Continuous Subarray Sum
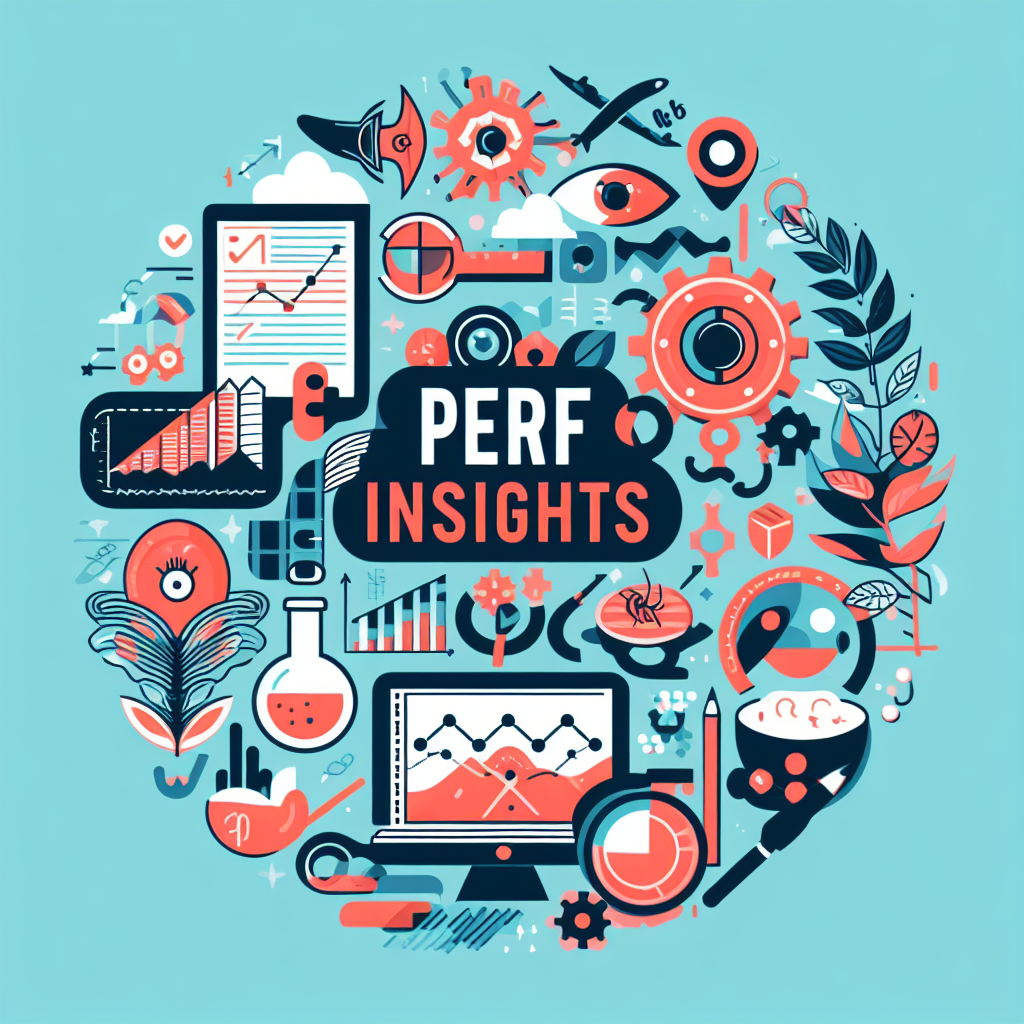
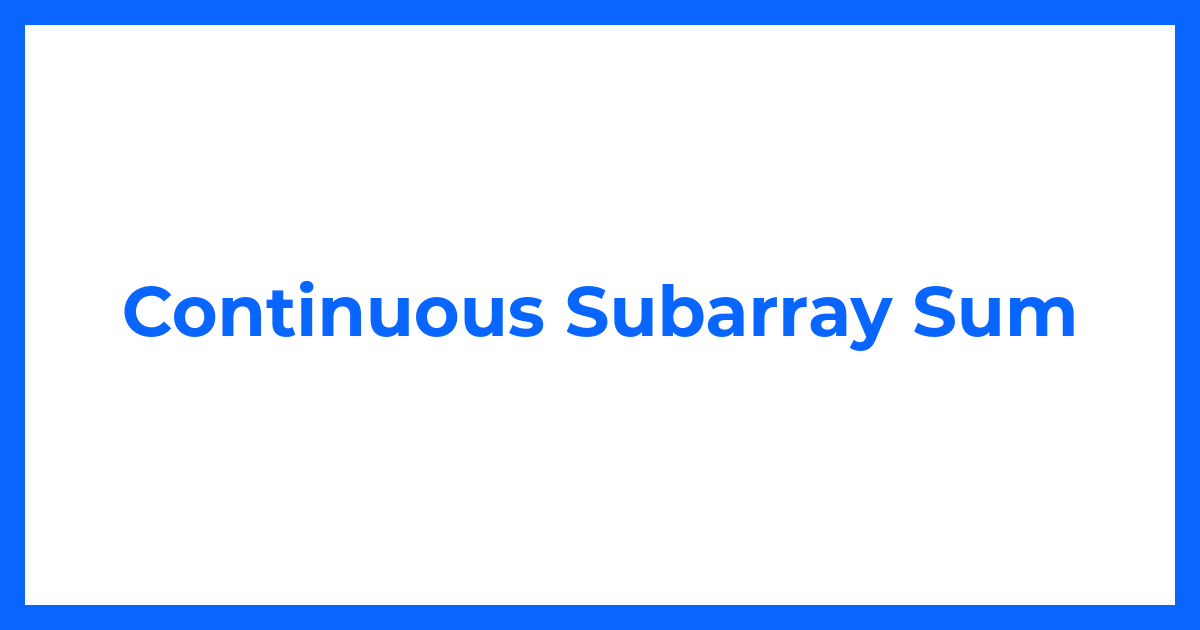
Given an integer array nums and an integer k, return true
if nums
has a good subarray or false
otherwise.
A good subarray is a subarray where:
its length is at least two, and
the sum of the elements of the subarray is a multiple of
k
.
Note that:
A subarray is a contiguous part of the array.
An integer
x
is a multiple ofk
if there exists an integern
such thatx = n * k
.0
is always a multiple ofk
.
LeetCode Problem - 523
class Solution {
public boolean checkSubarraySum(int[] nums, int k) {
// Initialize a map to store the remainders and their indices
Map<Integer, Integer> mp = new HashMap<>();
// Add a base case to handle the case where the subarray starts at the beginning
mp.put(0, -1);
// Initialize a variable to keep track of the cumulative sum
int sum = 0;
// Iterate over the array
for (int i = 0; i < nums.length; i++) {
// Update the cumulative sum
sum += nums[i];
// Calculate the remainder of the sum divided by k
int remainder = sum % k;
// If the remainder is found in the map, check the subarray length
if (mp.containsKey(remainder)) {
// If the subarray length is greater than 1, return true
if (i - mp.get(remainder) > 1) return true;
} else {
// If the remainder is not in the map, add it with the current index
mp.put(remainder, i);
}
}
// If no valid subarray is found, return false
return false;
}
}
Subscribe to my newsletter
Read articles from Gulshan Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
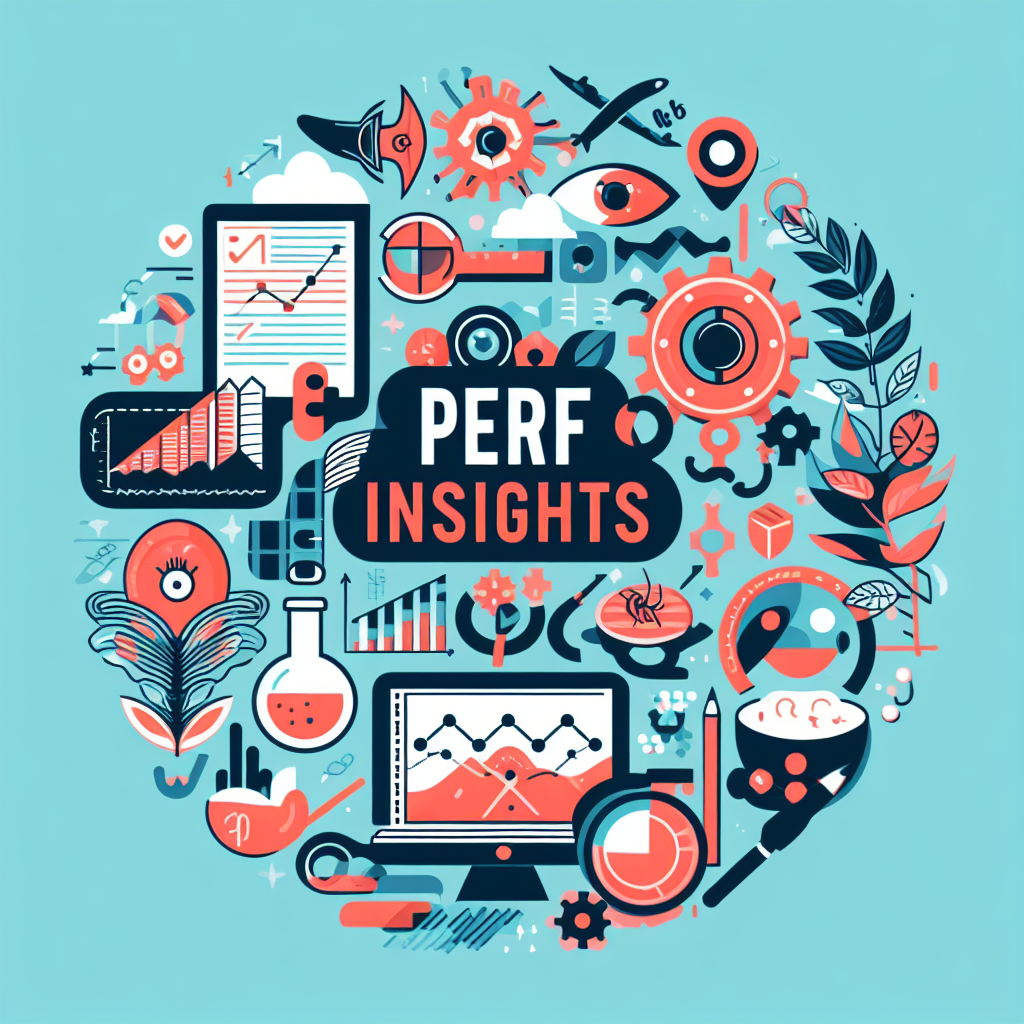
Gulshan Kumar
Gulshan Kumar
As a Systems Engineer at Tata Consultancy Services, I deliver exceptional software products for mobile and web platforms, using agile methodologies and robust quality maintenance. I am experienced in performance testing, automation testing, API testing, and manual testing, with various tools and technologies such as Jmeter, Azure LoadTest, Selenium, Java, OOPS, Maven, TestNG, and Postman. I have successfully developed and executed detailed test plans, test cases, and scripts for Android and web applications, ensuring high-quality standards and user satisfaction. I have also demonstrated my proficiency in manual REST API testing with Postman, as well as in end-to-end performance and automation testing using Jmeter and selenium with Java, TestNG and Maven. Additionally, I have utilized Azure DevOps for bug tracking and issue management.