Var, let and const
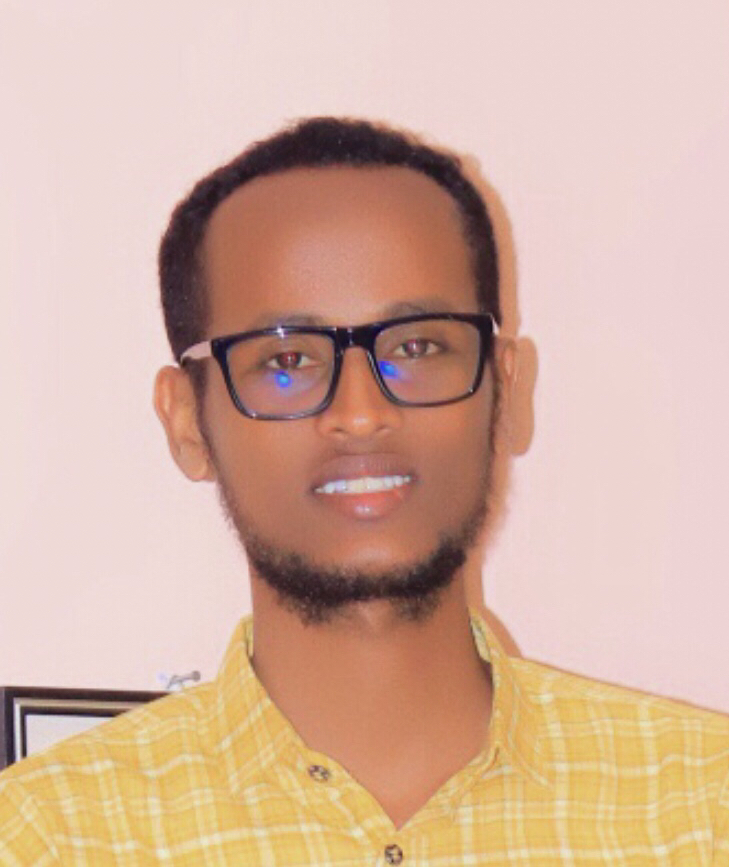
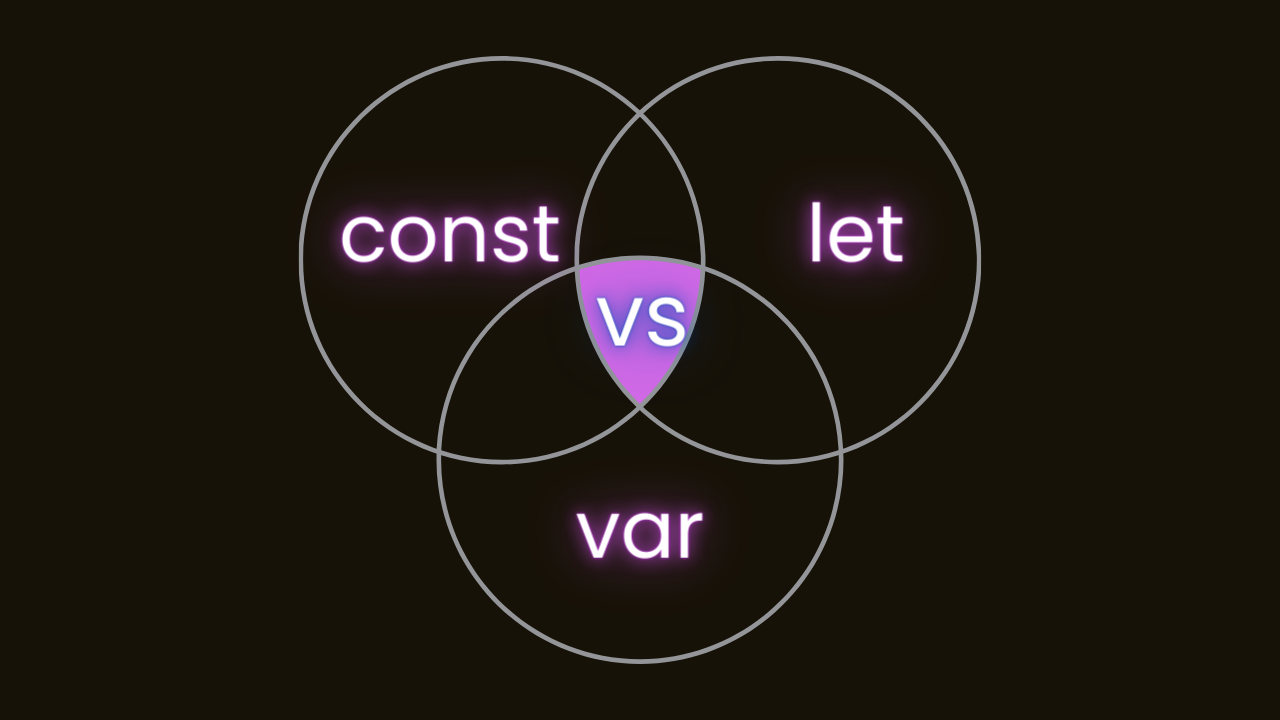
JavaScript provides three primary ways to declare variables: var
, let
, and const
. While they may seem similar at first glance, each has distinct characteristics and use cases.
Each one of these has its own rule, and if you understand them well, it may help to avoid mistakes.
var:
var
was the original way to declare variables in JavaScript. It has function scope, meaning variables declared with var
are accessible throughout the entire function they are declared in, regardless of block scope.
Example:
function exampleFunction() {
if (true) {
var num = 24;
}
console.log(num); // Output: 24
}
exampleFunction();
let:
Introduced in ES6 (ECMAScript 2015), let
allows block scoping. Variables declared with let
are limited to the block (enclosed by {}
) in which they are defined.
Example:
function exampleFunction() {
if (true) {
let num = 24;
console.log(num); // Output: 24
}
console.log(num); // Error: num is not defined
}
exampleFunction();
const:
Similar to let
, const
was also introduced in ES6. However, const
is used to declare constants whose values cannot be reassigned after initialization. Like let
, const
also has block scope.
Example:
function exampleFunction() {
const name = 'Ali';
console.log(name); // Output: Ali
name = 'John'; // Error: Assignment to constant variable
}
exampleFunction();
Conclusion
Understanding the differences between var
, let
, and const
is crucial for writing clean and maintainable JavaScript code. Use var
sparingly due to its lack of block scope, prefer let
for variables that may need to be reassigned, and use const
for variables that should remain constant throughout the program.
Thank you. please share to others.
Subscribe to my newsletter
Read articles from Mohamed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
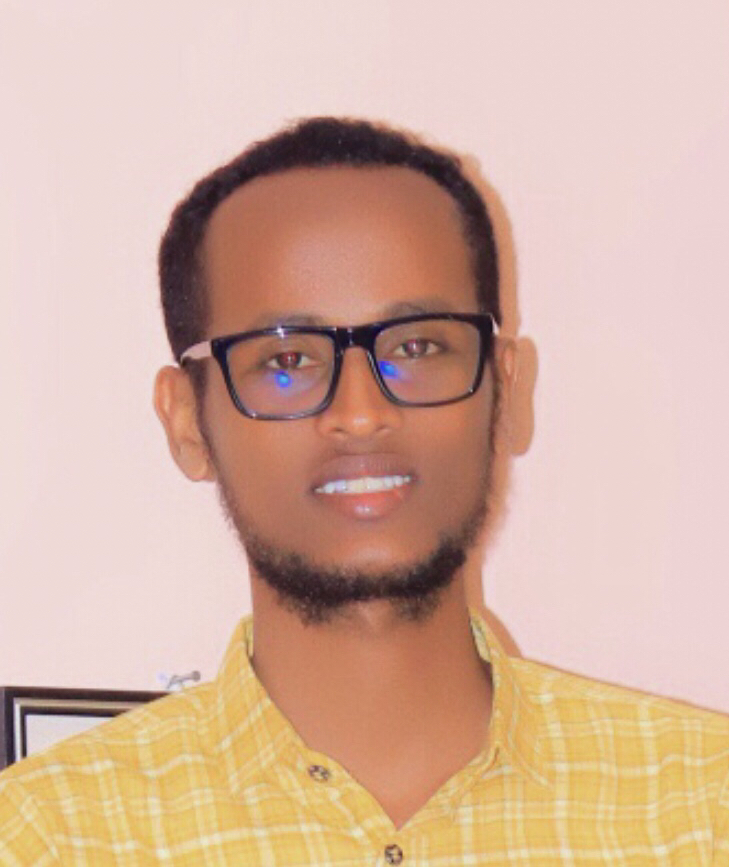
Mohamed
Mohamed
I am Mohamed and I am website designer and developer.