Loops, Arrays, Objects and Functions
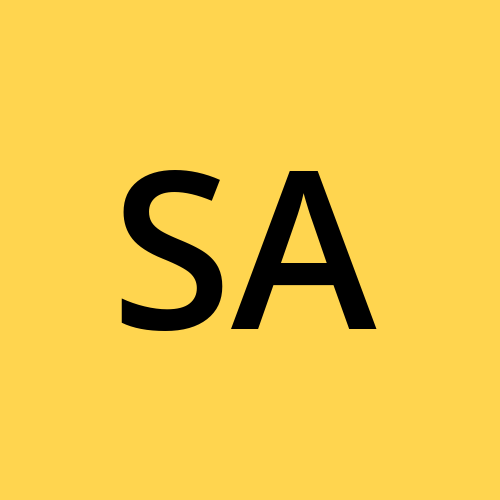
Loops
Loops are used to execute a block of code repeatedly as long as a specified condition is true. JavaScript supports several types of loops:
For Loop
The for
loop is used to run a block of code a specific number of times. It consists of three parts: initialization, condition, and increment/decrement.
Syntax:
for (initialization; condition; increment/decrement) {
// code block to be executed
}
for (let i = 0; i < 5; i++) {
console.log(i);
}
// Output: 0, 1, 2, 3, 4
In the loop, it will first create a variable named as
i
and assign a value to0
, now it will check whether0
is lesser than5
or not, if the condition is true then it will increment the variablei
value with1
, at the starting of this loop, before matching the condition,i
's value was0
, but after the condition came as true, its value changed to1
, and now we are inside thefor
loops body, and simply we logged out ouri
value. Now again it went back and this time it did not create a variable, it just jumped to the condition part, it checked whether1
is lesser than5
or not, yes it is lesser than5
so it again incrementedi
value with one and went tofor
loops body and logged out ouri
's value. This process will repeat for 5 times, on the 5th and last time it checked the value ofi
which is 5, is lesser than the condition5
, no its not lesser than5
, both are equal, now it will increment the value ofi
with one and break/come out of loop.You might be wondering why did it increment the value of
i
if the condition became false on the last loop, Well that is how JavaScript works in loops.
While Loop
The while
loop runs a block of code as long as the specified condition is true.
syntax:
while (condition) {
// code block to be executed
}
let i = 0;
while (i < 5) {
console.log(i);
i++;
}
// Output: 0, 1, 2, 3, 4
- The
while
loop works similar tofor
loop, only the way we write our condition is different, other than that, all of the logic I explained onfor
loop works the same here.
Do-While Loop
The do-while
loop is similar to the while
loop, but it guarantees that the code block will be executed at least once since the condition is evaluated after the block is executed.
syntax:
do {
// code block to be executed
} while (condition);
let i = 0;
do {
console.log(i);
i++;
} while (i < 5);
// Output: 0, 1, 2, 3, 4
- As it was mentioned above, the
do-while
loop will be executed at least once even if the condition does not match, let us suppose:
let i = 6;
do {
console.log(i);
i++;
} while (i < 5);
// Output: 6
- In this example
i
's value is6
and it is certain that6
is lesser than5
in our condition yet the loop will be executed once and print it in our log then break/come out of loop. This loop can be helpful if we want some of our logic to be executed at least for once even if our condition is not matching.
break
in for
loop
for (let i = 0; i < 5; i++) {
if (i == 3) {
break
}
console.log(i)
}
// Output: 0, 1, 2, 4
- Remember how the
for
loop works, it is working on the same logic, but we have a new keywordbreak
inside of ourif
condition, what we are saying in the condition is that if the value ofi
is equals to3
then break out of the whole loop and stop the execution there. Hence we got our output as0, 1, 2
and after that the loop got broken.
continue
in for
loop
for (let i = 0; i < 5; i++) {
if (i == 3) {
continue;
}
console.log(i);
}
// Output: 0, 1, 2, 4
- It is again working on the same logic of
for
loop, but we again have a new keywordcontinue
, what actually happening is that in ourif
condition we are checking if the value ofi
is equals to3
, if its correct then it will skip the value of3
and then go back to the loop and continue the execution while skipping the number3
. Hence we got our output as0, 1, 2, 4
.
Understanding loops is very important because suppose we have n number of data in our database and we want to render it out in our frontend, we can never be sure how many times we will have to write it down in frontend logic, so that's where loops come in help, it will count how many data's are there in database and then run the loop for that number of time's. It is purely executing dynamically.
Arrays
Arrays are used to store multiple values in a single variable. JavaScript arrays are dynamic, meaning they can grow and shrink in size. Here are some array methods and properties:
Creating an Array
let fruits = ['Apple', 'Banana', 'Cherry'];
- Writing them in single quotes
''
or double quotes""
does not really make any difference, JavaScript will make it as single quotes even if written in double quotes.
Array Properties and Methods
.length
The .length
property returns the number of elements quantity in an array.
let fruits = ['Apple', 'Banana', 'Cherry'];
console.log(fruits.length); // Output: 3
- It will count how many element's are there in the array.
.push()
The .push()
method adds one or more elements to the end of an array and returns the new length of the array.
let fruits = ['Apple', 'Banana', 'Cherry'];
fruits.push('Pineapple');
console.log(fruits); // Output: ['Apple', 'Banana', 'Cherry', 'Pineapple']
.pop()
The .pop()
method removes the last element from an array and returns that element. This method changes the length of the array.
let fruits = ['Apple', 'Banana', 'Cherry'];
let lastFruit = fruits.pop();
console.log(lastFruit); // Output: 'Cherry'
console.log(fruits); // Output: ['Apple', 'Banana']
.sort()
The .sort()
method sorts the elements of an array in ascending order and returns the sorted array. By default, the sort order is according to string Unicode code points.
let fruits = ['Banana', 'Apple', 'Cherry'];
fruits.sort();
console.log(fruits); // Output: ['Apple', 'Banana', 'Cherry']
- As mentioned above, it can only sort in String, it cannot sort number's in order for example:
let nums = [100, 63, 245];
nums.sort();
console.log(nums); // Output: [ 100, 245, 63 ]
- In this case the sort function will first convert the numbers into String, such as
['100', '63', '245']
and then it will only focus on first Number ex:'1', '6', '2'
, then it will sort the numbers according to that, hence it gave us the output of[100, 245, 63]
.
To sort the numbers we can do:
let nums = [100, 63, 245];
nums.sort((a, b) => a - b);
console.log(nums); // Output: [ 63, 100, 245 ]
- We have used an Arrow Function inside our
.sort()
function.
.includes()
The .includes()
method determines whether an array contains a certain element, returning true
or false
as appropriate.
let fruits = ['Apple', 'Banana', 'Cherry'];
console.log(fruits.includes('Banana')); // Output: true
console.log(fruits.includes('Grape')); // Output: false
.indexOf()
The .indexOf()
method returns the first index at which a given element can be found in the array, or -1
if it is not present.
let fruits = ['Apple', 'Banana', 'Cherry'];
console.log(fruits.indexOf('Banana')); // Output: 1
console.log(fruits.indexOf('Grape')); // Output: -1
.splice()
The .splice()
method changes the contents of an array by removing or replacing existing elements and/or adding new elements.
let fruits = ['Apple', 'Banana', 'Cherry'];
// Remove 1 element at index 1
let removed = fruits.splice(1, 1);
console.log(fruits); // Output: ['Apple', 'Cherry']
console.log(removed); // Output: ['Banana']
// Add 'Grape' at index 1
fruits.splice(1, 0, 'Grape');
console.log(fruits); // Output: ['Apple', 'Grape', 'Cherry']
fruites.splice(remove from which index, how many element to remove)
, so in our first example we said remove from index1
and remove only1
element from there.Index's count always start from
0
and it can go as far as your Arrays length. Here the element'Apple'
is at index0
and'Banana'
at index of1
so we said to remove'Banana'
from the Array and if we were to remove till'Cherry'
then we would have specified2
in our.splice()
's second value, But we wanted to remove only the'Banana'
so we said1
in the second value.
.forEach()
The .forEach()
method executes a provided function once for each array element.
let fruits = ['Apple', 'Banana', 'Cherry'];
fruits.forEach((fruit, index) => {
console.log(`${index}: ${fruit}`);
});
// Output:
// 0: Apple
// 1: Banana
// 2: Cherry
forEach()
is a looping function for Arrays, we have two values as an argument in our Arrow Function, first argument will hold our each element of our Array and second will hold its index number. The loop will keep looping through the Array till the Array ends and do a certain activity which we have given.
Objects
Objects are one of the fundamental building blocks in JavaScript, used to store collections of data and more complex entities. An object is a collection of properties, where a property is an association between a key (name) and a value.
Creating an Object
Objects are used to store multiple key and values in a single variable. As mentioned above unlike Arrays here we have key
instead of index
's and we can name the key as anything we want. For example:
let userData = {
name: "Aquib",
age: 22,
country: "India"
};
- Here the
name
is ourkey
and"Aquib"
is our value of that key, and so on. We can store as many data as we want inside the object.
Accessing Object Properties
console.log(userData.name); //Output: Aquib
console.log(userData.age); //Output: 22
- To access any objects property the most famous method is using
dot
, the name of the variable then adot
and thekey
of the object you want to access.
Adding and Modifying Properties
//Adding new data in our object
userData.job = 'Developer';
// Modifying our existing property
userData.age = 21;
Removing Properties
delete userData.job;
console.log(userData.job); //Undefined
For-In
Loop
The for-in
loop is used to iterate over the enumerable properties of an object. It is primarily used with objects, but it can also be used to iterate over the indexes of an array.
syntax
for (variable in object) {
// code block to be executed
}
When used with an object, the for-in
loop iterates over all enumerable properties of the object, including those inherited through the prototype chain.
let userData = {
name: "Aquib",
age: 22,
country: "India"
};
for (let key in userData) {
console.log(`${key}: ${userData[key]}`);
}
//Output:
//name: Aquib
//age: 22
//country: India
Example with Arrays
Although for-in
can be used to iterate over array indices, it's generally not recommended for arrays due to potential issues with the order of iteration and enumerability of properties. For arrays, for-of
or other methods like forEach
are usually better.
let fruits = ['Apple', 'Banana', 'Cherry'];
for (let index in fruits) {
console.log(`${index}: ${fruits[index]}`);
}
// Output:
// 0: Apple
// 1: Banana
// 2: Cherry
Functions
Functions are one of the fundamental building blocks in JavaScript. A function is a set of statements that perform a task or calculate a value. Functions can be defined in various ways, and JavaScript supports different types of functions, including named functions, anonymous functions, and arrow functions.
Function Declaration
function functionName(parameters) {
// function body
}
//Creating a function
function myFunc() {
console.log("Hello");
}
//Calling a function
myFunc();
Function while taking arguments in parameters:
function greeting(name) {
console.log("Hello, " + name);
}
greeting("Aquib"); //Output: Hello, Aquib
- Inside the parameters we can name anything and then it will greet us according to the given argument.
Functions with Return
Functions can return values using the return
statement. Once a return
statement is executed, the function stops executing and the value is returned to the caller.
function add(a, b) {
return a + b;
}
let result = add(3, 5);
console.log(result); // Output: 8
Anonymous Functions
Anonymous functions are functions without a name. They are often used as arguments to other functions or assigned to variables.
let greet = function(name) {
return "Hello, " + name;
};
console.log(greet("Aquib")); // Output: Hello, Aquib
Arrow Functions
Arrow functions provide a shorter syntax for writing functions. They are always anonymous and do not have their own this
, arguments
, super
, or new.target
.
syntax
(parameters) => {
// function body
}
We can write it in a single line as well, like:
(parameters) => expression
let greet = (name) => {
return "Hello, " + name;
};
console.log(greet("Aquib")); // Output: Hello, Aquib
- First we are saving our function inside of a variable then as you may have noticed in this Arrow function we don't have the keyword of
function
, instead we are using parentheses()
then an arrow sign=>
then our body of our function surrounded by curly braces{}
. This is a new way of writing our functions, introduced in ES6.
Writing the function in single line:
let greet = name => "Hello, " + name;
console.log(greet("Aquib")); // Output: Hello, Aquib
Subscribe to my newsletter
Read articles from Syed Aquib Ali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
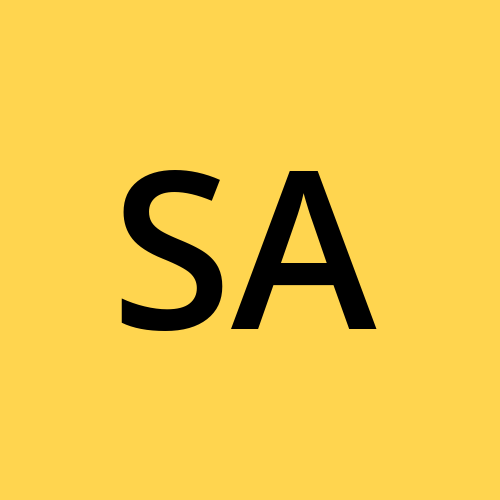
Syed Aquib Ali
Syed Aquib Ali
I am a MERN stack developer who has learnt everything yet trying to polish his skills 🥂, I love backend more than frontend.