Mastering Graph Algorithms: From BFS and DFS to Dijkstra and A*
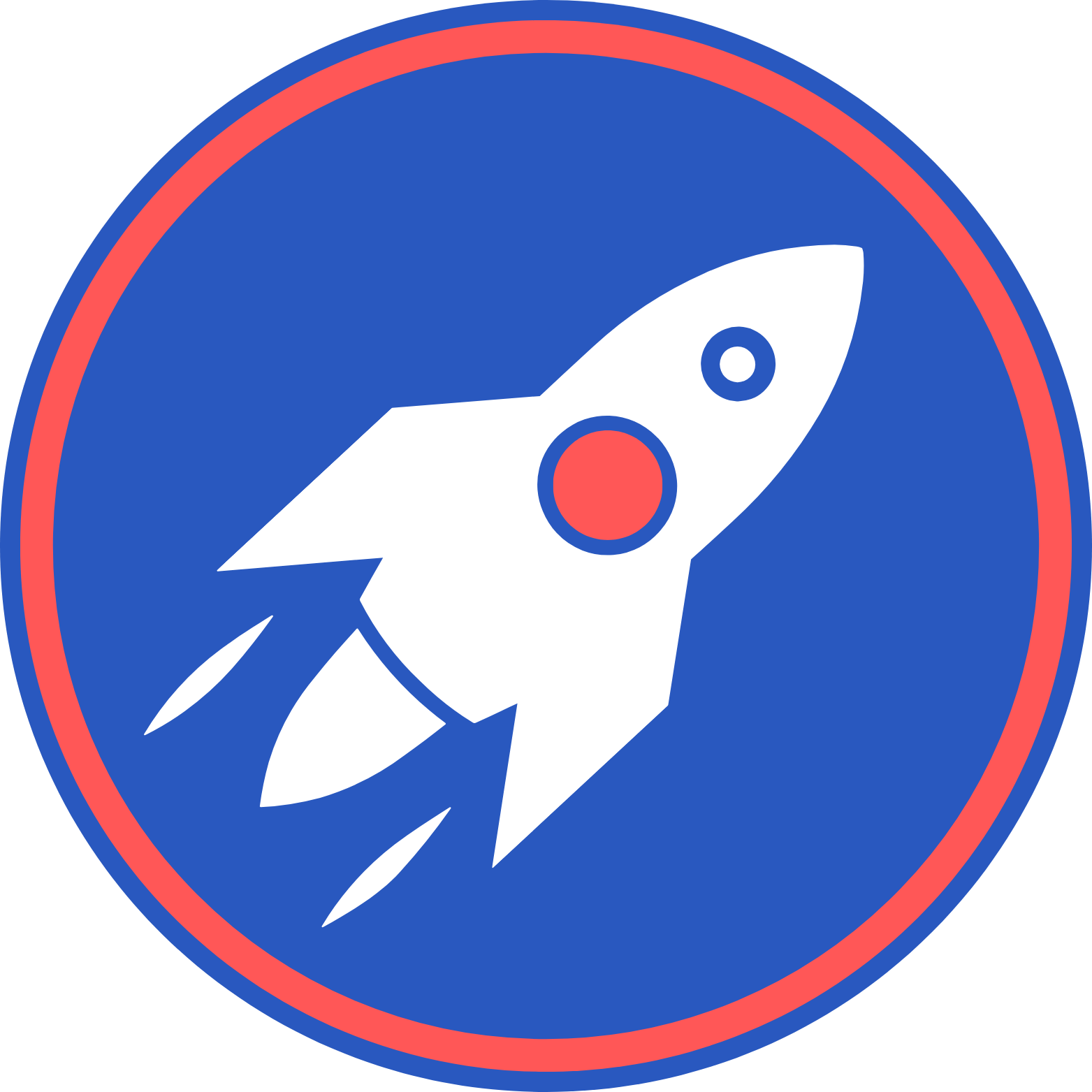
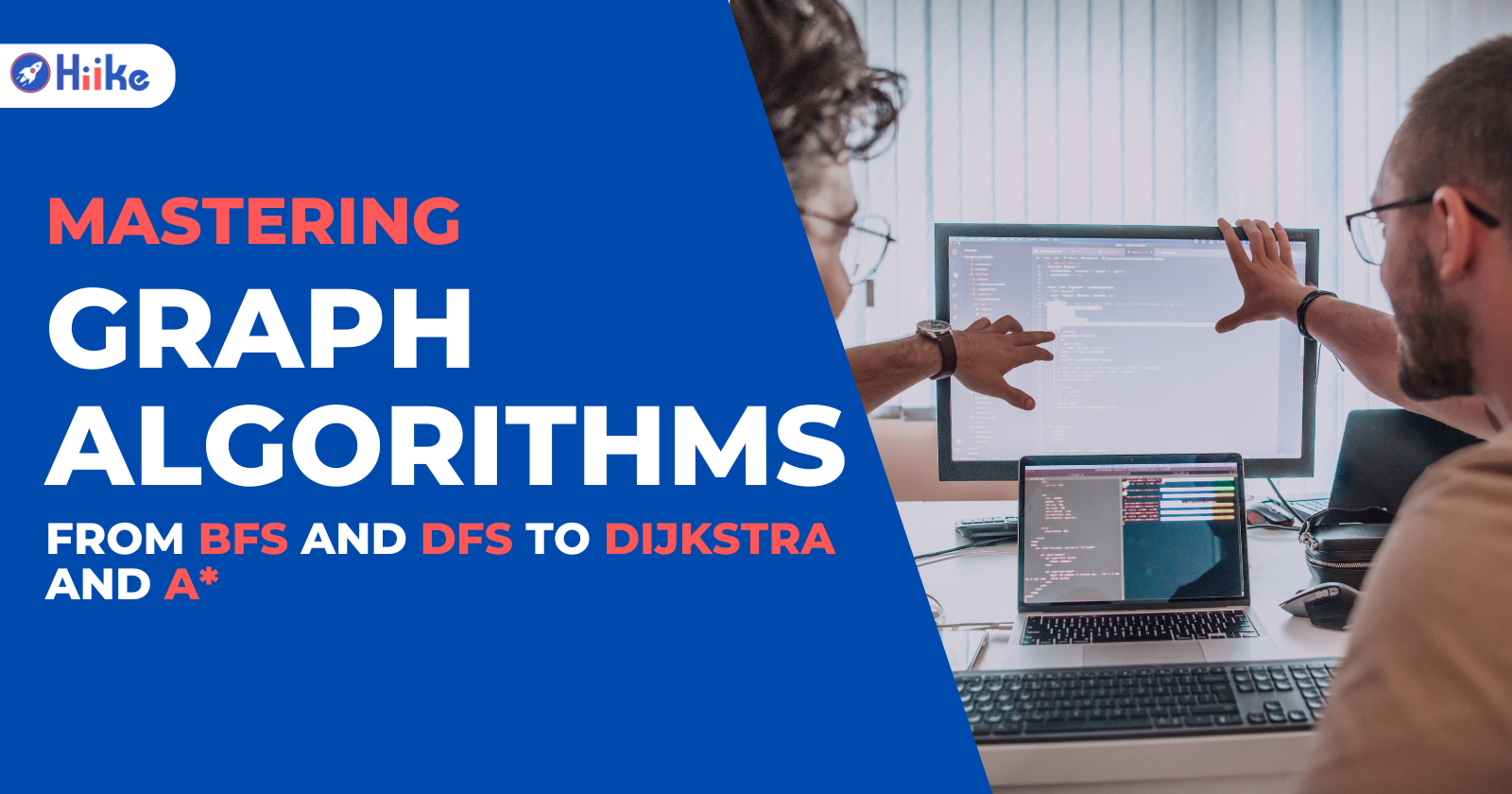
Graph algorithms are a cornerstone of computer science, crucial for solving a variety of complex problems related to network connectivity, pathfinding, and more. This blog post will introduce you to key graph algorithms, specifically Breadth-First Search (BFS), Depth-First Search (DFS), Dijkstra's algorithm, and the A* algorithm. We'll delve into their workings, provide practical examples and exercises, and discuss their applications and use cases.
Introduction to Graph Algorithms
Graphs are data structures consisting of nodes (vertices) connected by edges. They are used to model relationships and structures in a wide range of applications, from social networks to transportation systems. Graph algorithms are designed to traverse, search, and find optimal paths in these structures.
Explanation of BFS and DFS
Breadth-First Search (BFS)
BFS is an algorithm for traversing or searching tree or graph data structures. It starts at a selected node (often referred to as the root) and explores all its neighbors at the present depth level before moving on to nodes at the next depth level.
Steps:
Start at the root node.
Explore all neighbor nodes at the present level.
Move to the next level and repeat until all nodes are visited.
Depth-First Search (DFS)
DFS is an algorithm for traversing or searching tree or graph data structures. It starts at the root node and explores as far along each branch as possible before backtracking.
Steps:
Start at the root node.
Explore each branch completely before moving to the next branch.
Backtrack as necessary until all nodes are visited.
Detailed Look at Dijkstra and A* Algorithms
Dijkstra's Algorithm
Dijkstra's algorithm is used to find the shortest path between nodes in a graph, which may represent, for example, road networks.
Steps:
Initialize distances from the start node to all other nodes as infinity, except the start node itself, which is zero.
Use a priority queue to continually extract the node with the smallest distance.
Update the distance to each neighbor of the current node.
Repeat until all nodes have been visited.
A* Algorithm
A* is a pathfinding and graph traversal algorithm, which is often used in computer science for pathfinding and graph traversal, the process of plotting an efficiently traversable path between multiple nodes.
Steps:
Initialize the open list (nodes to be evaluated) and closed list (nodes already evaluated).
Add the start node to the open list.
Loop until the destination node is found or the open list is empty.
Consider the node with the lowest cost in the open list.
Generate its successors and evaluate them.
If a successor is the destination, stop.
Otherwise, update the costs and continue.
Practical Examples and Exercises
Exercise 1: Implementing BFS and DFS
Create a graph representation and implement BFS and DFS algorithms to traverse it. Test your implementation with different starting nodes and graphs to understand the traversal process.
Exercise 2: Shortest Path with Dijkstra's Algorithm
Implement Dijkstra's algorithm on a weighted graph and find the shortest path from a starting node to all other nodes. Visualize the graph and paths to better understand how the algorithm works.
Exercise 3: Pathfinding with A*
Implement the A* algorithm to find the shortest path between two nodes in a graph. Experiment with different heuristic functions and graph structures to see how they affect the algorithm's performance.
Applications and Use Cases
BFS and DFS: Used in social networks for friend suggestions, web crawling, and finding connected components.
Dijkstra's Algorithm: Commonly used in GPS navigation systems to find the shortest route.
A Algorithm:* Widely used in game development for pathfinding and in robotics for obstacle avoidance and navigation.
Conclusion
Mastering graph algorithms is essential for tackling a wide range of computational problems. BFS and DFS are foundational algorithms for graph traversal, while Dijkstra's and A* algorithms are powerful tools for finding the shortest paths in weighted graphs. Understanding these algorithms and their applications will enhance your problem-solving skills and prepare you for advanced technical roles.
At Hiike, we are committed to helping you master these essential skills through our comprehensive Data Structures and Algorithms programs. Our Top 30 Program offers advanced training, practical application, and real-world scenarios, ensuring you are thoroughly prepared for your career. Join Hiike today and take the next step towards mastering graph algorithms and beyond.
For more information, visit our website and explore our courses designed to elevate your skills and open doors to exciting career opportunities in the tech industry.
Subscribe to my newsletter
Read articles from Hiike directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
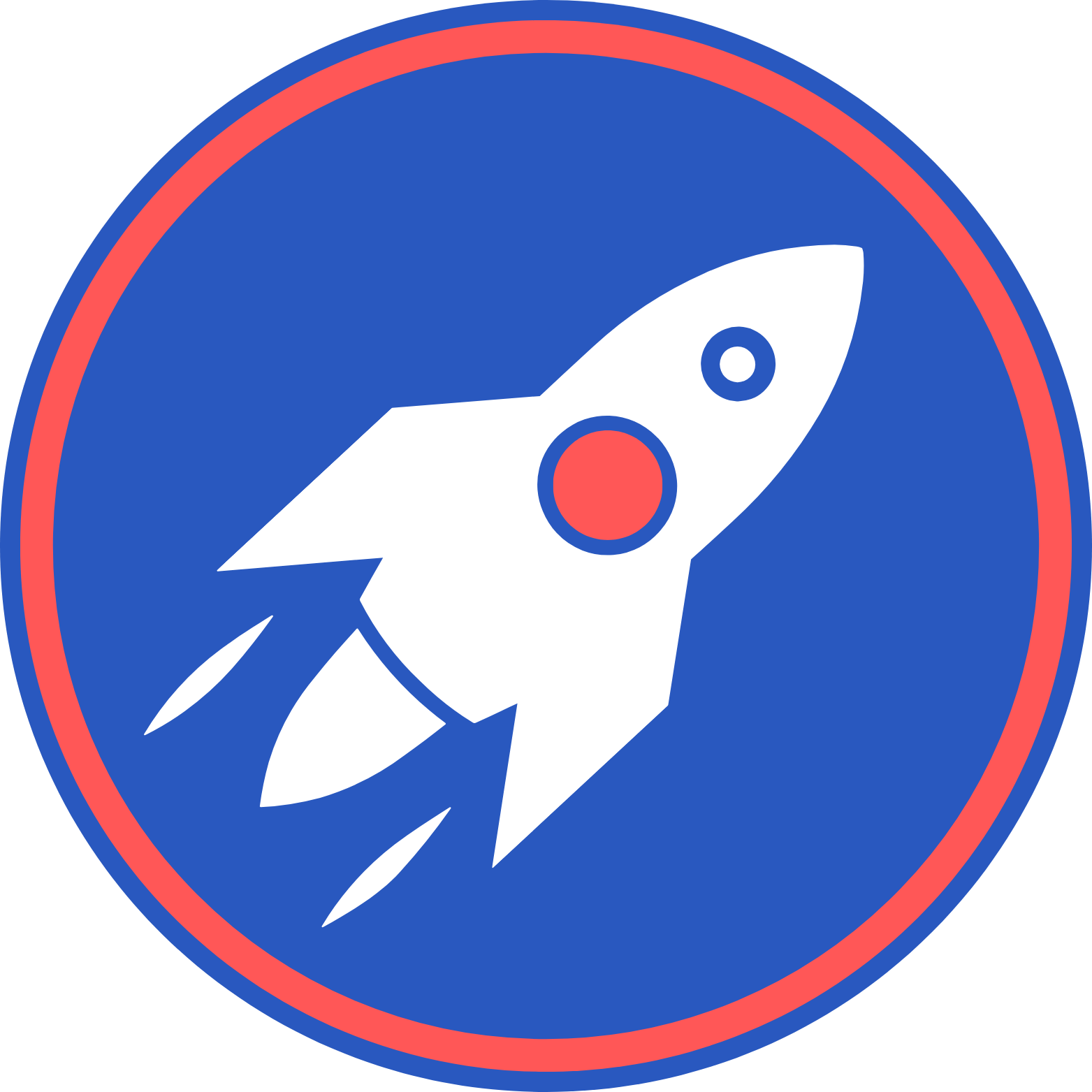
Hiike
Hiike
๐๐๐ข๐ข๐ค๐ - Crafting Your Tech Odyssey! ๐ Join Hiike for immersive learning, expert mentorship, and accelerated career growth. Our IITian-led courses and vibrant community ensure you excel in Data Structures, Algorithms, and System Design. Transform your tech career with Hiike today!