Effective JavaScript Validation: Using Chaining, some, and trim method
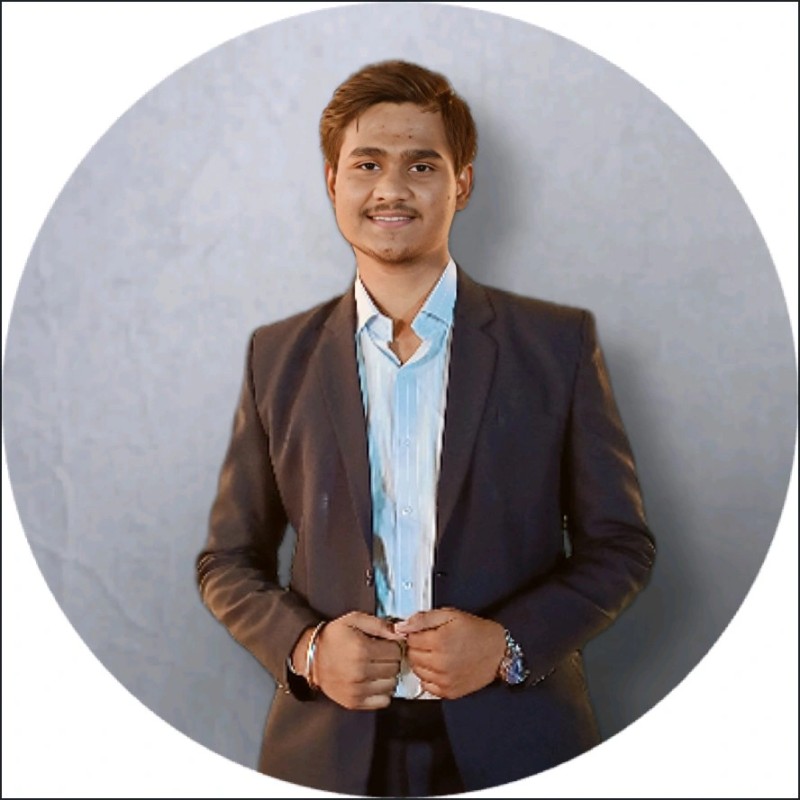
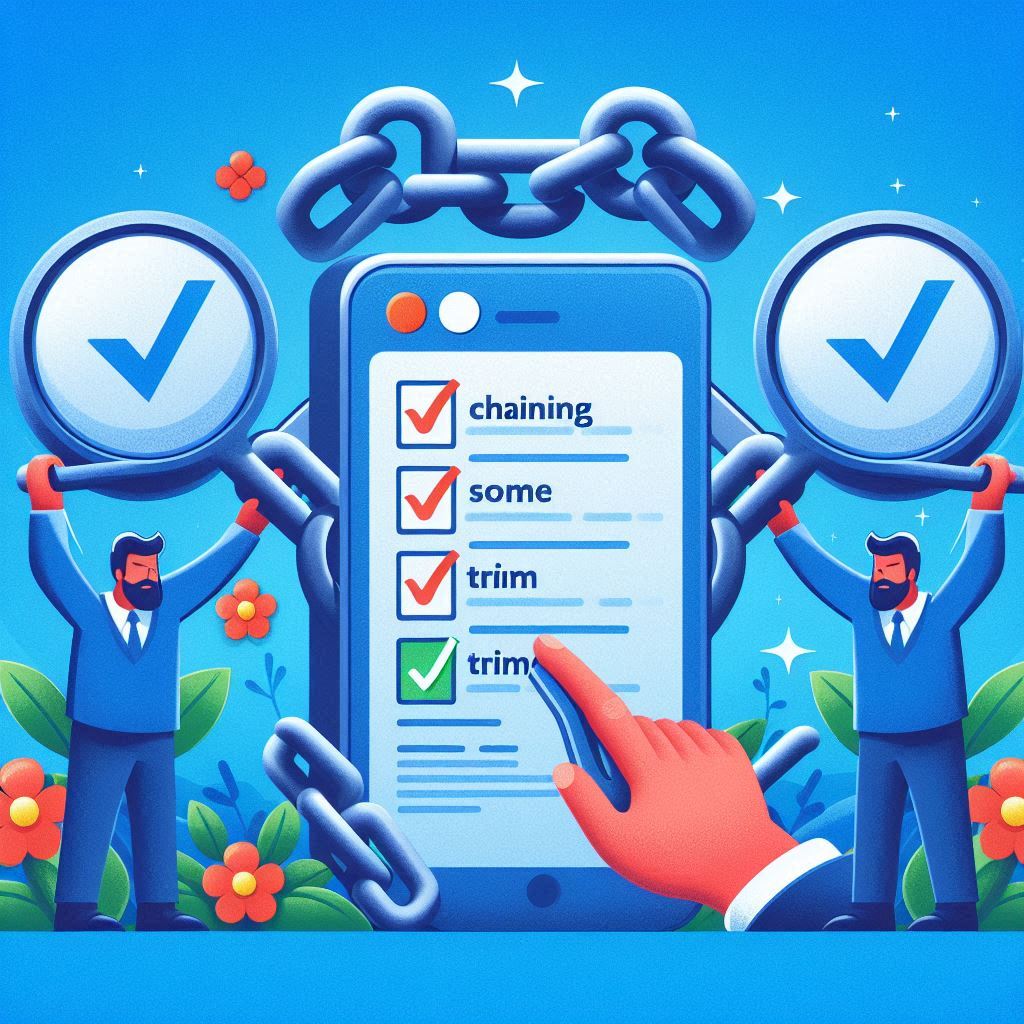
Validating User Input in JavaScript: Ensuring All Fields Are Filled
In web development, ensuring that all required fields are filled out correctly by the user is a crucial step. Let's explore how to perform this validation in JavaScript using a concise and effective method. We will break down the following code snippet to understand its functionality step-by-step:
javascriptCopy codeif (
[fullName, email, username, password].some((field) => field?.trim() === "")
) {
throw new ApiError(400, "All fields are required");
}
The Context
Imagine you are building a registration form that collects the user's full name, email, username, and password. These fields are essential, and you need to ensure that none of them are left blank or filled with only whitespace.
Step-by-Step Breakdown
Step 1: Creating an Array of Fields
The first part of the code creates an array containing the values of the fields to be validated:
javascriptCopy code[fullName, email, username, password]
This array holds the variables fullName
, email
, username
, and password
. Let's assume these variables hold the following values:
javascriptCopy codeconst fullName = "John Doe";
const email = "john.doe@example.com";
const username = " ";
const password = "password123";
Here, the username
field is intentionally set to a space to simulate an input error.
Step 2: Using the some
Method
The .some()
method is called on the array to check if at least one element meets a specific condition:
javascriptCopy code.some((field) => field?.trim() === "")
Understanding .some()
The
.some()
method tests whether at least one element in the array passes the provided function. It returnstrue
if any element passes the test, andfalse
otherwise.The function provided to
.some()
is an arrow function that performs the following check for each field:
javascriptCopy code(field) => field?.trim() === ""
Step 3: Using the Arrow Function
Let's dissect the arrow function:
javascriptCopy code(field) => field?.trim() === ""
field?.trim()
: This uses optional chaining (?.
) to safely call the.trim()
method onfield
. Optional chaining ensures that iffield
isnull
orundefined
, it doesn't cause an error. Instead, it returnsundefined
, and the.trim()
method is not called..trim()
: This method removes whitespace from both ends of a string. For example:javascriptCopy code" hello ".trim() // returns "hello" " ".trim() // returns ""
field?.trim() === ""
: This condition checks if the trimmed value offield
is an empty string. Iffield
isnull
,undefined
, or a string consisting only of whitespace characters,field?.trim() === ""
will betrue
.
Step 4: Applying the Check
The .some()
method applies the arrow function to each element in the array [fullName, email, username, password]
. Let's see how this works for each field in our example:
For
fullName = "John Doe"
:javascriptCopy code"John Doe".trim() === "" // false
For
email = "
john.doe@example.com
"
:javascriptCopy code"john.doe@example.com".trim() === "" // false
For
username = " "
:javascriptCopy code" ".trim() === "" // true
For
password = "password123"
:javascriptCopy code"password123".trim() === "" // false
Since the username
field results in true
, the entire .some()
method call returns true
.
Step 5: Throwing an Error
The if
statement checks the result of the .some()
method:
javascriptCopy codeif ([fullName, email, username, password].some((field) => field?.trim() === "")) {
throw new ApiError(400, "All fields are required");
}
Because
.some()
returnstrue
(as theusername
field is invalid), the condition inside theif
statement istrue
.Consequently, an
ApiError
with a 400 status code and the message "All fields are required" is thrown. This indicates that the form submission or input validation failed due to one or more required fields being empty or containing only whitespace.
Conclusion
This code snippet provides a powerful and concise way to validate that all required fields in a form are filled out correctly. By creating an array of fields and using the .some()
method with an appropriate check function, you can quickly identify any empty or whitespace-only inputs. This ensures robust input validation in your JavaScript applications, improving the overall user experience and data integrity
Connect : /https://linktr.ee/Akash_11
Subscribe to my newsletter
Read articles from Akash Satpute directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
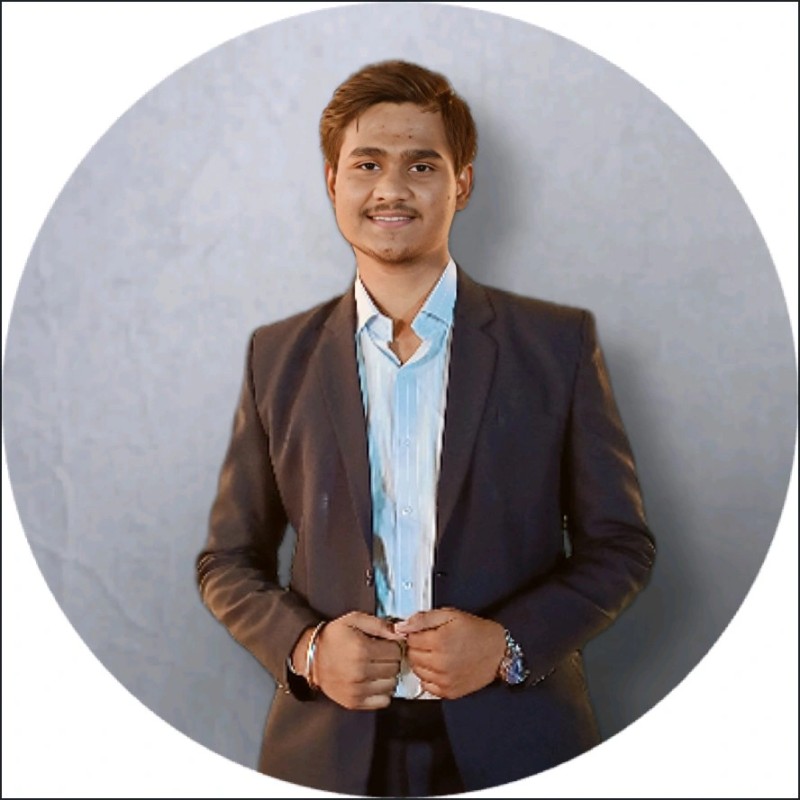