How to Connect PostgreSQL Database to Your Django Project: Step-by-Step
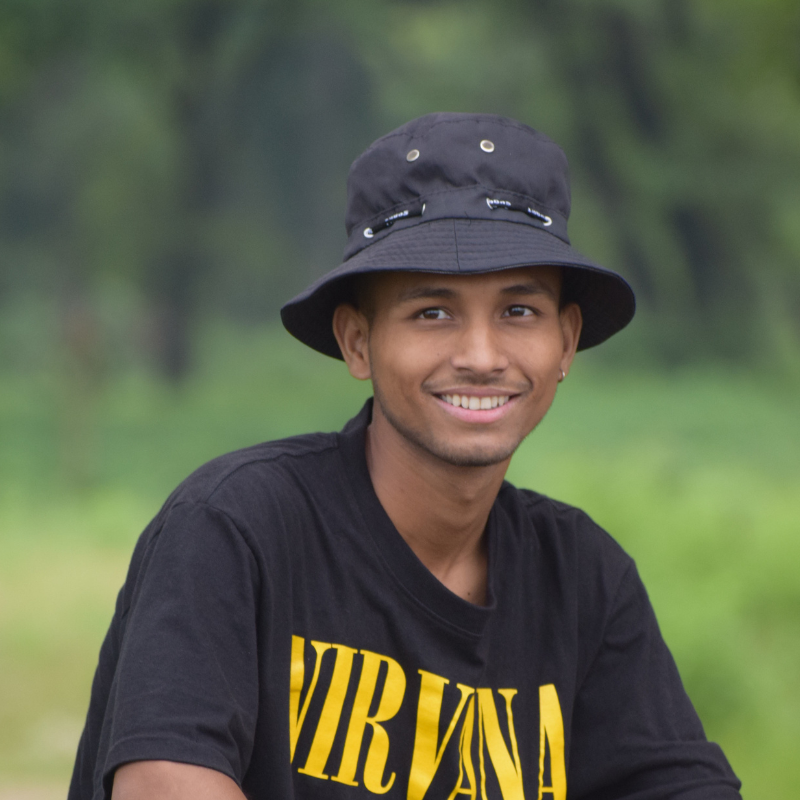
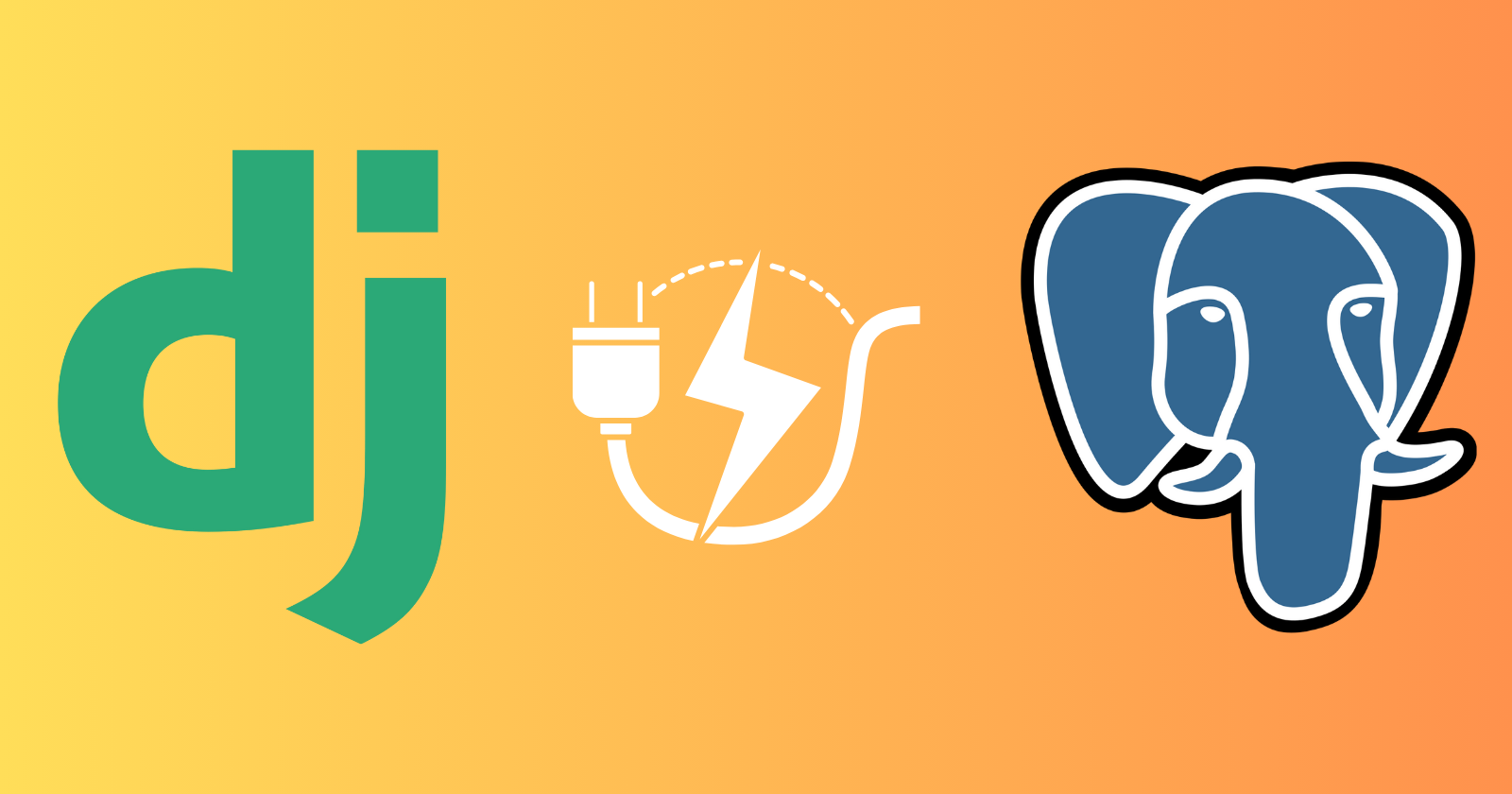
If you're starting a Django project and want to use PostgreSQL as your database backend, you're making a wise choice. PostgreSQL is a robust, open-source relational database management system that integrates seamlessly with Django, providing excellent performance and scalability. In this guide, we'll walk through the process of connecting a PostgreSQL database with a Django project, covering everything from installation to configuration.
Step 1: Install PostgreSQL
The first step is to install PostgreSQL on your system if you haven't already. Download the installer from the official PostgreSQL website and Follow the installation instructions provided for your operating system.
Step 2: Create a Database
Once PostgreSQL is installed, open your terminal or command prompt and log in to the PostgreSQL shell using the command:
psql -U postgres
Replace 'postgres' with your PostgreSQL username if it's different. Then, create a new database for your Django project:
CREATE DATABASE mydatabase;
Replace 'mydatabase' with your preferred database name.
Step 3: Create a Directory and Virtual Environment
Create a directory for your Django project and set up a virtual environment within it.
mkdir myproject
cd myproject
python3 -m venv venv
source venv/bin/activate # On Windows use `venv\Scripts\activate`
Step4 : Install Django and psycopg2
Before proceeding, with the virtual environment activated make sure you have Django installed. If not, you can install it using pip:
pip install Django
You also need to install psycopg2, which is the PostgreSQL adapter for Python:
pip install psycopg2
Step 5: Start the Django Project
Create a new Django project within your directory.
django-admin startproject myproject .
Step 6: Configure Django Settings
In your Django project directory, navigate to the settings.py file within your project's main app folder. Update the DATABASES configuration with your PostgreSQL database details:
Default Database Setting
By default, Django uses SQLite as the database. Here is the default configuration:
# myproject/settings.py
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': BASE_DIR / 'db.sqlite3',
}
}
New PostgreSQL Database Setting
Change the configuration to use PostgreSQL:
# myproject/settings.py
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.postgresql',
'NAME': 'mydatabase',
'USER': 'myuser',
'PASSWORD': 'mypassword',
'HOST': 'localhost',
'PORT': '5432',
}
}
Here's what each part of this configuration means:
ENGINE: Specifies the database backend to use. In this case, it's set to
'django.db.backends.postgresql'
, which tells Django to use the PostgreSQL backend.NAME: The name of your database. This should match the database name you created in PostgreSQL (
mydatabase
in this example).USER: The database user that Django should use to connect to the database. This should match the username you created in PostgreSQL (
myuser
in this example).PASSWORD: The password for the database user. This should match the password you assigned to the user in PostgreSQL (
mypassword
in this example).HOST: The host address of your PostgreSQL database. If your database is running on the same machine as your Django application, use
'
localhost
'
. For remote databases, use the appropriate IP address or domain name.PORT: The port number on which your PostgreSQL database is listening. The default port for PostgreSQL is
5432
.
Replace 'mydatabase', 'myuser', 'mypassword' with your actual database name, PostgreSQL username, and password respectively. The HOST should be 'localhost' if PostgreSQL is running locally. If you're using a different host or port, update those fields accordingly.
Step 7: Migrate and Create Superuser
Next, run the following commands in your terminal to migrate your Django project's initial database schema and create a superuser:
python manage.py migrate
python manage.py createsuperuser
Follow the prompts to create your superuser account, which you can use to access the Django admin interface.
Step 8: Test the Connection
To ensure that your Django project is successfully connected to the PostgreSQL database, start your Django development server:
python manage.py runserver
Open your web browser and navigate to http://127.0.0.1:8000/admin/. Log in using the superuser credentials you created earlier. If you can access the Django admin interface without any errors, congratulations! Your PostgreSQL database is successfully connected to your Django project.
Conclusion
In this guide, we've covered the entire process of connecting a PostgreSQL database with a Django project. From installing PostgreSQL and configuring Django settings to testing the connection, you now have a solid foundation to build upon. PostgreSQL's reliability and performance combined with Django's powerful features make for a robust and scalable web application framework. Happy coding!
Subscribe to my newsletter
Read articles from Pradip Thapa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
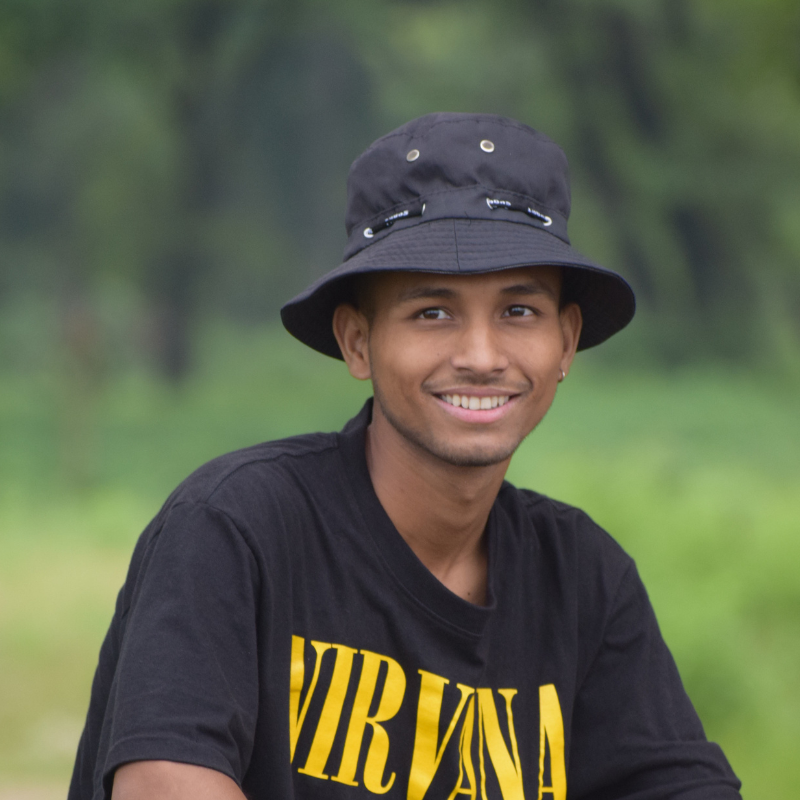
Pradip Thapa
Pradip Thapa
I am a self-taught programmer with a great passion for learning, exploring and developing new things. I also love to keep updated with the latest technologies.