Web 3 Connect Button: A Simple Building Tutorial
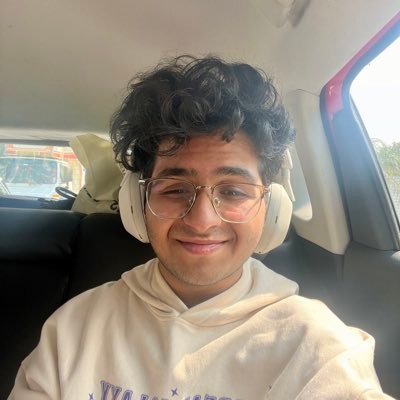
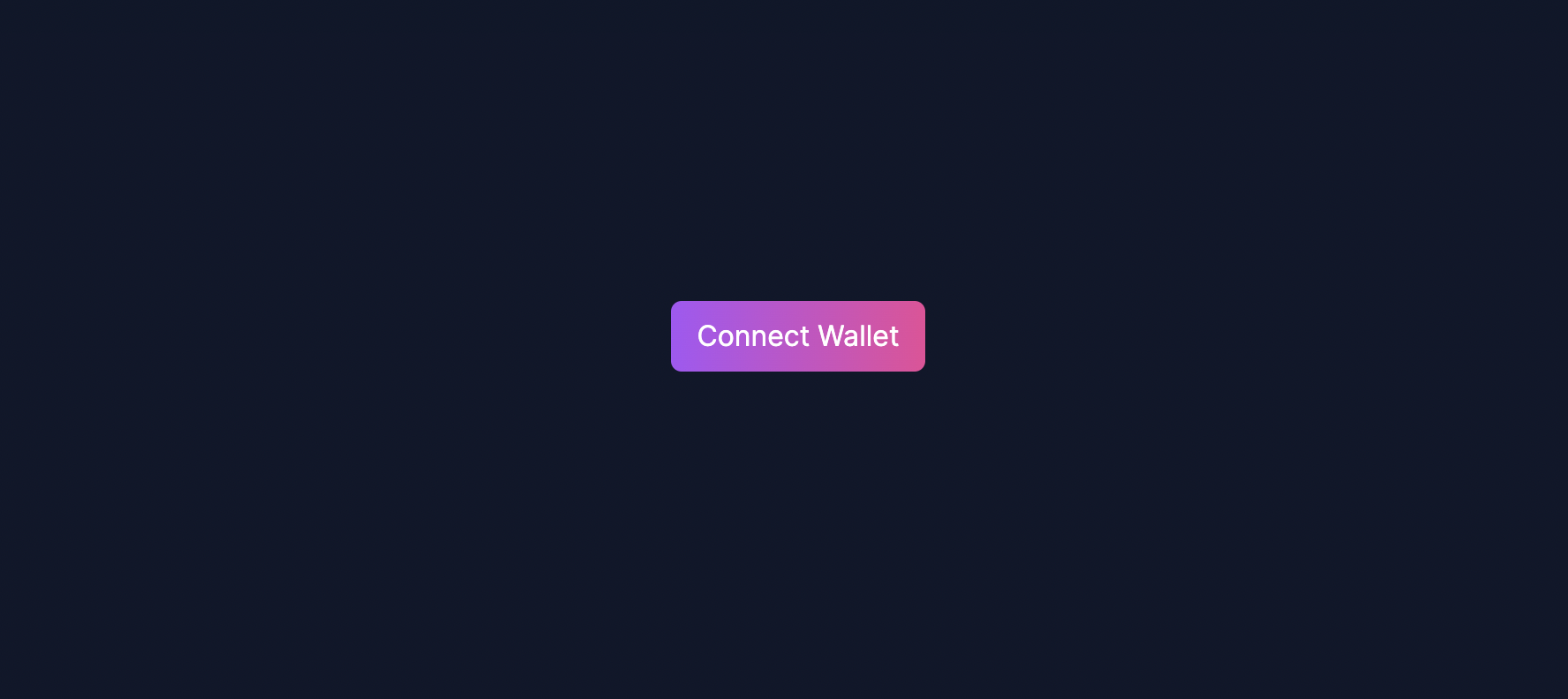
We will be building a web 3 wallet connect button, this blog is for beginners, who have just started to build exciting projects.
๐ Here's a Preview of what are we going to build
Initialise a NextJS Project
npx create-next-app web3Button
We would be using WalletConnect as our primary tool to connect users to our Web3 Wallet. It has a very compact and easy to use SDK with tons of extra features, which can further help us in our web3 projects.
๐ Here is the link to WalletConnect
๐ Simply create an account, and log on to your personal dashboard, once opened, create a new project. After that copy your project ID and keep it safe.
Open your NextJS and create a file context/web3modal.tsx
Paste the Project ID below that you copied before in 'YOUR_PROJECT_ID' .
'use client'
import { createWeb3Modal, defaultConfig } from '@web3modal/ethers/react'
const projectId = 'YOUR_PROJECT_ID'
// 2. Set chains
const mainnet = {
chainId: 1,
name: 'Ethereum',
currency: 'ETH',
explorerUrl: 'https://etherscan.io',
rpcUrl: 'https://cloudflare-eth.com'
}
// 3. Create a metadata object
const metadata = {
name: 'My Website',
description: 'My Website description',
url: 'https://mywebsite.com', // origin must match your domain & subdomain
icons: ['https://avatars.mywebsite.com/']
}
// 4. Create Ethers config
const ethersConfig = defaultConfig({
/*Required*/
metadata,
/*Optional*/
enableEIP6963: true, // true by default
enableInjected: true, // true by default
enableCoinbase: true, // true by default
rpcUrl: '...', // used for the Coinbase SDK
defaultChainId: 1 // used for the Coinbase SDK
})
// 5. Create a Web3Modal instance
createWeb3Modal({
ethersConfig,
chains: [mainnet],
projectId,
enableAnalytics: true, // Optional - defaults to your Cloud configuration
enableOnramp: true // Optional - false as default
})
export function Web3Modal({ children }) {
return children
}
Now we need to make some modifications in our layout.tsx
file.
import type { Metadata } from "next";
import { Inter } from "next/font/google";
import "./globals.css";
import { Web3Modal } from "@/context/web3modal";
const inter = Inter({ subsets: ["latin"] });
export const metadata: Metadata = {
title: "Web3 Modal",
description: "Generated by create next app",
};
export default function RootLayout({
children,
}: Readonly<{
children: React.ReactNode;
}>) {
return (
<html lang="en">
<body className={inter.className}>
<Web3Modal>{children}</Web3Modal>
</body>
</html>
);
}
Create a new file components/ConnectButton.tsx
"use client";
import React from "react";
import { useWeb3Modal, useWeb3ModalAccount } from "@web3modal/ethers/react";
import { truncateWalletAddress } from "@/utils/constants";
function ConnectButton() {
const { address, chainId, isConnected } = useWeb3ModalAccount();
const { open, close } = useWeb3Modal();
const connectWallet = () => {
open();
};
return (
<div
onClick={connectWallet}
className="bg-gradient-to-r from-purple-500 to-pink-500 p-2 w-36 rounded-md text-white text-center cursor-pointer hidden md:flex items-center justify-center"
>
<p className="text-center">
{isConnected ? truncateWalletAddress(address) : "Connect Wallet"}
</p>
</div>
);
}
export default ConnectButton;
There is a compact function that we built to shorten the user's address, I personally put this function to a constants file utils/constants.ts
export function truncateWalletAddress(
walletAddress: any,
startLength = 6,
endLength = 4
) {
const truncatedStart = walletAddress.slice(0, startLength);
const truncatedEnd = walletAddress.slice(-endLength);
return `${truncatedStart}...${truncatedEnd}`;
}
Here's how your main page.tsx
should look like.
import ConnectButton from "@/components/ConnectButton";
import Image from "next/image";
export default function Home() {
return (
<main className="flex min-h-screen flex-col items-center justify-center p-24">
<ConnectButton />
</main>
);
}
๐ And guess what we have a completely functioning Web3 Wallet
Subscribe to my newsletter
Read articles from Khushaal Choithramani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
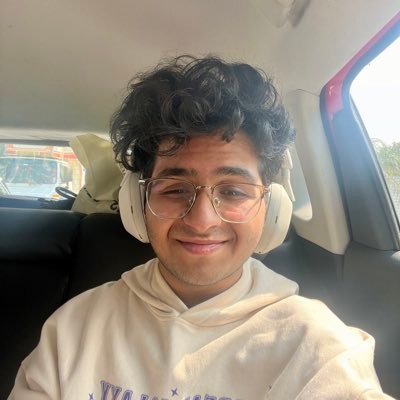