Building a Number Guessing Game in C
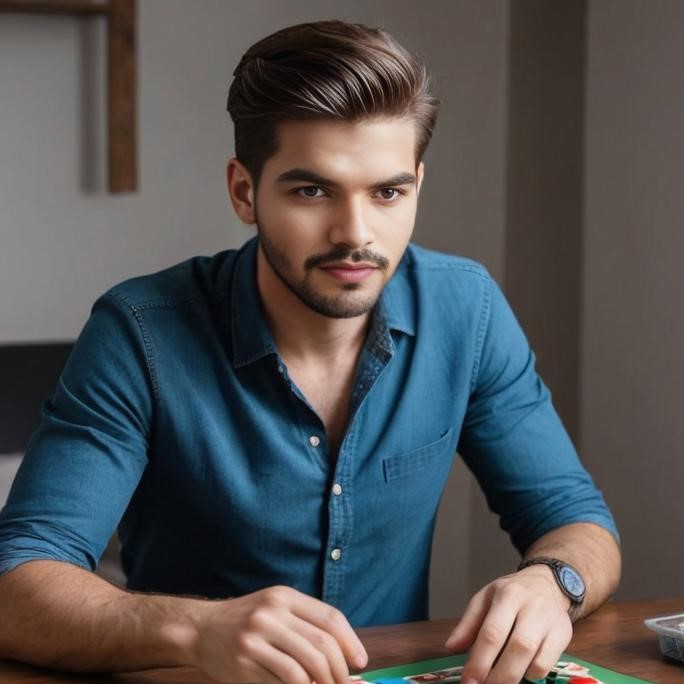
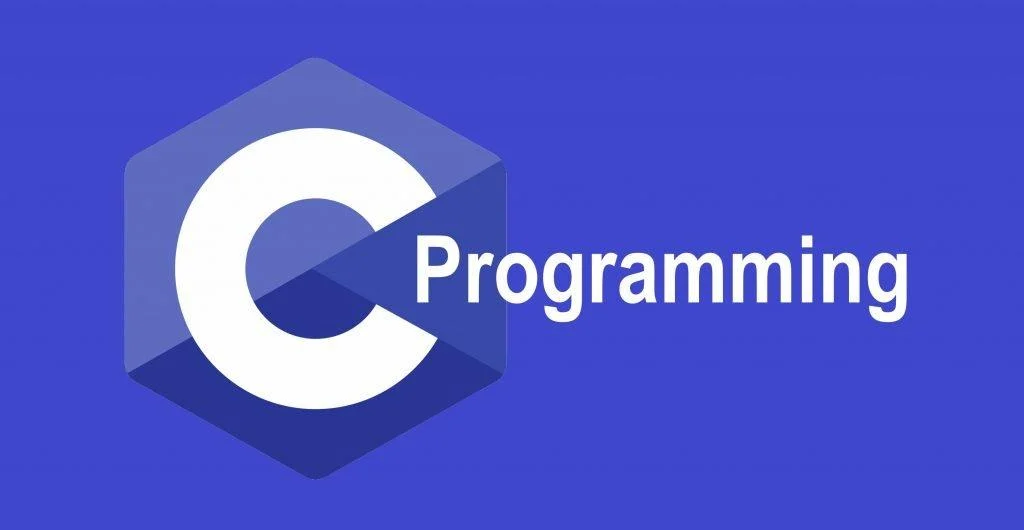
Introduction
Introduce the Number Guessing Game and its objective to guess a random number within a specified range.
Code Overview
Explain the code structure and key functionalities of the Number Guessing Game:
Random Number Generation: Use
rand()
andsrand(time(0))
to generate a random number between 1 and 100.Guessing Mechanism: Implement the guessing logic and track the number of attempts.
Main Function
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main() {
// Variable declarations
int number, guess, attempts = 0;
srand(time(0)); // Seed the random number generator
// Generate a random number between 1 and 100
number = rand() % 100 + 1;
// Game introduction
printf("Welcome to the Guessing Game!\n");
printf("Guess a number between 1 and 100\n");
// Guessing loop
do {
printf("Enter your guess: ");
scanf("%d", &guess);
attempts++;
// Compare the guess with the random number
if (guess > number) {
printf("Too high! Try again.\n");
} else if (guess < number) {
printf("Too low! Try again.\n");
} else {
printf("Congratulations! You guessed the number in %d attempts.\n", attempts);
}
} while (guess != number);
return 0;
}
Explanation
Random Number Generation: Seed the random number generator using
srand(time(0))
and generate a random number between 1 and 100.Game Loop: Prompt the user to guess the number and provide feedback based on their guess (too high, too low, or correct).
Victory Condition: Display a congratulatory message when the user guesses the number correctly and indicate the number of attempts.
Conclusion
Summarize the functionality of the Number Guessing Game and its entertainment value as a simple yet engaging game.
Final Thoughts
Encourage readers to explore and modify the game, such as changing the range of numbers or adding features like a high-score tracker.
This structure provides a clear explanation of the Number Guessing Game code and its functionality, making it accessible and informative for readers.
Output:
Subscribe to my newsletter
Read articles from Ashutosh Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
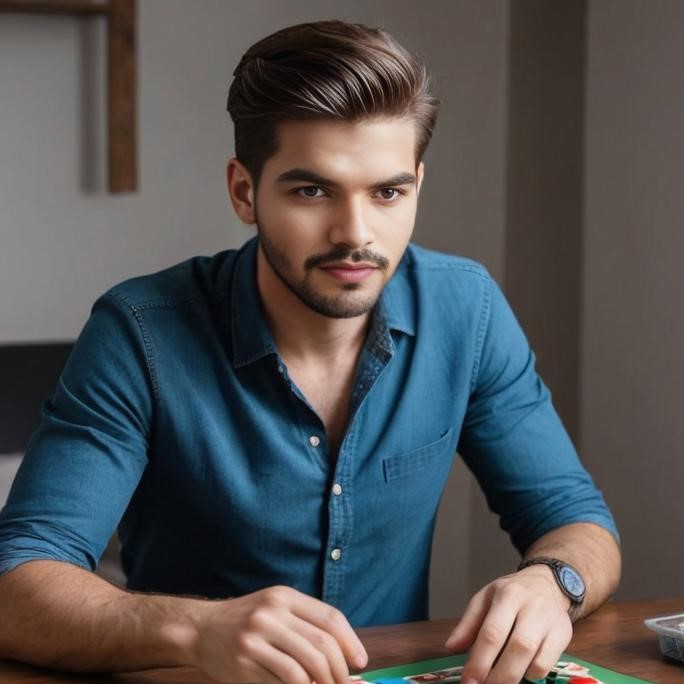
Ashutosh Kumar
Ashutosh Kumar
Creative Full Stack Web Developer & Designer specializing in crafting captivating websites & apps. With a focus on user-centric design, creative development, and effective collaboration, I can elevate brand presence with my design expertise. Till Now I have built 50+ web apps. Most importantly, I'm a reliable designer you can rely on for all your design needs. Currently Building DevDisplay - Paradise For Developers!