Introduction to Go (Golang)
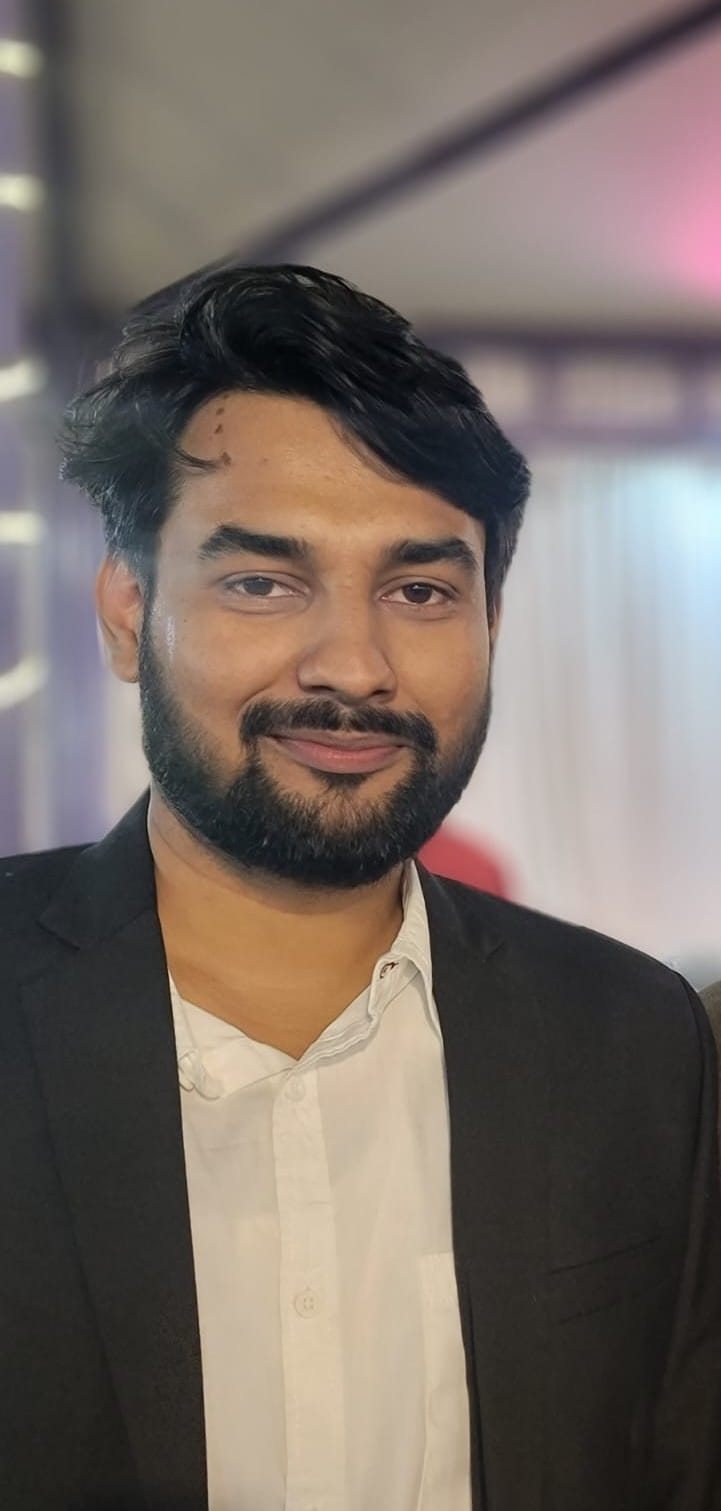
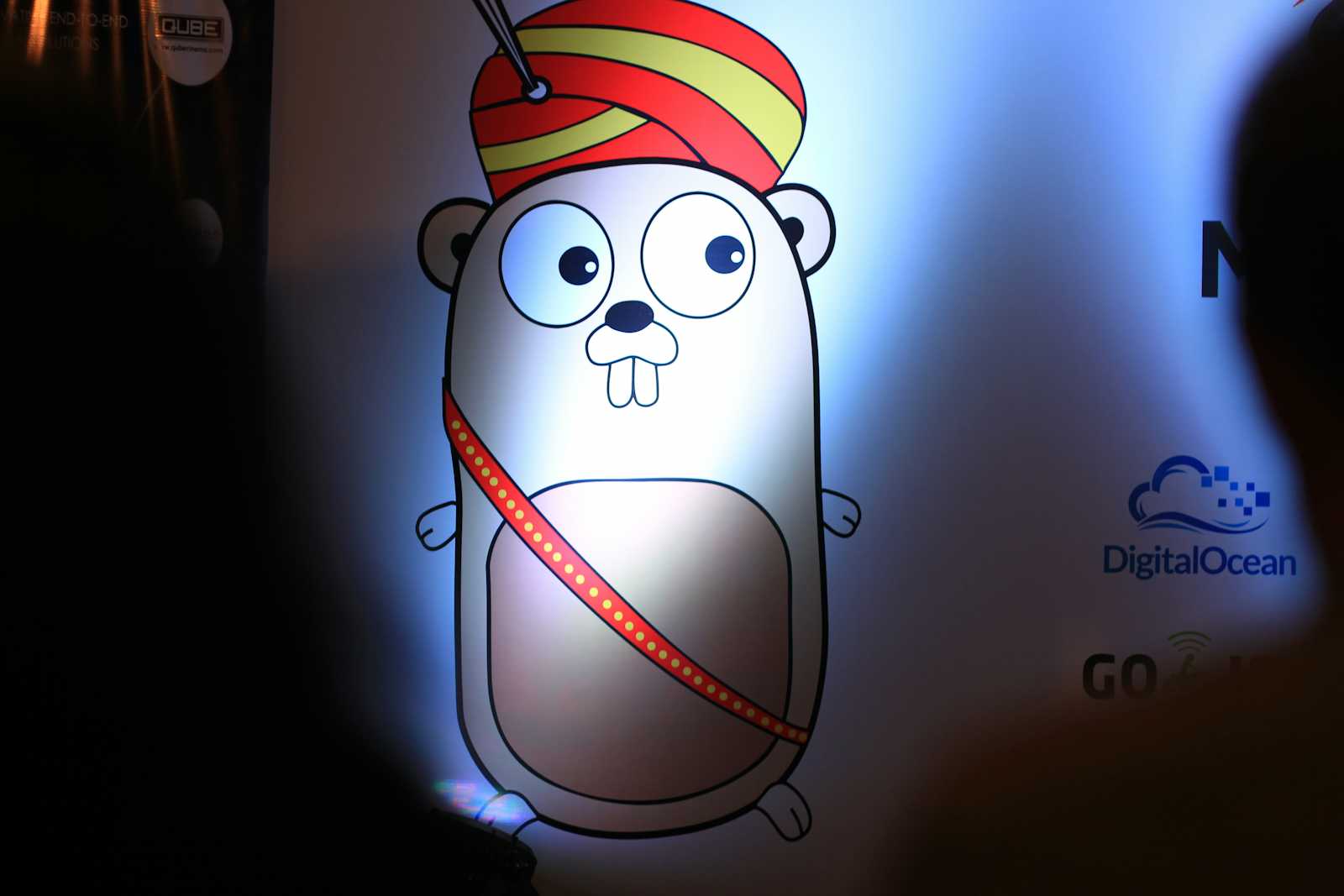
Go, also known as Golang, is a powerful and efficient programming language developed by Google. It has gained significant popularity for its simplicity, performance, and robust concurrency support. In this article, we'll explore the history and features of Go, guide you through setting up your development environment, and walk you through creating your first "Hello World" program.
History of Go
Go was created by Robert Griesemer, Rob Pike, and Ken Thompson at Google and was first released in November 2009. The language was designed to address common issues faced by developers, such as slow compilation times, cumbersome dependencies, and the complexities of multithreading. The primary goal was to create a language that combined the ease of programming of dynamically typed languages with the efficiency and safety of statically typed languages.
Key Milestones in Go's History:
2007: Initial design and development began at Google.
2009: Go was officially announced to the public.
2012: Version 1.0 of Go was released, marking its stability for production use.
2015: Go 1.5 was released, removing the last dependency on C code.
2019: Go celebrated its 10th anniversary with significant adoption in the tech industry.
Features of Go
Go's design incorporates several features that make it an attractive choice for modern software development:
Simplicity: Go has a clean syntax and a minimalistic design, making it easy to learn and use.
Performance: Compiled to native machine code, Go programs offer excellent performance.
Concurrency: Built-in support for concurrent programming with goroutines and channels makes Go ideal for scalable, high-performance applications.
Garbage Collection: Automatic memory management simplifies development and reduces the risk of memory leaks.
Strong Standard Library: Go includes a rich standard library that supports various tasks, from web servers to cryptography.
Statically Typed: Go's static typing helps catch errors at compile time, enhancing reliability.
Fast Compilation: Go's compilation speed is designed to be as fast as possible, improving developer productivity.
Setting Up the Go Environment
To start developing with Go, you need to set up your development environment. Here are the steps to get started:
Download and Install Go:
Visit the official Go website at golang.org and download the appropriate installer for your operating system (Windows, macOS, or Linux).
Follow the installation instructions provided for your OS.
Set Up Your Workspace:
Create a directory for your Go projects. This directory is often referred to as the
$GOPATH
.Inside your
$GOPATH
, create three subdirectories:src
(source files),bin
(compiled binaries), andpkg
(package objects).
Configure Environment Variables:
Add the Go binary to your system's
PATH
to make thego
command available from the terminal.Set the
GOPATH
environment variable to the path of your workspace directory.
Verify Installation:
- Open a terminal and run the command
go version
to verify that Go is installed correctly and to see the installed version.
- Open a terminal and run the command
Writing Your First Go Program: Hello World
Let's create a simple "Hello World" program to ensure everything is set up correctly.
Create the Program File:
Inside your
$GOPATH/src
directory, create a new directory for your project, for example,hello
.Inside the
hello
directory, create a file namedmain.go
.
Write the Code:
Open
main.go
in your preferred text editor and add the following code:package main import "fmt" func main() { fmt.Println("Hello, World!") }
Run the Program:
Open a terminal, navigate to the
hello
directory, and run the command:go run main.go
You should see the output:
Hello, World!
Congratulations! You've successfully written and executed your first Go program.
Conclusion
Go (Golang) is a modern programming language that offers simplicity, performance, and robust support for concurrency. By understanding its history and features, setting up your development environment, and writing your first "Hello World" program, you have taken the first steps toward mastering Go. Keep exploring and building with Go to leverage its full potential in your projects.
Subscribe to my newsletter
Read articles from Lokesh Jha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
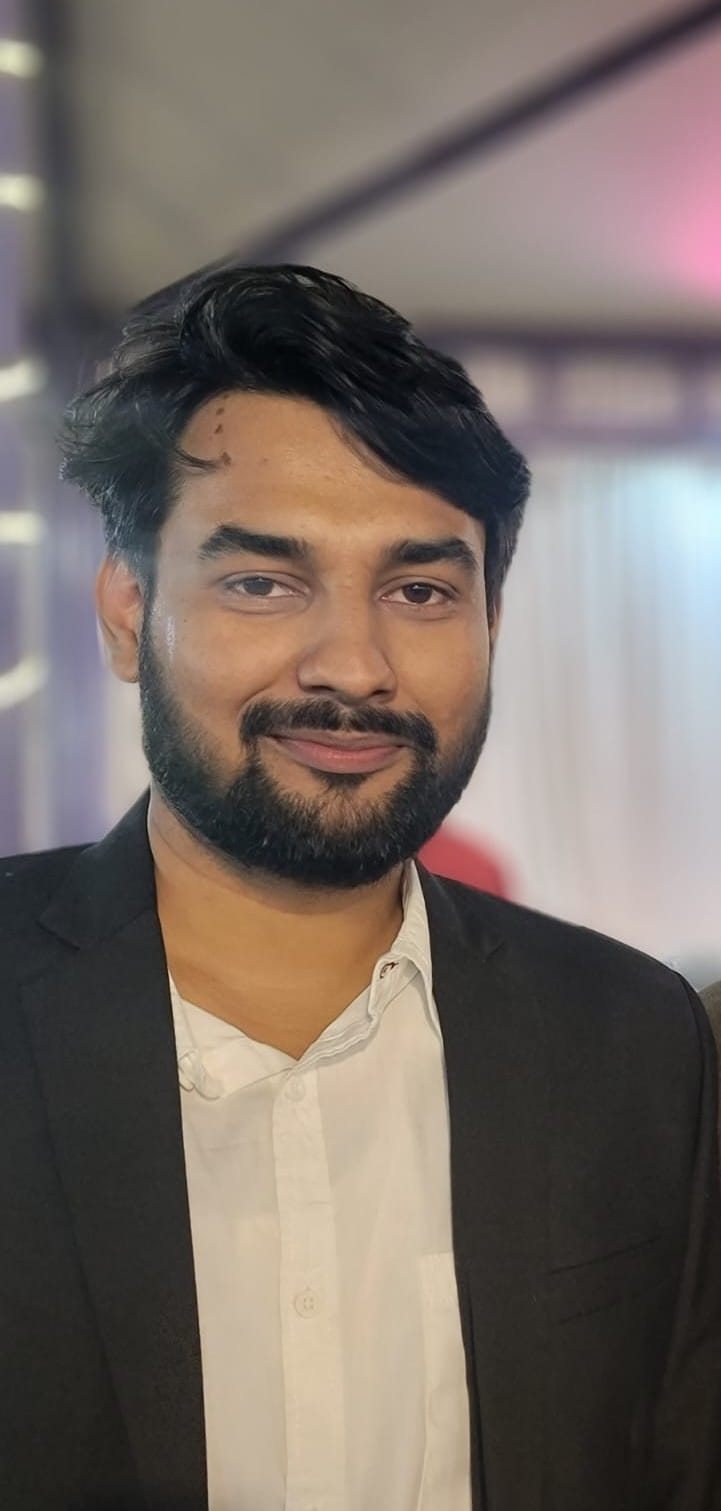
Lokesh Jha
Lokesh Jha
I am a senior software developer and technical writer who loves to learn new things. I recently started writing articles about what I've learned so that others in the community can gain the same knowledge. I primarily work with Node.js, TypeScript, and JavaScript, but I also have past experience with Java and C++. Currently, I'm learning Go and may explore Web3 in the future.