Daily Hack #day66 - Fast File Copy using Java NIO
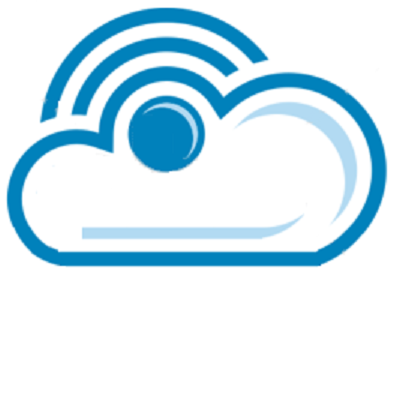
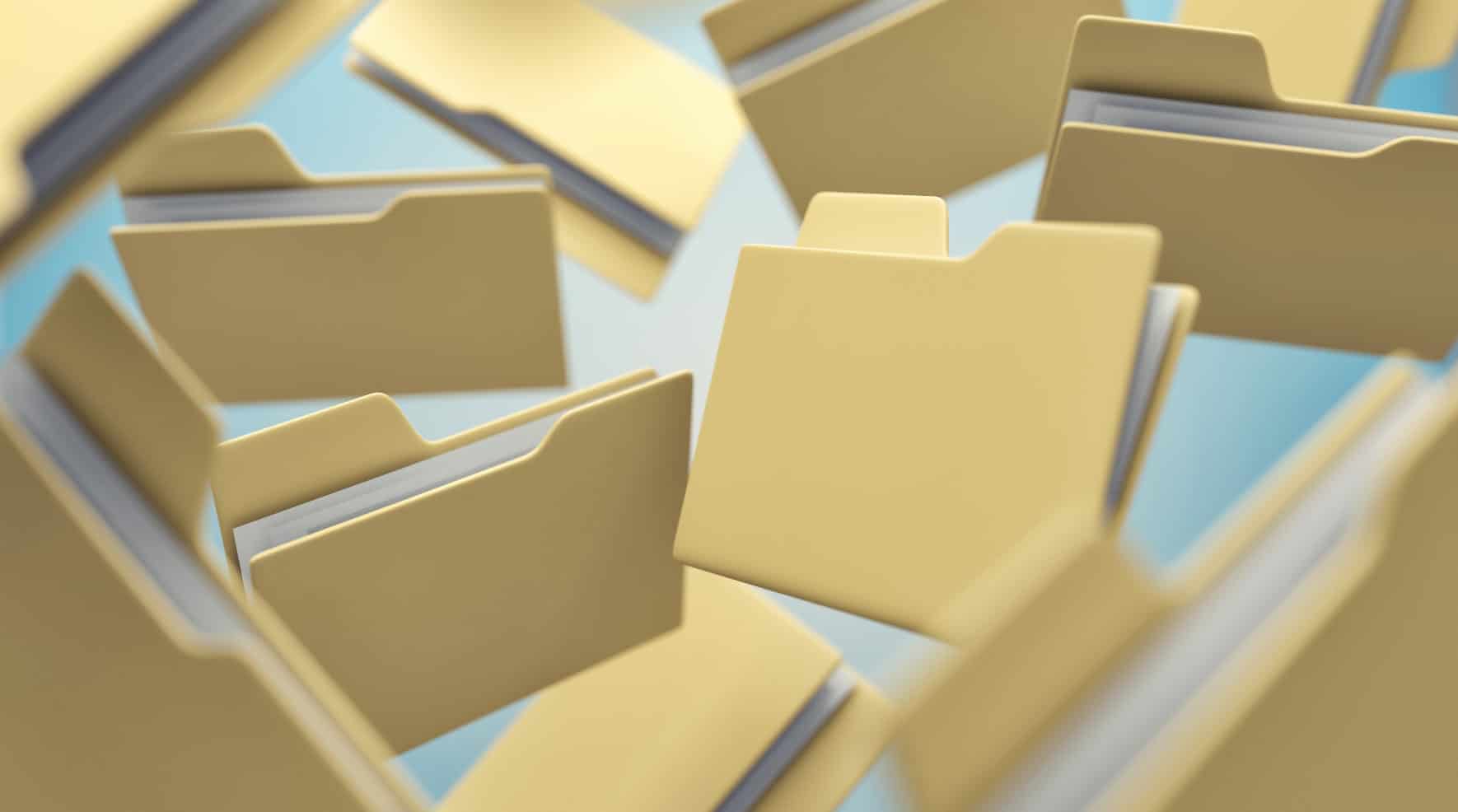
Java NIO (New Input/Output) provides a powerful set of APIs to handle file operations efficiently. For fast file copying, we can use the FileChannel
class which is part of the NIO package. The FileChannel
class allows for high-performance file operations, including transferring data between channels directly.
Here's an example of how to perform fast file copying using Java NIO:
Fast File Copy using Java NIO
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardCopyOption;
import java.nio.channels.FileChannel;
import java.nio.file.StandardOpenOption;
public class FastFileCopy {
public static void main(String[] args) {
if (args.length < 2) {
System.out.println("Usage: FastFileCopy <source file> <destination file>");
return;
}
Path sourcePath = Paths.get(args[0]);
Path destinationPath = Paths.get(args[1]);
try {
copyFileUsingFileChannel(sourcePath, destinationPath);
System.out.println("File copied successfully.");
} catch (IOException e) {
e.printStackTrace();
System.out.println("Failed to copy the file.");
}
}
private static void copyFileUsingFileChannel(Path source, Path destination) throws IOException {
// Create parent directories if they do not exist
if (Files.notExists(destination.getParent())) {
Files.createDirectories(destination.getParent());
}
try (FileChannel sourceChannel = FileChannel.open(source, StandardOpenOption.READ);
FileChannel destinationChannel = FileChannel.open(destination, StandardOpenOption.CREATE, StandardOpenOption.WRITE)) {
// Transfer data from source to destination
long position = 0;
long size = sourceChannel.size();
while (position < size) {
long transferred = sourceChannel.transferTo(position, size - position, destinationChannel);
position += transferred;
}
}
}
}
Explanation
Imports: We import necessary classes from
java.nio.file
andjava.nio.channels
.Main Method:
We check if the user provided both source and destination file paths.
We convert the provided file paths to
Path
objects.
copyFileUsingFileChannel Method:
Create Parent Directories: If the destination's parent directories do not exist, we create them using
Files.createDirectories()
.File Channels: We open a
FileChannel
for the source file in read mode and another for the destination file in write mode. TheStandardOpenOption.CREATE
option is used to create the destination file if it does not exist.Transfer Data: We use the
transferTo
method ofFileChannel
to transfer data from the source to the destination channel. This method is efficient as it can take advantage of low-level system operations.
Exception Handling: Proper exception handling ensures that any I/O errors during the file operations are caught and reported.
Usage
Compile and run the program with the source and destination file paths as arguments:
javac FastFileCopy.java
java FastFileCopy sourceFilePath destinationFilePath
Replace sourceFilePath
and destinationFilePath
with the actual paths of the files you want to copy.
This approach ensures a fast and efficient file copy operation using Java NIO's FileChannel
.
Subscribe to my newsletter
Read articles from Cloud Tuned directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
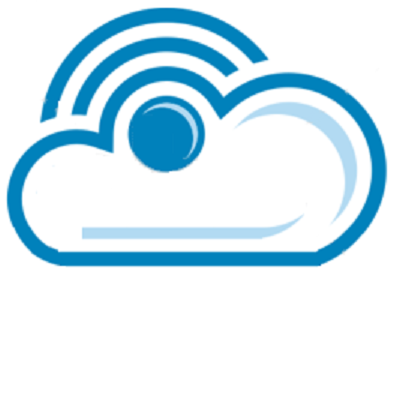