Essential Redis Interview Questions and Answers
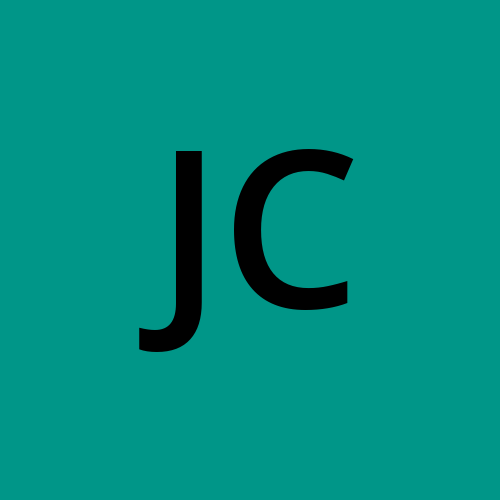
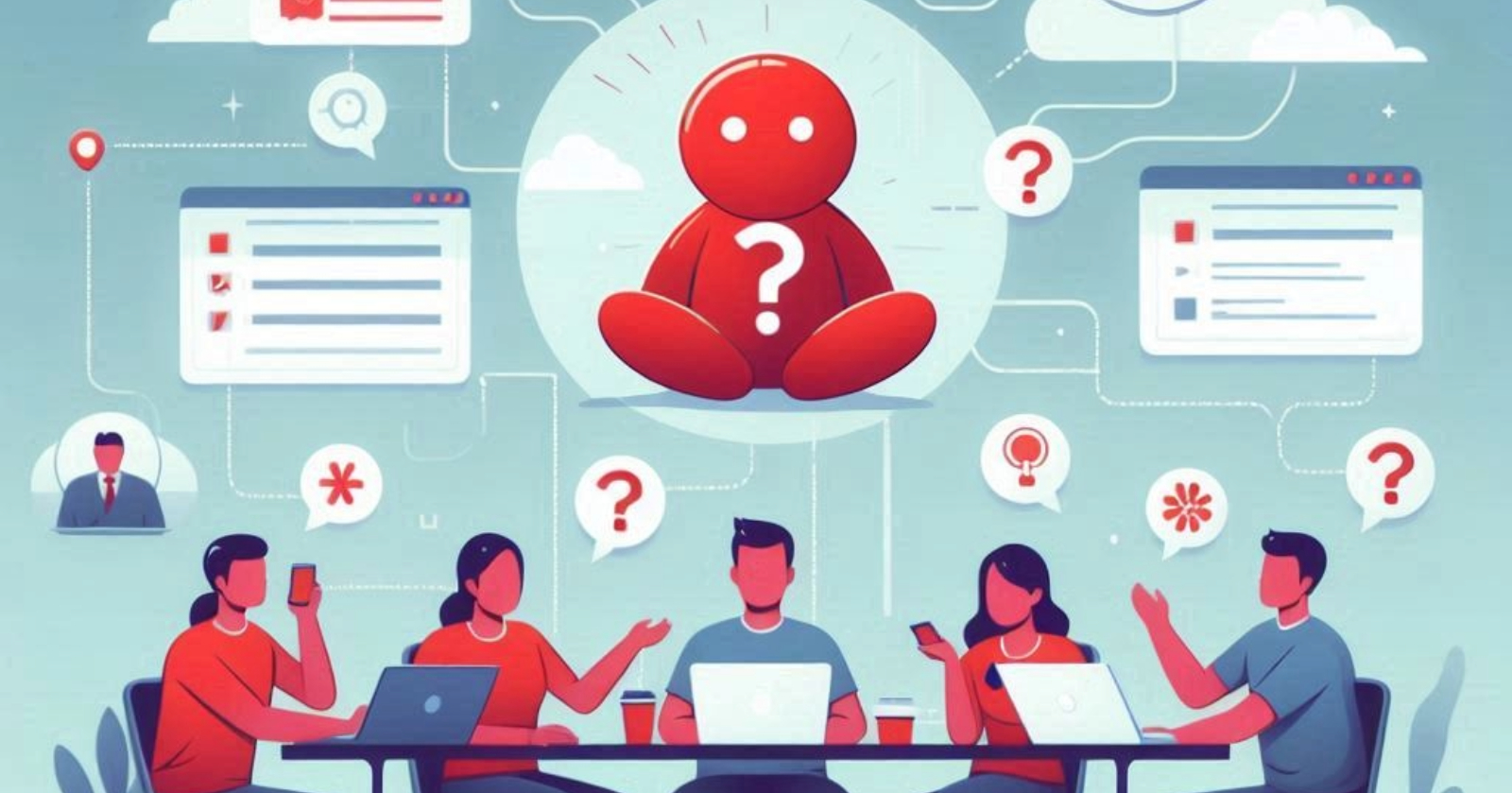
Redis, an open-source in-memory data store, is a popular choice for caching, real-time analytics, messaging, and more. When preparing for a Redis-related interview, it's important to understand both the fundamental concepts and advanced features. This article covers essential Redis interview questions and answers to help you succeed in your next interview.
Basic Redis Concepts
1. What is Redis and how does it work?
Redis (REmote DIctionary Server) is an in-memory data structure store, often used as a database, cache, and message broker. It works by storing data in memory, allowing for fast read and write operations. Redis supports various data structures like strings, lists, sets, hashes, and more, which makes it versatile for different use cases.
2. What are the primary features of Redis?
In-memory data storage for fast access
Data persistence options like RDB snapshots and AOF logs
Replication to provide high availability
Pub/Sub messaging for real-time communication
Atomic operations on data structures
Support for transactions using MULTI/EXEC
Lua scripting for server-side execution
Clustering for horizontal scaling
Data Structures and Commands
3. What data types does Redis support?
Redis supports:
Strings: Basic text or binary data.
Lists: Ordered collections of strings.
Sets: Unordered collections of unique strings.
Sorted Sets: Sets where each string is associated with a score.
Hashes: Maps between string fields and string values.
Bitmaps: Strings that can be treated as a sequence of bits.
HyperLogLogs: Probabilistic data structures for cardinality estimation.
Streams: Log data structures for managing messages.
4. Explain the difference between SET and GET commands in Redis.
SET: Sets the value of a key. If the key already exists, it updates the value.
SET mykey "Hello"
GET: Retrieves the value associated with a key.
GET mykey # Returns "Hello"
5. How do you implement caching with Redis?
To implement caching:
Store the data: Use the
SET
command to store data with a key.Set an expiration: Use the
EXPIRE
orSETEX
command to set a timeout.Retrieve the data: Use the
GET
command to retrieve cached data.Cache miss: Fetch from the original source if the key is not found and update the cache.
Example:
client.setex('mykey', 3600, 'some value'); // Cache for 1 hour
Advanced Features
6. What is Redis persistence and why is it important?
Redis persistence allows data to be saved to disk, ensuring that it can be recovered in case of a system failure. This is important for maintaining data integrity and preventing data loss. Redis supports two types of persistence:
RDB (Redis Database File): Saves snapshots of the dataset at specified intervals.
AOF (Append-Only File): Logs every write operation, allowing for more granular recovery.
7. What is Redis replication and how does it work?
Replication in Redis allows you to create replica (slave) instances that copy the data from a primary (master) instance. This provides redundancy, high availability, and can offload read operations to the replicas. Redis replication is asynchronous, meaning that replicas may lag behind the master.
Configuration:
SLAVEOF master_host master_port
8. Explain the Redis Pub/Sub system.
Redis Pub/Sub is a messaging pattern where messages are published to channels and subscribers receive messages from channels they are subscribed to. This is useful for real-time communication and event-driven systems.
Example commands:
SUBSCRIBE: Subscribe to a channel.
SUBSCRIBE mychannel
PUBLISH: Publish a message to a channel.
PUBLISH mychannel "Hello World"
Performance and Scaling
9. How do you optimize Redis performance?
Use proper data structures: Choose the right data structure for the job.
Data eviction policies: Configure appropriate eviction policies.
Memory management: Monitor and optimize memory usage.
Partitioning: Distribute data across multiple Redis instances using clustering.
Pipelining: Batch multiple commands to reduce the number of round-trips.
10. What is Redis clustering and how does it work?
Redis clustering provides a way to automatically distribute data across multiple Redis nodes, allowing for horizontal scaling and fault tolerance. Clustering partitions data into slots and assigns these slots to different nodes. This way, data is distributed evenly and can be retrieved from multiple nodes.
Setup example:
redis-server --cluster-enabled yes --cluster-config-file nodes.conf --cluster-node-timeout 5000
Practical Scenarios
11. How would you implement a rate limiter using Redis?
A rate limiter can be implemented using Redis to limit the number of actions a user can perform in a given timeframe. This can be done using the INCR
command to count actions and EXPIRE
to set a time limit.
Example:
const key = `rate_limit:${user_id}`;
const limit = 100; // Max 100 requests
const ttl = 60; // 1 minute
client.incr(key, (err, count) => {
if (count === 1) {
client.expire(key, ttl); // Set TTL only on first increment
}
if (count > limit) {
// Exceeded limit
console.log('Rate limit exceeded');
} else {
// Allowed
console.log('Request allowed');
}
});
12. Describe a scenario where Redis is not a suitable choice.
Redis is not suitable for:
Heavy computational tasks: Redis is optimized for fast data access, not computation-heavy tasks.
Large datasets: Redis stores all data in memory, making it costly for extremely large datasets.
Complex querying: Redis lacks the complex querying capabilities found in relational databases.
Strict data consistency: Redis replication is asynchronous, which may not suit applications requiring strict consistency.
13. How do you secure a Redis instance?
Bind to localhost: Restrict access to the local machine.
bind 127.0.0.1
Require authentication: Use the
requirepass
directive in the Redis configuration file.requirepass my_secure_password
Use SSL/TLS: Encrypt communications between clients and the server.
Disable dangerous commands: Use the
rename-command
directive to disable potentially harmful commands likeFLUSHALL
.
Best Practices
14. What are some best practices for using Redis in production?
Monitor performance: Use Redis monitoring tools to track metrics and health.
Set appropriate timeouts: Ensure commands have timeouts to prevent blocking.
Use backups: Regularly back up data to prevent loss.
Optimize memory usage: Monitor memory and optimize data structures to avoid excessive usage.
Plan for failure: Implement redundancy and failover strategies.
15. Explain Redis pipelining and when to use it.
Redis pipelining allows you to send multiple commands to the server without waiting for a response for each command. This reduces the number of round-trips between the client and server, improving performance.
Example:
client.pipeline()
.set('key1', 'value1')
.set('key2', 'value2')
.get('key1')
.get('key2')
.exec((err, results) => {
console.log(results);
});
Conclusion
Redis is a versatile and powerful tool for various use cases in modern applications. Understanding its core concepts, advanced features, and best practices can greatly enhance your chances of succeeding in a Redis-related interview. By mastering these interview questions and answers, you'll be well-prepared to demonstrate your knowledge and skills in working with Redis.
Subscribe to my newsletter
Read articles from Jay Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
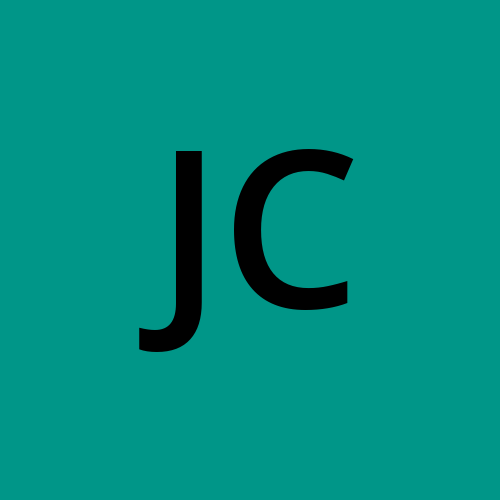
Jay Kumar
Jay Kumar
Jay Kumar is a proficient JavaScript and Node.js developer with 7 years of experience in building dynamic and scalable web applications. With a deep understanding of modern web technologies, Jay excels at creating efficient and innovative solutions. He enjoys sharing his knowledge through blogging, helping fellow developers stay updated with the latest trends and best practices in the industry.