Week of June 3 2024 - Mindmap Recap

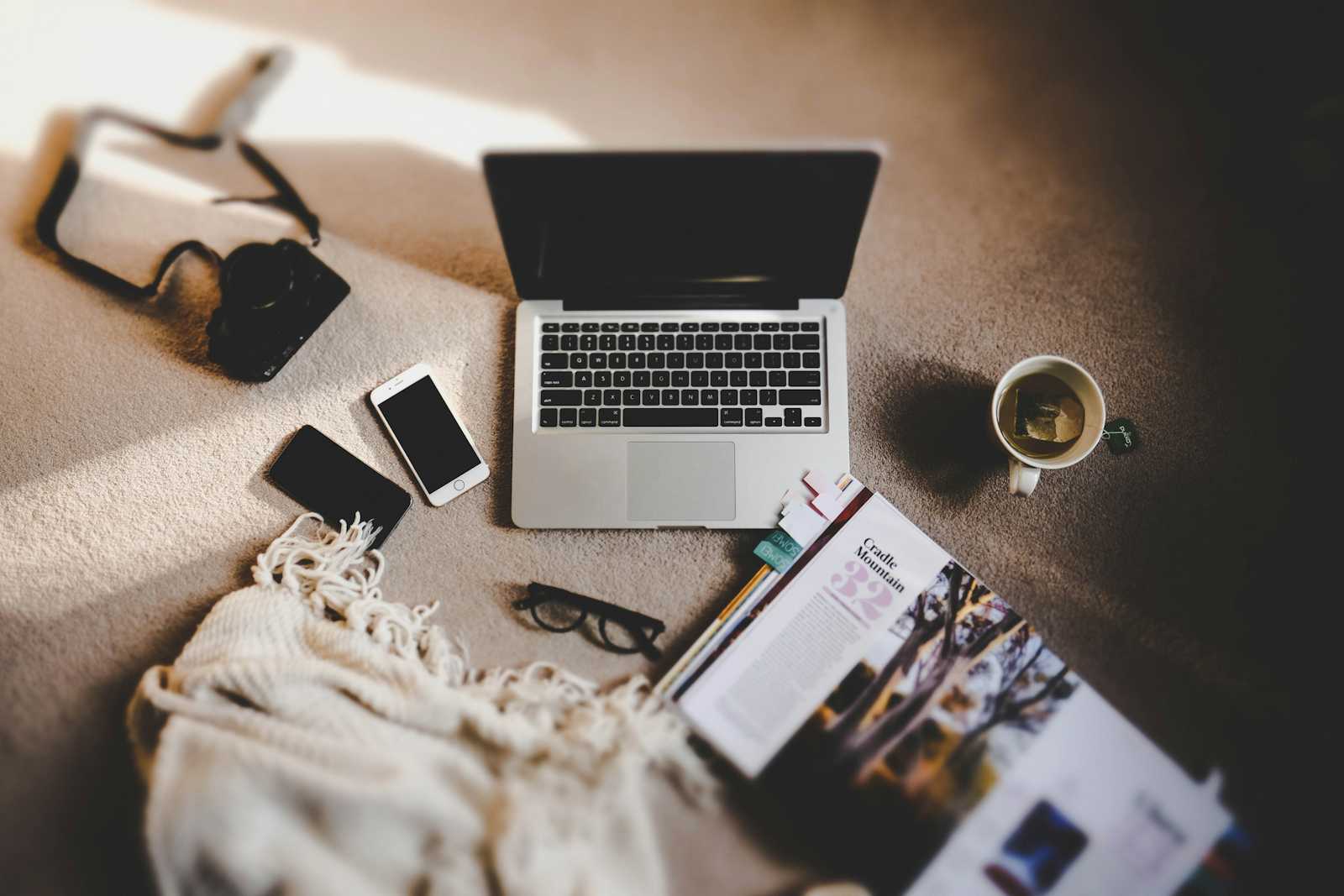
The importance of abstraction, reusability, error handling, efficient data manipulation, robust string handling, and performance optimization.
Adopting these principles leads to cleaner, more maintainable, and high-performance code that becomes crucial for building scalable and reliable software systems.
TSQL - Date and Time Manipulation
Using DATEADD
in T-SQL:
- DATEADD
illustrates the importance of having flexible and powerful date manipulation functions within a database.
- It highlights the need for precise time-based calculations, which are critical for applications involving scheduling, logging, and time-based analytics.
TSQL - Efficient Data Operations
Inserting Multiple Rows in T-SQL:
- Using a single INSERT
statement to add multiple rows demonstrates an efficient way to perform bulk operations.
- This practice reduces the number of database round-trips, improving performance and reducing latency.
C# - Abstraction and Code Reusability
Returning Empty Collections and Tasks:
- Using Enumerable.Empty<T>()
and Task.CompletedTask
are examples of abstraction. These methods provide a standard way to return empty results, enhancing code readability and reducing the need for custom implementations.
- This encourages reusability and consistency across the codebase.
C# - Error Handling and Robustness
Handling Failed Tasks:
- Task.FromException(new Exception())
demonstrates a standardized way to handle and propagate errors in asynchronous programming.
- Emphasizes the importance of robust error handling mechanisms to ensure that exceptions are managed in a predictable manner.
C# - String Handling and Comparison
String Comparisons:
- Using String.Equals
and StringComparison.OrdinalIgnoreCase
underlines the importance of handling null
values and case sensitivity in string operations.
- Ensures that string comparisons are accurate and robust, preventing potential bugs due to case differences or null references.
Standardization and Best Practices
Across all the topics, there is a common theme of following best practices and standardization:
- Consistent Methods: Using standard library methods (like Enumerable.Empty<T>()
and Task.CompletedTask
) ensures that the codebase adheres to widely accepted practices.
- Predictable Behavior: Functions like DATEADD
and String.Equals
provide predictable and reliable behavior, which is essential for maintaining software stability.
Performance Optimization
Efficient Data Operations and Asynchronous Programming:
- By using efficient data operations (like batch inserts) and asynchronous programming patterns (like returning completed or failed tasks), the software can handle larger loads and perform better under stress.
Subscribe to my newsletter
Read articles from Joubin Najmaie directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
