Building a Basic Banking System in C
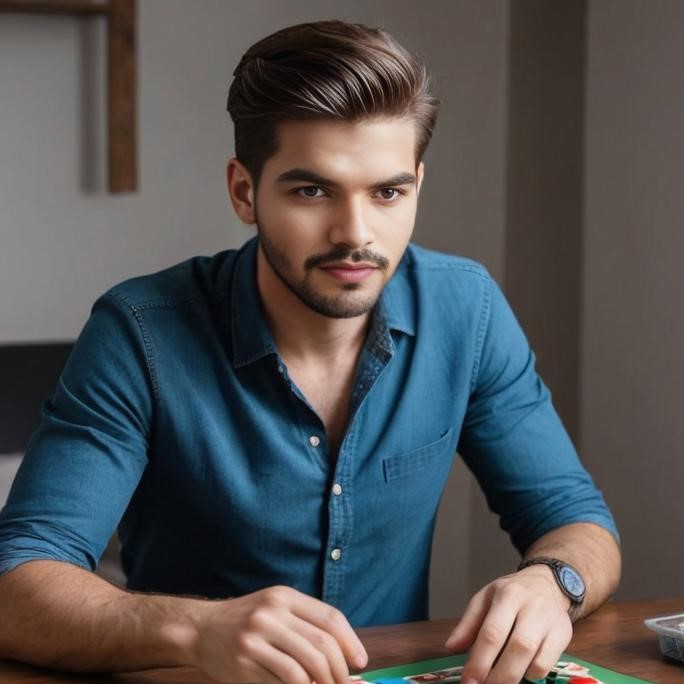
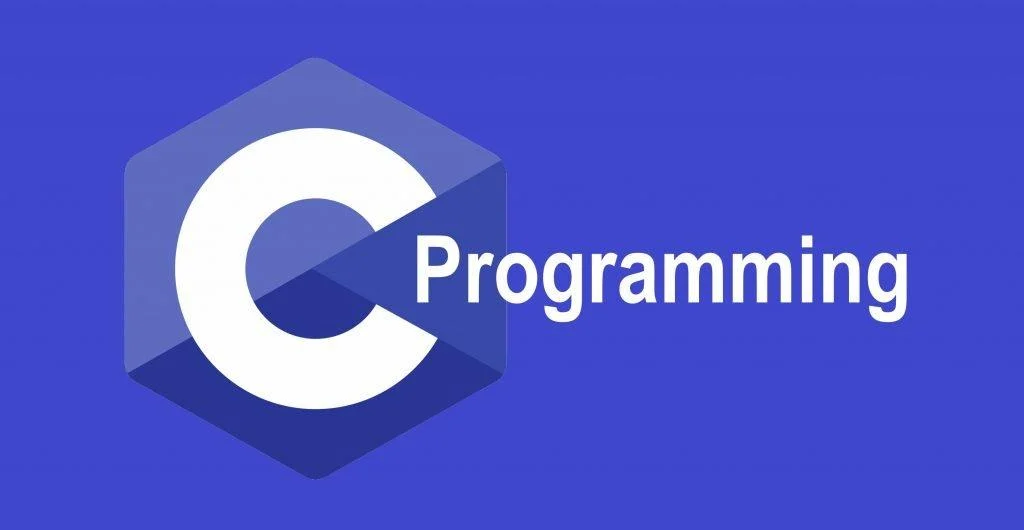
Table of contents
Introduction:
Discuss the importance of banking systems in managing financial transactions.
Introduce the concept of a basic banking system implemented in C.
Outline the functionalities to be covered in the code.
Code Overview:
Explain the purpose of each header file included (
stdio.h
,stdlib.h
,string.h
).Define constants for maximum accounts and maximum transactions.
Define structures for transactions and accounts, including arrays to store transaction history.
Functions:
Create Account Function
Create a new account with a unique account number, name, and zero balance.
Add the account to the accounts array.
void createAccount() {
// Code for createAccount function
}
Deposit Function
Add a specified amount to the balance of a given account.
Record the deposit transaction in the account's transaction history.
void deposit(int accountNumber, float amount) {
// Code for deposit function
}
Withdraw Function
Deduct a specified amount from the balance of a given account.
Check for sufficient balance before processing the withdrawal.
Record the withdrawal transaction in the account's transaction history.
void withdraw(int accountNumber, float amount) { // Code for withdraw function }
Transfer Function
Transfer a specified amount from one account to another.
Check for sufficient balance in the sender's account before processing the transfer.
Record transfer transactions in both sender and recipient accounts' transaction histories.
void transfer(int fromAccount, int toAccount, float amount) {
// Code for transfer function
}
View Transactions Function
- Display the transaction history for a given account.
void viewTransactions(int accountNumber) {
// Code for viewTransactions function
}
Main Function
Present a menu to the user with options for banking operations.
Handle user input using a do-while loop and a switch statement.
Call the appropriate functions based on the user's choice.
int main() {
// Code for main function
}
Conclusion
Summarize the functionalities and capabilities of the basic banking system implemented in C.
Discuss potential enhancements or additional features that could be added to the system.
Encourage further exploration and customization of the code for practical applications
Does this structure with the code functions presented in a more attractive format suit your blog post about the banking system in C?
Output:
Subscribe to my newsletter
Read articles from Ashutosh Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
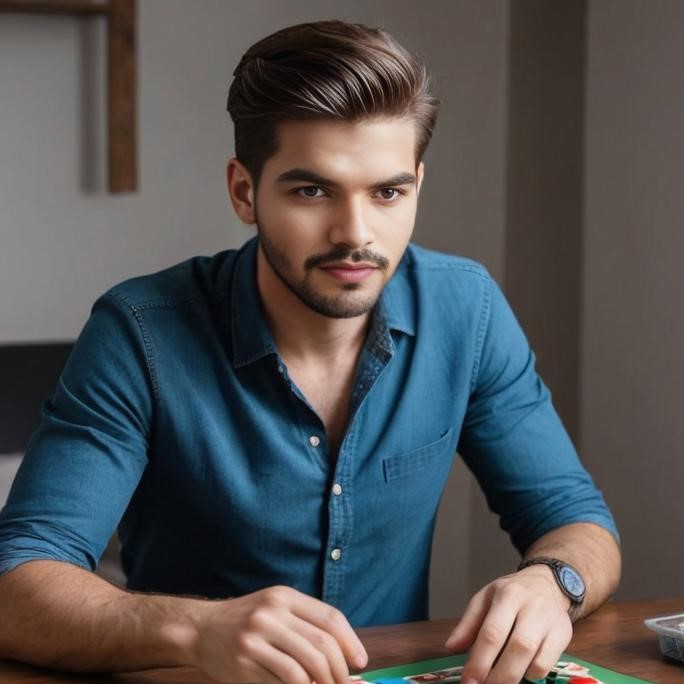
Ashutosh Kumar
Ashutosh Kumar
Creative Full Stack Web Developer & Designer specializing in crafting captivating websites & apps. With a focus on user-centric design, creative development, and effective collaboration, I can elevate brand presence with my design expertise. Till Now I have built 50+ web apps. Most importantly, I'm a reliable designer you can rely on for all your design needs. Currently Building DevDisplay - Paradise For Developers!