Level Up Your Laravel with Dependency Injection: A Guide for Cleaner, Testable Code
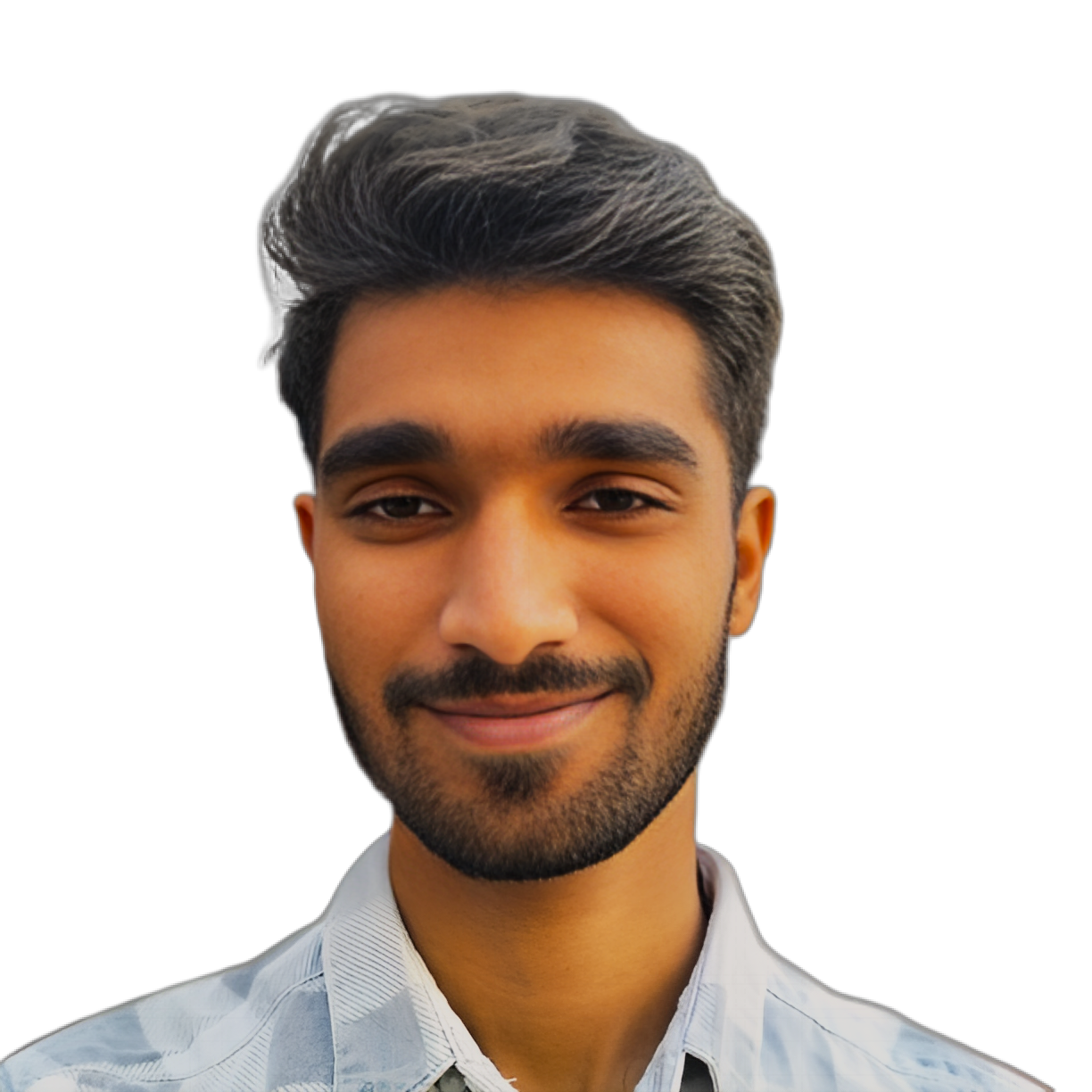
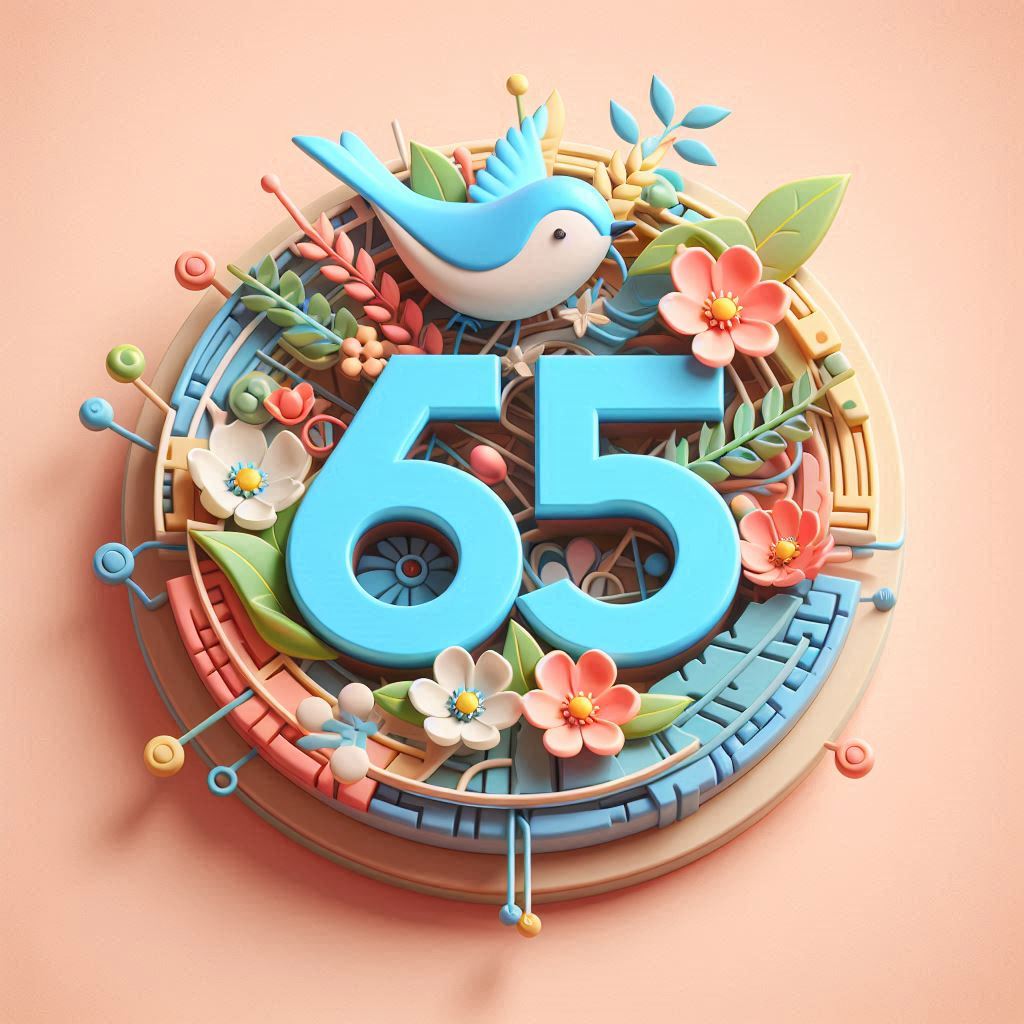
Hey there, Laravel developers! Buckle up, because we're diving into the fantastic world of Dependency Injection (DI) and how it can supercharge your Laravel projects. Get ready to write code that's clean, modular, and a breeze to test – all thanks to the magic of DI.
What's the Deal with Dependency Injection?
Imagine you have a class responsible for managing users. Traditionally, it might create a database connection itself to fetch user data. But what if you could tell this class exactly what it needs to function, like handing it a pre-made database connection object? That's the essence of DI – providing a class with its dependencies instead of making it create them on its own.
This approach offers several benefits:
Loose Coupling: Classes become less dependent on how their dependencies are created. This makes them more flexible and easier to work with in different contexts.
Improved Testability: When a class doesn't create its own dependencies, you can easily inject mock objects during testing. This isolates the functionality you're testing and ensures things work as expected.
Laravel's Service Container: The DI Powerhouse
Laravel provides a built-in service container, essentially a registry for objects and their dependencies. This container takes care of object creation and injection, making your life as a developer much easier. Here's the cool part: Laravel can automatically resolve dependencies based on the type hints you specify in your class constructors.
Let's see this in action with a simple example:
Our User Service Class:
PHP
class UserService
{
private $userRepository;
public function __construct(UserRepository $userRepository)
{
$this->userRepository = $userRepository;
}
public function getUser($id)
{
return $this->userRepository->find($id);
}
}
Binding the UserRepository in Laravel (config/app.php):
PHP
App::bind(UserRepository::class, UserRepository::class);
In this example, the UserService
class relies on a UserRepository
to interact with user data. By type-hinting UserRepository
in the constructor, we tell Laravel what dependency this class needs. When we create a new UserService
instance, Laravel automatically retrieves the corresponding UserRepository
object from the service container and injects it during construction. This eliminates the need for manual object creation and keeps our code clean.
Benefits Galore: Why You Should Embrace DI
Now that you've seen DI in action, let's explore the goodies it brings to your Laravel projects:
Testing Made Easy: As mentioned before, DI makes unit testing a breeze. You can mock dependencies like the
UserRepository
for isolated testing, ensuring yourUserService
functions as expected without relying on external factors.Maintainability Magic: DI promotes cleaner code by separating object creation logic from core functionality. Classes become more focused on what they do best, leading to better maintainability and reusability of your codebase. No more spaghetti code nightmares!
Reusable Goodness: By injecting dependencies, you avoid code duplication. Need user data in multiple places? Inject the
UserRepository
wherever it's needed, keeping your code DRY (Don't Repeat Yourself).
Putting It All Together: A Step-by-Step Tutorial
Ready to get your hands dirty with DI? Here's a step-by-step guide to get you started:
Define Your Interfaces: Create interfaces for your dependencies. This promotes loose coupling and allows for flexibility in implementing these dependencies.
Implement Your Concrete Classes: Build the actual classes that fulfill the defined interfaces. These classes will handle the specific logic for interacting with databases, services, etc.
Type-Hint in Constructors: When creating a class that relies on another, specify the dependency type in its constructor using type hinting (e.g.,
public function __construct(UserRepository $userRepository)
).Bind in Service Container (Optional): If your dependency class doesn't have complex logic, Laravel might be able to resolve it automatically. However, for more intricate dependencies, you can explicitly bind them in the
config/app.php
file usingApp::bind()
.Enjoy the Benefits! With DI in place, you can now leverage constructor injection and the service container to manage dependencies effectively. Write cleaner, more maintainable, and highly testable code that will make your future self (and your colleagues) very happy.
Bonus Tip: Explore Advanced Techniques
As you become more comfortable with DI in Laravel, here are some advanced techniques to consider incorporating into your projects:
- Method Injection: While constructor injection is the most common approach, DI can also be achieved through method injection. This involves injecting dependencies using setter methods in your class. This approach can be useful for situations where you need more control over when and how dependencies are injected.
Here's an example of method injection:
PHP
class UserService
{
private $userRepository;
public function setUserRepository(UserRepository $userRepository)
{
$this->userRepository = $userRepository;
}
public function getUser($id)
{
return $this->userRepository->find($id);
}
}
In this example, the UserService
class doesn't require the UserRepository
in its constructor. Instead, it provides a setUserRepository
method that allows you to inject the dependency later. This can be useful for scenarios where the dependency might not be available during object creation.
- Singleton vs. Transient Bindings: By default, Laravel uses transient bindings for dependencies. This means a new instance is created each time the dependency is resolved. However, you can also define singleton bindings in the service container using
App::singleton()
. Singletons ensure only one instance of a class exists throughout the application's lifecycle.
Here's an example of a singleton binding:
PHP
// config/app.php
App::singleton(Cache::class, function ($app) {
return new Cache(config('cache.default'));
});
In this example, the Cache
class is bound as a singleton. This means only one instance of the Cache
object will be created and used throughout the application. This can be beneficial for services like caching where you want a single point of access to cached data.
Remember, choosing between transient and singleton bindings depends on the specific needs of your application and the lifecycle of your dependencies.
By exploring these advanced techniques, you can further enhance the flexibility and control you have over dependency management in your Laravel projects.
Conclusion: DI – Your Key to Maintainable and Testable Laravel Code
Dependency Injection is a powerful tool that can significantly improve the quality of your Laravel code. By embracing DI, you can write cleaner, more maintainable, and highly testable codebases. So, the next time you're building a Laravel application, remember the magic of DI and leverage it to its full potential. Happy coding!
Subscribe to my newsletter
Read articles from Asis Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
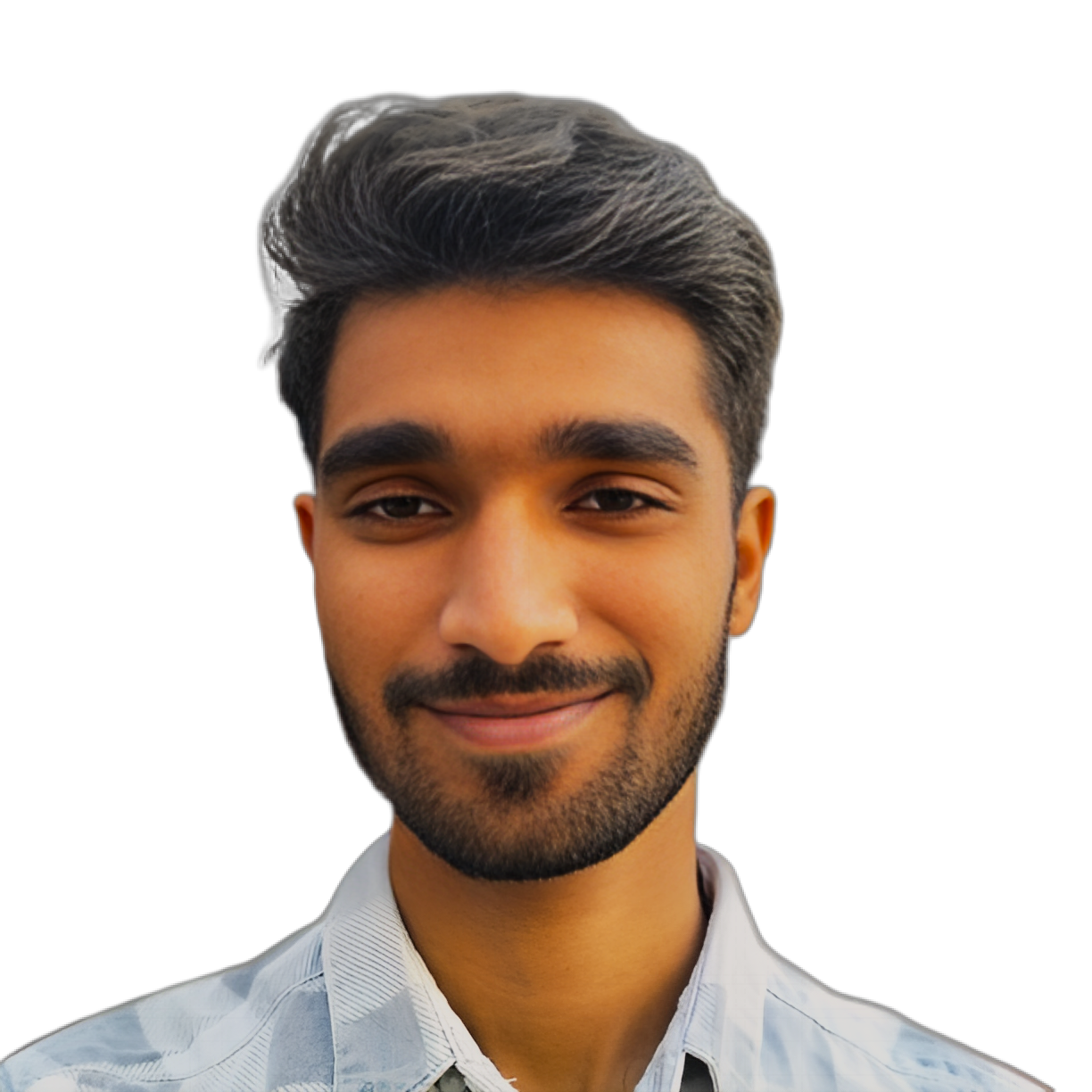
Asis Sharma
Asis Sharma
I'm an ambitious and motivated software developer, eager to apply my knowledge and skills to contribute to the development of innovative and cutting-edge software solutions. I'm well-versed in a variety of programming languages, including Javascript, C++, and Node.js, and have a understanding of object-oriented programming and algorithms. I'm passionate about developing and I am eager to learn and collaborate with others.