Dynamic Routing in Next.js: Creating Flexible and Scalable Apps
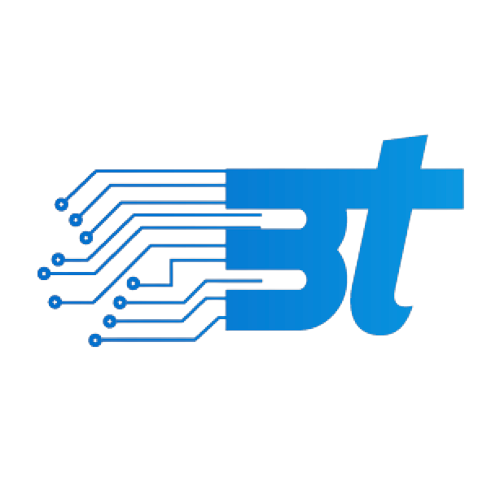
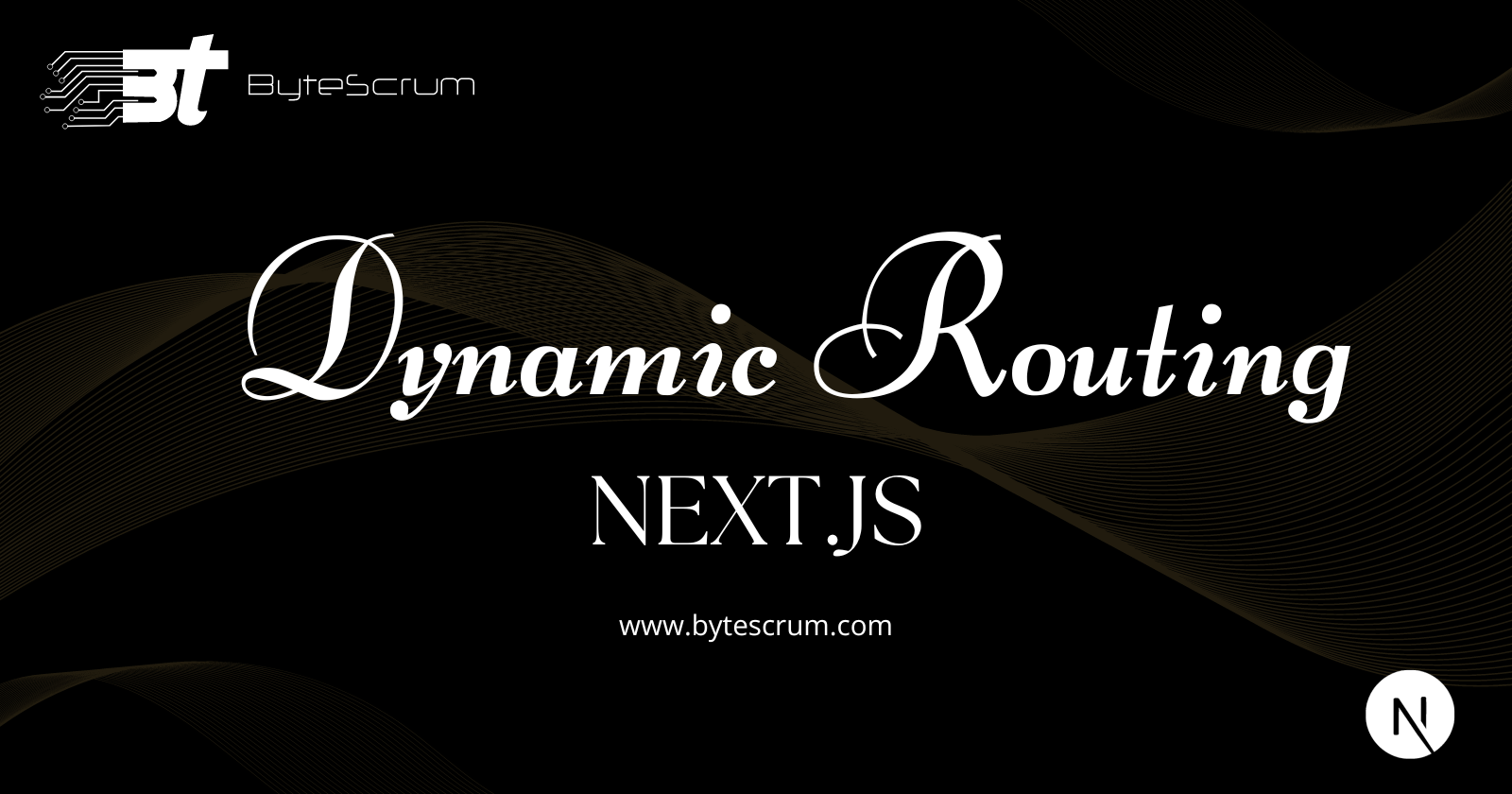
Dynamic routing is a powerful feature in Next.js that allows you to create flexible and scalable applications. It enables you to handle complex URL structures, making it easier to build applications with dynamic content, such as blogs, e-commerce sites, and more. In this guide, we’ll explore how to implement dynamic routing in Next.js and create a flexible and scalable app.
What is Dynamic Routing ?
Dynamic routing in Next.js allows you to create routes that can change based on the parameters passed in the URL. This is useful for creating pages that need to display different content based on the input, such as user profiles, product details, or blog posts.
Setting Up Dynamic Routes
Step 1: Create a Dynamic Route
In Next.js, you can create a dynamic route by using square brackets ([ ]
) in the file name within the pages
directory.
Example: To create a route for user profiles, create a file named [id].js
in the pages/users
directory.
/pages
/users
[id].js
Step 2: Access Route Parameters
Next.js uses the useRouter
hook to access the route parameters. You can use this hook inside your dynamic route component to get the parameter value.
Example:
// pages/users/[id].js
import { useRouter } from 'next/router';
const UserProfile = () => {
const router = useRouter();
const { id } = router.query;
return (
<div>
<h1>User Profile</h1>
<p>User ID: {id}</p>
</div>
);
};
export default UserProfile;
Step 3: Fetch Data Based on Route Parameters
You often need to fetch data based on the route parameters. Next.js provides getStaticProps
and getServerSideProps
to fetch data at build time or request time.
Example with getStaticProps
and getStaticPaths
:
// pages/users/[id].js
import { useRouter } from 'next/router';
const UserProfile = ({ user }) => {
const router = useRouter();
// If the page is not yet generated, this will be displayed initially until getStaticProps completes.
if (router.isFallback) {
return <div>Loading...</div>;
}
return (
<div>
<h1>User Profile</h1>
<p>User ID: {user.id}</p>
<p>Name: {user.name}</p>
</div>
);
};
export async function getStaticPaths() {
// Fetch all user IDs from an API or database
const res = await fetch('https://api.example.com/users');
const users = await res.json();
// Generate paths for each user ID
const paths = users.map((user) => ({
params: { id: user.id.toString() },
}));
return { paths, fallback: true };
}
export async function getStaticProps({ params }) {
// Fetch user data based on the ID
const res = await fetch(`https://api.example.com/users/${params.id}`);
const user = await res.json();
return {
props: { user },
};
}
export default UserProfile;
Handling Nested Routes
You can also create nested dynamic routes by using multiple levels of square brackets.
Example: To create a nested route for user posts:
/pages
/users
[id]
posts
[postId].js
Access both id
and postId
in your component:
// pages/users/[id]/posts/[postId].js
import { useRouter } from 'next/router';
const UserPost = () => {
const router = useRouter();
const { id, postId } = router.query;
return (
<div>
<h1>User Post</h1>
<p>User ID: {id}</p>
<p>Post ID: {postId}</p>
</div>
);
};
export default UserPost;
Dynamic API Routes
Next.js also supports dynamic API routes, which are useful for creating API endpoints that need to handle dynamic parameters.
Example: Create a dynamic API route:
/pages
/api
/users
[id].js
Access the dynamic parameter in the API route:
// pages/api/users/[id].js
export default function handler(req, res) {
const { id } = req.query;
res.status(200).json({ userId: id });
}
Conclusion
Explore the official Next.js documentation for more details and advanced configurations. Happy coding!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
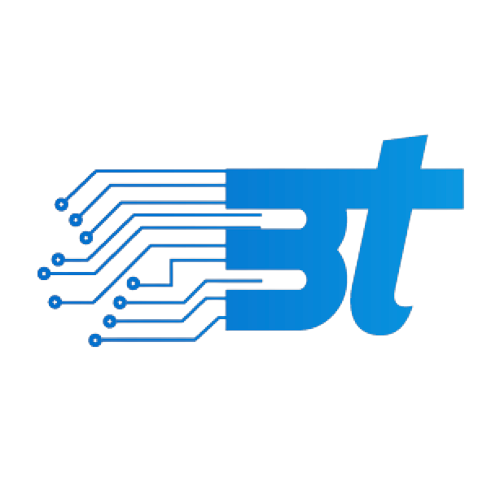
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.