Exploring Lists in OCaml: A Pocket Guide
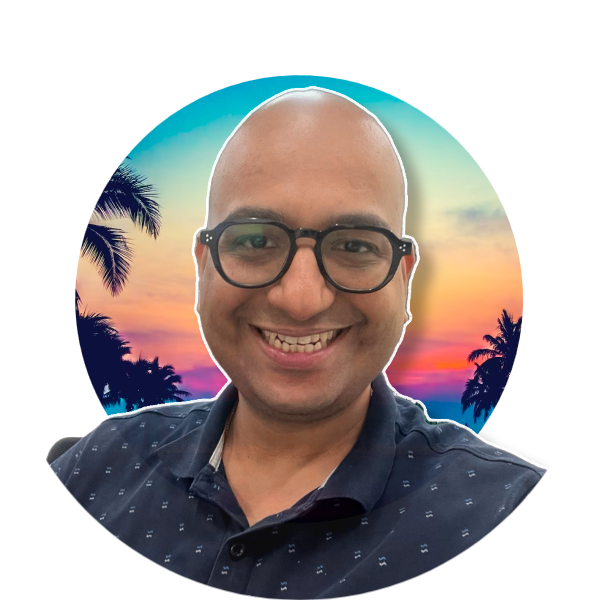
Table of contents
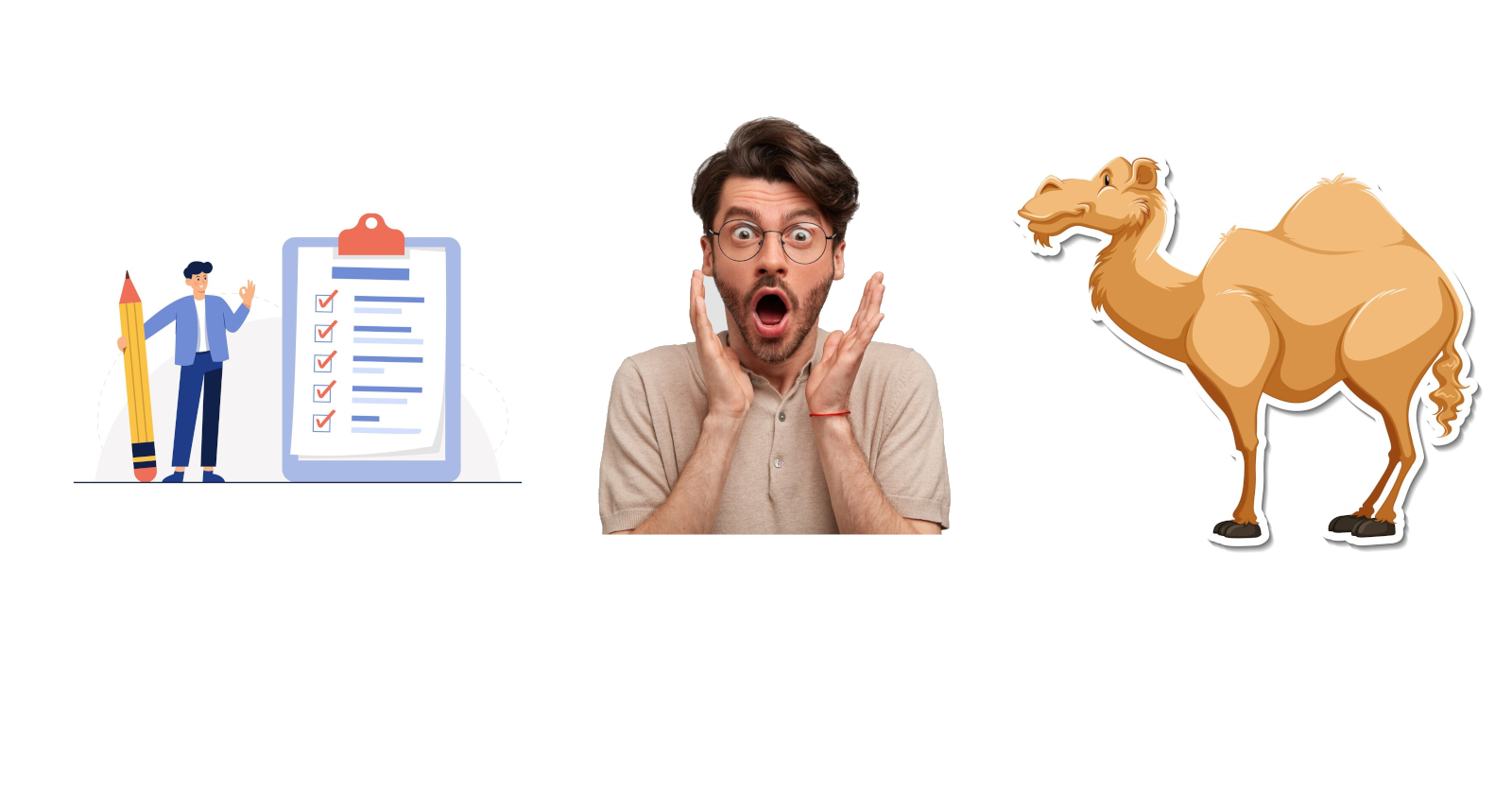
Introduction
Lists are a fundamental data structure in OCaml, embodying the language's strengths in functional programming. A list in OCaml is an ordered sequence of elements, where each element must be of the same type. From beginners exploring basic list operations to seasoned developers leveraging advanced features, lists serve as versatile tools in various programming scenarios. Understanding how to efficiently manipulate and use lists can significantly enhance your OCaml programming skills, ensuring you can write cleaner, more effective code. This article endeavors to provide a comprehensive tutorial on lists in OCaml, covering basic concepts, useful features, and practical examples to elucidate their application.
Lists in OCaml
Imagine an OCaml list as a row of lockers, where each locker holds a value but all lockers must hold the same type of item. You can easily retrieve items from specific lockers, add new items to the front, or chain rows of lockers together. Whether you're counting items, transforming them, or sorting them, OCaml offers straightforward operations to handle these tasks efficiently.
Features of OCaml Lists
Basic List Declaration and Access
Lists are declared using square brackets, with elements separated by semicolons. For example,
[1; 2; 3]
is a list of integers.Elements are accessed by pattern matching:
let x::xs = [1; 2; 3]
assigns1
tox
and[2; 3]
toxs
.
Empty List and Polymorphism
The empty list is written as
[]
and works with any type ('a list
is a list of any typea
).Example:
let empty_list = []
Appending Elements
Use the
::
operator to add elements to the front of a list.Example:
let new_list = 1 :: [2; 3] (* [1; 2; 3] *)
Combining Lists
Use the
@
operator to concatenate lists.Example:
let combined_list = [1] @ [2; 3] (* [1; 2; 3] *)
Higher-Order Functions:
map
andfold
Functions like
map
apply a function to each list element, returning a new list.Example:
let squares = List.map (fun x -> x * x) [1; 2; 3] (* [1; 4; 9] *)
Tail Recursion for Efficiency
Tail-recursive functions prevent stack overflow on long lists.
Example:
let rec length acc = function | [] -> acc | _::t -> length (acc + 1) t
Association Lists
Simple key-value pairs can be handled using lists of tuples.
Example:
let alist = [(1, "one"); (2, "two")] let value = List.assoc 1 alist (* "one" *)
Sorting and Searching
The
List.sort
function sorts lists using a custom comparison function.Example:
let sorted_list = List.sort compare [3; 1; 2] (* [1; 2; 3] *)
Takeaway
OCaml lists offer powerful, flexible ways to handle ordered collections of elements. By understanding basic list syntax, operations like appending and concatenation, and leveraging higher-order functions like map
and fold
, you can perform complex manipulations simply and efficiently. Lists being able to hold polymorphic types and the ability to create efficient, tail-recursive functions for large list operations are particularly beneficial. Additionally, features like association lists and built-in sort functions add versatility, making OCaml a potent language for functional programming. Whether for simple tasks or intricate data processing, mastering lists in OCaml equips you with a crucial tool in your functional programming arsenal.
References
Image attribution
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
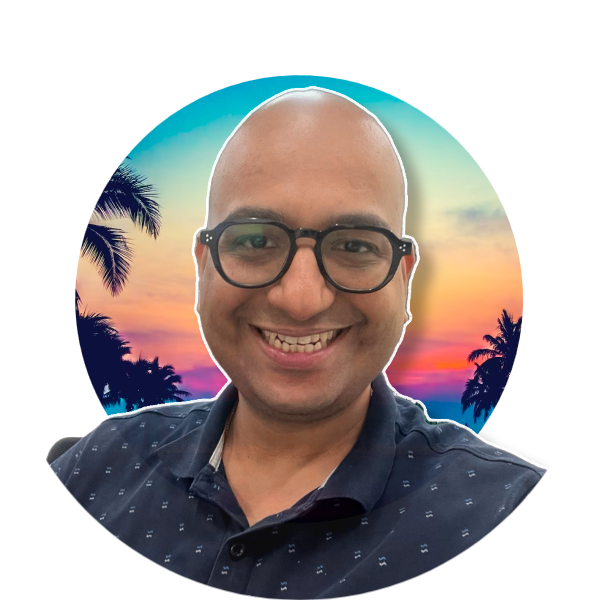
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.