Mastering Access and Refresh Tokens in JavaScript
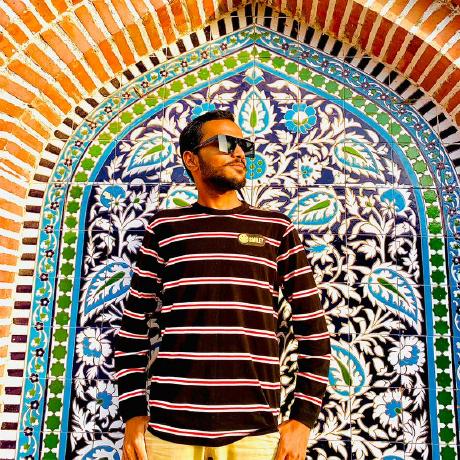
In modern web development, security is very important. One way to keep user sessions secure is by using access and refresh tokens. If you're a JavaScript developer, it's important to understand these tokens to create secure authentication and authorization in your apps. Let's explain this with simple terms and examples.
What are Access and Refresh Tokens?
Access Token: A short-lived token that grants access to protected resources (e.g., user data). It's usually sent with each request to the server to verify the user's identity.
Refresh Token: A long-lived token used to obtain a new access token once the current one expires. It helps keep the user logged in without requiring them to re-enter their credentials frequently.
How They Work Together
User Logs In: When a user logs in, they provide their credentials (username and password) to the server.
Server Responds: The server verifies the credentials and, if correct, generates an access token and a refresh token.
Access Protected Resources: The user’s application (frontend) uses the access token to make requests to protected resources.
Token Expiry: Access tokens typically have a short lifespan (e.g., 15 minutes). When it expires, the server rejects further requests made with it.
Refresh Token Usage: Instead of logging in again, the application sends the refresh token to the server to get a new access token.
Server Validates and Responds: The server verifies the refresh token. If valid, it issues a new access token, allowing continued access without requiring a new login.
Example Implementation in JavaScript
Let's walk through a simple example using JavaScript and the Fetch API for making HTTP requests.
Step 1: User Logs In:
async function login(username, password) {
const response = await fetch('
https://api.example.com/login
',
{ method: 'POST', headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ username, password }) });
if (response.ok)
{ const data = await response.json();
localStorage.setItem('accessToken', data.accessToken); localStorage.setItem('refreshToken', data.refreshToken);
} else { console.error('Login failed'); } }
Step 2: Making Authenticated Requests:
async function fetchProtectedResource()
{ let accessToken = localStorage.getItem('accessToken');
const response = await fetch('
https://api.example.com/protected
',
{ headers: { 'Authorization': Bearer ${accessToken} } });
if (response.status === 401) {
// Access token expired, try to refresh it
await refreshAccessToken();
accessToken = localStorage.getItem('accessToken');
// Retry the request with the new access token return fetch('
https://api.example.com/protected
',
{ headers: { 'Authorization': Bearer ${accessToken} } }); }
return response; }
Step 3: Refreshing the Access Token:
async function refreshAccessToken()
{ const refreshToken = localStorage.getItem('refreshToken');
const response = await fetch('
https://api.example.com/refresh-token
',
{ method: 'POST', headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ refreshToken }) });
if (response.ok) {
const data = await response.json();
localStorage.setItem('accessToken', data.accessToken);
} else { console.error('Failed to refresh access token');
// Optionally, log the user out or ask them to log in again
}
}
Key Points to Remember
Security: Always keep access and refresh tokens secure. Do not expose them in URLs or store them in insecure locations.
Expiration: Design your application to handle token expiration gracefully by using refresh tokens to get new access tokens.
Storage: Use secure storage mechanisms like
localStorage
,sessionStorage
, or secure cookies based on your application's requirements.
Conclusion
Access and refresh tokens are essential tools for managing secure user sessions in web applications. By understanding how to implement and use them, you can enhance the security and user experience of your applications. With the provided examples, you should now have a clear understanding of how to work with these tokens in JavaScript.
Subscribe to my newsletter
Read articles from Farrukh Ali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
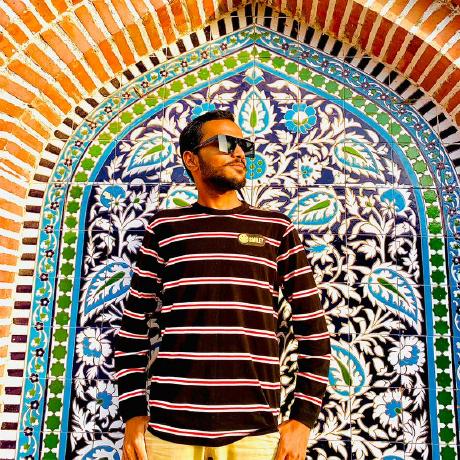