Understanding Access Tokens and Refresh Tokens
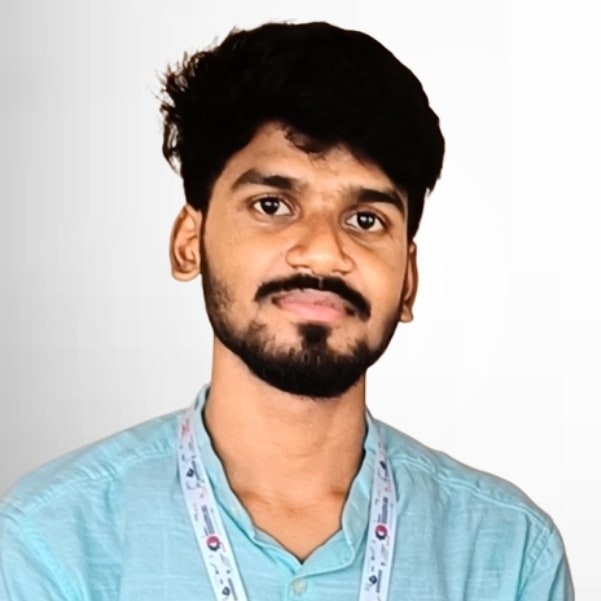
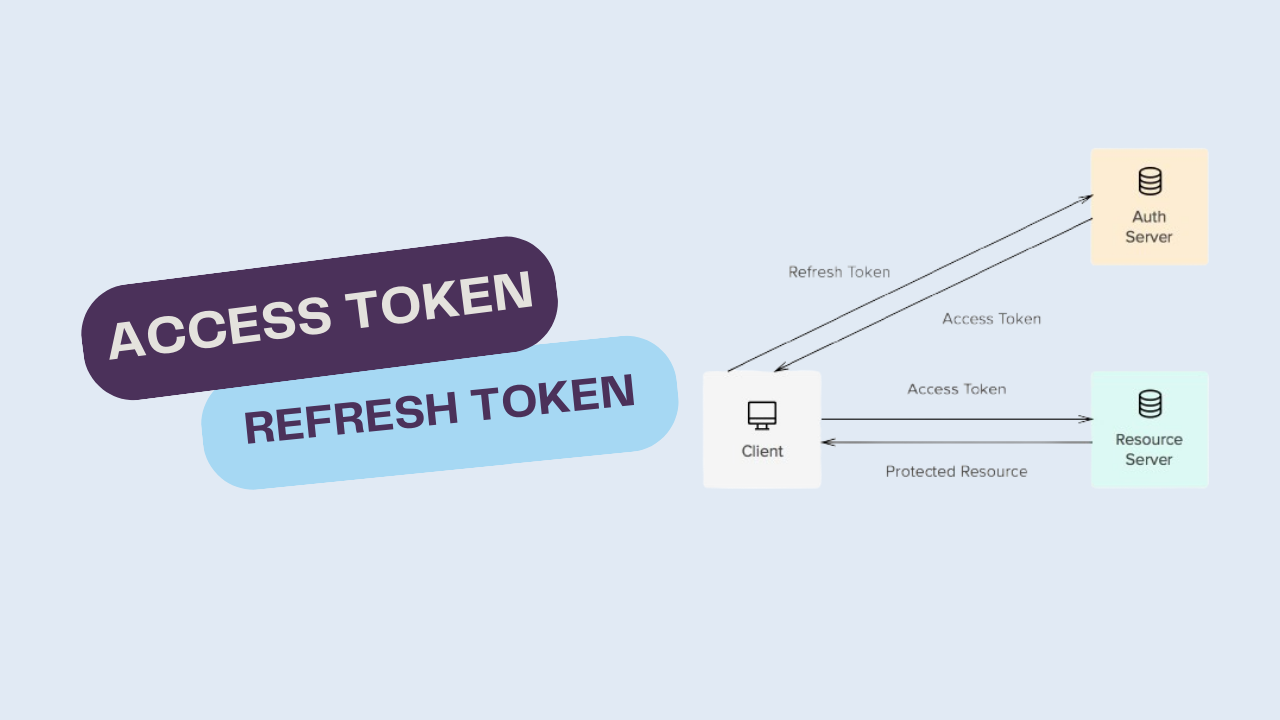
In modern web and mobile applications, security is a paramount concern. A significant part of securing applications involves managing user authentication and authorization effectively. Two critical components in this process are access tokens and refresh tokens. This article explores what these tokens are, their use cases, and the differences between them.
What Are Access Tokens?
Access tokens are short-lived tokens used to access protected resources. When a user logs in, the authentication server generate an access token, which the client (e.g., a web application or mobile app) can use to authenticate subsequent requests to the server. The access token typically contains encoded information, such as the user's identity and permissions, and is often implemented using JSON Web Tokens (JWT).
Characteristics of Access Tokens:
Access tokens have a limited lifetime, usually ranging from a few minutes to a few hours.
The token is passed with each request to authenticate the user.
The server does not need to store the token; instead, it validates the token on each request.
What Are Refresh Tokens?
Refresh tokens are long-lived tokens used to obtain new access tokens once the original access token expires. When the access token expires, the client can send the refresh token to the authentication server to request a new access token without requiring the user to re-authenticate.
Characteristics of Refresh Tokens:
Refresh tokens have a longer lifespan, typically lasting days, weeks, or even months.
Refresh tokens must be stored securely, usually in HTTP-only cookies or secure storage mechanisms.
The token is exchanged for a new access token and potentially a new refresh token, making it less vulnerable to replay attacks.
Use Cases for Access and Refresh Tokens
Access Tokens
Single Sign-On (SSO):
- Allow users to authenticate once and access multiple related services without re-authenticating.
APIs:
- Secure API endpoints by requiring clients to include access tokens in their requests.
Mobile and Web Applications:
- Maintain user sessions by passing access tokens with each request to access user-specific data and functionality.
Refresh Tokens
Session Management:
- Maintain user sessions without requiring frequent re-authentication, enhancing user experience.
Token Rotation:
- Implement token rotation strategies to improve security by issuing new tokens regularly and invalidating old ones.
Improved Security:
- Reduce the risk of long-term exposure by using short-lived access tokens while maintaining session persistence with refresh tokens.
Differences Between Access Tokens and Refresh Tokens
Feature | Access Token | Refresh Token |
Lifespan | Short (minutes to hours) | Long (days to months) |
Usage | Sent with each request to access resources | Used to obtain new access tokens |
Storage | Client-side (localStorage, cookies) | Secure storage (HTTP-only cookies, secure storage) |
Security | More frequent exposure (each request) | Less frequent exposure (only during refresh) |
Token Rotation | Not typically used for rotation | Used to rotate access tokens for improved security |
Implementing Access and Refresh Tokens
To implement access and refresh tokens, follow these steps:
User Authentication:
- Authenticate the user and issue an access token and a refresh token upon successful login.
Token Storage:
Store the access token in memory or a non-persistent storage mechanism.
Store the refresh token in a secure manner, such as an HTTP-only cookie.
Request Handling:
Attach the access token to each request that requires authentication.
Verify the access token on the server to grant access to protected resources.
Token Refresh:
When the access token expires, send the refresh token to the server to obtain a new access token.
Optionally, issue a new refresh token and invalidate the old one to enhance security.
Logout:
- Invalidate both the access token and the refresh token upon user logout.
Code Sample for Generating Access and Refresh Tokens
// User model methods
UserSchema.methods.generateAccessToken = function() {
try {
return jwt.sign({ id: this._id }, process.env.ACCESS_TOKEN_SECRET, { expiresIn: '15m' });
} catch (error) {
console.error("Error generating access token:", error);
throw new Error("Token generation failed");
}
};
UserSchema.methods.generateRefreshToken = function() {
try {
return jwt.sign({ id: this._id }, process.env.REFRESH_TOKEN_SECRET, { expiresIn: '7d' });
} catch (error) {
console.error("Error generating refresh token:", error);
throw new Error("Token generation failed");
}
};
const generateAccessAndRefreshToken = async (userId) => {
try {
const user = await User.findById(userId);
if (!user) {
console.error(`User not found for ID: ${userId}`);
throw new ApiError(404, "User not found");
}
const accessToken = user.generateAccessToken();
const refreshToken = user.generateRefreshToken();
if (!accessToken) {
console.error("Failed to generate access token");
throw new ApiError(500, "Failed to generate access token");
}
if (!refreshToken) {
console.error("Failed to generate refresh token");
throw new ApiError(500, "Failed to generate refresh token");
}
user.refreshToken = refreshToken;
await user.save({ validateBeforeSave: false }).catch(err => {
console.error("Error saving user with refresh token:", err);
throw new ApiError(500, "Failed to save refresh token to user");
});
return { accessToken, refreshToken };
} catch (error) {
console.error('Detailed error in generateAccessAndRefreshToken:', error);
throw new ApiError(500, "Something went wrong while generating refresh and access token");
}
};
Conclusion
Access tokens and refresh tokens are essential components of modern authentication and authorization mechanisms. Access tokens provide a secure way to authenticate user requests without burdening the server with session management, while refresh tokens allow for seamless session persistence and improved security. Understanding their differences and use cases helps developers implement robust security strategies in their applications.
By leveraging access and refresh tokens effectively, developers can enhance the security, performance, and user experience of their applications, ensuring a smooth and secure interaction for users.
Subscribe to my newsletter
Read articles from KIRTI KAMAL directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
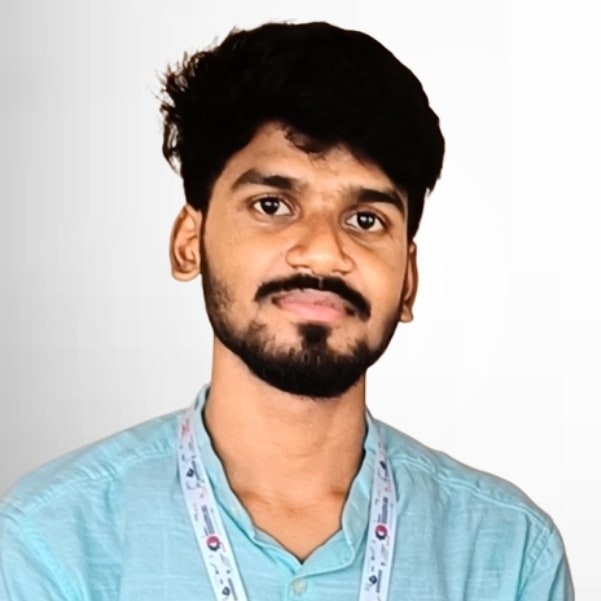
KIRTI KAMAL
KIRTI KAMAL
Kirti Kamal is a tech enthusiast and visionary community leader who is pursuing a Bachelor of Technology in Computer Science and Engineering at Trident Academy of Technology. As a Beta Microsoft Learn Student Ambassador, Kirti helps fellow students learn new technologies and develop their career skills for the future. Kirti also leads the Trident ACM Student Chapter and the Trident Hackathon Club, organizing engaging workshops, events, and hackathons for the tech community. With a strong dedication to open-source, Kirti has established an inclusive platform to guide newcomers through their tech journey. Kirti has also acquired multiple certifications and skills in DevOps, Networking, Linux, Docker, and Frontend Development, demonstrating technical expertise and a passion for continuous learning. Kirti strives to inspire others to explore, innovate, and excel in the ever-evolving realm of technology, by blending leadership skills, collaboration, and creativity.