Getting Started with Next.js: A Beginner's Guide
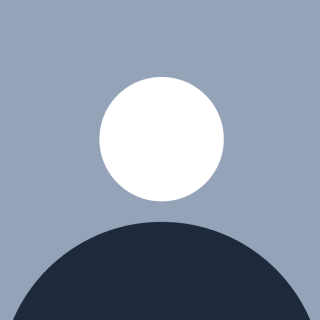
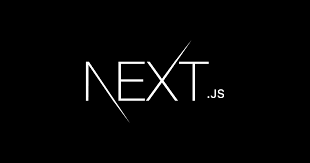
Next.js is a powerful framework built on top of React that provides an enhanced developer experience with features like server-side rendering (SSR), static site generation (SSG), and easy API routes. This guide will help you get started with Next.js, covering key concepts and providing code snippets to illustrate essential features.
Installation and Setup
To create a new Next.js project, use the following command:
npx create-next-app@latest my-next-app
cd my-next-app
npm run dev
This will set up a new Next.js application and start the development server on http://localhost:3000
.
File Structure
Next.js enforces a specific file structure to organize your application. Key directories include:
pages/
: Contains your application's pages. Each file in this directory becomes a route.public/
: Static files like images and fonts.styles/
: CSS and Sass files.
Creating Pages
Creating a new page is simple. Just add a new file in the pages
directory. For example, to create an "About" page:
// pages/about.js
import React from 'react';
const About = () => {
return (
<div>
<h1>About Us</h1>
<p>This is the about page.</p>
</div>
);
};
export default About;
Navigate to http://localhost:3000/about
to see your new page.
Dynamic Routes
Next.js supports dynamic routing. Create a dynamic route by using square brackets in the file name. For example, to create a blog post page:
// pages/posts/[id].js
import { useRouter } from 'next/router';
const Post = () => {
const router = useRouter();
const { id } = router.query;
return (
<div>
<h1>Post ID: {id}</h1>
</div>
);
};
export default Post;
Access this page with http://localhost:3000/posts/1
where 1
can be any ID.
API Routes
Next.js allows you to create API endpoints within your application. Add a new file in the pages/api
directory:
// pages/api/hello.js
export default function handler(req, res) {
res.status(200).json({ message: 'Hello, world!' });
}
Access this API route at http://localhost:3000/api/hello
.
Conclusion
Next.js streamlines the development process by combining the best parts of React with powerful built-in features like SSR, SSG, and API routes. By understanding the basics of setting up pages, dynamic routing, and creating API routes, you can quickly build robust applications. Explore more features and dive deeper into Next.js to fully leverage its capabilities for your next project.
Subscribe to my newsletter
Read articles from Yash Mandi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Yash Mandi
Yash Mandi
ms grad. software engineer intern. java. springboot. currently working on mern stack. freelancer