Introduction to JavaScript
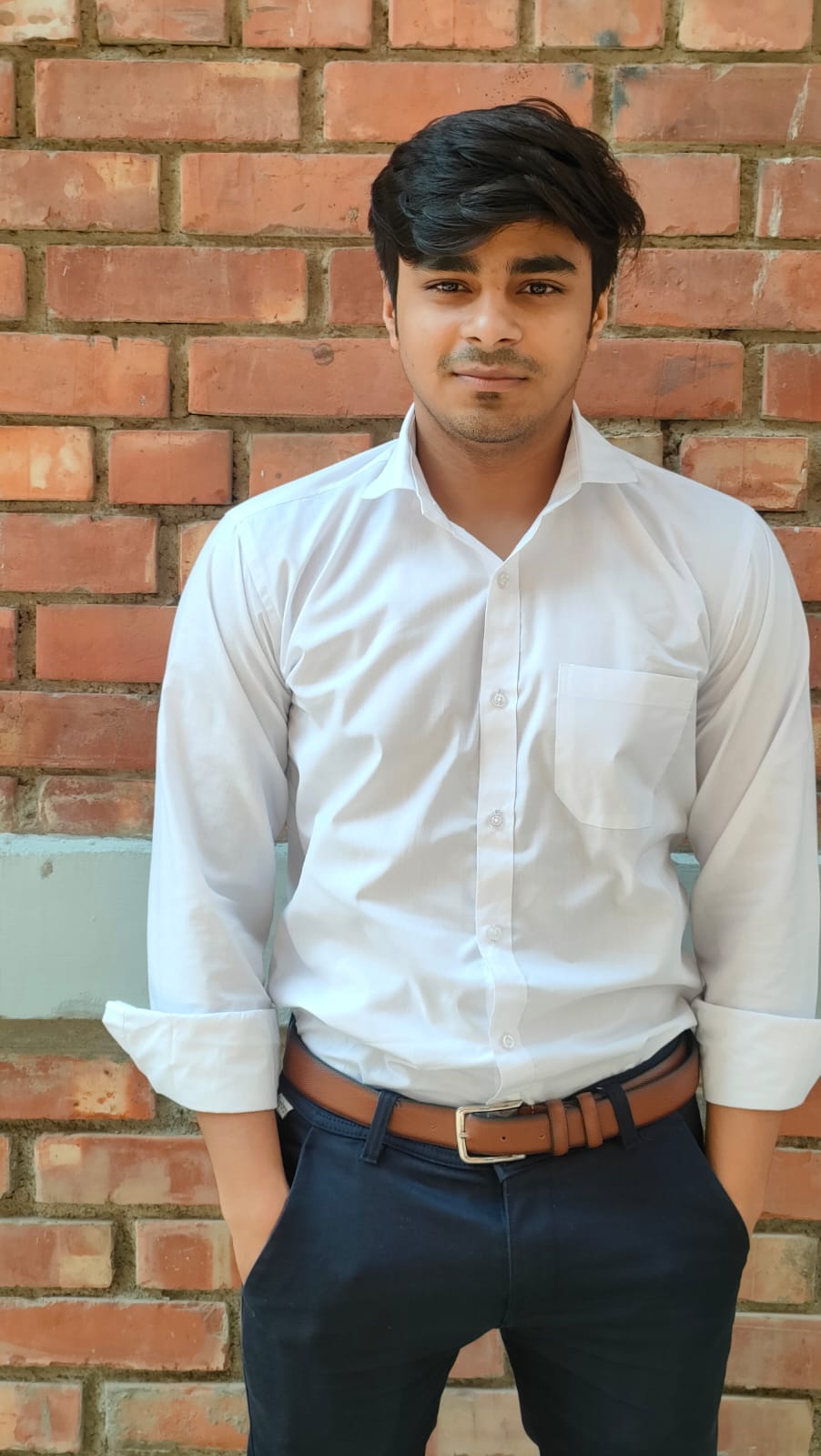
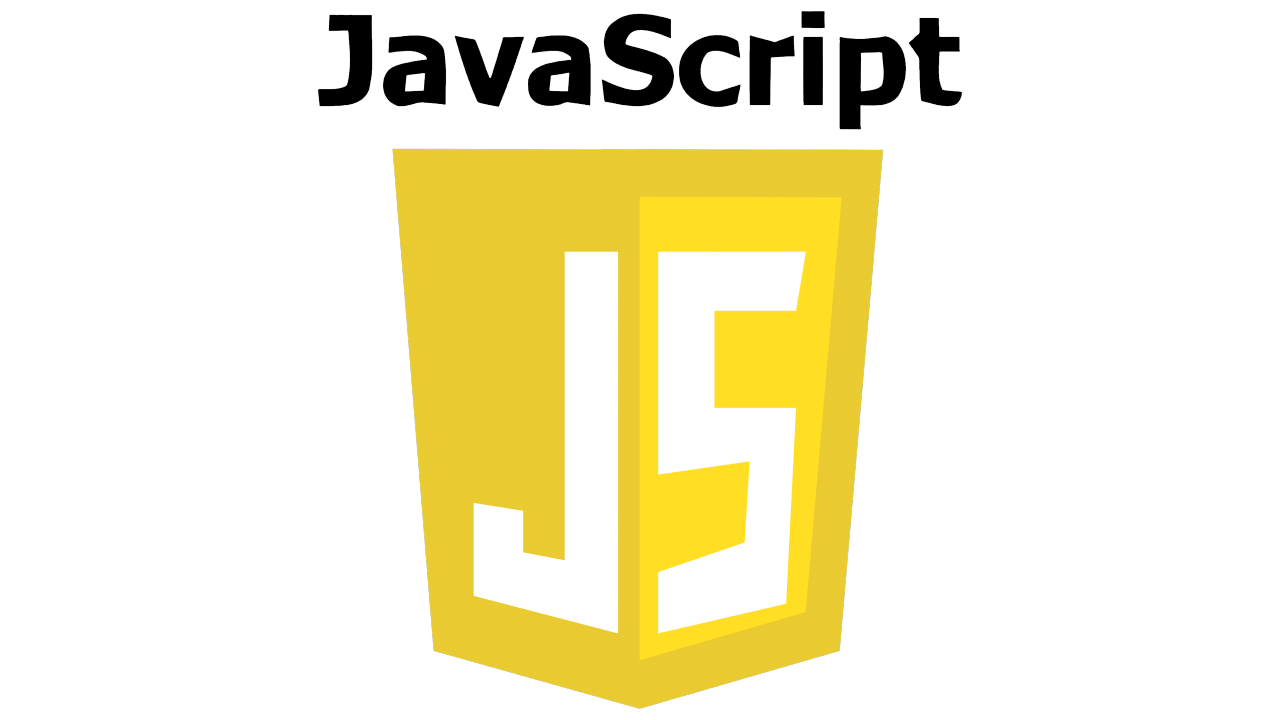
Hi Folks, In this article we are going to learn about basics of JavaScript. JavaScript is majorly used in web development, mobile app development etc. It uses in frontend and in backend as well which makes it most important part of web development.
Let's start with variables:-
Variables
Many people thinks variable is container which stores our data, which is partially correct because it does not stores data rather it actually points our data. Refer below image for better understanding
In any programming languages, to store a data in memory we need a reference that points our data, e.g. If I want to store a name then I cannot just write "Bharat Singh", there are some set of rules or we can say syntax of programming language.
I have to declare a variable with declarative e.g. let myName = "Bharat Singh";
, "Bharat Singh" has been storing in the memory and myName
is pointing that memory block and let
is a declaratives.
If I want to use this name in my code then I will call it by calling variable name, let greet = "Hello from " + myName;
, now greet is pointing towards "Hello from Bharat Singh" , this is how we uses variables.
Keywords
Keywords are predefined words which has some specific meaning and we cannot use them to make variables.
e.g. let, var, const, for, catch, await, async, etc.
Declaratives
Declaratives helps in declare a variable, without declarative we can't declare a variable. In JavaScript, we have three types of declaratives:-
Let
var
const
Every declarative comes with its unique features, basically they decide where our variable can be accessed. We'll learn about declaratives in detail in next blog.
Data Type
As name suggests, "type of data", we have data of different types e.g. "1234" it is number, "ABCD" it is a string, etc. Every data we write in the code, it has some type.
Primitives
Basic data types which cannot be broken down further are called primitives or we can say smallest unit in the language are primitives.
we have 7 types of Primitives
Number
String
Boolean
Null
Undefined
Symbol
BigInt
1. Number
As name suggests, a no. is any numeric value e.g. 123, 1.2, 1254 etc
2. String
A string is a character or collection of character, e.g. "a" , "abc" , "Bharat Singh", we always write string in double apostrophes ("abc") and a character in single apostrophes ('a').
3. Boolean
A boolean is true or false value.
4. Null
Null data type mean our variable has no value, let name = null;
means our variable is empty.
5. Undefined
Undefined means our variable has been declared but has not been assigned yet. let name;
6. Symbol
Symbol
is a built-in object whose constructor returns a symbol
primitive — also called a Symbol value or just a Symbol. (MDN Docs Definition). You'll understand about symbol once you study about constructors and objects.
7. BigInt
BigInt means, very large numeric value, e.g 1234524526314789 etc.
For Your Information
You are rarely going to use symbol
and BigInt
.
Difference between Undefined and Null
You'll find many people confused between undefined and Null data type,
Null is assigned value, which means null has to be given explicitly, e.g.
let num = null;
, it means num variable has no value, it is empty.Undefined is not assigned value, which means whenever we declare variable without assigning a value then our data has not been defined yet, so our variable points to undefined.
let num;
Let's understand the real life difference between undefined and Null through a real life example:-
Suppose you made a weather app and it shows a temperature which is of Number type , you want to add a feature which will be, whenever app is unable to identify a temperature then it should return some value and what if it returns zero in this case, which is logically wrong because zero is a temperature. So, in this case you'll use a Null
data type, it will return null when it is unable to identify a temperature.
Rules to write variable names
we can write variable name as a to z , A to Z, 0-9. ✅
We can use underscore(_) , $ , all special characters. ✅
We cannot start our variable name by a no. e.g. 1Bharat ❌
We cannot use keywords (for, let , var) ❌
Ways to declare variables
Normal :-
let bharatsingh = 10;
CamelCase:-
let BharatSingh = 10;
Snake_Case:-
let Bharat_SIngh = 10;
Print Function'
If we want to print anything in the JavaScript then we have print function. i.e. console.log();
, whatever we want to print we write inside the print function.
let num = 10;
console.log(num); // print 10
//As we are calling num varible, so it is printing it's value i.e. 10
//------------------------------------------------------------------------
console.log("Bharat Singh"); // print Bharat Singh
console.log("100"); // print 100
//whenever we write something under double quote then
// it prints that particular statement
Note:-
JavaScript is the very much important in our web development journey. I would suggest spend as much time as you can in learning fundamentals and basics of JavaScript, This note is for mee too 😊 .
Thank you so much for reading it completely, Go and get your hands dirty on it, try all the code by yourself.
If you liked it do like, comment, share and follow for more content. Comment for any feedback.
Subscribe to my newsletter
Read articles from Bharat Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
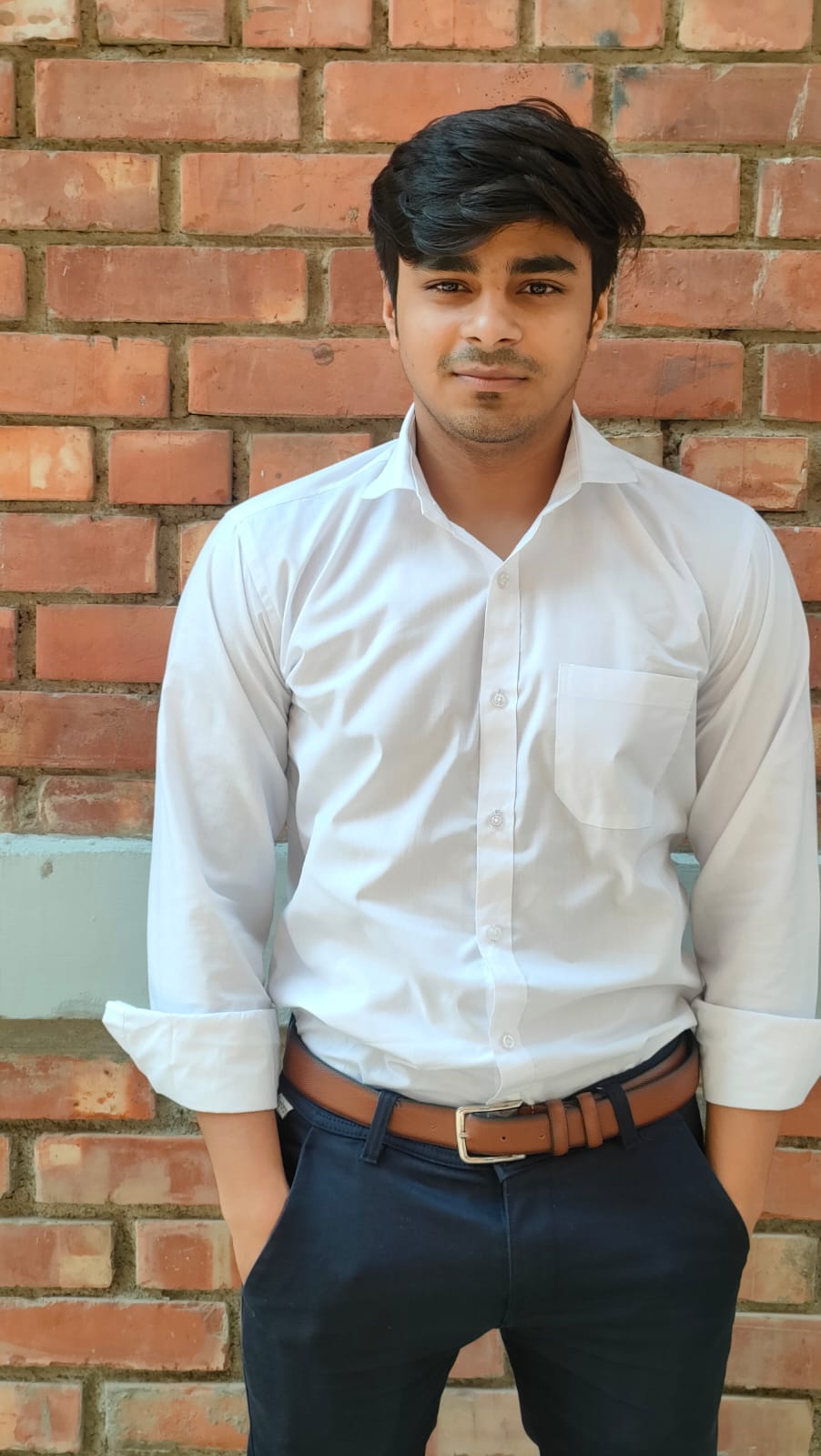
Bharat Singh
Bharat Singh
I am an engineering student , currently learning web development .