Higher Order Components in React
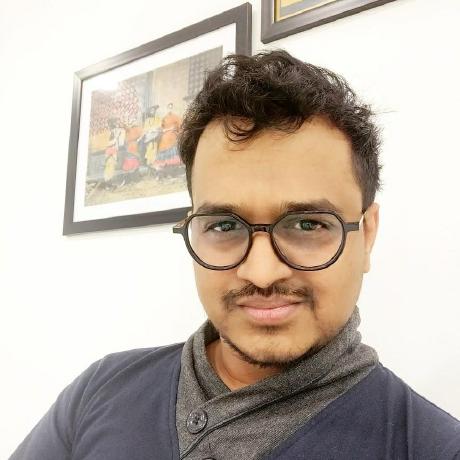
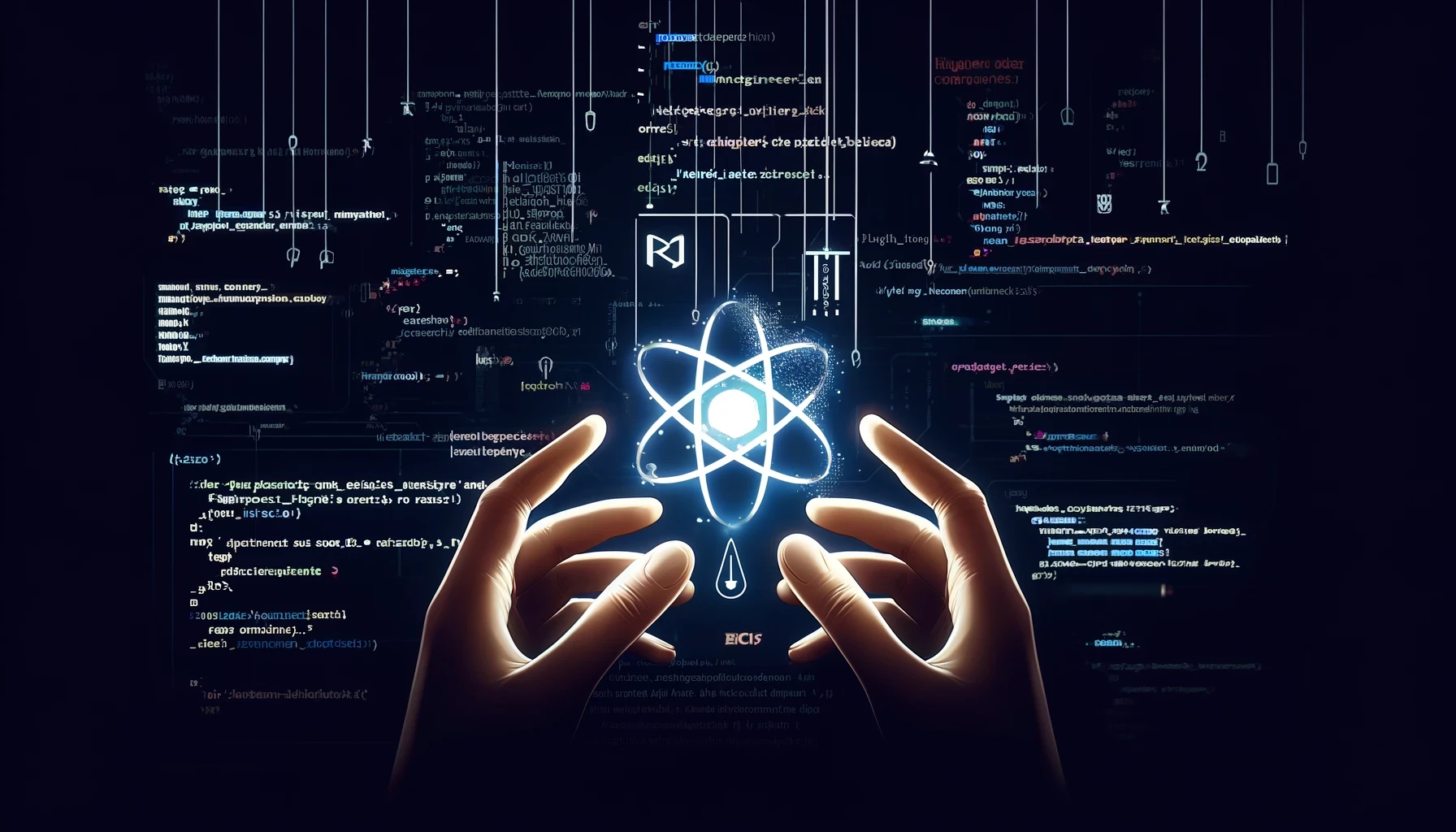
Introduction
React and its component-based architecture
React, a popular JavaScript library for building user interfaces, has revolutionized the way developers create web applications. One of the key features that makes React so powerful and efficient is its component-based architecture. This architectural pattern allows developers to break down complex user interfaces into smaller, reusable pieces called components.
The magic of React's component-based architecture lies in its ability to simplify the development process, enhance code reusability, and improve the overall maintainability of the application. By dividing the UI into self-contained components, developers can focus on building each component independently, making the code more modular and easier to understand.
Reusability: Components can be easily reused throughout the application, reducing code duplication and promoting consistency in the user interface [React Documentation - Components and Props].
Modularity: Each component encapsulates its own structure, logic, and styling, making the codebase more modular and easier to manage.
Composability: Components can be composed together to create more complex user interfaces, allowing for a hierarchical and intuitive structure.
Maintainability: With a component-based approach, fixing bugs and updating features becomes more targeted and efficient, as changes can be made to specific components without affecting the entire application.
Testability: Components can be individually tested, enabling developers to catch and fix issues early in the development process [Kent C. Dodds Video].
Higher Order Components (HOCs)
Definition and basic concept
Higher-Order Components (HOCs) are a technique in React for reusing component logic across multiple components. They are not part of the React API but rather a pattern that emerges from React's compositional nature. HOCs are essentially functions that take a component as an argument and return a new enhanced component with some additional functionality
The concept of Higher-Order Components is inspired by Higher-Order Functions in JavaScript, which are functions that either take one or more functions as arguments or return a function as a result.
Advantages of HOCs
Code reuse
HOCs allow you to reuse component logic across multiple components without modifying their implementation. This promotes code reuse and helps maintain a DRY (Don't Repeat Yourself) codebase.
Logic separation and abstraction
HOCs provide an abstraction layer that allows you to define common functionality in a single place and share it among multiple components. This abstraction improves code maintainability and readability.
Enhance functionality
HOCs can manipulate and enhance the props passed to the wrapped component. They can add, remove, or modify props before passing them down to the wrapped component.
Simple example of a HOC
Here's a simple example of a Higher-Order Component that adds a loading state to a component.
function withLoading(WrappedComponent) {
return class extends React.Component {
state = {
isLoading: true,
};
componentDidMount() {
// Simulating an async operation
setTimeout(() => {
this.setState({ isLoading: false });
}, 2000);
}
render() {
const { isLoading } = this.state;
return isLoading ? <div>Loading...</div> : <WrappedComponent {...this.props} />;
}
};
}
Real-world use case
Let's build a fancy rating HOC that adds a rating widget into a passed component.
const withRatingWidget = (WrappedComponent, options) => {
const { scale = 5, icon = "bar" } = options || {};
const RatingWidget = (props) => {
const [rating, setRating] = useState(0);
const handleRatingChange = (newRating) => {
setRating(newRating);
};
// index <= rating ? "fa-solid" : "fa-reglar"
const renderRatingIcon = (index) => {
if (icon === "star") {
return (
<i
className={`fa-star ${
index <= rating ? "fa-solid" : "fa-regular"
} `}
></i>
);
} else if (icon === "moon") {
return (
<i
className={`fa-moon ${index <= rating ? "fa-solid" : "fa-regular"}`}
></i>
);
} else {
const filledChar = "▓";
const unfilledChar = "▒";
if (index <= rating) {
return <span className="bar filled">{filledChar}</span>;
} else {
return <span className="bar unfilled">{unfilledChar}</span>;
}
}
};
const ratingIcons = Array.from({ length: scale }, (_, index) => (
<span
key={index}
className="rating-icon"
onClick={() => handleRatingChange(index + 1)}
>
{renderRatingIcon(index + 1)}
</span>
));
return (
<div>
<WrappedComponent {...props} />
<div className="rating-widget">
<h3>Rate this item:</h3>
<div className="rating-icons">{ratingIcons}</div>
<p>Your rating: {rating}</p>
</div>
</div>
);
};
return RatingWidget;
};
Now let's see a usage Example.
Word of Advice
While Higher-Order Components (HOCs) can be a powerful technique for reusing and composing functionality in React, they also have some drawbacks and potential issues to consider. Here are some of the cons of using Higher-Order Components:
Complexity and Readability:
HOCs introduce an additional level of abstraction and indirection, which can make the code more complex and harder to understand, especially for developers who are new to the codebase.
The usage of HOCs can lead to deeply nested component structures, making it challenging to trace the flow of props and understand the component hierarchy.
Overusing HOCs or creating complex HOC chains can reduce the readability and maintainability of the code.
Naming Collisions:
HOCs wrap the original component and pass down props. If the HOC and the wrapped component have props with the same name, it can lead to naming collisions and unexpected behavior.
To avoid naming collisions, developers need to be careful when choosing prop names and may need to use techniques like prop renaming or careful prop merging.
Debugging and Error Tracing:
When an error occurs within an HOC or a component wrapped by an HOC, the stack trace and error messages can be more difficult to interpret and debug.
The additional layers introduced by HOCs can make it harder to pinpoint the exact location of an error or understand the context in which it occurred.
Performance Overhead:
HOCs introduce additional components in the component tree, which can slightly impact performance, especially if the HOCs are complex or numerous.
Each HOC adds an extra layer of indirection, and the overhead of rendering and reconciling these additional components can accumulate, particularly in large and complex applications.
Testing Challenges:
Testing components wrapped with HOCs can be more challenging because the HOC introduces additional complexity and may require mocking or stubbing of the HOC's behavior.
The separation of concerns between the HOC and the wrapped component can make it harder to write isolated and focused tests for each component.
Ref Forwarding Limitations:
HOCs do not automatically forward refs to the wrapped component. If the wrapped component needs to expose a ref to its parent, additional configuration and techniques like
forwardRef
are required.This limitation can make it more challenging to work with refs and access the underlying DOM elements or component instances when using HOCs.
Static Composition:
HOCs are static in nature, meaning they are applied to components at the time of definition rather than dynamically at runtime.
This static composition can limit the flexibility and adaptability of the components, as the HOC's functionality is determined at the time of component creation and cannot be easily modified or removed later.
Despite these drawbacks, Higher-Order Components can still be a valuable pattern when used judiciously and in appropriate situations. It's important to weigh the benefits against the potential downsides and consider alternative patterns like Render Props or Hooks when they may be more suitable for the specific use case.
As with any programming pattern, it's crucial to use HOCs thoughtfully, keep the component hierarchy manageable, and prioritize code readability and maintainability.
Subscribe to my newsletter
Read articles from Jagadish K. directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
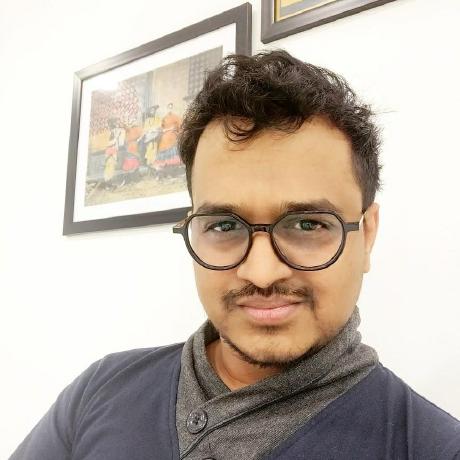
Jagadish K.
Jagadish K.
I have been fiddling with HTML,CSS and Javascript for about 15 years. In other words, I am almost ancient. I enjoy solving problems and coming up with creatie solutions in given constraints.