Understanding Huffman Coding in C
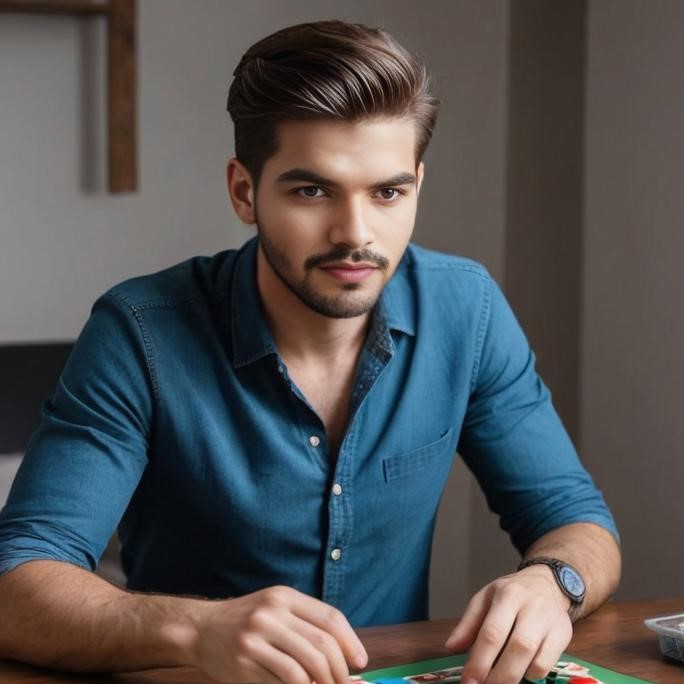
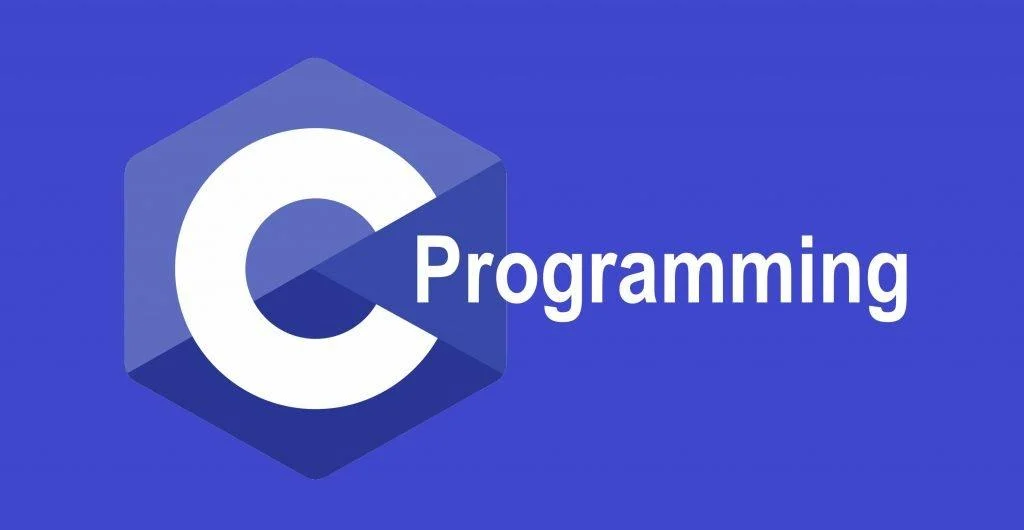
Introduction:
- Briefly explain Huffman coding and its importance in data compression.
Code Overview:
Define structures for nodes and the min heap.
Implement functions for creating nodes, min heap operations, and building the Huffman tree.
Functions:
Create Node
- Creates a new node with specified data and frequency.
struct Node* createNode(char data, int frequency) {
// Code for createNode function
}
Min Heap Operations
Explain// Functions for min heap operations
void swapNode(struct Node** a, struct Node** b);
void minHeapify(struct MinHeap* minHeap, int idx);
int isSizeOne(struct MinHeap* minHeap);
struct Node* extractMin(struct MinHeap* minHeap);
void insertMinHeap(struct MinHeap* minHeap, struct Node* node);
void buildMinHeap(struct MinHeap* minHeap);
Build Huffman Tree
struct Node* buildHuffmanTree(char data[], int frequency[], int size) {
// Code for buildHuffmanTree function
}
Print Huffman Codes
void printCodes(struct Node* root, int arr[], int top) {
// Code for printCodes function
}
Main Function:
Initializes data and frequency arrays.
Calls the Huffman coding function and prints the codes.
In Short:
Demonstrates Huffman coding in C for data compression.
Utilizes a min heap to build the Huffman tree efficiently.
Prints the Huffman codes for characters based on their frequencies.
Conclusion
Summarizes the code's purpose in generating Huffman codes.
Discusses the role of Huffman coding in efficient data representation.
Final Thoughts
- Encourages further exploration of data compression techniques and algorithms.
Is this brief explanation with code snippets sufficient for your needs regarding Huffman coding in C?
Output:
Subscribe to my newsletter
Read articles from Ashutosh Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
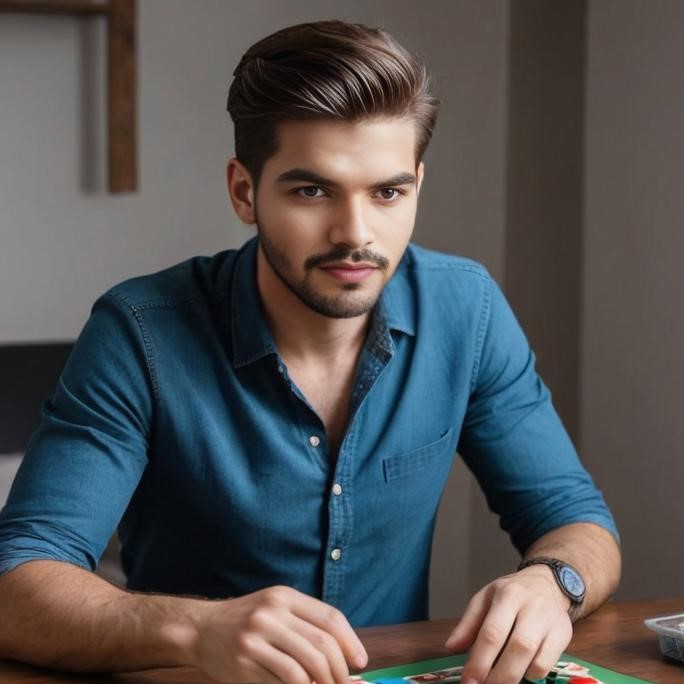
Ashutosh Kumar
Ashutosh Kumar
Creative Full Stack Web Developer & Designer specializing in crafting captivating websites & apps. With a focus on user-centric design, creative development, and effective collaboration, I can elevate brand presence with my design expertise. Till Now I have built 50+ web apps. Most importantly, I'm a reliable designer you can rely on for all your design needs. Currently Building DevDisplay - Paradise For Developers!