Creating a Simple Snake Game in C
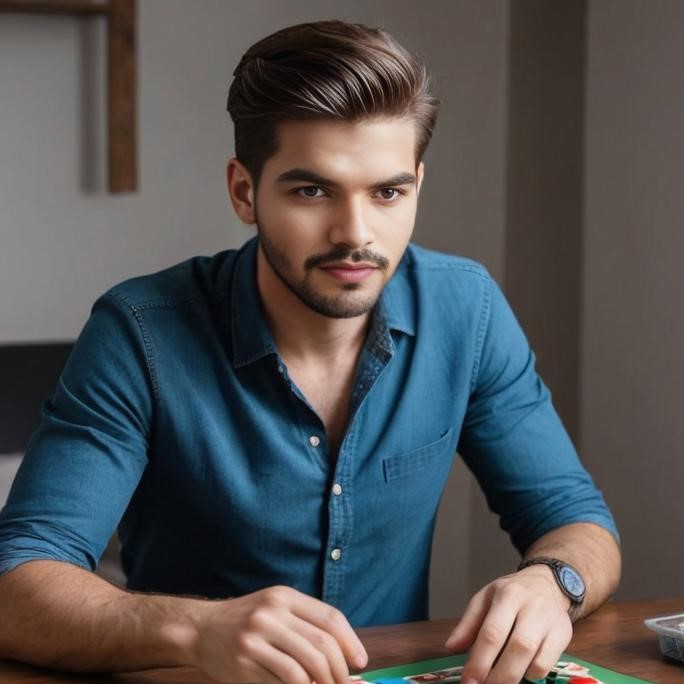
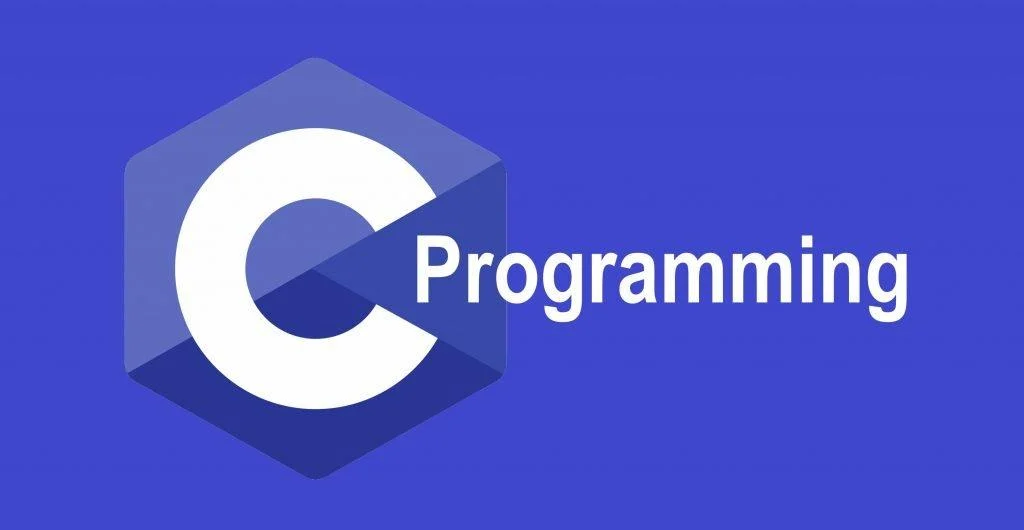
Introduction
Introduce the concept of the Snake game and its popularity as a classic arcade game. Explain that the blog will guide readers through creating a basic version of the Snake game in C.
Code Overview
Explain the structure of the code and its key components:
Setup: Initialize game variables such as snake position, length, food position, and gameover status.
Draw: Display the game screen, including the snake, food, walls, and game instructions.
Input: Handle user input to control the snake's movement using WASD or arrow keys.
Logic: Implement game logic for snake movement, food consumption, collision detection with walls, and self-collision detection.
Main Function: Manage the game loop and interactions between setup, draw, input, and logic functions.
Functions
Setup
Initialize game variables such as snake position, length, food position, and gameover status.
c
void setup() {
// Implementation for game setup
}
Draw
Display the game screen, including the snake, food, walls, and game instructions.
c
void draw() {
// Implementation for drawing game screen
}
Input
Handle user input to control the snake's movement using WASD or arrow keys.
c
void input() {
// Implementation for handling user input
}
Logic
Implement game logic for snake movement, food consumption, collision detection with walls, and self-collision detection.
c
void logic() {
// Implementation for game logic
}
Main Function
Contains the game loop to continuously update and render the game until the gameover condition is met.
c
int main() {
// Main function code
}
Explanation
Setup: Initializes game variables and positions for starting the game.
Draw: Displays the game screen with the snake, food, walls, and instructions.
Input: Handles user input to control the snake's movement.
Logic: Implements game rules for snake movement, food consumption, and collision detection.
Main Function: Manages the game loop and interactions between different game components.
Conclusion
Summarize the implementation of the Snake game in C and encourage readers to explore further enhancements and features.
Final Thoughts
Encourage readers to experiment with adding features like score tracking, levels, and graphical improvements to enhance the game experience.
This structure provides a clear explanation of the Snake game code and its functionality, making it informative and accessible for readers interested in game development in C programming.
Output:
Subscribe to my newsletter
Read articles from Ashutosh Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
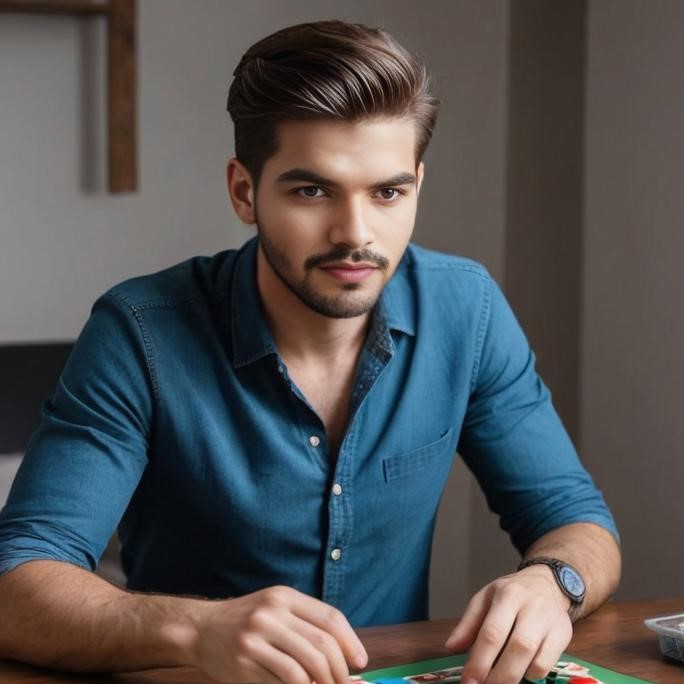
Ashutosh Singh
Ashutosh Singh
A Creator & Learner who like to share her learning, knowledge and creativity.