Concurrency in Golang: Simple Explanations of Mutex, Select, and WaitGroup
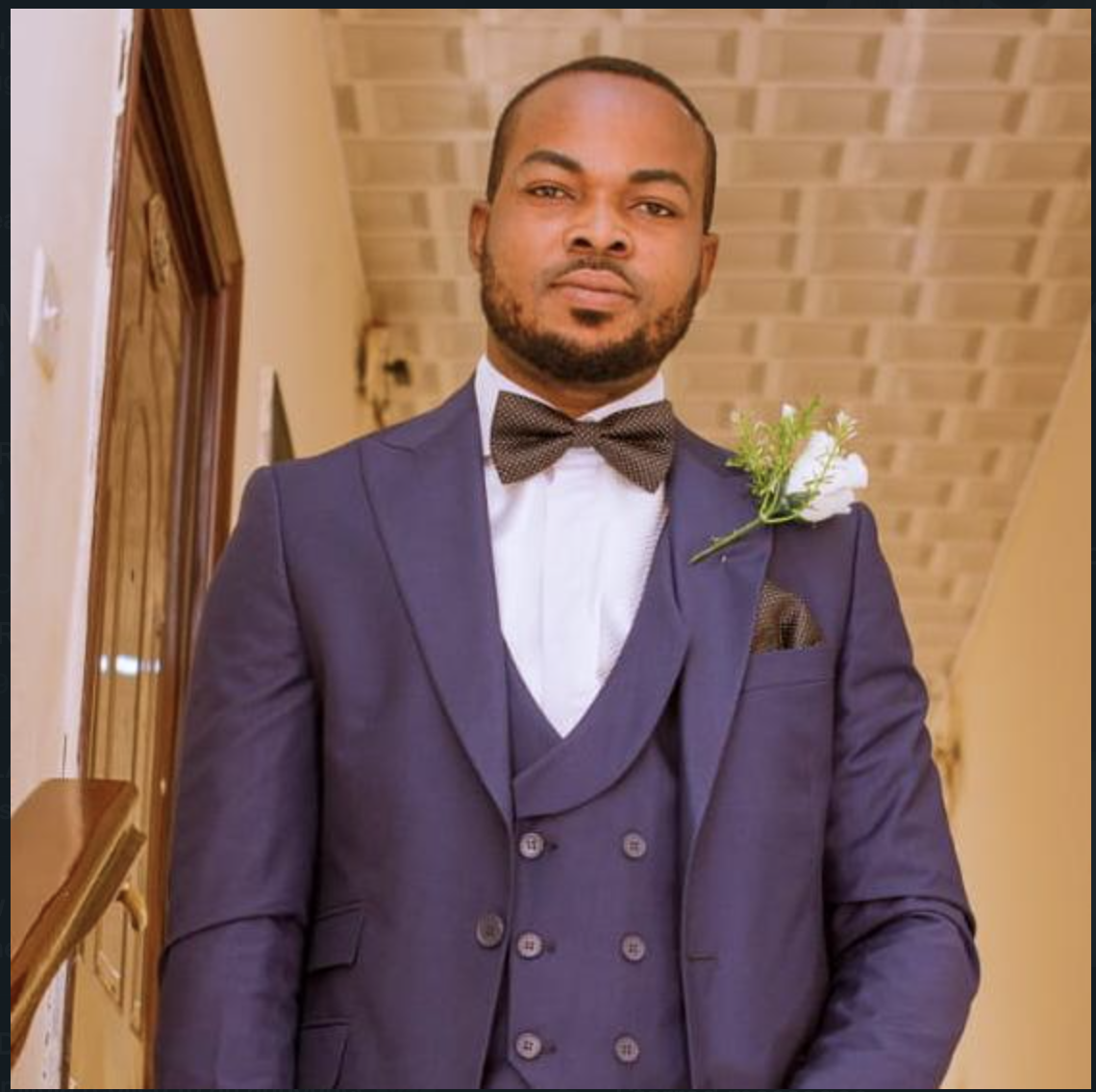
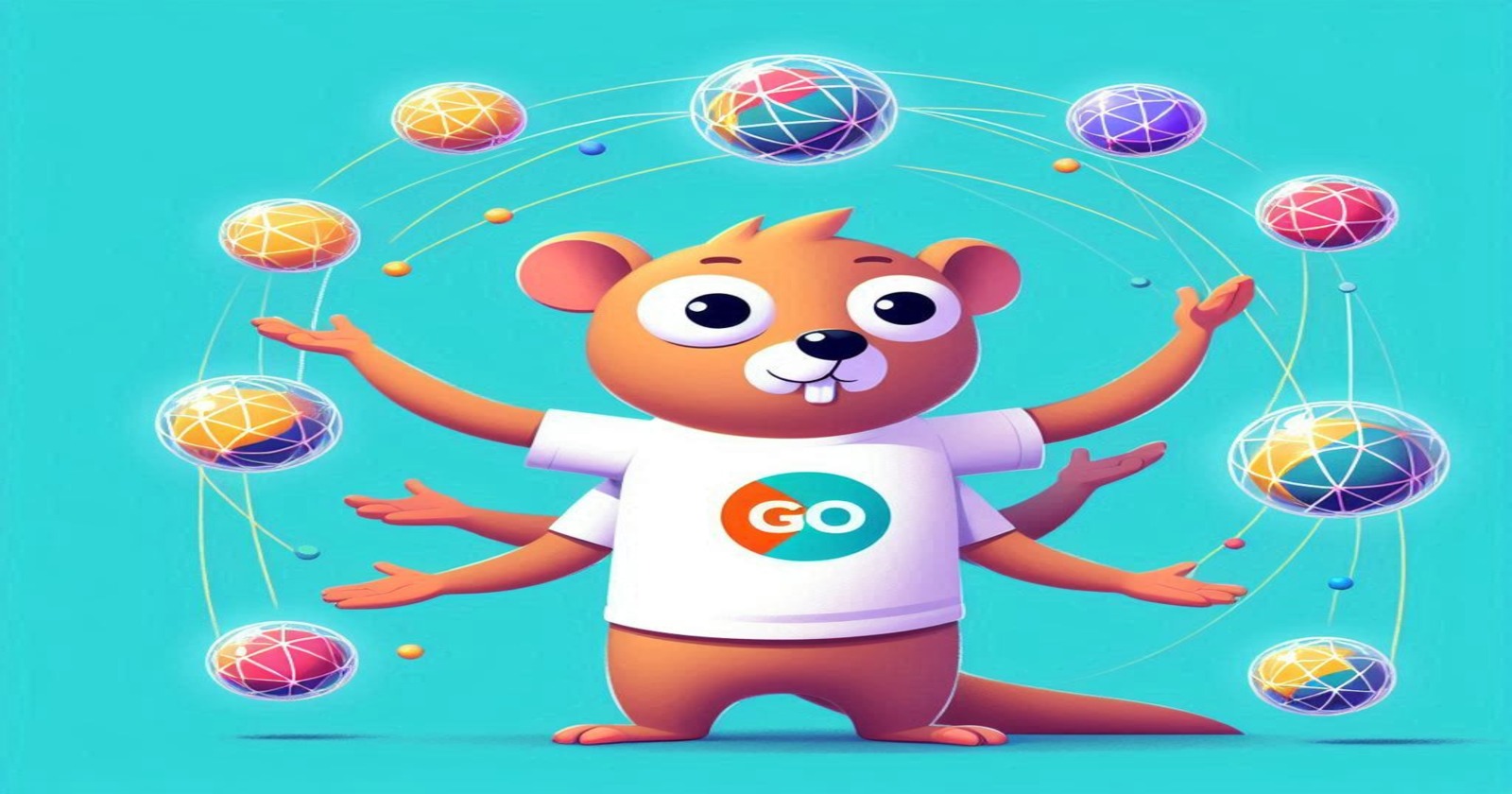
Today, we’ll talk about three important concepts: Mutex, Select, and WaitGroup. Let’s break them down in a way that’s fun and easy to understand.
1. What is a Mutex?
Imagine you and your friends are baking cookies in the kitchen. There’s only one oven, so only one person can use it at a time. A Mutex (short for mutual exclusion) in Go is like a sign on the oven that says, "In Use." When one person starts baking, they put up the sign so no one else can use the oven until they’re done. This prevents chaos and ensures the cookies bake perfectly.
In Go, a Mutex helps prevent multiple parts of a program from messing up shared resources (like variables or files) by making sure only one part of the program can access them at a time.
Here’s how you can use a Mutex in Go:
package main
import (
"fmt"
"sync"
)
var counter = 0
var mutex = &sync.Mutex{}
func increment() {
mutex.Lock() // Lock the mutex
counter++
mutex.Unlock() // Unlock the mutex
}
func main() {
var wg sync.WaitGroup
for i := 0; i < 10; i++ {
wg.Add(1)
go func() {
defer wg.Done()
increment()
}()
}
wg.Wait()
fmt.Println("Final Counter:", counter)
}
The reason for using a mutex in the code above is to make sure that only one goroutine can access the counter variable at a time. This is important because if multiple goroutines try to increment the counter at the same time, it could cause a race condition. A race condition happens when the result of a program depends on the timing of events. In this code, a race condition could occur if two goroutines try to read, increment, and write back the counter value at the same time. If this happens, one goroutine's update could overwrite the other's, leading to an incorrect counter value.
By using a mutex, we make sure that only one goroutine can access the counter variable at a time. This means only one goroutine can run the critical section (the code protected by the mutex) at once. This keeps the counter safe and ensures it is incremented correctly.
2. What is Select?
Think of Select like a switchboard operator who connects phone calls. It helps you listen to multiple channels (or Go routines) and picks the one that’s ready to talk first. This is super useful when you have different tasks that might finish at different times, and you want to handle them as soon as they’re ready.
Here’s an example using Select in Go:
package main
import (
"fmt"
"time"
)
func main() {
ch1 := make(chan string)
ch2 := make(chan string)
go func() {
time.Sleep(1 * time.Second)
ch1 <- "Hello from channel 1"
}()
go func() {
time.Sleep(2 * time.Second)
ch2 <- "Hello from channel 2"
}()
for i := 0; i < 2; i++ {
select {
case msg1 := <-ch1:
fmt.Println(msg1)
case msg2 := <-ch2:
fmt.Println(msg2)
}
}
}
Why Use select
?
Concurrent Waiting on Multiple Channels:
The
select
statement allows the program to wait for messages from eitherch1
orch2
without knowing which one will receive a message first. This is essential in concurrent programming where the exact timing of message arrivals is uncertain.Without
select
, you would need to wait on one channel at a time, potentially blocking and missing messages from the other channel.
Non-blocking Communication:
select
helps to handle channels in a non-blocking way. It listens to all specified channels and proceeds with the case that is ready. If both channels are ready, one of the cases is chosen at random.
Simplified Code for Handling Multiple Channels:
- Using
select
simplifies the logic for handling multiple channels. It reduces the need for complex synchronization and ensures that the program can react to multiple asynchronous events efficiently.
- Using
3. What is a WaitGroup?
A WaitGroup in Go is like a team leader who makes sure everyone on the team finishes their task before the team can move on to the next activity. It’s a way to keep track of a bunch of Go routines (small functions running at the same time) and wait for all of them to finish.
Here’s how you can use a WaitGroup in Go:
package main
import (
"fmt"
"sync"
"time"
)
func worker(id int, wg *sync.WaitGroup) {
defer wg.Done()
fmt.Printf("Worker %d starting\n", id)
time.Sleep(time.Second)
fmt.Printf("Worker %d done\n", id)
}
func main() {
var wg sync.WaitGroup
for i := 1; i <= 3; i++ {
wg.Add(1)
go worker(i, &wg)
}
wg.Wait()
fmt.Println("All workers done")
}
Why Use WaitGroup
?
Synchronization:
WaitGroup
is used to synchronize the completion of multiple goroutines. It ensures that themain
function waits until all worker goroutines have finished their work before proceeding.
Tracking Goroutine Completion:
wg.Add(1)
increments the counter to indicate that a new goroutine is starting.wg.Done()
decrements the counter when a goroutine completes its work.wg.Wait()
blocks the calling goroutine (in this case, themain
function) until the counter becomes zero, meaning all tracked goroutines have completed.
Concurrency Management:
Without
WaitGroup
, themain
function could exit before the worker goroutines finish their tasks, resulting in incomplete execution and potentially losing output.WaitGroup
provides a simple and effective way to manage concurrency, ensuring that the main program waits for all concurrent tasks to complete before proceeding.
Wrapping Up
In this article, we’ve covered:
Mutex: Keeping things orderly by making sure only one thing happens at a time.
Select: Handling multiple tasks by listening to whichever one is ready first.
WaitGroup: Making sure everyone finishes their task before moving on.
These tools help make Go a powerful language for handling multiple tasks at once, making your programs run faster and smoother. Happy coding! 🚀
Subscribe to my newsletter
Read articles from Oluwajuwon Falore directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
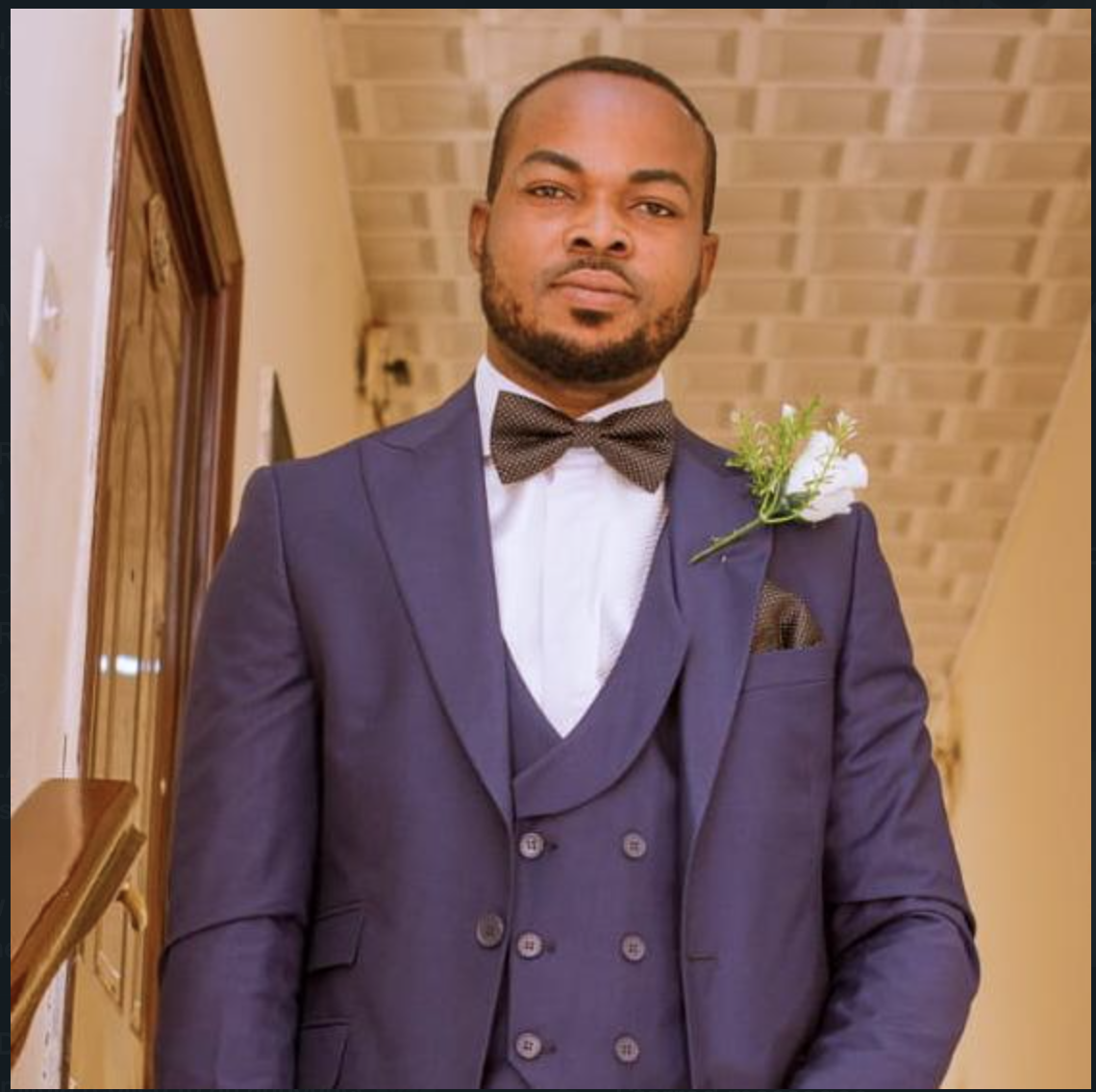
Oluwajuwon Falore
Oluwajuwon Falore
I am a full-Stack (backend leaning) software developer. Experienced with all stages of the development cycle for dynamic web projects. Well-versed in programming languages including HTML5, CSS, JAVASCRIPT, NODEJS, GOLANG, REACTJS, PYTHON, ANGULAR and IONIC.