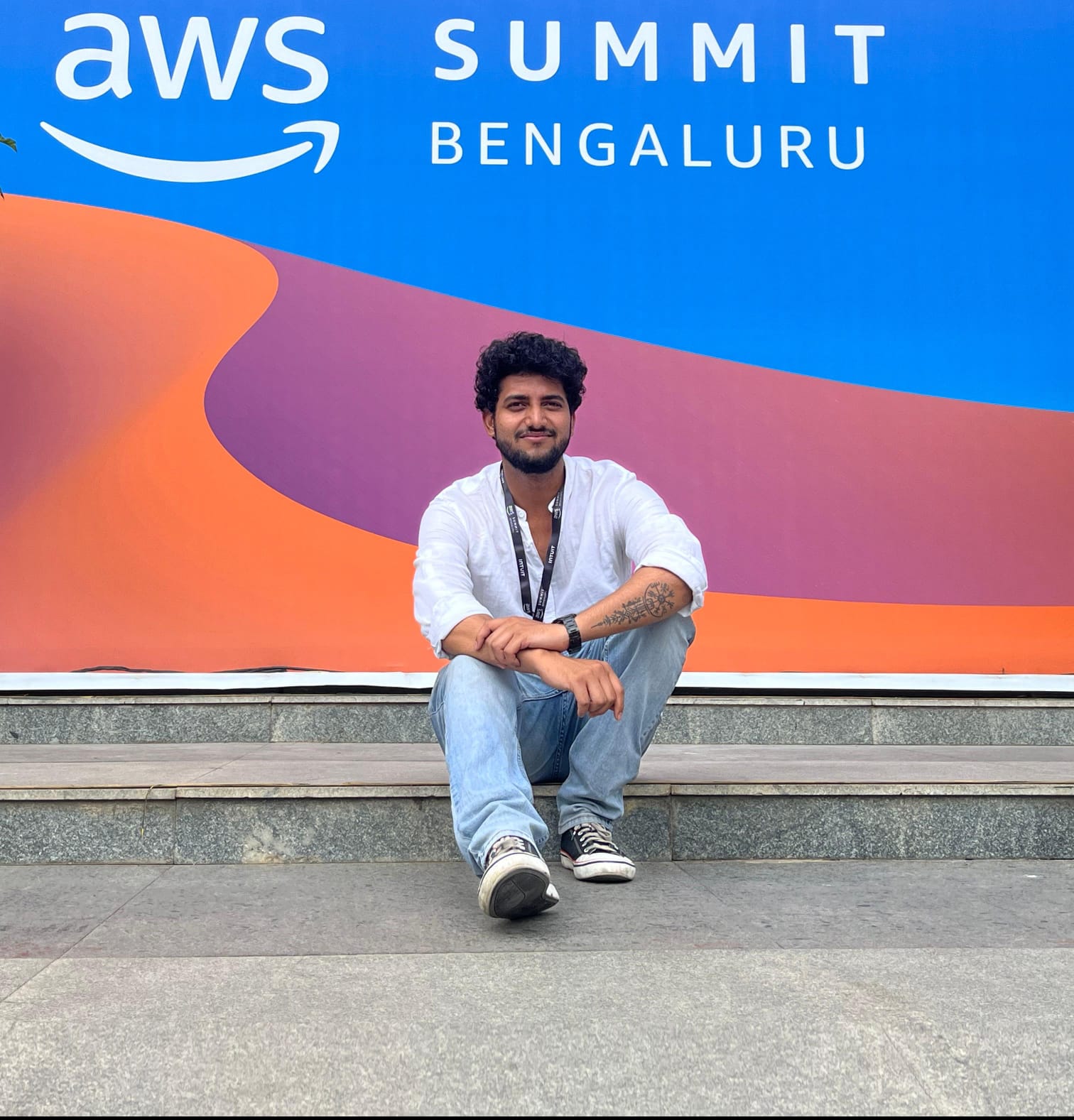
Brijesh Pandey
Brijesh Pandey
I'm a passionate software engineer with a strong background in web development, including both frontend and backend technologies. I'm particularly excited about the potential of Web 3.0 and enjoy working on cutting-edge projects in this space. Beyond coding, I'm an adventurous individual who loves to travel and explore new places, cultures, and cuisines. I'm also athletic and enjoy playing football, cricket, and badminton. I'm always up for a challenge and believe in trying new things!