Angular Series: Key Concepts in a Flash
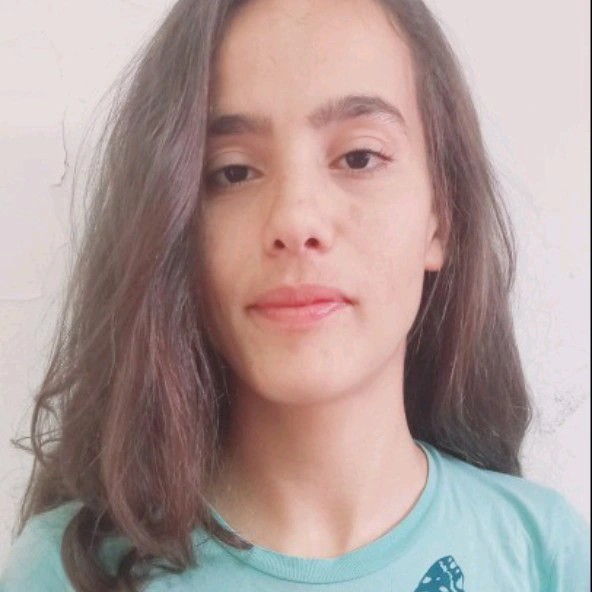
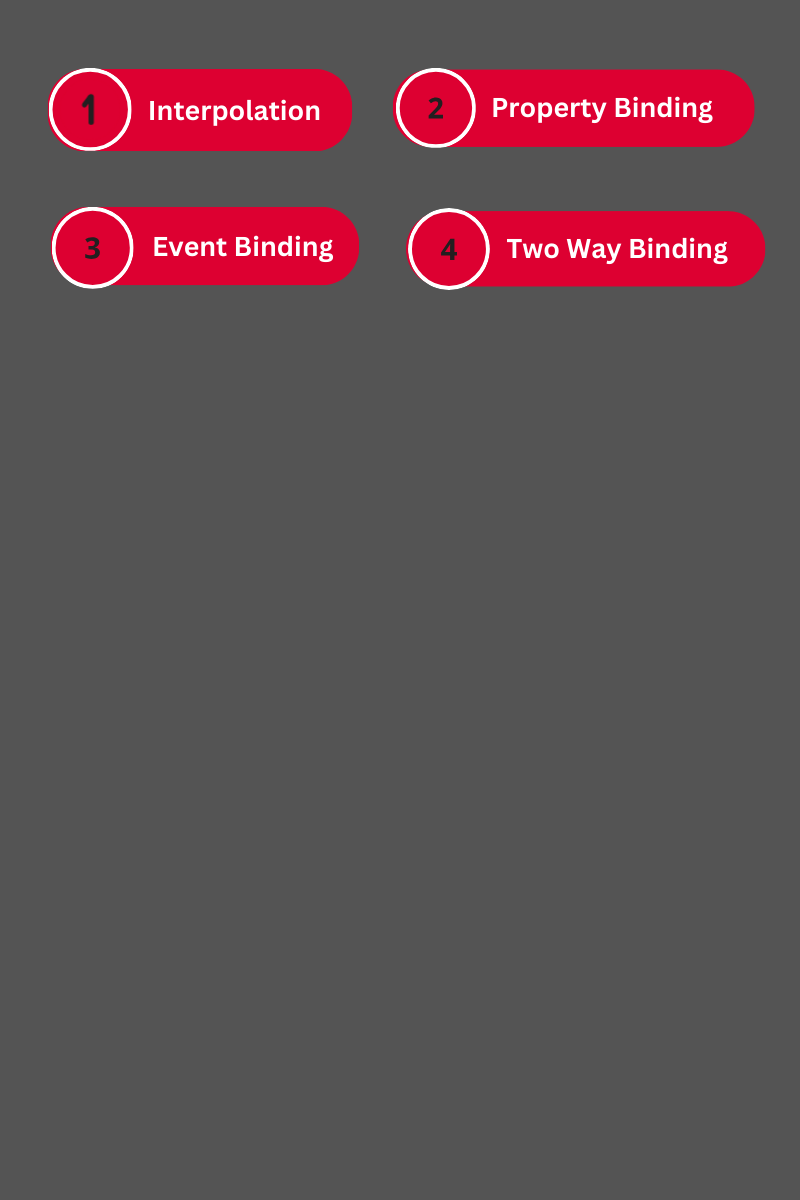
What Is Data Binding?
Data binding in Angular is a feature that allows automatic synchronization of data between the component class and the view, ensuring that any changes made in one are instantly reflected in the other. It simplifies the development process by minimizing the manual manipulation of the DOM and improving the responsiveness of web applications.
1.Interpolation: {{}}
Interpolation is a form of one-way data binding in Angular that allows you to insert a component’s property value into the view template. This is done using double curly braces {{}}. Any data placed within these curly braces are automatically converted to a string and displayed in the view.
Here's an example, you can set component property called title and initialize it :
@Component({
selector: 'app-first-component',
templateUrl: './first-component.component.html',
styleUrls: ['./first-component.component.css']
})
export class FirstComponentComponent {
title = "Angular Key Concepts in a Flash"
}
To display it in a heading element :
<h1>{{title}}</h1>
2.Property Binding:
Property binding is a type of one-way data binding in Angular that lets you assign a component property value to an HTML element’s property. This is achieved using square brackets []. This approach dynamically connects a component property with an HTML element’s property, ensuring that any changes in the component property are automatically updated in the HTML element's property value. This enhances the flexibility and interactivity of the web application.
For example, you can link a component property, such as imageURL, to an image element’s src property in the following way:
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-first-component',
templateUrl: './first-component.component.html',
styleUrls: ['./first-component.component.css']
})
export class FirstComponentComponent {
// title = "Angular Key Concepts in a Flash"
isDisabled = true;
imageURL = 'https://web/assets/images/angular/data-angular.png';
}
<!-- <h1>{{title}}</h1> -->
<button [disabled]="isDisabled">click-me</button>
<img [src]="imageURL"/>
3.Event Binding:
Event binding enables the connection of component methods to various user events such as clicks, hovers, and touches. This creates a one-way flow from the view to the component. By doing so, one can ensure that the component stays synchronized with the view by capturing and responding to user events.
For instance, when a user interacts with elements such as a text box, we can track input changes and trigger corresponding actions in the component. This allows us to update the component’s model and perform necessary validations, ensuring a seamless and responsive user experience. Event binding empowers developers to efficiently handle user interactions, enabling effective communication between the view and the component in a web application.
For example, it can be connected to a button element’s click event in the following way:
import { Component} from '@angular/core';
@Component({
selector: 'app-first-component',
templateUrl: './first-component.component.html',
styleUrls: ['./first-component.component.css']
})
export class FirstComponentComponent {
message: string = 'Not clicked'
displayMessage() {
this.message = 'You clicked the button!'
}
}
<button style="background: #de2828;" (click)="displayMessage()">{{this.message}}</button>
4.Angular Two-Way Data Binding:
Two-way data binding in Angular is a powerful mecanism that combines property binding and event binding. It allows you to establish a connection between a component property and an input element, enabling real-time synchronization between them.
To implement two-way data binding, you can use the "ngModel" directive. This directive binds the component property to the input element’s value and listens for changes to the input element, updating the component property accordingly. This bidirectional communication ensures that any modifications made to the input element by the user are instantly reflected in the component property, and vice versa.
Using the "ngModel" directive simplifies the process of managing data between the component and the view, resulting in a more responsive and interactive user experience.
For example, if you have a component property called message for example , you can bind it to an input element like this:
import { Component} from '@angular/core';
@Component({
selector: 'app-first-component',
templateUrl: './first-component.component.html',
styleUrls: ['./first-component.component.css']
})
export class FirstComponentComponent {
message:string = ""
}
<input [(ngModel)]= "message">
<p>{{ message }}</p>
let's summarize
Data binding in Angular is a robust feature that enables seamless communication between the component and the view. Whether employing one-way binding for efficient data flow or two-way binding for real-time synchronization, Angular's data binding mechanisms address a variety of requirements. By mastering event binding and property binding, developers can effortlessly build dynamic and interactive web applications.
Interpolation: {{}} – Used for one-way binding to display a component property value into the view template.
Property Binding: [] – Also a one-way binding. It allows you to set the value of an HTML element’s property based on a component class property.
Event Binding: () – Enables you to link component methods to user events (e.g., hovers, clicks , touches) in the view.
Two-way Binding: [()] – Two-way data binding in Angular combines property binding and event binding, providing bidirectional synchronization between a component property and an input element using the ngModel
directive.
Subscribe to my newsletter
Read articles from Meriem Trabelsi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
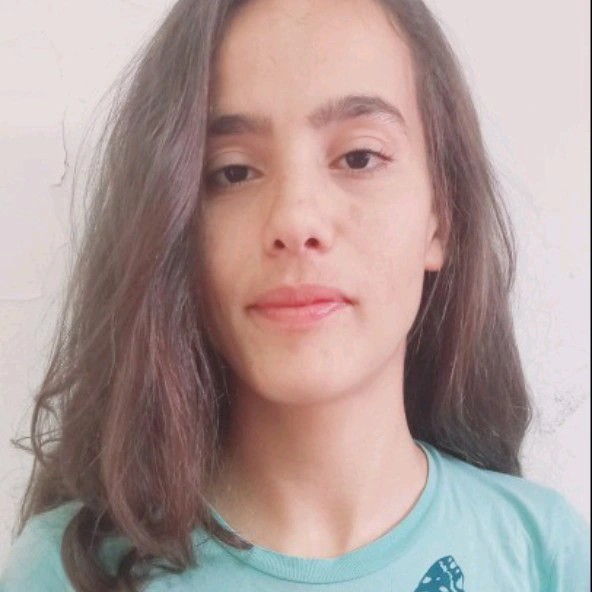
Meriem Trabelsi
Meriem Trabelsi
I am a curious, ambitious young woman passionate about the IT world 🙂. Acutually, I am software Engineering Student @ISI. More motivated to discover new technologies. I am interested in project management and the different approaches, business intelligence, web/mobile development, ISTQB (software testing) and so on ..