A Guide to JavaScript String Methods
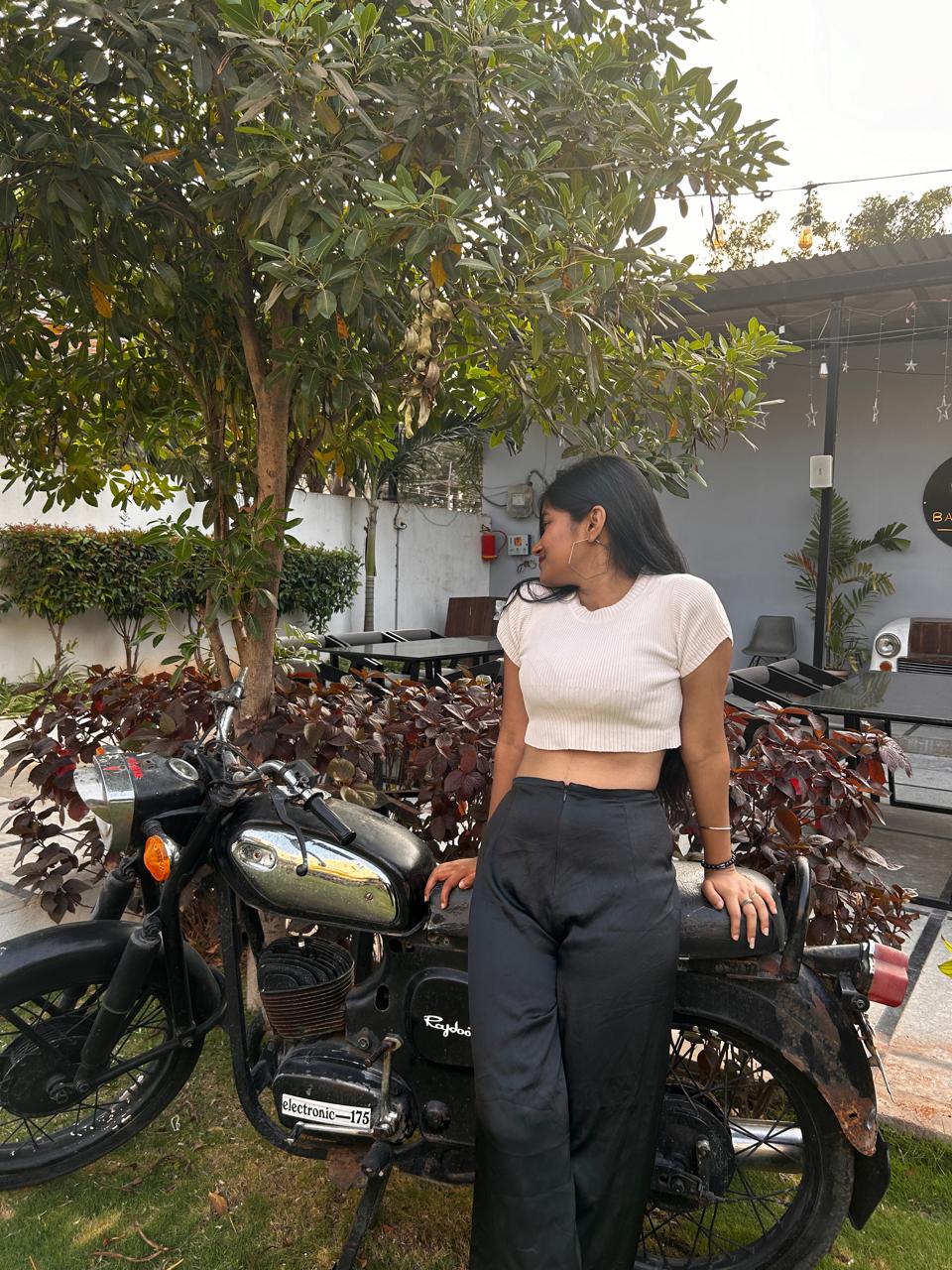
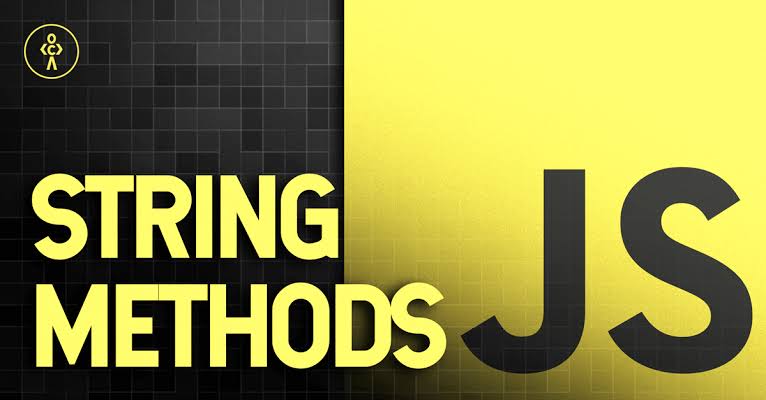
In this Article you will learn about the essential String Methods used in JavaScript.
The Prerequisites to understand this Article completely are :
Basic Javascript Syntax
Basic Knowledge of Datatypes
What are the Methods?
Methods are the same thing as Functions. The difference between Functions and Methods are that Methods are Functions inside objects and are attached to them, whereas Functions can exist on their own while methods cannot.
JavaScript has built in pre-defined Methods,they are used to manipulate,remove,add,replace parts of strings ,also perform mathematical operations and other computations.
String Object
What is String Object?? How are strings objects??
Valid question,Valid doubt.
Strings are surely strings but Javascript automatically creates an String objects that consists of all the characters present in the string with their Indexes.
Hence why Strings can have methods.But the methods are of String Object rather than Strings
String Methods
The most frequently used and important string Methods are mentioned below :
String Length
This property returns the length of the string.This is also called Instance Method.
let str = "Hello, world!";
let length = str.length;
console.log(length); // Output: 13
Methods to extract Characters in Strings
String charCodeAt()
String charAt()
String at()
Using [ ]
String charCodeAt()
This method returns the code of the character at a specified index mentioned inside the parenthesis.
It returns the UTF-16 code of the character.
let str="Hello, Javascript";
let charCode= str.charCodeAt(4);
console.log(charCode); // Output: 111 (ASCII code for 'o
String charAt()
This method returns the character at a specific index in a string.
This method takes one parameter - the index.
let str="Hello, Javascript";
let charAt= str.charAt(3);
console.log(charAt); //Output : l
String at()
ES2022 introduced this method.
It performs the same function as string charAt() but is shorter ,hence more efficient to use.
let str="Hello, Javascript";
let atpositive = str.at(3);
let atnegative = str.at(-3);
console.log(atpositive);// output : l
console.log(atNegative);// output : i
[ ] Property
It is not consistent in nature.
As you can see in the code it makes the string looks like arrays and it returns undefined when the string is empty.
let str="Hello, Javascript";
let str2 ="";
let char = str[3];
let char2 = str2[3];
console.log(char);// output : l
console.log(char2);// output :undefined
Methods to extract String Parts
String slice()
String substring()
String substr()
String slice ()
This method first extracts the part we need and then returns us the part of the string in a new string.
It takes two parameters -(index of start , index of end) [the end is not included]
let str="Hello, Javascript";
let string2=str.slice(2,5);//output= llo
let string3=str.slice(2);//output=llo, Javascript
let string4=str.slice(-5);//output=cript
let string5=str.slice(-5,-2);//output=cri
console.log(string2);
console.log(string3);
console.log(string4);
console.log(string5);
String substring ()
This method and slice method has the same function and works the same way most of the time
But in substring () if either of the index value is less than 0(negative) it treats them as 0.
let str="Hello, Javascipt";
let str1=str.substring(7);// output=Javascript
let str2=str.substring(7,11);// output= Java
console.log(str1);
console.log(str2);
String substr()
This methods works the same except the second parameter specifies the length of the string and does not act like the termination index.
let string = "Hello, World!";
let substring1 = string.substr(7);
console.log(substring1); // Output: "World!"
let substring2 = string.substr(7, 5);
console.log(substring2); // Output: "World"
String toUpperCase() and String toLowerCase()
String toUpperCase () converts the whole string to Uppercase
let string = "hello, Javascript!";
let uppercaseString = string.toUpperCase();
console.log(uppercaseString); // Output: "HELLO, JAVASCRIPT!"
String to LowerCase () converts the whole string to LowerCase
let string = "hello, Javascript!";
let lowercaseString = string.toLowerCase();
console.log(lowercaseString); // Output: "HELLO, JAVASCRIPT!"
String Concat
This method is used to join two or more string.
let string1 = "Hello, ";
let string2 = "Javascript!";
let concatenatedString = string1.concat(string2);
console.log(concatenatedString); // Output: "Hello, Javascript!"
It's preferred over '+' operator and looks more formal and readable.
let string1 = "Hello, ";
let string2 = "javascript!";
let string3 = " How are you?";
let concatenatedString = string1.concat(string2, string3);
console.log(concatenatedString); // Output: "Hello, javascript! How are you?"
Trim Methods
Trim methods are used to remove whitespaces from a string.
String trim()
String trimEnd() -Added by ECMAScript2019
String trimStart() - Added by ECMAScript2019
String trim()
This method removes whitespaces from both tne end and starting of the string.
let stringWithWhitespace = " Hello, world! ";
let trimmedString = stringWithWhitespace.trim();
console.log(trimmedString); // Output: "Hello, world!"
String trimEnd()
This method removes whitespaces from the end of the string.
let stringWithWhitespace = " Hello, javascript! ";
let trimmedEndString = stringWithWhitespace.trimEnd();
console.log(trimmedEndString); // Output: " Hello, javascript!"
String trimStart()
This method removes whitespaces from the start of the string.
let stringWithWhitespace = " Hello, javascript! ";
let trimmedStartString = stringWithWhitespace.trimStart();
console.log(trimmedStartString); // Output: "Hello, javascript! "
Padding Methods
ECMAScript2017 added these methods.
String padStart()
This method pads the string from the start with another string mentioned in the parameter.
It pads the string as many times as the user asks for.
It takes two parameters -(number of times we need to add the string in the start,"string to be added")
let paddedString = "5".padStart(4, "0"); // Output: "0005"
String padEnd()
This method pads the string from the end with another string mentioned in the parameter.
It takes two parameters -(number of times we need to add the string in the end,"string to be added")
let paddedString = "5".padEnd(4, "0"); // Output: "5000"
String Repeat
This method returns a new string with the number of copies of a string specified by the user.
It takes one parameter - (number of copies to be made/number of times to repeat the string)
let repeatedString = "abc".repeat(3); // Output: "abcabcabc"
Replace Methods
String replace()
String replaceAll()
String replace()
This method replaces a specificed value or part of string with another value in a string.
It takes two parameters - ("value to be replaced","value by which it is to be replaced")
String replace() only replaces the first matching value and not all of them.
let originalString = "Hello, world!";
let replacedString = originalString.replace("world", "universe");
console.log(replacedString); // Output: "Hello, universe!"
String replaceAll ()
This method replaces a specificed value or part of string with another value in a string.
It takes two parameters - ("value to be replaced","value by which it is to be replaced")
String replaceAll() replaces all the matching values.
let originalString = "Hey world...Hello, world!";
let replacedString = originalString.replace(/world/g, "universe");
console.log(replacedString); // Output: "Hey universe...Hello, universe!"
String split()
This method converts string into arrays.
It can be split on the basis of the operator specified in the parameter.
let string = "apple,banana,orange";
let fruitsArray = string.split(",");
console.log(fruitsArray); // Output: ["apple", "banana", "orange"]
More on String Methods
Lesser user methods include the following:
String includes()
Returns true if the string contains the given string, otherwise false.It takes one parameter - ("string we are searching for")
let sentence = 'The quick brown fox jumps over the lazy dog.';
let word = sentence.includes("fox");
console.log(word);
Refer to MDN Strings to explore more string methods .
Summary
The articles tells us about what are string methods,here we used javascript as our language for code blocks.
We talk about how to extract characters from a string,how to extract parts of strings,removing whitespaces,manipulations,padding methods,how to replace certain parts of string and how to convert a string into array.
Subscribe to my newsletter
Read articles from Sreehitha Thati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
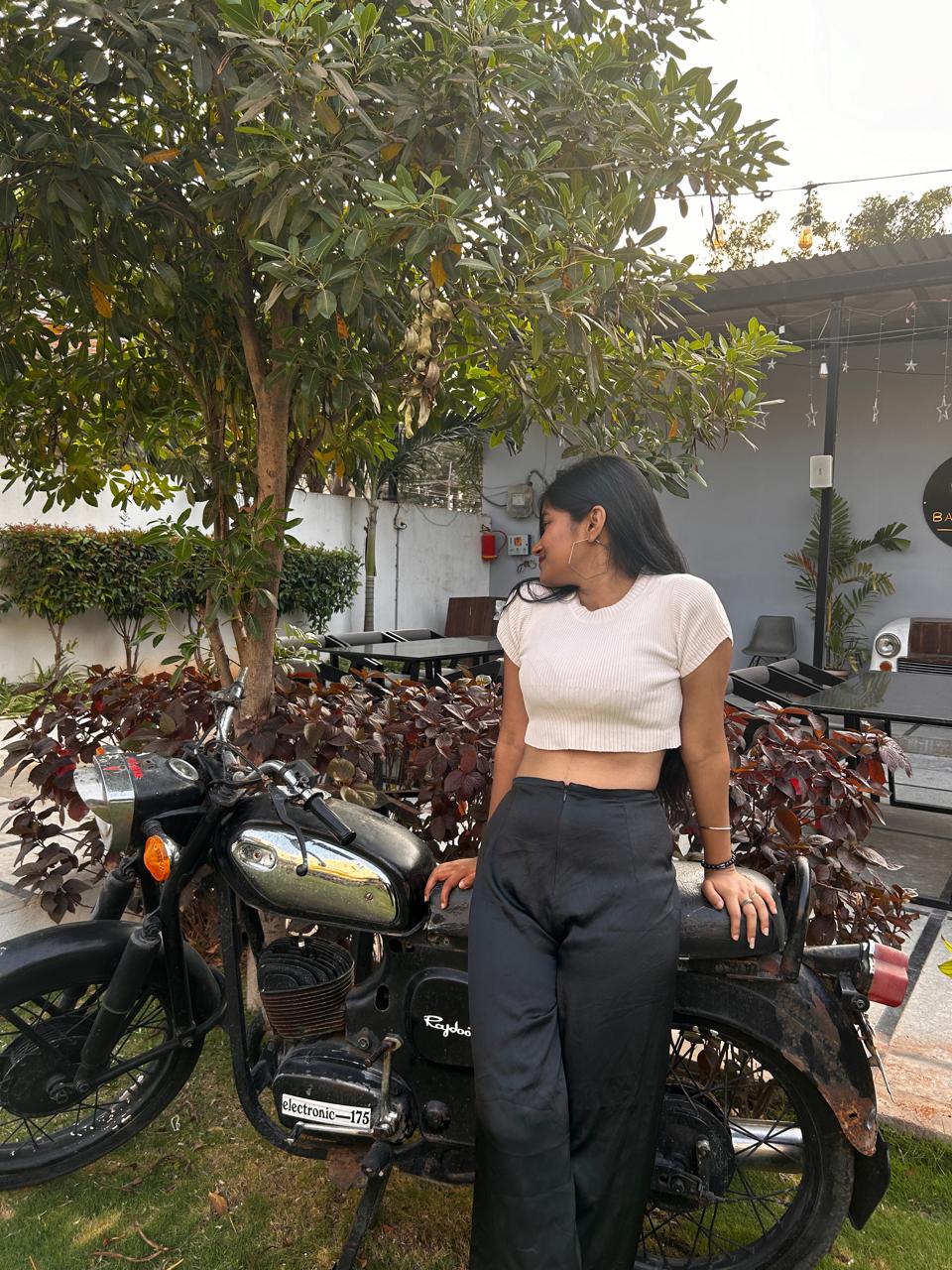