π Step-by-Step Guide to Building an Employee Management System with Spring Boot
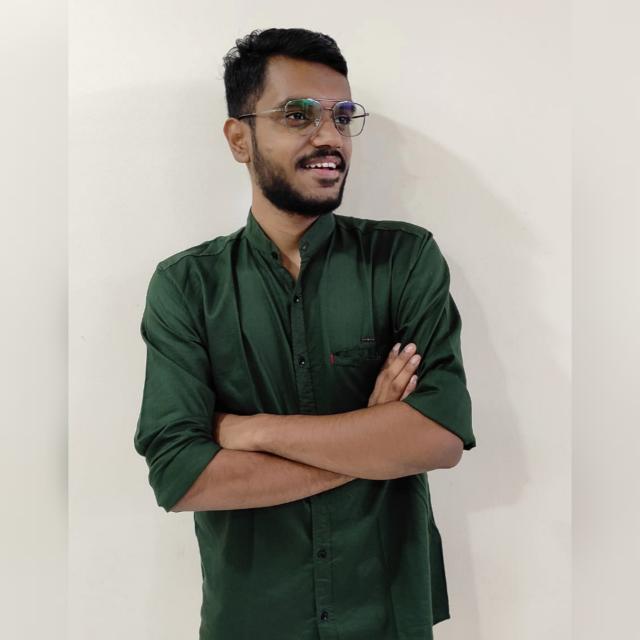
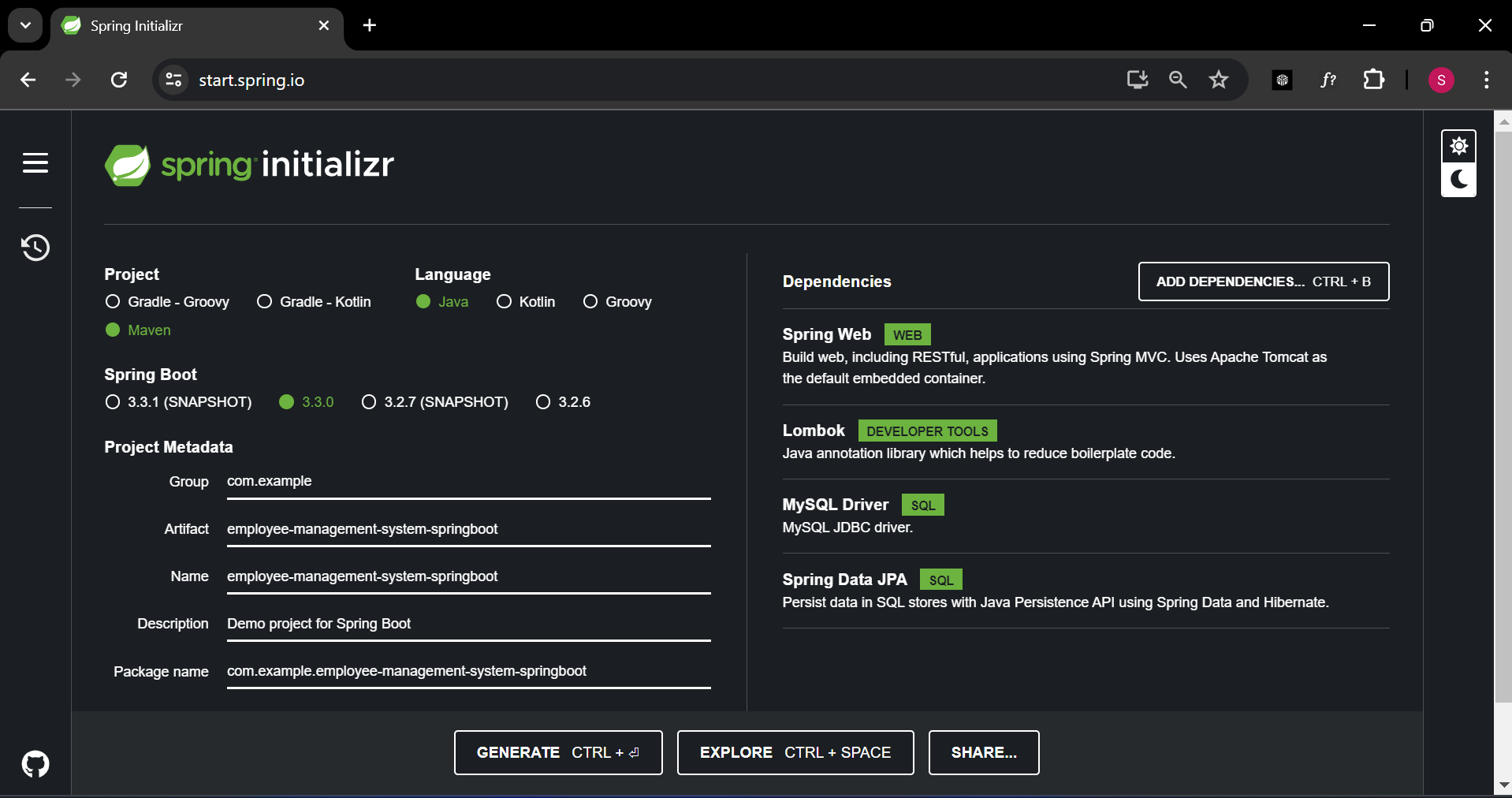
Hello, developers! π
Today, I'm excited to share with you a project I've been working on - an Employee Management System built using Spring Boot, REST API, and MySQL. This project allows you to manage employees and departments efficiently with CRUD operations and showcases @ManyToOne relationships. Let's dive in! π»
π Overview
The Employee Management System is a Spring Boot REST API application designed to manage employees and departments within an organization. It supports CRUD (Create, Read, Update, Delete) operations for both employees and departments and demonstrates the use of @ManyToOne mapping between employees and departments.
β¨ Features
Employee Management:
Add new employees
Retrieve all employees or by ID
Update employee details
Delete employees by ID
Department Management:
Add new departments
Retrieve all departments or by ID
Update department details
Delete departments by ID
Relationships:
- Demonstrates @ManyToOne mapping between employees and departments, showing how an employee can belong to one department.
π₯ Features of Spring Boot
Auto-configuration: Automatically configures your Spring application based on the jar dependencies you have added.
Standalone: Spring Boot applications can be run independently using the embedded server.
Production-ready: Comes with various production-ready features like metrics, health checks, and externalized configuration.
Opinionated defaults: Provides default configurations to get started quickly.
ποΈ Spring Boot Architecture
Spring Boot follows a layered architecture where each layer communicates with the layers directly below or above it. Hereβs a quick overview:
Presentation Layer: Handles HTTP requests, translates them into model calls, and returns the views.
Business Layer: Implements the business logic, handles the business rules, and processes data.
Persistence Layer: Deals with the database operations like CRUD and database connectivity.
Database Layer: The actual database where data is stored.
π οΈ Technologies Used
Java: The primary programming language used.
Spring Boot: Simplifies the setup and development of the application.
Spring Data JPA: Provides an abstraction layer for working with JPA entities.
Hibernate: The ORM (Object-Relational Mapping) framework used.
MySQL: The relational database management system.
RESTful API: For creating and consuming APIs in a stateless manner.
π Project Structure
Here's a quick overview of the project structure:
src/main/java/com/EmpManagement βββ Controller β βββ EmployeeController.java β βββ DepartmentController.java βββ Entity β βββ Employee.java β βββ Department.java βββ Repository β βββ EmployeeRepository.java β βββ DepartmentRepository.java βββ Service β βββ EmployeeService.java β βββ DepartmentService.java βββ EmpManagementApplication.java
π οΈ Step-by-Step Guide
1. Create Project Boilerplate and Add Dependencies
Go toSpring Initializr.
SelectMaven Project and Java language.
Give a Group (package) and Artifact (application) name to your project.
Add dependencies for Spring Web, MySQLDriver, Lombok, and Spring Data JPA.
Click on Generate.
The project zip file will get downloaded. Now, unzip your folder and open it in your favorite IDE.
2. Create the MySQL Database
Open MySQL Command Line Client and create a new database named
employee_db
using the following SQL query:CREATE DATABASE employee_management;
3. Configuring the Database into Your Project
Open the unzipped project in your favorite IDE (e.g., IntelliJ IDEA) and add database configuration to the
application.properties
file:spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver spring.datasource.url=jdbc:mysql://localhost:3307/employee_db spring.datasource.username=root spring.datasource.password=root1234 spring.jpa.show-sql=true spring.jpa.hibernate.ddl-auto=update spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQL8Dialect
4. Creating the Employee Entity
Create a new class
Employee.java
in thecom.EmpManagement.Entity
package:package com.EmpManagement.Entity; import jakarta.persistence.Column; import jakarta.persistence.Entity; import jakarta.persistence.GeneratedValue; import jakarta.persistence.GenerationType; import jakarta.persistence.Id; import jakarta.persistence.JoinColumn; import jakarta.persistence.ManyToOne; import jakarta.persistence.Table; import lombok.AllArgsConstructor; import lombok.Data; import lombok.NoArgsConstructor; @Entity @Data @AllArgsConstructor @NoArgsConstructor @Table(name = "employee") public class Employee { @Id @GeneratedValue(strategy = GenerationType.AUTO) @Column(name = "employee_id") private int employee_id; @Column(name = "employee_name") private String employee_name; @Column(name = "employee_salary") private int salary; @ManyToOne @JoinColumn(name = "department_id") private Department department; }
5. Creating the Department Entity
Create a new class
Department.java
in thecom.EmpManagement.Entity
package:
package com.EmpManagement.Entity;
import jakarta.persistence.Column;
import jakarta.persistence.Entity;
import jakarta.persistence.GeneratedValue;
import jakarta.persistence.GenerationType;
import jakarta.persistence.Id;
import jakarta.persistence.Table;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
@Entity
@Data
@AllArgsConstructor
@NoArgsConstructor
@Table(name = "department")
public class Department {
@Id
@Column(name = "department_id")
@GeneratedValue(strategy = GenerationType.AUTO)
private int department_id;
@Column(name = "short_name")
private String short_name;
@Column(name = "department_name")
private String department_name;
}
6. Creating the Repositories
Create two interfaces EmployeeRepository.java
and DepartmentRepository.java
in the com.EmpManagement.Repository
package:
package com.EmpManagement.Repository;
import com.EmpManagement.Entity.Employee;
import org.springframework.data.jpa.repository.JpaRepository;
public interface EmployeeRepository extends JpaRepository<Employee, Integer> {
}
DepartmentRepository.java
package com.EmpManagement.Repository;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
import com.EmpManagement.Entity.Department;
@Repository
public interface DepartmentRepository extends JpaRepository<Department, Integer>{
}
7. Creating the Services
Create two classes EmployeeService.java
and DepartmentService.java
in the com.EmpManagement.Service
package:
EmployeeService.java
package com.EmpManagement.Service;
import com.EmpManagement.Entity.Employee;
import com.EmpManagement.Repository.EmployeeRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
@Service
public class EmployeeService {
@Autowired
private EmployeeRepository employeeRepository;
// Add Employee
public void addEmployee(Employee emp) {
employeeRepository.save(emp);
}
// Get all Employees
public List<Employee> getAllEmployees() {
List<Employee> employees = employeeRepository.findAll();
return employees;
}
// Find By Id
public Optional<Employee> getEmployee(int id) {
return employeeRepository.findById(id);
}
// Update Employee Details
public Employee updateEmployeeById(int id, Employee employee) {
Optional<Employee> employee1 = employeeRepository.findById(id);
if (employee1.isPresent()) {
Employee originalEmployee = employee1.get();
if (Objects.nonNull(employee.getEmployee_name()) && !"".equalsIgnoreCase(employee.getEmployee_name())) {
originalEmployee.setEmployee_name(employee.getEmployee_name());
}
if (Objects.nonNull(employee.getSalary()) && employee.getSalary() != 0) {
originalEmployee.setSalary(employee.getSalary());
}
return employeeRepository.save(originalEmployee);
}
return null;
}
// Delete Employee By Id
public String deleteEmployeeByID(int id) {
if (employeeRepository.findById(id).isPresent()) {
employeeRepository.deleteById(id);
return "Employee deleted successfully";
}
return "No such employee in the database";
}
}
DepartmentService.java
package com.EmpManagement.Service;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.EmpManagement.Entity.Department;
import com.EmpManagement.Repository.DepartmentRepository;
@Service
public class DepartmentService {
@Autowired
private DepartmentRepository departmentRepository;
// Add Department
public void addDepartment(Department d) {
departmentRepository.save(d);
}
// Get All Departments
public List<Department> getAllDepartments() {
List<Department> depts = (List<Department>) departmentRepository.findAll();
return depts;
}
// Get Department By Id
public Optional<Department> getDepartment(int id) {
return departmentRepository.findById(id);
}
// Update Department Details
public Department updateDepartment(int id, Department department) {
Optional<Department> department1 = departmentRepository.findById(id);
if (department1.isPresent()) {
Department originalDepartment = department1.get();
if (Objects.nonNull(department.getDepartment_name())
&& !"".equalsIgnoreCase(department.getDepartment_name())) {
originalDepartment.setDepartment_name(department.getDepartment_name());
}
if (Objects.nonNull(department.getShort_name()) && !"".equals(department.getShort_name())) {
originalDepartment.setShort_name(department.getShort_name());
}
return departmentRepository.save(originalDepartment);
}
return null;
}
// Delete Department
public String deleteDepartmentById(int id) {
if (departmentRepository.findById(id).isPresent()) {
departmentRepository.deleteById(id);
return "Department deleted successfully";
}
return "No such Department in the database";
}
}
8. Creating the Controllers
Create two classes EmployeeController.java
and DepartmentController.java
in the com.EmpManagement.Controller
package:
package com.EmpManagement.Controller;
import com.EmpManagement.Entity.Employee;
import com.EmpManagement.Service.EmployeeService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
import java.util.Optional;
@RestController
public class EmployeeController {
@Autowired
private EmployeeService employeeService;
// Add Employee
@PostMapping("/addEmployees")
public void addEmployees(@RequestBody Employee employee) {
employeeService.addEmployee(employee);
}
// Get all Employees
@GetMapping("/employees")
public List<Employee> getAllEmployees() {
return employeeService.getAllEmployees();
}
// Find By Id
@GetMapping("/employees/{id}")
public Optional<Employee> getEmployee(@PathVariable int id) {
return employeeService.getEmployee(id);
}
// Update Employee Details
@PutMapping("/employees/{id}")
public Employee updateEmployee(@PathVariable("id") int id, @RequestBody Employee emp) {
return employeeService.updateEmployeeById(id, emp);
}
// Delete Employee By Id
@DeleteMapping("/employees/{id}")
public String deleteEmployee(@PathVariable("id") int id) {
return employeeService.deleteEmployeeByID(id);
}
}
DepartmentController.java
package com.EmpManagement.Controller;
import java.util.List;
import java.util.Optional;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
import com.EmpManagement.Entity.Department;
import com.EmpManagement.Service.DepartmentService;
@RestController
public class DepartmentController {
@Autowired
private DepartmentService departmentService;
// Add Department
@PostMapping("/departments")
public void addDepartment(@RequestBody Department department) {
departmentService.addDepartment(department);
}
// Get All Departments
@GetMapping("/departments")
public List<Department> getAllDepartment() {
return departmentService.getAllDepartments();
}
// Get Department By Id
@GetMapping("/departments/{id}")
public Optional<Department> getDepartment(@PathVariable int id) {
return departmentService.getDepartment(id);
}
// Update Department By id
@PutMapping("/departments/{id}")
public Department updateDepartment(@PathVariable("id") int id, @RequestBody Department dept) {
return departmentService.updateDepartment(id, dept);
}
// Delete Department by Id
@DeleteMapping("/departments/{id}")
public String deleteDepartmentById(@PathVariable("id") int id) {
return departmentService.deleteDepartmentById(id);
}
}
Testing the API Endpoints
Add Employee: POST
/addEmployees
Get All Employees: GET
/employees
Get Employee by ID: GET
/employees/{id}
Update Employee Details: PUT
/employees/{id}
Delete Employee by ID: DELETE
/employees/{id}
Add Department: POST
/departments
Get All Departments: GET
/departments
Get Department by ID: GET
/departments/{id}
Update Department by ID: PUT
/departments/{id}
Delete Department by ID: DELETE
/departments/{id}
Conclusion
This project demonstrates how to build a robust Employee Management System using Spring Boot and REST API. With CRUD operations for employees and departments, along with @ManyToOne mapping, it showcases the power and simplicity of Spring Boot for developing scalable applications. Explore the full source code to see it in action!
GitHub Access
You can access the complete code for this project here. Fork the repository and try it out yourself!
About Me
Subscribe to my newsletter
Read articles from Sumeet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
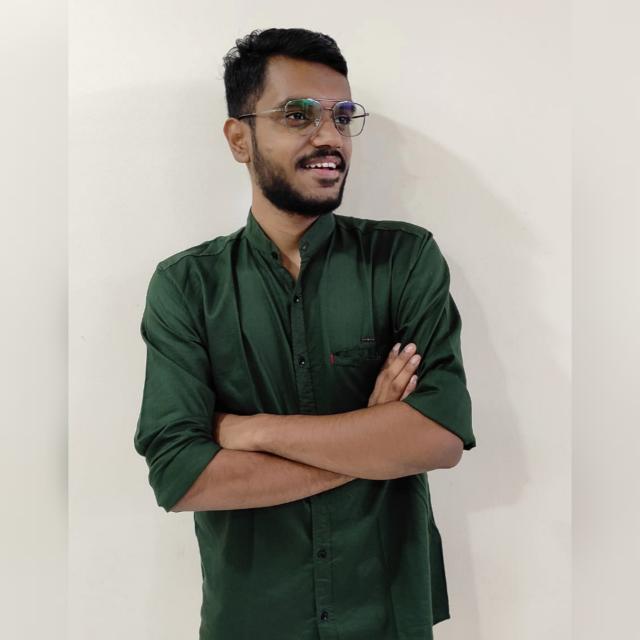