JavaScript Flow Control Statement
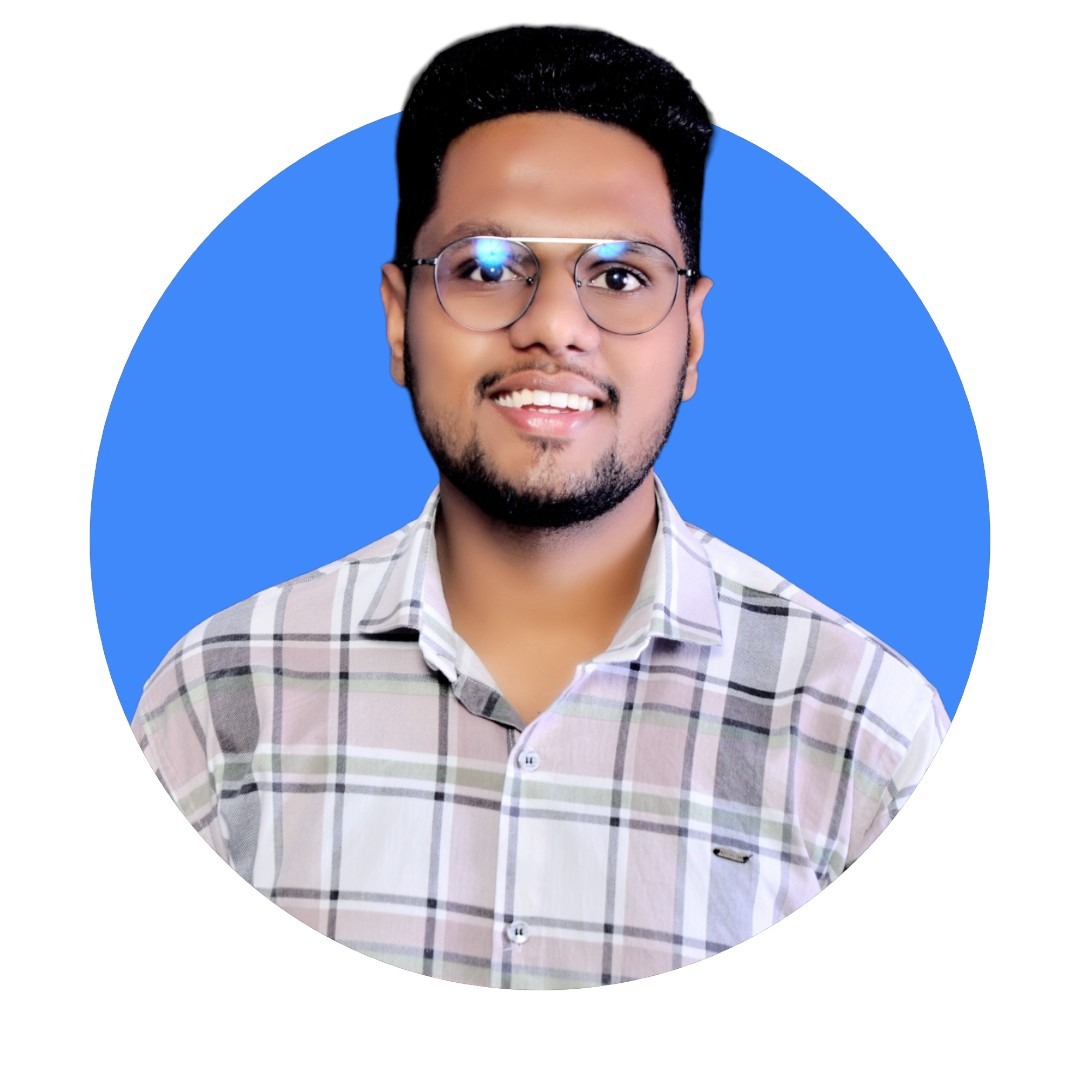
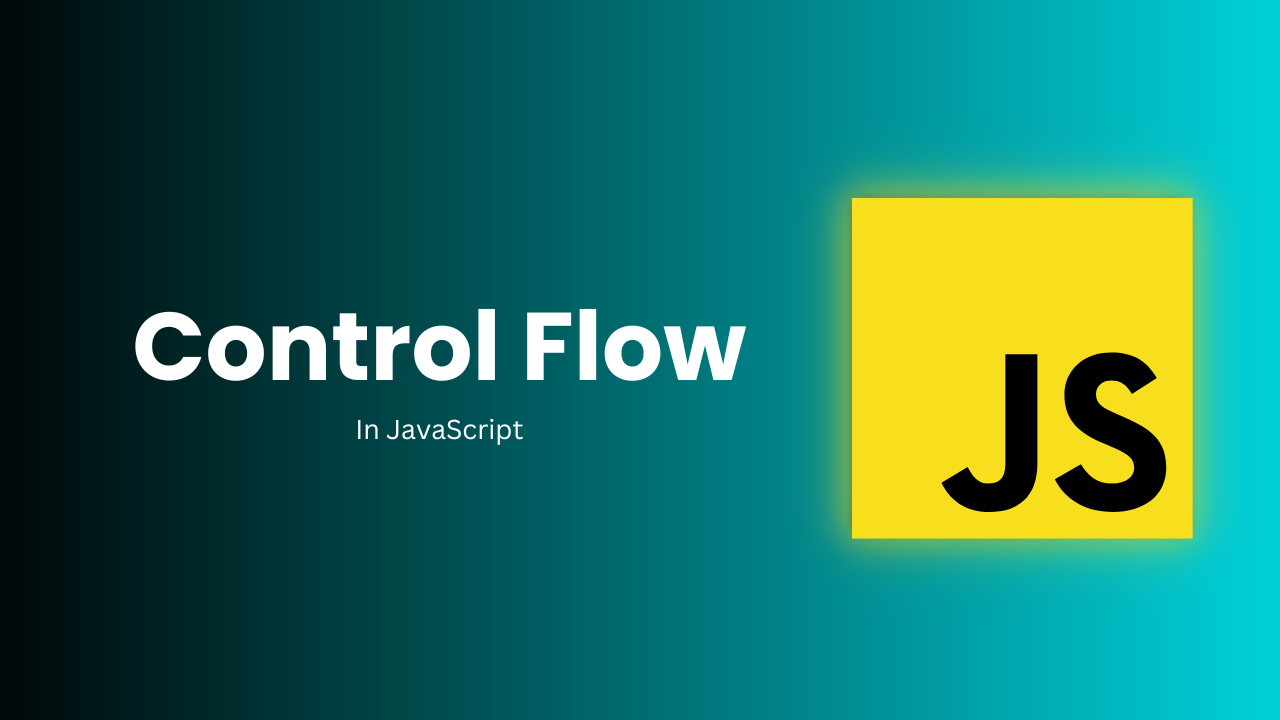
Classification Of Statements:
Conditional Statement :
There are three forms of if statement in JavaScript.
If Statement
If else statement
if else if statement
JavaScript If statement :
It evaluates the content only if expression is true. The signature of JavaScript if statement is given below.
if(expression){
//content to be evaluated
}
Flowchart of JavaScript If statement :
Let’s see the simple example of if statement in javascript.
<html>
<body>
<script>
var a=20;
if(a>10){
document.write("value of a is greater than 10");
}
</script>
</body>
</html>
Output:
JavaScript If...else Statement :
It evaluates the content whether condition is true of false.
The syntax of JavaScript if-else statement is given below.
if(expression){
//content to be evaluated if condition is true
}
else{
//content to be evaluated if condition is false
}
Flowchart of JavaScript If...else statement :
JavaScript If...else if statement:
It evaluates the content only if expression is true from several expressions.
The signature of JavaScript if else if statement is given below.
if(expression1){
//content to be evaluated if expression1 is true
}
else if(expression2){
//content to be evaluated if expression2 is true
}
else if(expression3){
//content to be evaluated if expression3 is true
}
else{
//content to be evaluated if no expression is true
}
JavaScript Switch Statement :
The JavaScript switch statement is used to execute one code from multiple expressions.
It is just like else if statement that we have learned in previous .
But it is convenient than if..else..if because it can be used with numbers, characters etc.
The signature of JavaScript switch statement is given below.
switch(expression){
case value1:
code to be executed;
break;
case value2:
code to be executed;
break;
......
default:
code to be executed if above values are not matched;
}
Let’s see the simple example of switch statement in javascript.
<!DOCTYPE html>
<html>
<body>
<script>
var grade='B';
var result;
switch(grade){
case 'A':
result="A Grade";
break;
case 'B':
result="B Grade";
break;
case 'C':
result="C Grade";
break;
default:
result="No Grade";
}
document.write(result);
</script>
</body>
</html>
Output:
Unconditional Statements:
Break and continue in JavaScript :
Like other programming languages, break and continue keywords are jump statements in JavaScript. Jump statements are also known as unconditional jumps. Now, let’s discuss the break and continue statements in detail.
Break in JavaScript :
The break in JavaScript is used to terminate or end the execution of the loop.
Depending upon some condition and the flow of control passes to the statement immediately after the loop, if any.
It is used with loops inside the if or else block. The break statement is defined using the break keyword.
Syntax of Break in JavaScript :
break;
Example of Break in JavaScript :
for (let i=0;i<10;i++)
{
if(i==5)
break;
console.log (i);
}
console.log ('Loop Terminated!');
Output:
0
1
2
3
4
//Loop Terminated!
Here, in the above example, we see that the value of i is printed up to i=4. When I become 5, the break statement is executed and the loop execution stops. The program control reaches the statement immediately after the loop and hence, the message Loop Terminated! is printed.
Flowchart :
Continue in JavaScript :
The continue in JavaScript is used to skip the execution of statements of the loop for the current iteration and continue with the next iteration of the loop.
Unlike the break statement, which terminates or ends the loop execution, the continue statement does not terminate the execution of the loop, it just skips the execution of the loop based on some conditions.
The continue statement is defined using the continue keyword.
Syntax of Continue in JavaScript :
continue;
Example of Continue in JavaScript :
for (let i=0;i<10;i++)
{
if(i==5)
continue;
console.log (i);
}
console.log ('Loop Terminated!');
Output:
0
1
2
3
4
6
7
8
9
//Loop Terminated!
Here, in the above Continue in JavaScript example, we see that the value of i is printed up to i=4. When I become 5, the continue statement is executed and the loop execution for the current iteration is skipped, that’s why 5 is not printed. The execution of the loop continues after i=5, and we are getting values of i.
Finally, when the loop condition fails i.e., i=11, the loop ends, and the program control reaches the statement immediately after the loop, and hence, the message Loop Terminated! is printed. Unlike break statements, we cannot use continue statements with switch statements in JavaScript.
Flowchart :
Whats Next ?
Javascript Looping Statement .
Subscribe to my newsletter
Read articles from Aditya Gadhave directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
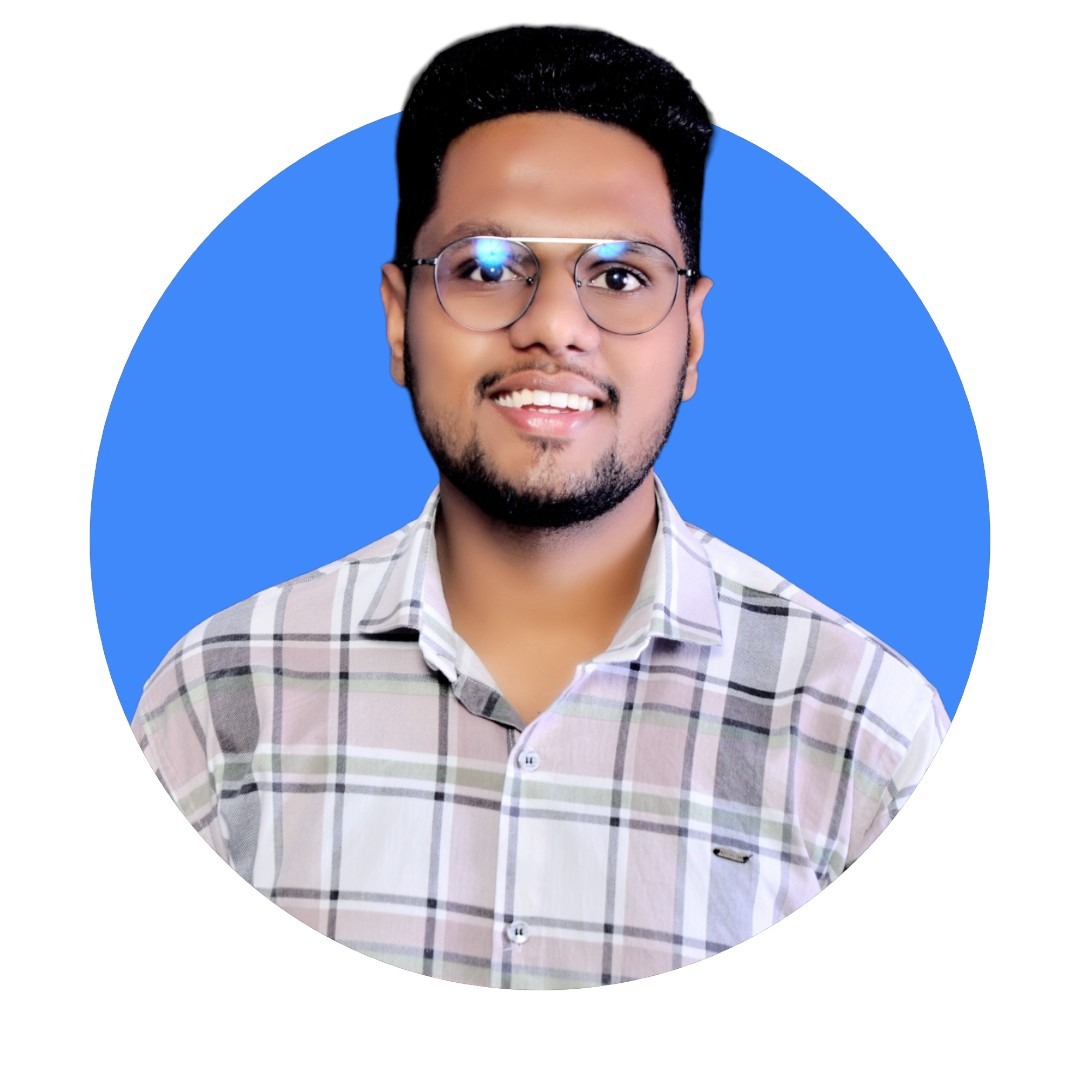
Aditya Gadhave
Aditya Gadhave
👋 Hello! I'm Aditya Gadhave, an enthusiastic Computer Engineering Undergraduate Student. My passion for technology has led me on an exciting journey where I'm honing my skills and making meaningful contributions.