Longest Palindrome
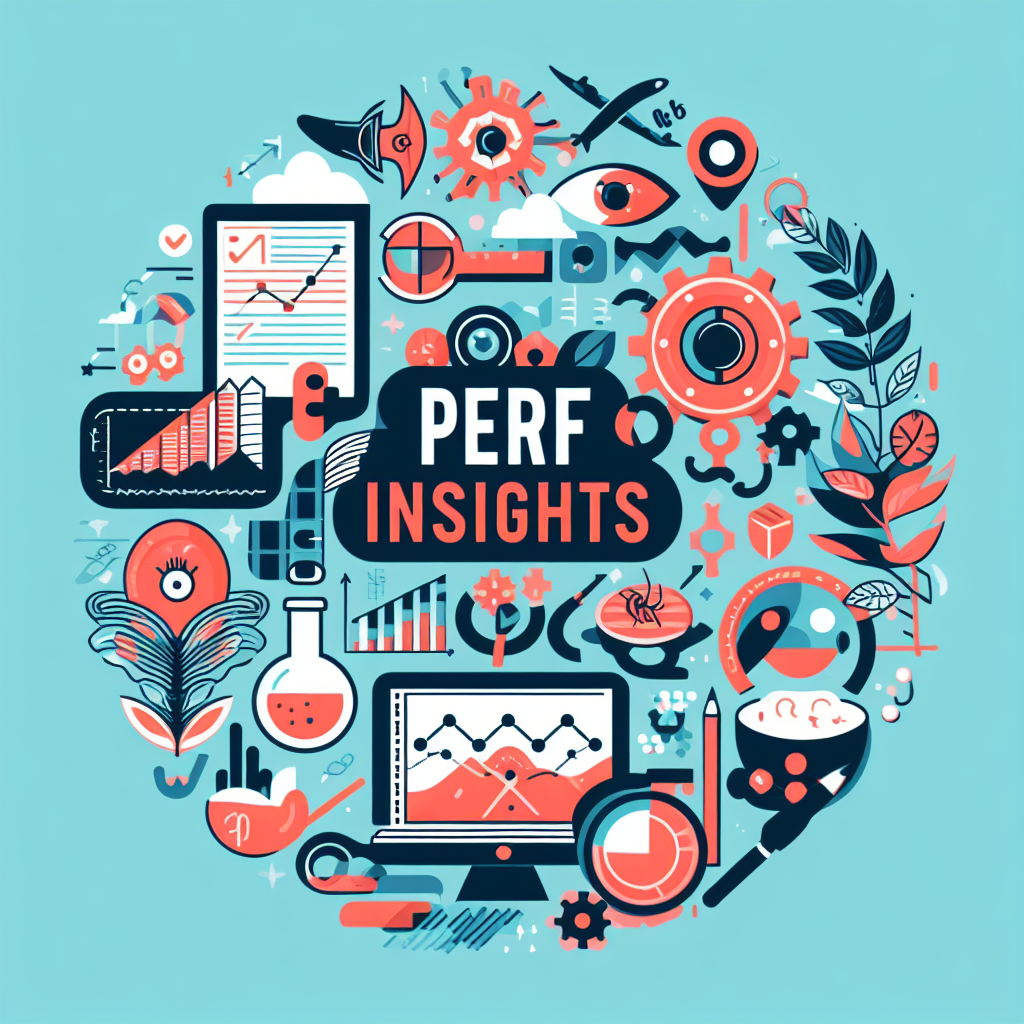
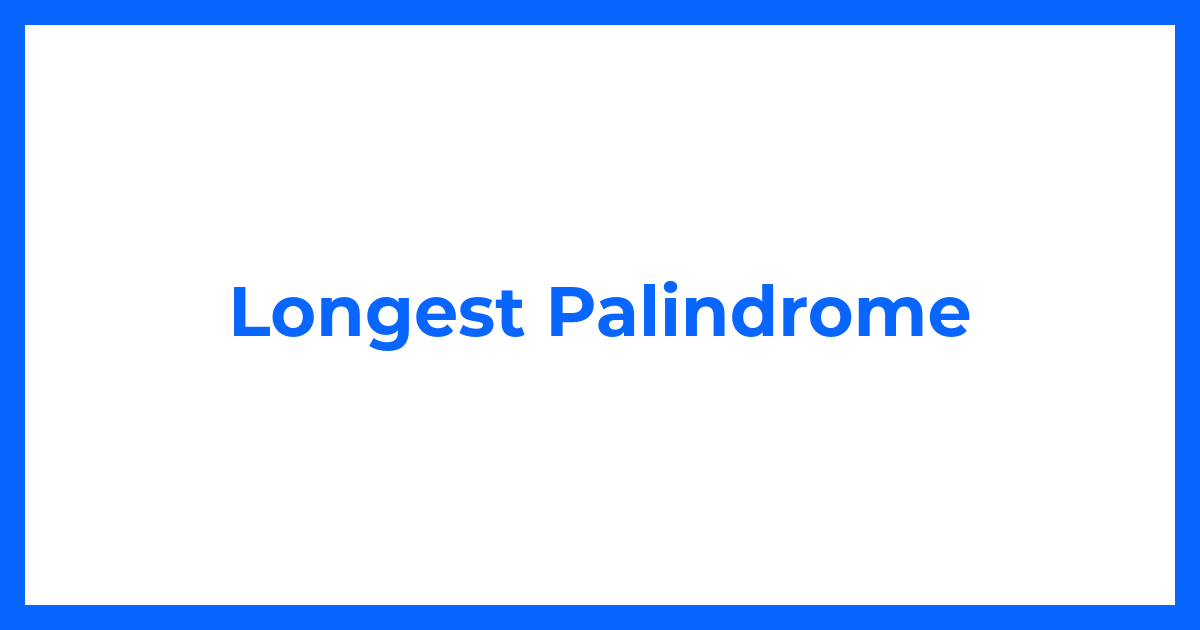
Given a string s
which consists of lowercase or uppercase letters, return the length of the longest palindrome that can be built with those letters.
Letters are case sensitive, for example, "Aa"
is not considered a palindrome.
class Solution {
public int longestPalindrome(String s) {
// Initialize a set to keep track of characters
Set<Character> charSet = new HashSet<>();
// Variable to keep track of the length of the palindrome
int palindromeLength = 0;
// Iterate over each character in the string
for(char c : s.toCharArray()) {
// If the character is already in the set, it means we have a pair
if(charSet.contains(c)) {
// Remove the character from the set and increase the palindrome length by 2
charSet.remove(c);
palindromeLength += 2;
} else {
// If the character is not in the set, add it to the set
charSet.add(c);
}
}
// If there are any characters left in the set, it means we can add one more character
// to the center of the palindrome (to make it odd-length)
if(!charSet.isEmpty()) palindromeLength += 1;
// Return the length of the longest palindrome that can be built
return palindromeLength;
}
}
Subscribe to my newsletter
Read articles from Gulshan Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
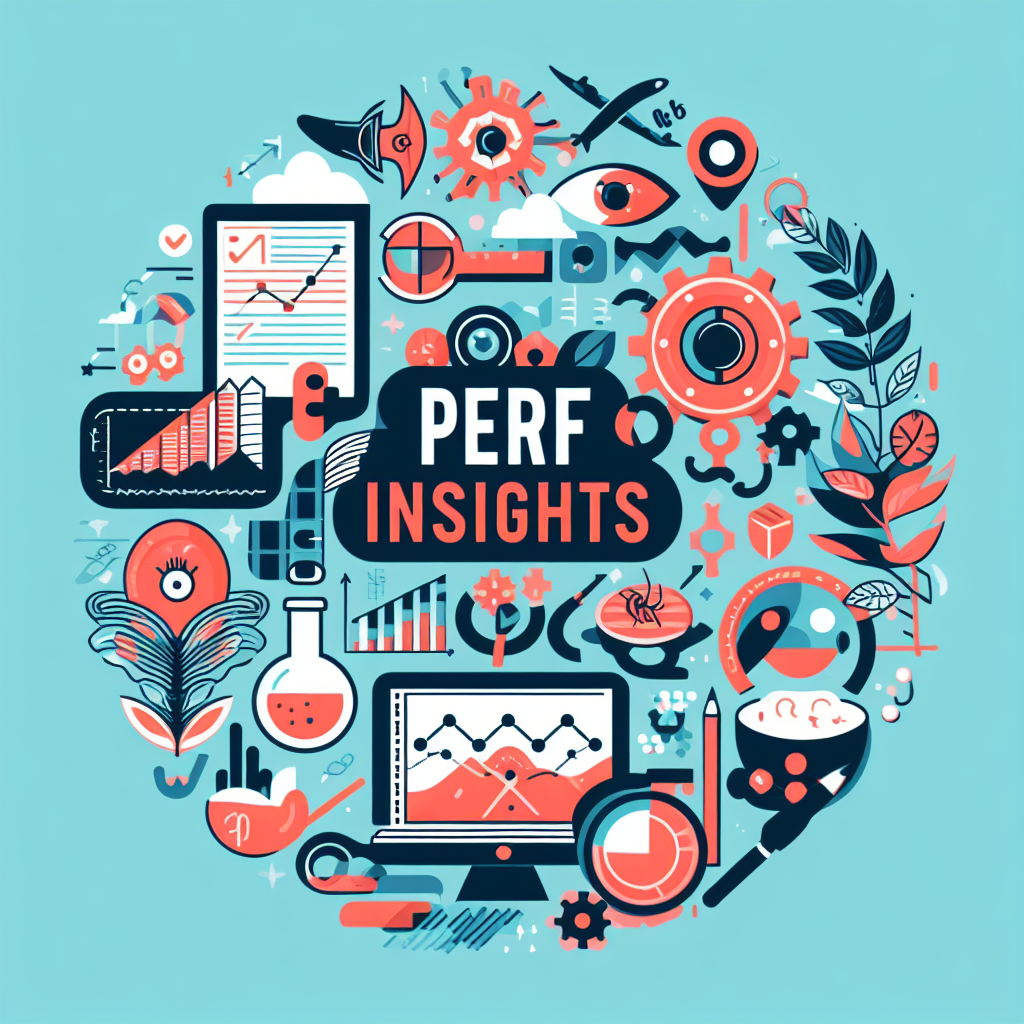
Gulshan Kumar
Gulshan Kumar
As a Systems Engineer at Tata Consultancy Services, I deliver exceptional software products for mobile and web platforms, using agile methodologies and robust quality maintenance. I am experienced in performance testing, automation testing, API testing, and manual testing, with various tools and technologies such as Jmeter, Azure LoadTest, Selenium, Java, OOPS, Maven, TestNG, and Postman. I have successfully developed and executed detailed test plans, test cases, and scripts for Android and web applications, ensuring high-quality standards and user satisfaction. I have also demonstrated my proficiency in manual REST API testing with Postman, as well as in end-to-end performance and automation testing using Jmeter and selenium with Java, TestNG and Maven. Additionally, I have utilized Azure DevOps for bug tracking and issue management.