Introduction to Objects
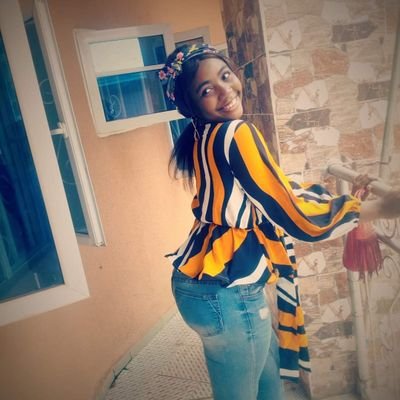
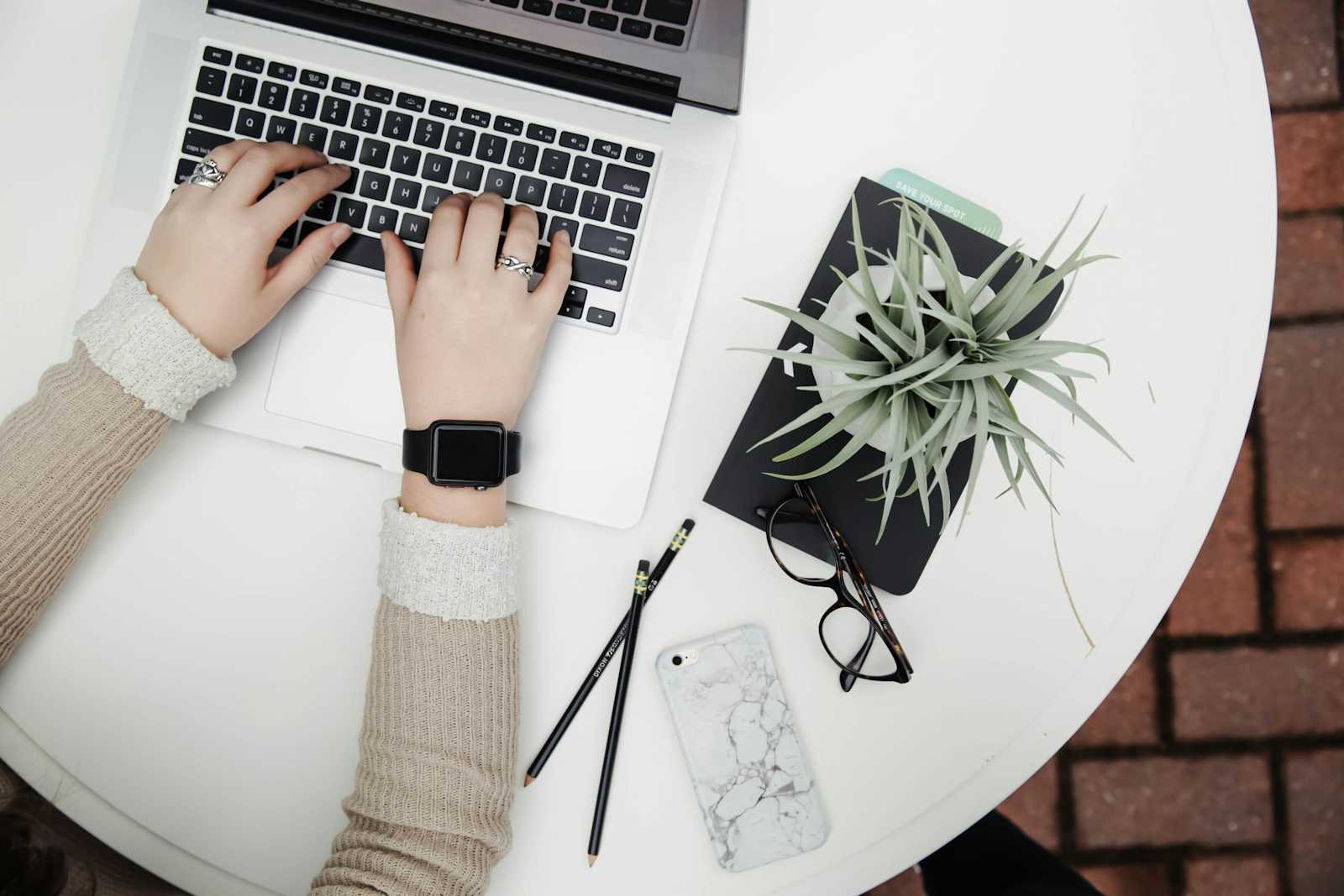
In the realm of programming, objects are important because they serve as a fundamental concept in object-oriented programming (OOP), a widely used paradigm in software development.
In this article, I am going to explain to you what objects in programming entail, how to create them, and their uses.
Pre-requiste
Before diving into the concept of objects, it's helpful to have a foundation in HTML, CSS, and basic JavaScript.
What are objects?
Objects can be defined as a collection of related properties. For example, if you want to build a character in a game, the character will possess certain characteristics or traits. The character is the object, and the character's characteristics and traits are its properties.
Creating Objects
One of the most common ways in which we can create objects in JavaScript is by using the object literal syntax {}, which means assigning an opening and a closing curly brace to the object you want to create.
To do this, we have to first save an empty object literal to a variable
//creating an object using object literal syntax. The object is teacher
var teacher = {};
As the object has been created, we can now start adding properties to it using the dot notation "." and use the assignment operator "=" to add values to those properties; for example,
teacher.greeting = "Good morning Students";
teacher.health = 100;
teacher.dressing = "Long gown"
So when we want to check our teacher object, we can simply console log the entire object
console.log(teacher);
Another method of creating an object is by listing the properties inside the object literal. For example, we want to create an object called a doctor and add its properties.
var doctor = {
health : 90,
patients : 56,
maintainence : 40
}
It is important to note that each property is followed by a colon, and its values are separated by a comma.
You can also update objects created using this format by using the dot notation
doctor.medicine = "fully booked";
Bracket Notation
An alternative syntax to the dot notation is the bracket notation. The only difference is that this time around, instead of using dots, I will be using brackets.
var teacher = {};
teacher["greeting"] = "Good morning Students";
teacher["health"] = 100;
teacher["dressing"] = "Long gown"
console.log(teacher);
You can both access and update properties on obects using either the dot notation, the bracket notation, or a combination of both.
One of the advantages of using the bracket notation over the dot notation is that with the brackets notation, you can add space characters inside the property name
teacher["number of subjects"] = 6;
Subscribe to my newsletter
Read articles from Chinonye Precious directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
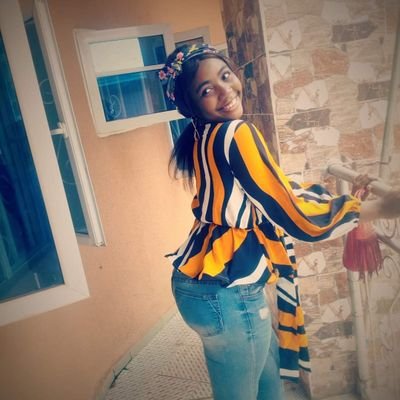