Parametrization - Inject Data From Excel
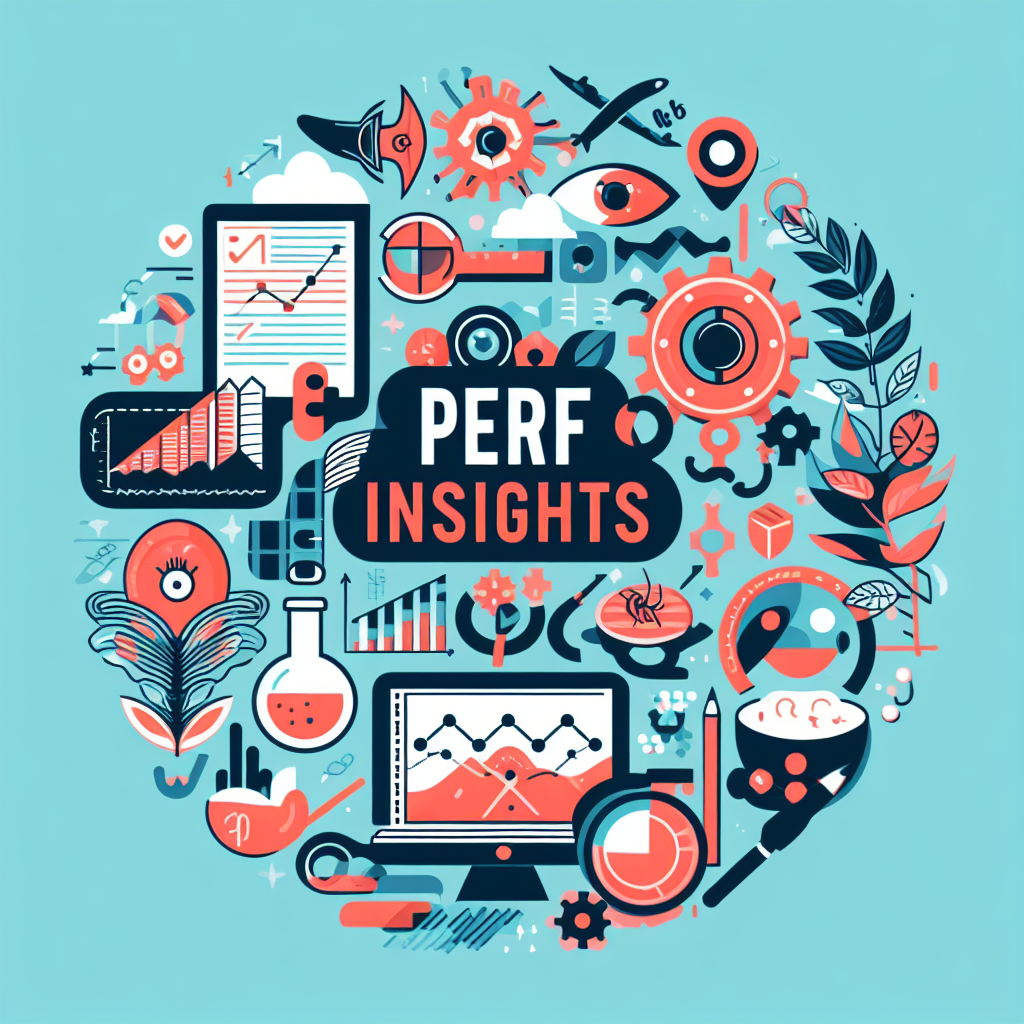
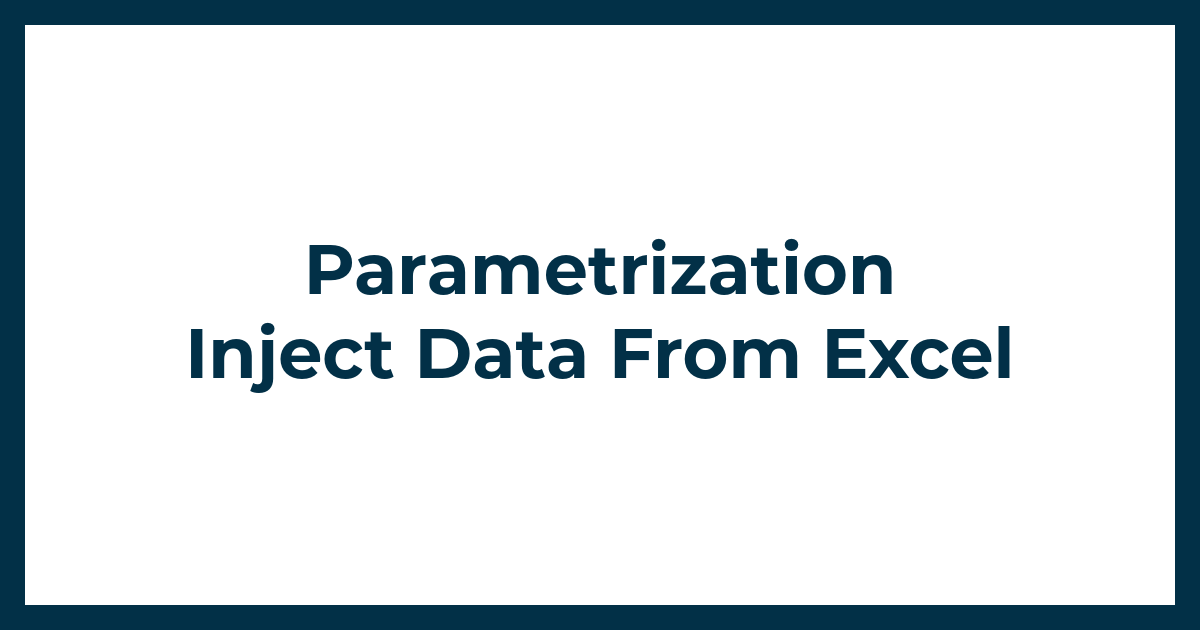
Introduction
Data driven testing is a smart way of testing the software, with the help of data driven testing we can make our code more re-usable and with the help of data driven testing we can run the same test with different data set. In this article I will try to explain why data driven testing is important and how to achieve it using selenium with Java.
For Example : If you want to test the login functionality of a E-Commerce website with different types of users, like admin regular users or with guest users then instead of writing separate test cases for each users, you can use data driven testing to achieve this by storing users information data into Excel file and then run the same test script for all users types, this makes our code more re- usable and easy to maintainable.
Advantages of Data Driven Testing
Comprehensive Coverage : Testing with multiple data set, ensures that all the edge scenarios are covered and the application is testing under different conditions and scenarios.
Validate data handling : Testing with different type of data including boundary values, valid or invalid data, ensures that application can handle all types of data correctly, validation of input and error handling.
Reliability : Running test with multiple data set increases the reliability of the application, It makes sure that test runs as expected with different data which ensures the robustness of the application.
Automation Efficiency : In automation testing, using multiple data sets in a single test case, maximize the efficiency of the test suite. You can inject multiple data set using @DataProvider and reduce the need of creating multiple test case for similar functionality.
Consistency and Accuracy : Multiple data set help in verifying the consistency and accuracy of the application's output. By comparing the output of the same test with different inputs testers can make sure that application works fine.
Running the Same Test with Multiple Sets of Data
Data Driven Testing : In selenium Data Driven Testing can be achieved by tools like TestNG or external libraries like Apache POI for Excel or JackSon for JSON to read the data from external files.
Parametrization : With the help of @DataProvider annotation parametrization can be achieved in selenium and this feature is provided by TestNG to run the same test with multiple data set by passing the values to methods as parameter.
Implementing Parameterization in Code
Below I have used Selenium with Java to achieve the Parameterization.
public class DataProviderDemo{
@Test(dataProvider = "loginCred")
public void loginTest(String userName , String pwd){
WebDriver driver = new FirefoxDriver();
driver.manage().window().maximize();
driver.get("https://www.saucedemo.com/");
driver.findElement(By.xpath("//input[@id='user-name']")).sendKeys(userName);
driver.findElement(By.xpath("//input[@id='password']")).sendKeys(pwd);
driver.findElement(By.xpath("//input[@id='login-button']")).click();
driver.quit();
}
@Test (dataProvider = "getExcelData")
public void loginTestWithExcelDAta(String userName , String pwd){
WebDriver driver = new FirefoxDriver();
driver.manage().window().maximize();
driver.get("https://www.saucedemo.com/");
driver.findElement(By.xpath("//input[@id='user-name']")).sendKeys(userName);
driver.findElement(By.xpath("//input[@id='password']")).sendKeys(pwd);
driver.findElement(By.xpath("//input[@id='login-button']")).click();
driver.quit();
}
@DataProvider (name="loginCred")
public String[][] dataset1(){
String[][] arr = { {"standard_user", "secret_sauce"},
{"error_user", "secret_sauce"}};
return arr;
}
@DataProvider(name = "getExcelData")
public String[][] getXLData() throws Exception {
File file = new File("C:\\Users\\aglsh\\Downloads\\Parametrization.xlsx");
FileInputStream fis = new FileInputStream(file);
XSSFWorkbook workbook = new XSSFWorkbook(fis);
XSSFSheet sheet = workbook.getSheetAt(0);
int numberOfRows = sheet.getPhysicalNumberOfRows();
String[][] credentials = new String[numberOfRows][2];
int rowIdx = 0;
for (int i=1; i<numberOfRows; i++){
if (sheet.getRow(i).getCell(0) != null && sheet.getRow(i).getCell(1)!=null){
String userName = sheet.getRow(i).getCell(0).getStringCellValue();
String pwd = sheet.getRow(i).getCell(1).getStringCellValue();
credentials[rowIdx][0] = userName; credentials[rowIdx][1] = pwd;
rowIdx++;
}
}
workbook.close();
return credentials;
}// Excel
}
Explanation of the Code
Login Test Method: This method logs into a website using provided username and password combinations.
@Test(dataProvider = "loginCred") public void loginTest(String userName , String pwd){ WebDriver driver = new FirefoxDriver(); driver.manage().window().maximize(); driver.get("https://www.saucedemo.com/"); // Entering username and password driver.findElement(By.xpath("//input[@id='user-name']")).sendKeys(userName); driver.findElement(By.xpath("//input[@id='password']")).sendKeys(pwd); // Clicking on login button driver.findElement(By.xpath("//input[@id='login-button']")).click(); // Quitting the browser driver.quit(); }
Login Test Method with Excel Data : This method also logs into a website using username and password combinations retrieved from an Excel file.
@Test(dataProvider = "getExcelData") public void loginTestWithExcelData(String userName , String pwd){ WebDriver driver = new FirefoxDriver(); driver.manage().window().maximize(); driver.get("https://www.saucedemo.com/"); // Entering username and password from Excel driver.findElement(By.xpath("//input[@id='user-name']")).sendKeys(userName); driver.findElement(By.xpath("//input[@id='password']")).sendKeys(pwd); // Clicking on login button driver.findElement(By.xpath("//input[@id='login-button']")).click(); // Quitting the browser driver.quit(); }
DataProvider for loginCred : This
@DataProvider
method provides login credentials as a 2D array directly in the code.@DataProvider(name="loginCred") public String[][] dataset1(){ String[][] arr = {{"standard_user", "secret_sauce"}, {"error_user", "secret_sauce"}}; return arr; }
DataProvider for getExcelData : This
@DataProvider
method reads login credentials from an Excel file and provides them as a 2D array.@DataProvider(name = "getExcelData") public String[][] getExcelData() throws Exception { File file = new File("C:\\Users\\aglsh\\Downloads\\Parametrization.xlsx"); FileInputStream fis = new FileInputStream(file); XSSFWorkbook workbook = new XSSFWorkbook(fis); XSSFSheet sheet = workbook.getSheetAt(0); int numberOfRows = sheet.getPhysicalNumberOfRows(); String[][] credentials = new String[numberOfRows][2]; int rowIdx = 0; for (int i=1; i<numberOfRows; i++){ if (sheet.getRow(i).getCell(0) != null && sheet.getRow(i).getCell(1)!=null){ String userName = sheet.getRow(i).getCell(0).getStringCellValue(); String pwd = sheet.getRow(i).getCell(1).getStringCellValue(); credentials[rowIdx][0] = userName; credentials[rowIdx][1] = pwd; rowIdx++; } } workbook.close(); return credentials; }
Conclusion
Data-driven testing is a valuable approach in software testing, offering multiple benefits such as comprehensive coverage, validation of data handling, reliability, automation efficiency, consistency, and accuracy. By using tools like TestNG and libraries like Apache POI for Excel, testers can implement parameterization easily in Selenium with Java, which allows for the efficient execution of tests with multiple datasets. This approach not only enhances code re-usability and maintainability but also ensures robustness in application performance.
Subscribe to my newsletter
Read articles from Gulshan Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
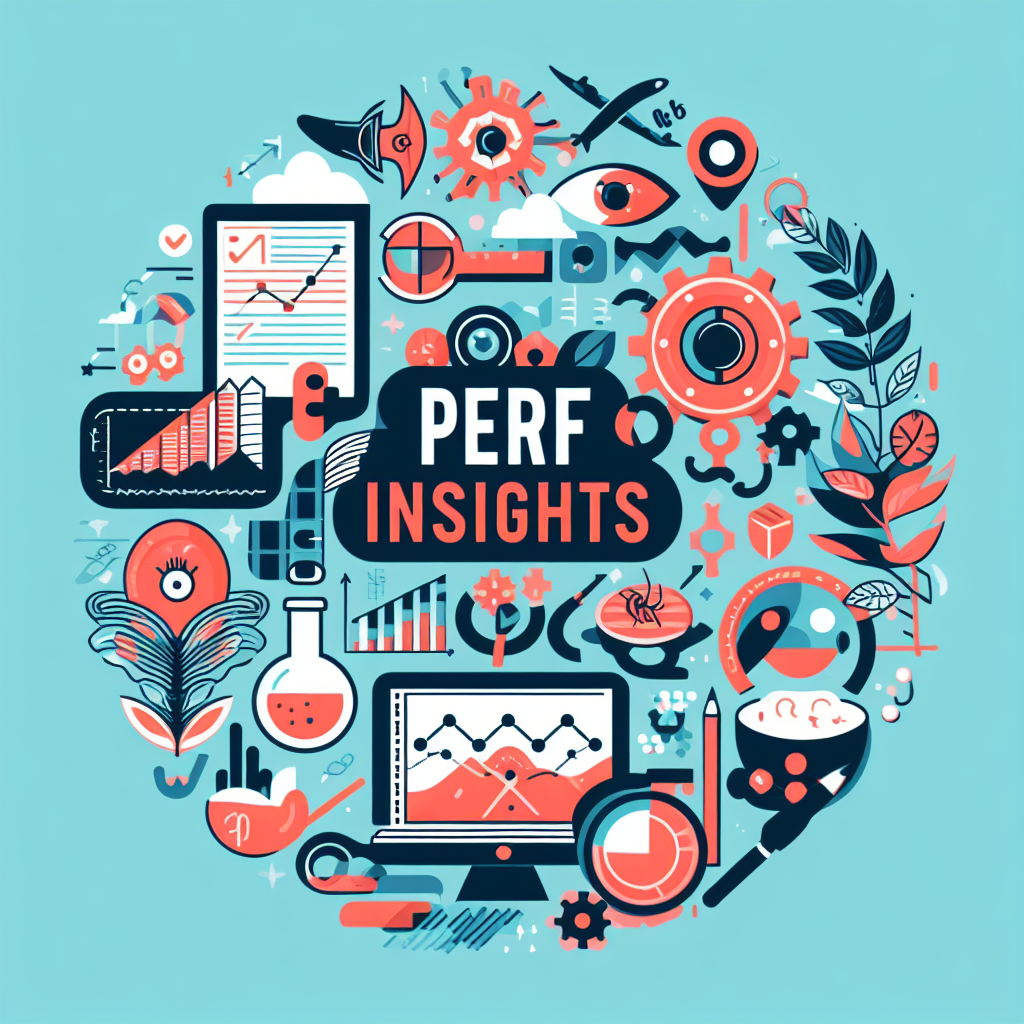
Gulshan Kumar
Gulshan Kumar
As a Systems Engineer at Tata Consultancy Services, I deliver exceptional software products for mobile and web platforms, using agile methodologies and robust quality maintenance. I am experienced in performance testing, automation testing, API testing, and manual testing, with various tools and technologies such as Jmeter, Azure LoadTest, Selenium, Java, OOPS, Maven, TestNG, and Postman. I have successfully developed and executed detailed test plans, test cases, and scripts for Android and web applications, ensuring high-quality standards and user satisfaction. I have also demonstrated my proficiency in manual REST API testing with Postman, as well as in end-to-end performance and automation testing using Jmeter and selenium with Java, TestNG and Maven. Additionally, I have utilized Azure DevOps for bug tracking and issue management.