Facial Recognition with Python: How to Build a Face Detection System
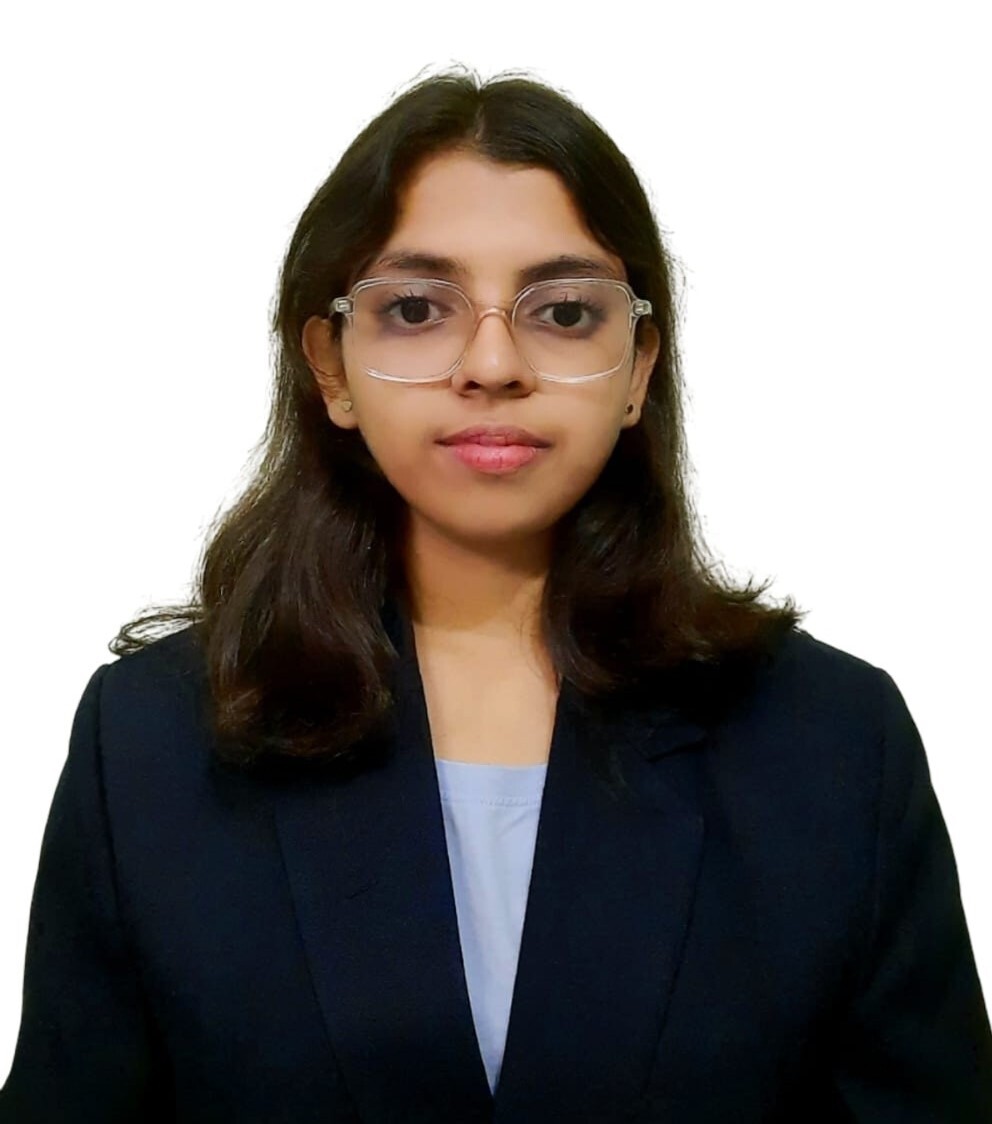
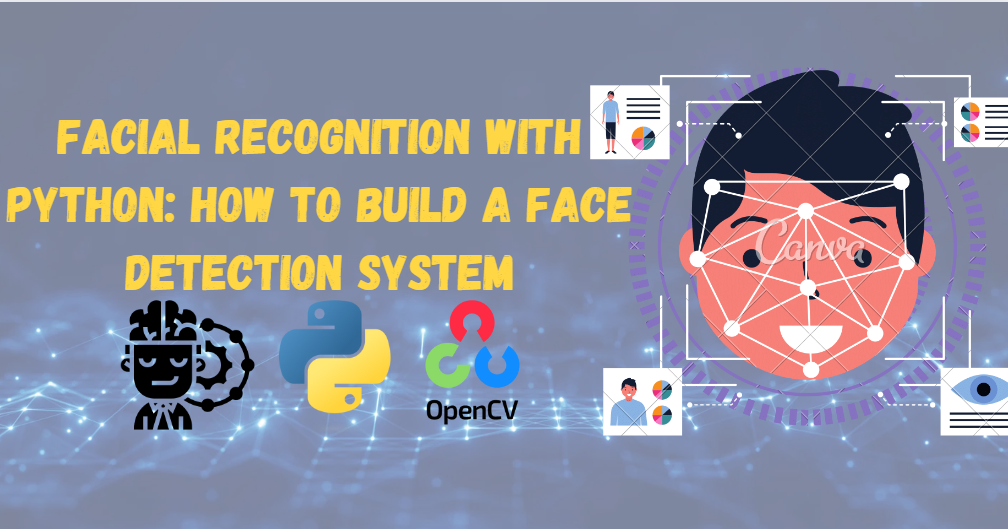
What is Facial Recognition?
Facial recognition is a type of technology that can detect and identify human faces in images or videos. It's like teaching a computer to recognize people, just like you do when you see your family and friends. This technology is used in various applications, such as unlocking your phone, tagging friends in photos on social media, and even in security systems.
How Does Facial Recognition Work?
Facial recognition involves several steps to accurately detect and identify faces. Let's break it down into simple steps:
Detection: Finding a face in an image.
Alignment: Positioning the face in a way that makes it easier to analyze.
Feature Extraction: Identifying unique features of the face.
Recognition: Comparing these features to a database of known faces to find a match.
Step-by-Step Process
Detection: This is the first step where the system scans an image to locate any faces. Imagine it as a scanner that goes over the image and marks any regions that look like a face.
Alignment: Once a face is detected, the system adjusts the face to a standardized position. This step ensures that features like the eyes, nose, and mouth are in expected locations, making the analysis easier and more accurate.
Feature Extraction: Here, the system identifies and measures key facial features. Think of it as drawing a map of the face, noting the distance between the eyes, the shape of the jawline, etc.
Recognition: Finally, the system compares the extracted features against a database of known faces to identify the person. It’s like comparing a fingerprint to a database to find a match.
Building a Face Detection System with Python
Now, let's dive into how you can build a basic face detection system using Python and a library called OpenCV. OpenCV is like a toolbox full of tools that help us process and understand images.
Step 1: Install OpenCV
First, you need to install the OpenCV library. You can do this using pip, a package manager for Python. Open your command prompt or terminal and type the following command:
pip install opencv-python
Step 2: Import Libraries
Next, let's import the necessary libraries in your Python script:
import cv2
Step 3: Load an Image
Let's start by loading an image that contains a face. You can use any image you like. Here, we'll load an image named face.jpg
:
# Load the image
image = cv2.imread('face.jpg')
Step 4: Convert the Image to Grayscale
Converting the image to grayscale makes it easier to detect faces, as it simplifies the image data. In grayscale images, each pixel represents a shade of gray, reducing the complexity compared to colored images.
# Convert the image to grayscale
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
Step 5: Load the Haar Cascade Classifier
OpenCV uses a pre-trained classifier to detect faces. This classifier is called a Haar Cascade. We need to load it first. Haar Cascades are like templates that help the system recognize patterns that look like faces.
# Load the Haar cascade file for face detection
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
Step 6: Detect Faces
Now, let's use the classifier to detect faces in the image. The detectMultiScale
function scans the image at different scales (sizes) to find faces.
# Detect faces in the image
faces = face_cascade.detectMultiScale(gray_image, scaleFactor=1.1, minNeighbors=5)
scaleFactor: This parameter compensates for faces that appear bigger or smaller than expected. A scale factor of 1.1 means the image size is reduced by 10% at each scale.
minNeighbors: This parameter helps filter out false positives (areas that aren't faces but might look like one). Higher values result in fewer detections but increase the quality of the detections.
Step 7: Draw Rectangles Around Detected Faces
We can now draw rectangles around the detected faces to highlight them. This helps us visualize where the faces are in the image.
# Draw rectangles around detected faces
for (x, y, w, h) in faces:
cv2.rectangle(image, (x, y), (x+w, y+h), (255, 0, 0), 2)
Here:
(x, y) represents the top-left corner of the rectangle.
w and h represent the width and height of the rectangle.
(255, 0, 0) specifies the color of the rectangle in BGR (Blue, Green, Red) format.
2 is the thickness of the rectangle's border.
Step 8: Display the Result
Finally, let's display the image with the detected faces. This allows us to see the results of our face detection system.
# Display the image with detected faces
cv2.imshow('Face Detection', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
cv2.imshow creates a window to display the image.
cv2.waitKey(0) waits for a key press to close the window.
cv2.destroyAllWindows closes all OpenCV windows.
Full Code
Here is the complete code to detect faces in an image:
import cv2
# Load the image
image = cv2.imread('face.jpg')
# Convert the image to grayscale
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Load the Haar cascade file for face detection
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
# Detect faces in the image
faces = face_cascade.detectMultiScale(gray_image, scaleFactor=1.1, minNeighbors=5)
# Draw rectangles around detected faces
for (x, y, w, h) in faces:
cv2.rectangle(image, (x, y), (x+w, y+h), (255, 0, 0), 2)
# Display the image with detected faces
cv2.imshow('Face Detection', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
Conclusion
Congratulations! You've just built a basic face detection system using Python and OpenCV. By following these steps, you can detect faces in images and highlight them. This is just the beginning of what you can do with facial recognition technology. With more advanced techniques and larger datasets, you can build more sophisticated systems that can recognize individual faces, analyze emotions, and much more.
Subscribe to my newsletter
Read articles from Trisha Banerjee directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
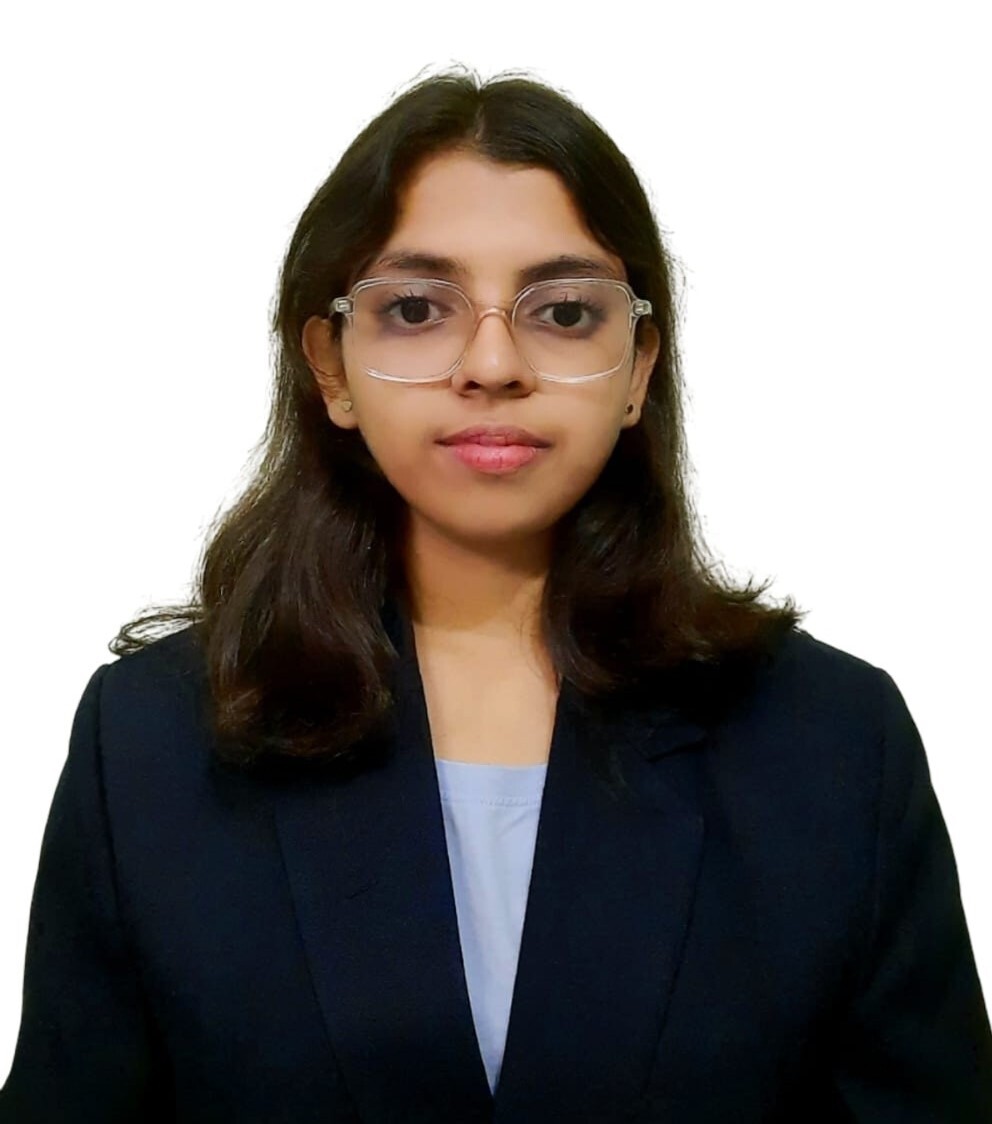
Trisha Banerjee
Trisha Banerjee
I'm Trisha, an enthusiastic AI/ML student with an insatiable curiosity for deciphering the intricacies of data. Currently immersed in the exhilarating journey of evolving into a Data Scientist, I harmonize the artistry of machine learning with the precision of AI. In the expansive realm of data science, my passions revolve around unlocking the transformative potential inherent in AI and ML. I firmly believe in the profound power of data to illuminate insights and drive informed decision-making. Join me as I navigate the dynamic landscape of artificial intelligence, embracing the endless possibilities that arise when passion meets data-driven exploration.