846. Hand of Straights

1 min read
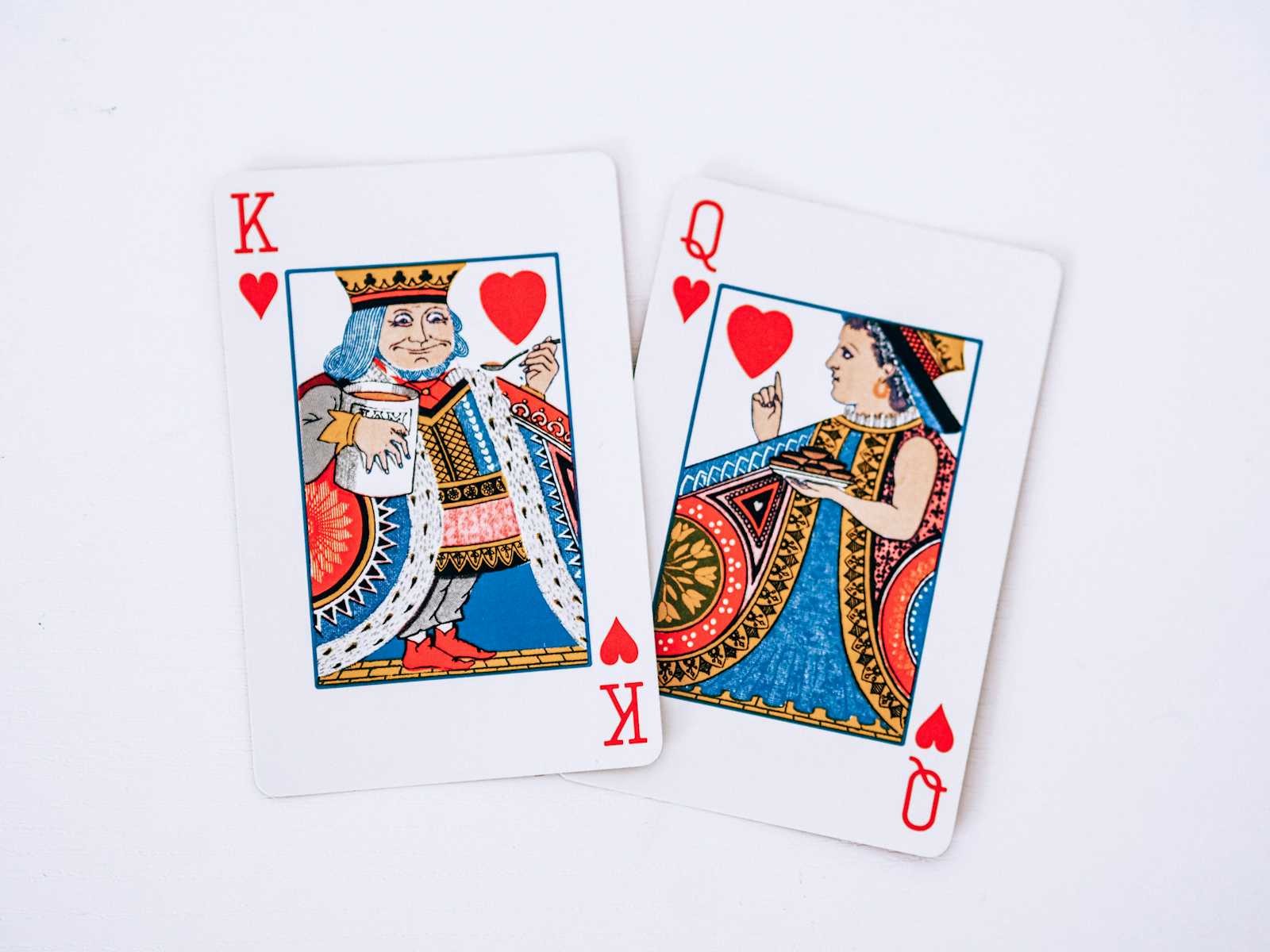
Using simple search
class Solution:
def isNStraightHand(self, hand: List[int], groupSize: int) -> bool:
# Check if divisible
if len(hand) % groupSize != 0:
return False
hand.sort()
# No. of groups
n = len(hand) // groupSize
def findAndRemove(num, c):
if c == groupSize:
return True
for e in hand:
if e - 1 == num:
hand.remove(e)
return findAndRemove(e, c + 1)
break
return False
for i in range(n):
first = hand.pop(0)
if not findAndRemove(first, 1):
return False
return True
Using dictionary
class Solution:
def isNStraightHand(self, hand: List[int], groupSize: int) -> bool:
# Check if divisible
if len(hand) % groupSize != 0:
return False
# Sort for order
hand.sort()
# No. of groups
n = len(hand) // groupSize
# Get counts
d = defaultdict(int)
for e in hand:
d[e] += 1
def findAndRemove(num, c):
if c == groupSize:
return True
count = d[num + 1]
if count > 0:
d[num + 1] -= 1
return findAndRemove(num + 1, c + 1)
return False
def findFirst():
for k, v in d.items():
if v > 0:
return k
for i in range(n):
first = findFirst()
d[first] -= 1
if not findAndRemove(first, 1):
return False
return True
0
Subscribe to my newsletter
Read articles from Tapan Rachchh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
