Comprehensive Beginner's Guide to Python Programming

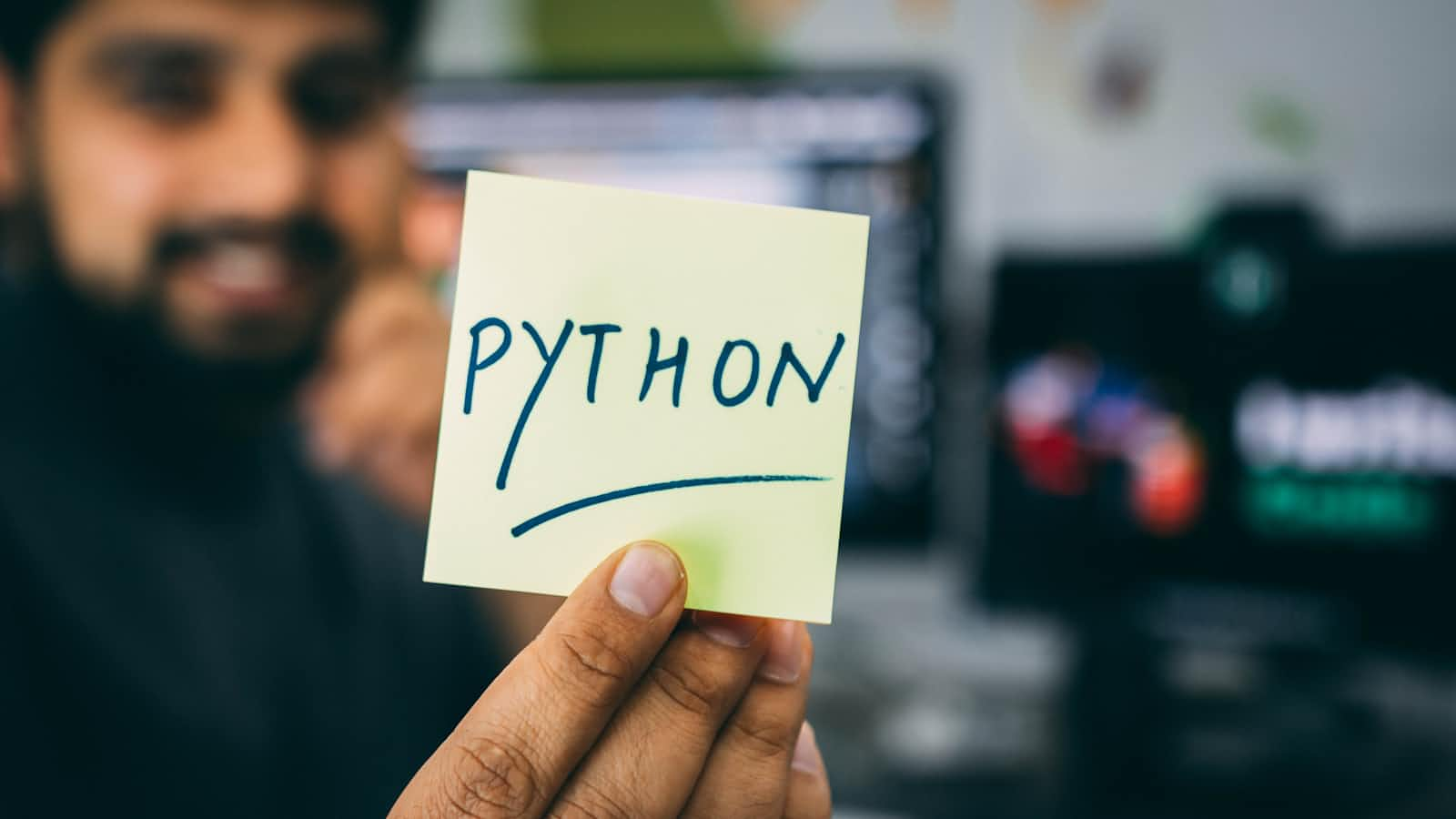
Python is a versatile and popular programming language known for its readability and ease of use. This guide will introduce you to the basics of Python programming.
My believe is after you complete this guide, you can boldly move to the intermediate concept of python programming.
Installing Python
Before you start coding, you need to have Python installed on your computer. You can download Python from the official website: python.org. Follow the instructions for your operating system to install Python.
Executing Python Code
Python code can be executed in different ways, depending on your preferences and requirements. Here are several options:
1. Running Python Code Interactively
You can run Python code interactively using the Python interpreter. Open your terminal or command prompt and type python
. You'll enter the Python interpreter, where you can type Python code directly and see the results immediately.
>>> print("Hello, World!")
Hello, World!
To exit the interpreter, type exit()
or press Ctrl + D
.
2. Running Python Files from Terminal
You can write Python code in a file and execute it from the terminal. Create a file named hello.py
with the following content:
print("Hello, World!")
Then, in the terminal, navigate to the directory containing hello.py
and run the file using:
python hello.py
3. Using a Python Integrated Development Environment (IDE)
IDEs provide a convenient environment for writing and executing Python code, with features like syntax highlighting, code completion, and debugging tools. Open your preferred IDE (e.g., PyCharm, VS Code, or Spyder), create a new Python file, write your code, and then run it from within the IDE.
4. Jupyter Notebooks
Jupyter Notebooks allow you to create and share documents containing live code, equations, visualizations, and explanatory text. Open Jupyter Notebook, create a new notebook, write your Python code in cells, and execute them interactively.
5. Online Python Interpreters
Several websites offer online Python interpreters where you can write and execute Python code directly in your web browser. Visit an online Python interpreter website (e.g., repl.it, Ideone), write your Python code in the editor, and run it to see the output.
Hello, World!
Let's start with the classic first program:
print("Hello, World!")
This line of code prints "Hello, World!" to the screen. In Python, the print
function is used to display text.
Variables
Variables are used to store data values. A variable is created the moment you first assign a value to it.
x = 10
y = "Hello"
print(x)
print(y)
Operators
Arithmetic Operators
x = 10
y = 5
print(x + y) # Addition
print(x - y) # Subtraction
print(x * y) # Multiplication
print(x / y) # Division
print(x % y) # Modulus(Remainder)
print(x ** y) # Exponentiation
print(x // y) # Floor division
Comparison Operators
x = 10
y = 5
print(x == y) # Equal to
print(x != y) # Not equal to
print(x > y) # Greater than
print(x < y) # Less than
print(x >= y) # Greater than or equal to
print(x <= y) # Less than or equal to
Logical Operators
a = True
b = False
print(a and b) # Logical AND
print(a or b) # Logical OR
print(not a) # Logical NOT
Datatypes
Numeric Types
Integer (
int
): Whole numbers, e.g.,5
Float (
float
): Decimal numbers, e.g.,5.0
Complex (
complex
): Complex numbers, e.g.,5 + 2j
a = 5 # int
b = 5.5 # float
c = 5 + 2j # complex
print(type(a), type(b), type(c))
Boolean Type
- Boolean (
bool
): RepresentsTrue
orFalse
is_true = True
is_false = False
print(type(is_true), is_true)
String Type
- String (
str
): Sequence of characters, e.g.,"Hello"
greeting = "Hello, World!"
print(type(greeting), greeting)
List (list
)
Lists are ordered, mutable collections of items, which can be of different types.
Used for storing sequences of items, such as a collection of objects, a range of numbers, or any ordered data.
fruits = ["apple", "banana", "cherry"]
numbers = [1, 2, 3, 4, 5]
print(fruits)
print(numbers)
Tuple (tuple
)
Tuples are ordered, immutable collections of items, which can be of different types.
Used for storing fixed collections of items that should not be changed, such as coordinates or database records.
coordinates = (10.0, 20.0)
user_info = ("Alice", 25, "New York")
print(coordinates)
print(user_info)
Dictionary (dict
)
Dictionaries are unordered collections of key-value pairs.
Used for storing data that is associated with unique keys, such as configurations, user data, or any mapping of unique keys to values.
person = {
"name": "Alice",
"age": 25,
"city": "New York"
}
print(person)
print(person["name"])
Set (set
)
Sets are unordered collections of unique items.
Used for storing unique items, removing duplicates, or performing set operations like union, and intersection.
unique_numbers = {1, 2, 3, 3, 4}
print(unique_numbers) # Output: {1, 2, 3, 4}
Frozenset (frozenset
)
Frozensets are immutable sets.
Used for storing unique items when immutability is required, such as in keys for dictionaries or other hashable collections.
immutable_set = frozenset([1, 2, 3, 3, 4])
print(immutable_set)
Bytes (bytes
)
Bytes are immutable sequences of bytes.
Used for binary data, such as images, files, or network communication.
byte_data = b"hello"
print(byte_data)
print(byte_data[0]) # Output: 104 (ASCII value of 'h')
Bytearray (bytearray
)
Bytearrays are mutable sequences of bytes.
Used for mutable binary data, where modifications are needed.
byte_array = bytearray(b"hello")
byte_array[0] = 72 # Changing 'h' to 'H'
print(byte_array)
NoneType (None
)
NoneType has a single value, None
, used to signify 'nothing' or 'no value here'.
Used as a default value, to signify the absence of a value, or as a placeholder.
result = None
if result is None:
print("No result")
Control Flow
If Statements
x = 10
if x > 5:
print("x is greater than 5")
elif x == 5:
print("x is 5")
else:
print("x is less than 5")
Loops
For Loop
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
While Loop
i = 1
while i < 6:
print(i)
i += 1
Functions
Functions are blocks of code that perform a specific task.
def greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
Importing Libraries
Python has a rich ecosystem of libraries that you can use to extend its capabilities. For example, you can use the math
library for mathematical operations:
import math
print(math.sqrt(16)) # square root
print(math.pi) # value of pi
File Handling
Reading a File
with open("example.txt", "r") as file:
content = file.read()
print(content)
Writing to a File
with open("example.txt", "w") as file:
file.write("Hello, World!")
If you enjoy this guide, give a like and follow us for more tech skill learning.
For question, shoot in the comment.
Subscribe to my newsletter
Read articles from Babatunde Daramola directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Babatunde Daramola
Babatunde Daramola
Over a decade in web development | PHP | Laravel | Js | Vue | React | nodejs | Educator. More = https://linktr.ee/ritechoice23