JavaScript Loops :
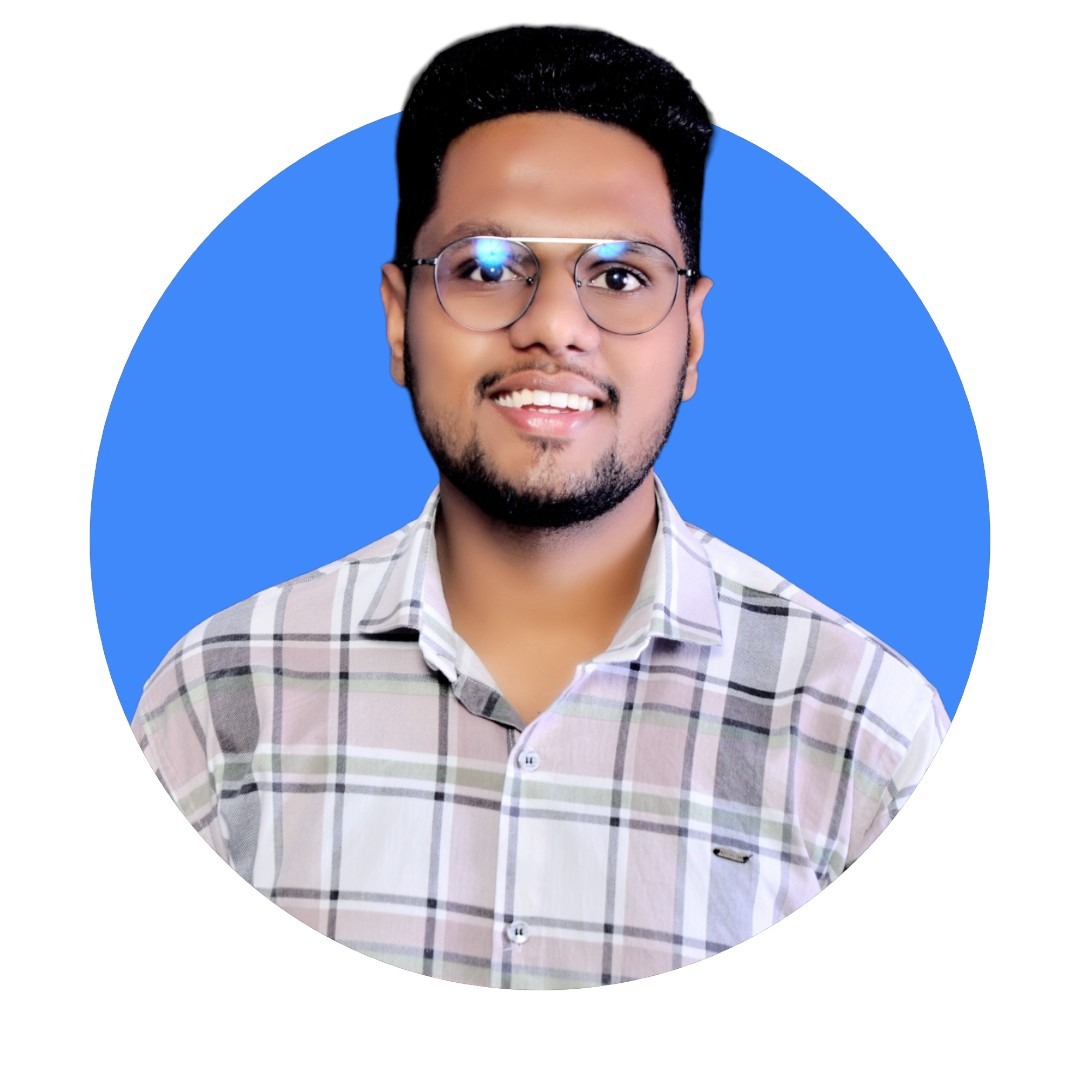
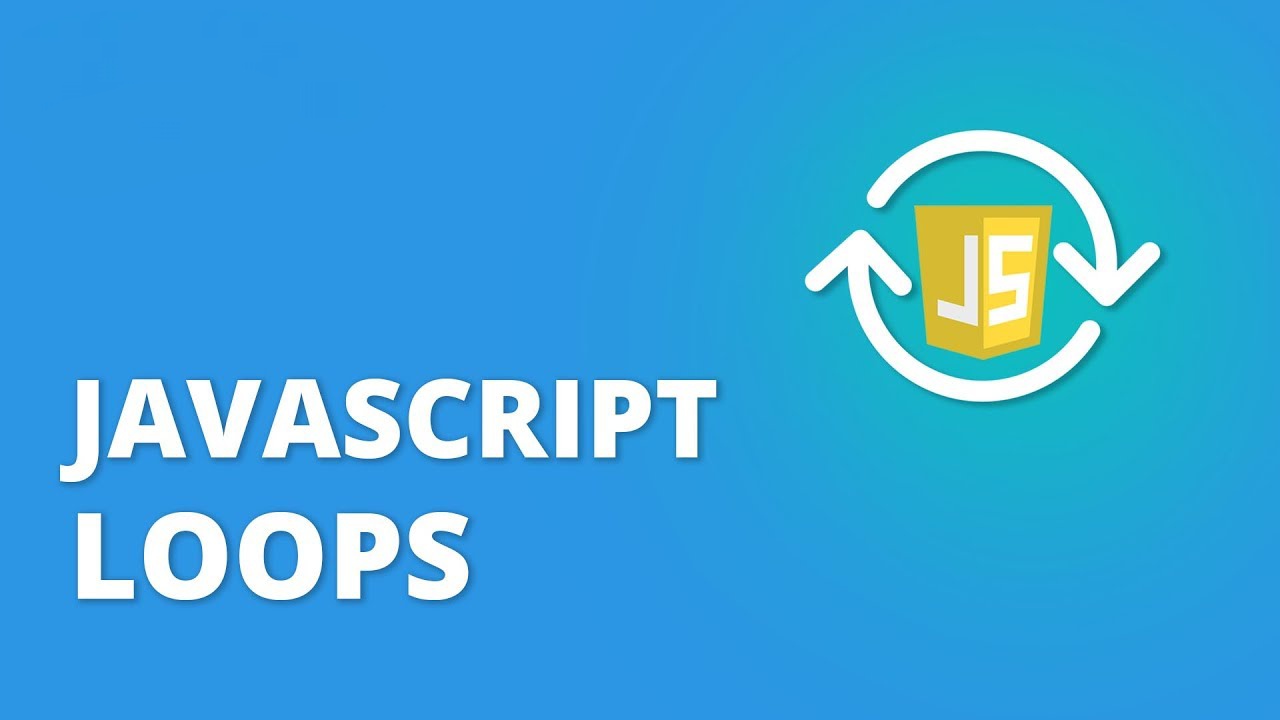
There are mainly two types of loops.
Entry Controlled loops:
In this type of loop, the test condition is tested before entering the loop body. For Loop and While Loops are entry-controlled loops.
Exit Controlled Loops:
In this type of loop the test condition is tested or evaluated at the end of the loop body. Therefore, the loop body will execute at least once, irrespective of whether the test condition is true or false. the do-while loop is exit controlled loop.
The JavaScript loops are used to iterate the piece of code using for, while, do while or for-in loops. It makes the code compact. It is mostly used in array.
There are four types of loops in JavaScript.
for loop
while loop
do-while loop
for-in loop
1) JavaScript For loop :
The JavaScript for loop iterates the elements for the fixed number of times.
It should be used if number of iteration is known.
for loop is a control flow statement that allows code to be executed repeatedly based on a condition.
It consists of three parts: initialization, condition, and increment/decrement.
This loop iterates over a code block until the specified condition is false.
The syntax of for loop is given below.
Syntax:
for (statement 1 ; statement 2 ; statement 3){
code here...
}
Statement 1: It is the initialization of the counter. It is executed once before the execution of the code block.
Statement 2: It defines the testing condition for executing the code block
Statement 3: It is the increment or decrement of the counter & executed (every time) after the code block has been executed.
Let’s see the simple example of for loop in javascript.
<!DOCTYPE html>
<html>
<body>
<script>
for (i=1; i<=5; i++)
{
document.write(i + "<br/>")
}
</script>
</body>
</html>
Output:
1
2
3
4
5
Flow chart
This flowchart shows the working of the for loop in JavaScript. You can see the control flow in the For loop.
2) JavaScript while loop :
JavaScript while loop iterates the elements for the infinite number of times.
It should be used if number of iteration is not known.
While Loop Syntax:
while (condition) {
Code block to be executed
}
Example:
<!DOCTYPE html>
<html>
<body>
<script>
var i=11;
while (i<=15)
{
document.write(i + "<br/>");
i++;
}
</script>
</body>
</html>
Output:
11
12
13
14
15
While loop starts with checking the condition. If it is evaluated to be true, then the loop body statements are executed otherwise first statement following the loop is executed. For this reason, it is also called the Entry control loop
Once the condition is evaluated to be true, the statements in the loop body are executed. Normally the statements contain an updated value for the variable being processed for the next iteration.
When the condition becomes false, the loop terminates which marks the end of its life cycle.
3) JavaScript do while loop :
The JavaScript do while loop iterates the elements for the infinite number of times like while loop.
But, code is executed at least once whether condition is true or false.
A do…while loop in JavaScript is a control structure where the code executes repeatedly based on a given boolean condition.
It’s similar to a repeating if statement. One key difference is that a do…while loop guarantees that the code block will execute at least once, regardless of whether the condition is met initially or not.
The syntax of do while loop is given below
Syntax:
do {
// Statements
}
while(conditions)compare the while and do…while loop
Flowchart :
The do-while loop starts with the execution of the statement(s). There is no checking of any condition for the first time.
After the execution of the statements and update of the variable value, the condition is checked for a true or false value. If it is evaluated to be true, the next iteration of the loop starts.
When the condition becomes false, the loop terminates which marks the end of its life cycle.
It is important to note that the do-while loop will execute its statements at least once before any condition is checked and therefore is an example of the exit control loop.
Example:
<!DOCTYPE html>
<html>
<body>
<script>
var i=21;
do{
document.write(i + "<br/>");
i++;
}while (i<=25);
</script>
</body>
</html>
Output:
21
22
23
24
25
compare the while and do…while loop
do while | while |
It is an exit-controlled loop | It is an entry-controlled loop. |
The number of iterations will be at least one irrespective of the condition | The number of iterations depends upon the condition specified |
The block code is controlled at the end | The block of code is controlled at starting |
4. JavaScript for-in Loop :
JS for-in loop is used to iterate over the properties of an object.
The for-in loop iterates only over those keys of an object which have their enumerable property set to “true”.
Syntax
for(let variable_name in object_name) {
// Statement
}
Whats Next ?
JS Object , Array , String , Function.
Subscribe to my newsletter
Read articles from Aditya Gadhave directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
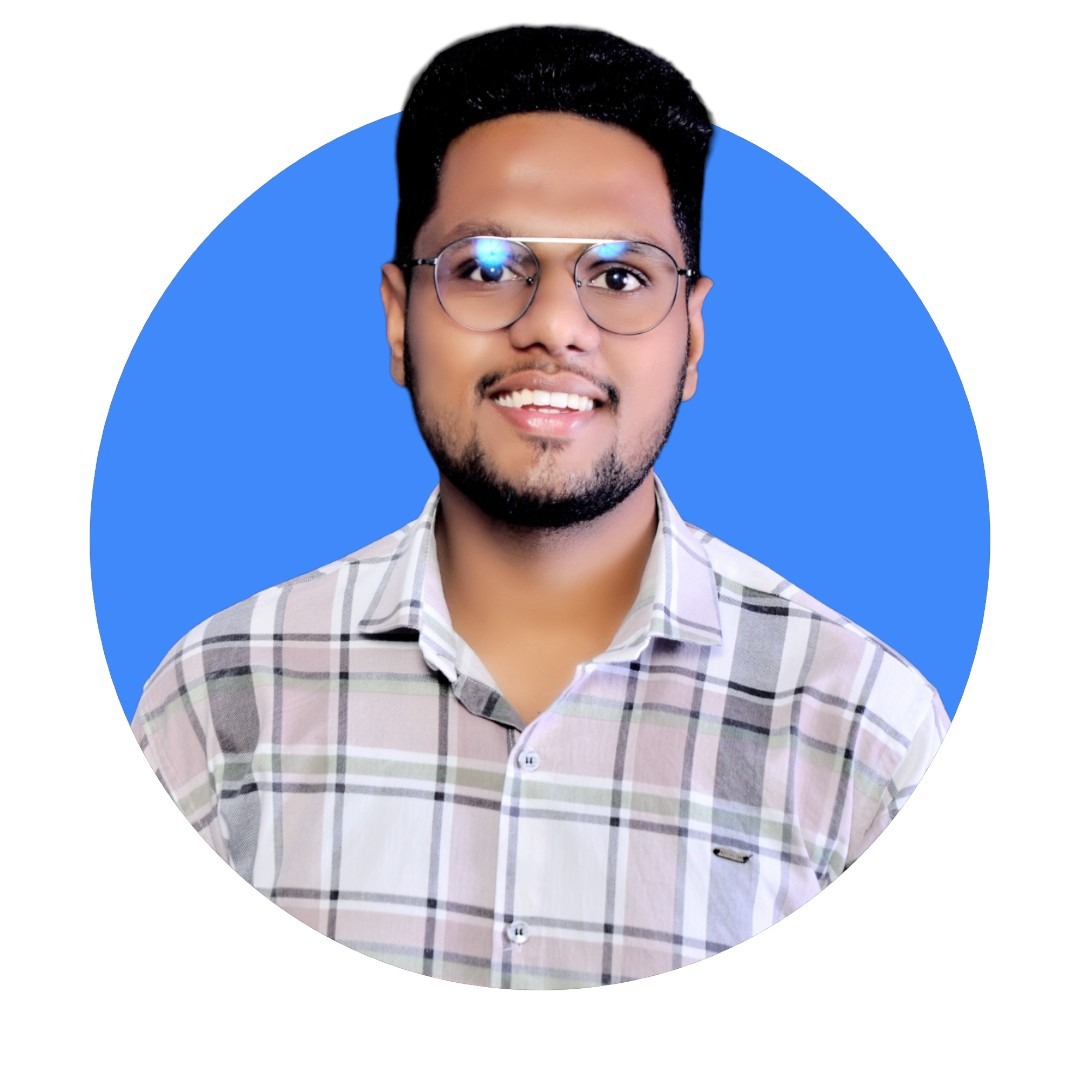
Aditya Gadhave
Aditya Gadhave
👋 Hello! I'm Aditya Gadhave, an enthusiastic Computer Engineering Undergraduate Student. My passion for technology has led me on an exciting journey where I'm honing my skills and making meaningful contributions.