Generics and Traits in Rust: A Friendly Guide
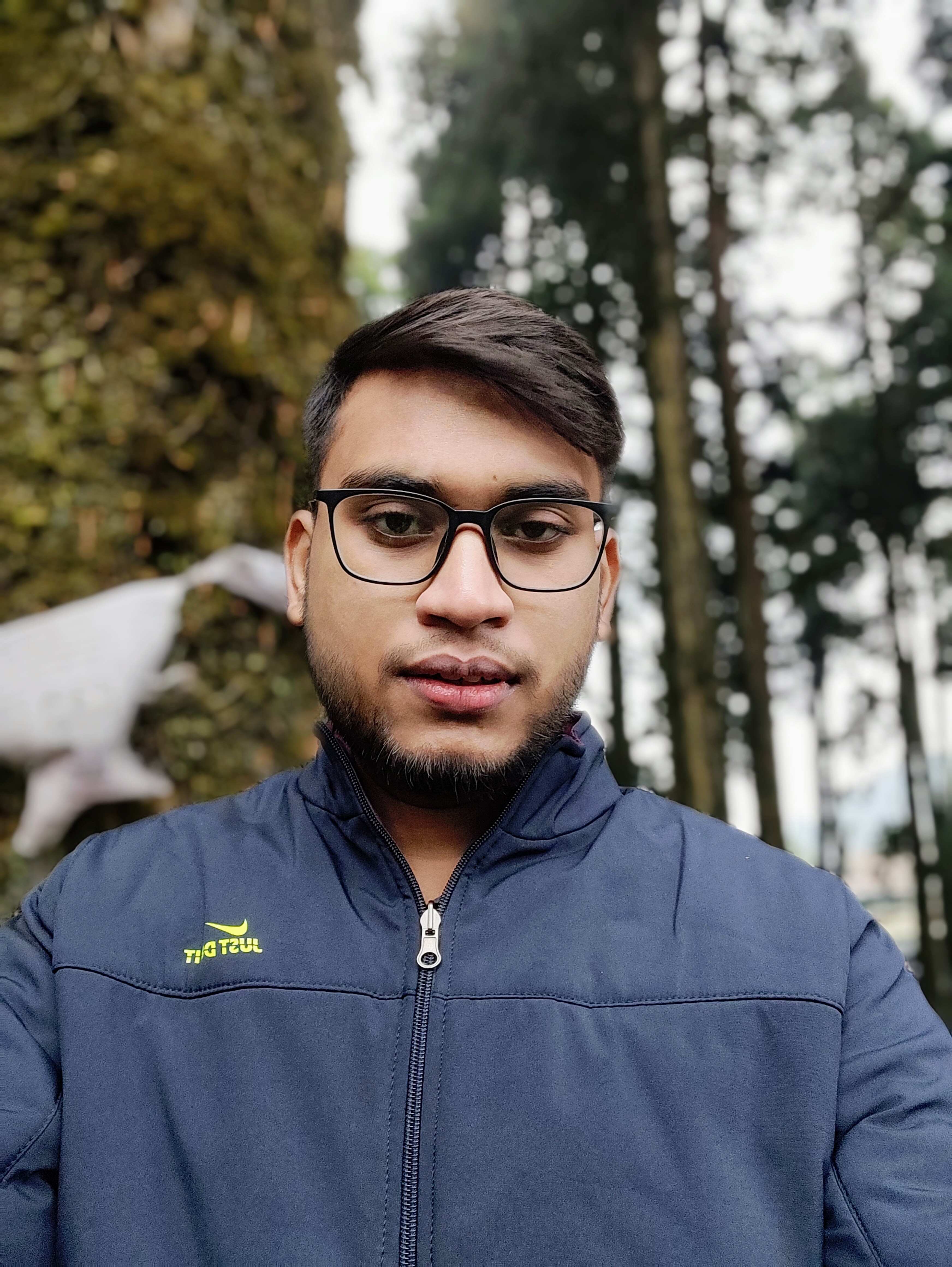
Table of contents
Hey there! Today, let's dive into some of Rust's more advanced concepts: Generic Types and Traits. These might sound a bit intimidating at first, but I'll walk you through each step with practical examples. By the end of this, you'll be able to use these features in your Rust projects confidently.
Follow me on Twitter @SoumitraSaha100, and connect on LinkedIn SOUMITRA SAHA
Explore the code on my Git Repository SOUMITRO-SAHA
Project Folder Structure
|-generics
| |-generic.rs
| |-mod.rs
|-traits
| |-mod.rs
| |-traits.rs
| |-trait_bounds_advance.rs
| |-trait_bounds_functions.rs
| |-trait_bounds_methods.rs
| |-trait_bounds_structs.rs
|-main.rs
Let's Break It Down:
Generics: Imagine a code toolbox where some tools can work on different materials. Generics are like those tools. They allow you to write code that can work with various types of data without needing a separate function for each type. Think of it as writing code that's like a chameleon, adapting to different situations!
Traits: These are blueprints that define what kind of behaviour a type should have. It's like having a set of instructions for building different kinds of furniture. A trait might say "This type needs to have a method called
do_something()
." Any type that follows those instructions (implements the trait) can be used with code that expects that behaviour.
Generics
Generics allow you to write flexible, reusable code for multiple types. Instead of specifying a concrete data type, you use a placeholder. This way, you can write functions, structs, enums, and more that can operate on various data types.
Let's create a new Rust project for our examples. Open your terminal and type:
cargo new generics_traits
cd generics_traits
Now, open src/
main.rs
and let's start with a simple example of generics.
Using Generics in Functions
Imagine you have a function that finds the largest number in a list. You'd write it like this:
fn largest<T: PartialOrd>(list: &[T]) -> &T {
let mut largest = &list[0];
for item in list {
if item > largest {
largest = item;
}
}
largest
}
fn main() {
let number_list = vec![34, 50, 25, 100, 65];
println!("The largest number is {}", largest(&number_list));
let char_list = vec!['y', 'm', 'a', 'q'];
println!("The largest char is {}", largest(&char_list));
}
In this code:
T
is a generic type parameter.T: PartialOrd
meansT
must implement thePartialOrd
trait, which allows comparison using the>
operator.
Using Generics in Structs
We can also define structs to use a generic type parameter in one or more fields using the <>
syntax. Let's see how this works.
struct Point<T> {
x: T,
y: T,
}
fn main() {
let integer = Point { x: 5, y: 10 };
let float = Point { x: 1.0, y: 4.0 };
}
Here, the Point<T>
struct holds x
and y
coordinate values of any type T
. This definition says that the Point<T>
struct is generic over some type T
, and both fields x
and y
are of that same type.
However, if we try to create a Point
instance with x
and y
of different types, the code won't compile.
struct Point<T> {
x: T,
y: T,
}
fn main() {
let wont_work = Point { x: 5, y: 4.0 };
}
To fix this, we can use multiple generic type parameters:
struct Point<T, U> {
x: T,
y: U,
}
fn main() {
let both_integer = Point { x: 5, y: 10 };
let both_float = Point { x: 1.0, y: 4.0 };
let integer_and_float = Point { x: 5, y: 4.0 };
}
Now, Point
can hold x
and y
values of different types.
Using Generics in Enums
Enums can also hold generic data types. Here's an example of the Option<T>
enum from the standard library:
enum Option<T> {
Some(T),
None,
}
This definition means Option<T>
is an enum that is generic over type T
and has two variants: Some
, which holds a value of type T
, and None
, which doesn't hold any value.
Another example is the Result<T, E>
enum:
enum Result<T, E> {
Ok(T),
Err(E),
}
The Result
enum is generic over two types, T
and E
. Ok
holds a value of type T
, and Err
holds a value of type E
. This makes it convenient to use the Result
enum for operations that might succeed or fail.
Using Generics in Methods
We can implement methods on structs and enums and use generic types in their definitions too. Here's the Point<T>
struct with a method named x
:
struct Point<T> {
x: T,
y: T,
}
impl<T> Point<T> {
fn x(&self) -> &T {
&self.x
}
}
fn main() {
let p = Point { x: 5, y: 10 };
println!("p.x = {}", p.x());
}
We can also implement methods for specific types. For instance:
impl Point<f32> {
fn distance_from_origin(&self) -> f32 {
(self.x.powi(2) + self.y.powi(2)).sqrt()
}
}
Here, distance_from_origin
is implemented only for Point<f32>
, not for other Point<T>
instances.
We can mix generic parameters in method signatures. For example, let's add a method mixup
to the Point<T, U>
struct:
struct Point<T, U> {
x: T,
y: U,
}
impl<T, U> Point<T, U> {
fn mixup<V, W>(self, other: Point<V, W>) -> Point<T, W> {
Point {
x: self.x,
y: other.y,
}
}
}
fn main() {
let p1 = Point { x: 5, y: 10.4 };
let p2 = Point { x: "Hello", y: 'c' };
let p3 = p1.mixup(p2);
println!("p3.x = {}, p3.y = {}", p3.x, p3.y);
}
Here, mixup
combines p1
and p2
into a new Point
instance p3
.
Traits
Traits are a way to define shared behaviour in Rust. You can think of them as interfaces in other languages. A trait tells the compiler about the functionality a type must provide.
Let's define a simple trait and implement it for a couple of types.
trait Summary {
fn summarize(&self) -> String;
}
struct Article {
headline: String,
content: String,
}
impl Summary for Article {
fn summarize(&self) -> String {
format!("{}: {}", self.headline, self.content)
}
}
struct Tweet {
username: String,
content: String,
}
impl Summary for Tweet {
fn summarize(&self) -> String {
format!("{}: {}", self.username, self.content)
}
}
fn main() {
let article = Article {
headline: String::from("Breaking News!"),
content: String::from("This is the content of the article."),
};
let tweet = Tweet {
username: String::from("user123"),
content: String::from("This is a tweet."),
};
println!("Article Summary: {}", article.summarize());
println!("Tweet Summary: {}", tweet.summarize());
}
In this example:
Summary
is a trait with asummarize
method.We implement
Summary
for bothArticle
andTweet
.
Trait Bounds
Trait bounds are a powerful feature in Rust that allows you to specify that a generic type must implement certain traits. This helps ensure that the types you work with provide the necessary behaviour defined by those traits.
What are Trait Bounds?
Trait bounds are constraints on generic types, specifying that a type must implement one or more traits. This way, you can guarantee that the type used in your generic code has the necessary methods and behaviours.
Using Trait Bounds in Functions
Let's start with a simple example where we use trait bounds in a function. Suppose we have a function that prints an item and it needs to display the item, which means the item must implement the Display
trait.
use std::fmt::Display;
fn print_item<T: Display>(item: T) {
println!("{}", item);
}
fn main() {
let number = 42;
let text = "Hello, world!";
print_item(number);
print_item(text);
}
In this example:
T: Display
specifies thatT
must implement theDisplay
trait.The
print_item
function can then use theDisplay
functionality.
Using Multiple Trait Bounds
You can specify multiple trait bounds using the +
syntax. This ensures that a generic type implements all the specified traits.
use std::fmt::Display;
use std::fmt::Debug;
fn print_debug_display<T: Display + Debug>(item: T) {
println!("Display: {}", item);
println!("Debug: {:?}", item);
}
fn main() {
let number = 42;
let text = "Hello, world!";
print_debug_display(number);
print_debug_display(text);
}
Here, T: Display + Debug
means that T
must implement both Display
and Debug
traits. The function print_debug_display
can now use methods from both traits.
Using Trait Bounds in Structs
Trait bounds can also be used in struct definitions to ensure that the types used in the struct implement certain traits.
use std::fmt::Display;
struct Pair<T: Display> {
x: T,
y: T,
}
impl<T: Display> Pair<T> {
fn new(x: T, y: T) -> Self {
Self { x, y }
}
fn display(&self) {
println!("x: {}, y: {}", self.x, self.y);
}
}
fn main() {
let pair = Pair::new(1, 2);
pair.display();
}
In this example:
Pair<T: Display>
ensures thatT
implements theDisplay
trait.The
display
method can then use theDisplay
functionality.
Using Trait Bounds in Methods
You can also use trait bounds in method implementations to constrain the types used within methods.
use std::fmt::Display;
struct Point<T> {
x: T,
y: T,
}
impl<T> Point<T> {
fn new(x: T, y: T) -> Self {
Self { x, y }
}
}
impl<T: Display> Point<T> {
fn display(&self) {
println!("x: {}, y: {}", self.x, self.y);
}
}
fn main() {
let point = Point::new(3, 4);
point.display();
}
Here:
- The
display
method is implemented only forPoint<T>
whereT
implementsDisplay
.
Advanced Trait Bounds: Where Clauses
When trait bounds become complex, you can use where
clauses to make your code more readable.
use std::fmt::Display;
fn print_pair<T, U>(pair: (T, U))
where
T: Display,
U: Display,
{
println!("First: {}, Second: {}", pair.0, pair.1);
}
fn main() {
let pair = (1, "hello");
print_pair(pair);
}
In this example:
The
where
clause is used to specify that bothT
andU
must implementDisplay
.This makes the function signature cleaner and easier to read.
Trait Bounds with Multiple Generics
You can also use trait bounds with multiple generic parameters, ensuring that each parameter meets certain constraints.
use std::fmt::Display;
struct Pair<T, U>
where
T: Display,
U: Display,
{
x: T,
y: U,
}
impl<T, U> Pair<T, U>
where
T: Display,
U: Display,
{
fn new(x: T, y: U) -> Self {
Self { x, y }
}
fn display(&self) {
println!("x: {}, y: {}", self.x, self.y);
}
}
fn main() {
let pair = Pair::new(1, "hello");
pair.display();
}
Here:
- The
Pair
struct and its methods use trait bounds to ensure that bothT
andU
implementDisplay
.
Most Common Doubts
Can I use multiple traits in a generic function?
Question: Can I specify multiple traits for a generic type in a function?
Answer: Yes, you can use the
+
syntax. For example,T: Display + Clone
meansT
must implement bothDisplay
andClone
traits.
What are the benefits of using traits?
Question: Why should I use traits in Rust?
Answer: Traits allow you to define shared behaviour in a clean, reusable way. They enable polymorphism and help you write more flexible and maintainable code.
Can generics cause performance overhead?
Question: Do generics cause performance overhead in Rust?
Answer: No, Rust's implementation of generics is zero-cost. The compiler generates specific implementations for each type, so there's no runtime overhead.
Subscribe to my newsletter
Read articles from SOUMITRA-SAHA directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
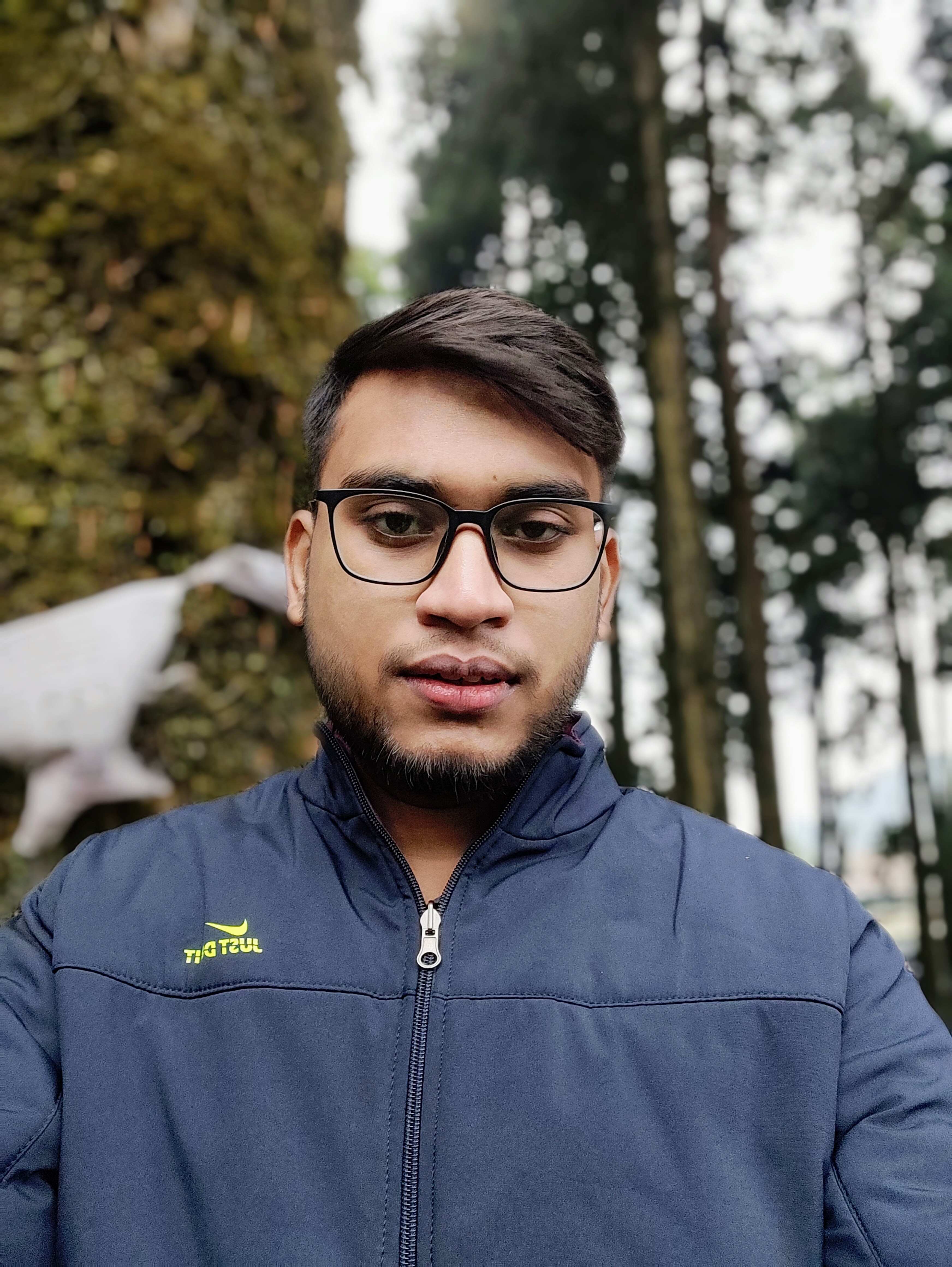
SOUMITRA-SAHA
SOUMITRA-SAHA
About Me I am a Full-Stack Developer with approximately two years of experience in the industry. My expertise spans both front-end and back-end development, where I have worked extensively to build and maintain dynamic, responsive web applications. Technical Skills Front-End Development: HTML, CSS, JavaScript, Typescript Frameworks: React, NextJs, Remix, Vite Libraries: TailwindCSS, Bootstrap Tools: Webpack, Babel Back-End Development: Languages: Typescript, Node.js, Python, Go, Rust Frameworks: NextJS, Remix, Express.js, Django, Go (Gin), Rust Databases: PostgreSQL, MySQL, MongoDB Tools: Docker, Kubernetes DevOps: Version Control: Git, GitHub CI/CD: Jenkins, CircleCI Infrastructure as Code: Terraform Cloud Services: AWS, Lamda Other Skills: RESTful API Development Test-Driven Development (TDD), (vitest, jest, Cypress) Agile Methodologies Personal Interests I am passionate about learning new technologies and keeping up with industry trends. I enjoy exploring innovative solutions to complex problems and continuously seek opportunities to expand my expertise. In my free time, I engage with the developer community, contributing to open-source projects and participating in tech meetups.