Beginner's Guide to Arrays: Learn and Understand
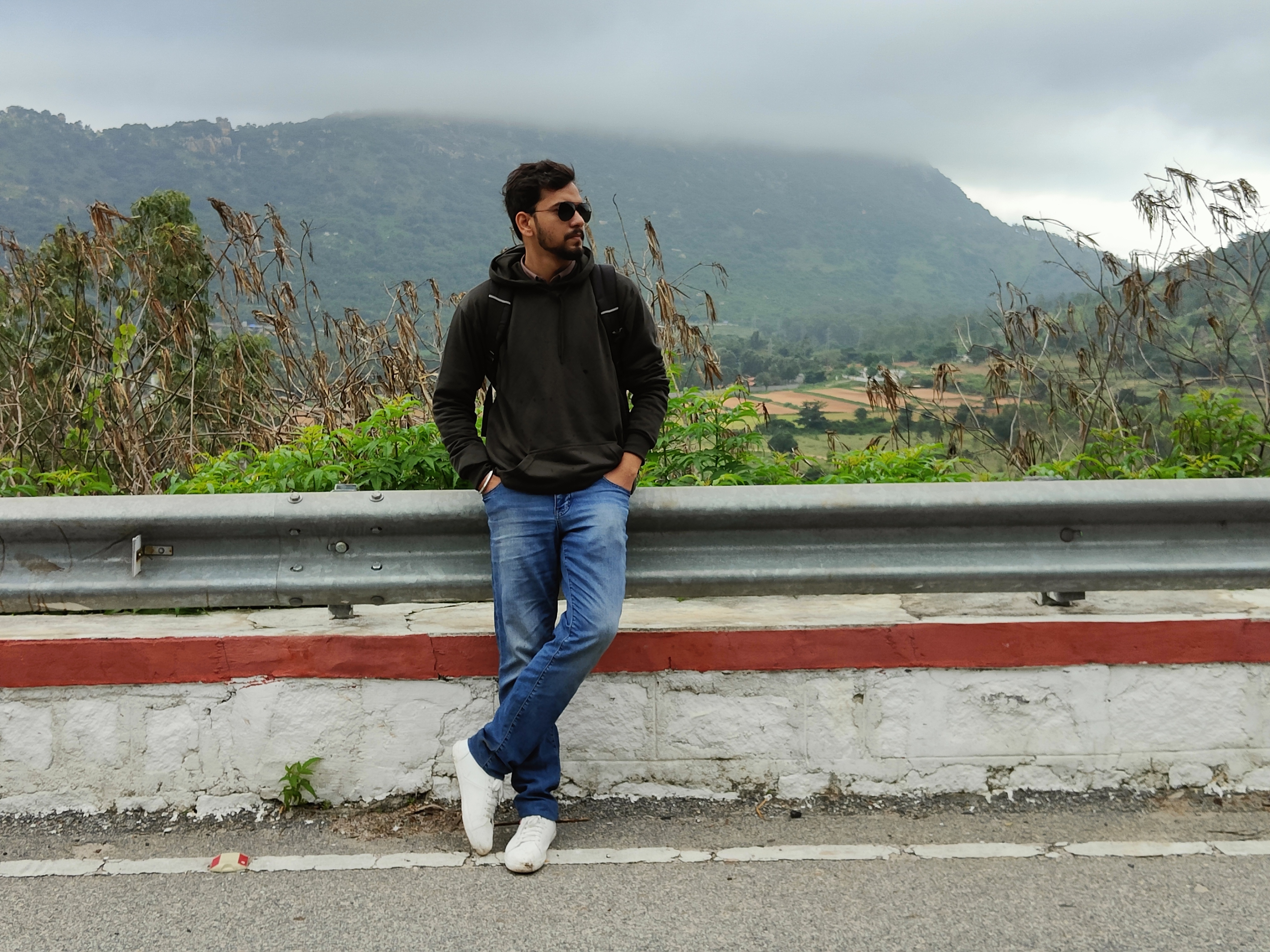
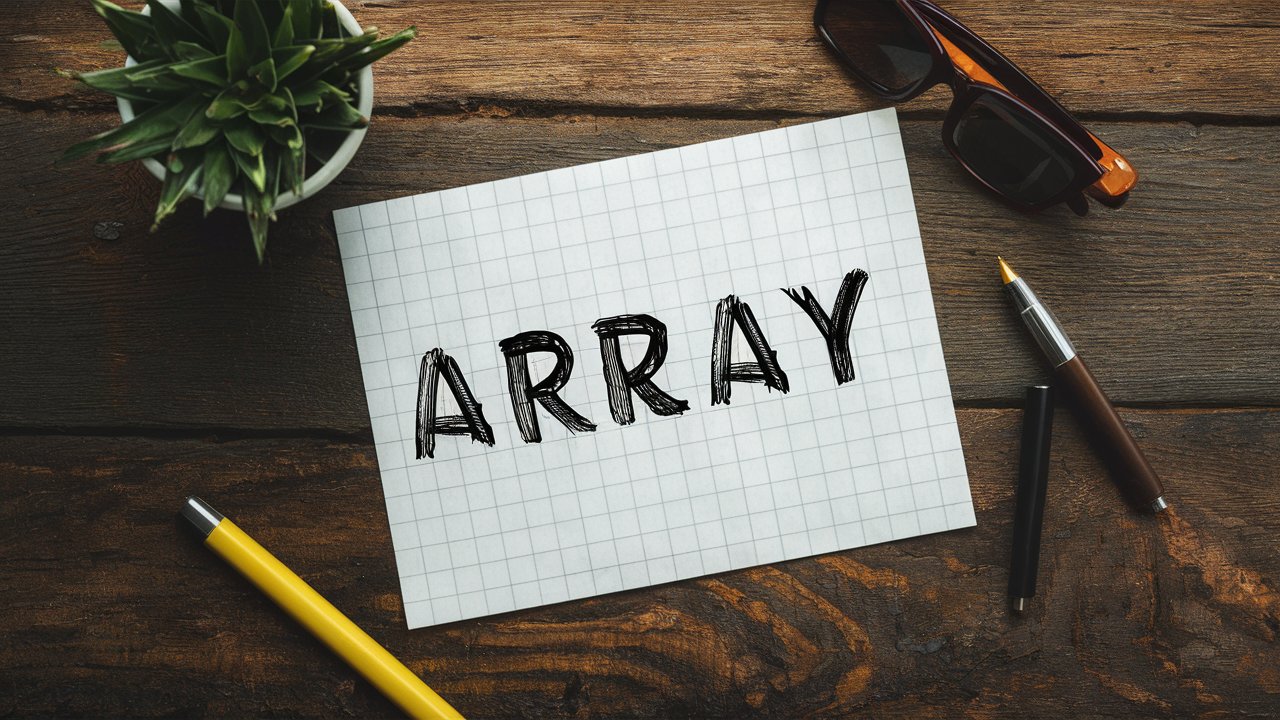
What is an Array?
An Array in C++ is a Data Structure that allows you to store a fixed-size sequential collection of elements of the same type. Arrays are commonly used in C++ programming due to their simplicity and efficiency.
Why is it important to learn the Array Data Structure?
Learning the array data structure is fundamental for several reasons:
Foundation of Other Data Structures: Arrays are the building blocks for more complex data structures such as lists, stacks, queues, heaps, and matrices. Understanding arrays is crucial to grasping these more advanced structures.
Efficient Data Storage and Access: Arrays store elements in contiguous memory locations, allowing for O(1) time complexity for accessing elements using an index. This makes arrays very efficient for tasks that require frequent access to data.
Simplifies Algorithm Implementation: Many algorithms, such as sorting and searching algorithms (e.g., quicksort, binary search), are easier to implement and understand when using arrays.
Memory Management: Arrays provide a straightforward way to manage and utilize memory, particularly in low-level programming where manual memory management is necessary.
Language and Library Support: Arrays are a fundamental part of most programming languages and are heavily used in libraries and frameworks. Knowing how to use arrays effectively is essential for utilizing these tools.
There are 2 types of Arrays
One-Dimensional Array
Two-Dimensional Array
One-Dimensional Array || 1D Array:
1D Array is a Data Structure that stores a sequence of elements of the same type in a linear arrangement. It is a simple and commonly used array format in many programming languages. Each element in a 1D array is accessed by its index, which typically starts at 0.
Characteristics:
Linear Structure: Elements are stored in a single line, and each element can be accessed using a single index.
Fixed Size: The size of the array is defined at the time of creation and cannot be changed.
Homogeneous: All Elements in the array are of the same type.
Indexing in Arrays:
Indexing in an Array Data Structure in C++ refers to accessing elements in the array using their position or index. Arrays in C++ are Zero-Indexed, meaning the first element has an index of 0, the second element has an index of 1, and so on.
In the above figure, an Array is declared of size 5 having 5 elements(10,20,30,40,50) in it. We access each element in Array using indexing which starts from 0->4.
Why does indexing in an array start from 0?
In low-level programming like C and C++, arrays are closely related to pointers. The name of an array often represents the address of its first elements.
The expression 'array[i]' (where 'i' represents indexing , 0,1,2..etc) implemented as '*(array(base address) + sizeOfDataType * i)'. Here 'array' is a pointer to the first element, and adding 'i' to this pointer gives the address of the 'i' -th element.
For example - If an array is given of type int (int takes 4 bytes in memory), let's take an address as 104 the next address would be 108. If we calculate through the formula, (104 + 4 * 1) = 108.
If arrays were 1-based additional arithmetic would be required to compute addresses, e.g., '*(array + (i + 1))', which would complicate both the compiler and the programmer's mental model.
Input and Output in an Array:
Handling Input and Output with Arrays in C++ involves reading values into an array from the user and then displaying those values.
Here's a complete program that:
Declares an Array
Takes input from the user to fill the Array
Prints the array of Elements
Define the size of the Array:
In the above figure size of an array is 5. (int arr[5]) declares an array of integers with a size specified within[].
Reading Values into the Array:
There are two ways to read inputs for Array. One way is to directly take the value on a specified index. 2nd way uses a 'for loop' to iterate from 0 to 'size-1'.
cin >> arr[i] reads an integer value from the user and stores it in the 'i' -th position of the array.
Displaying the Array Elements:
Another 'for loop' iterates from 0 to 'size-1'. cout << arr[i] prints the value at the 'i' -th position of the array along with its index.
OUTPUT:
The fill()
Function in Arrays:
The 'fill()' function in C++ is used to fill an Array or a container with a specific value.
include the <algorithm> header:
To use 'std::fill', you need to include the '<algorithm>' header.
Use fill() to fill the Array:
fill() can be used to fill an array with a specific value. You need to provide the beginning and ending pointers or iterators of the array.
Output the Array:
A Simple loop prints each element of the array to verify that it has been correctly filled with the value '23'.
Functions with Arrays:
Functions in C++ can be used to perform operations on Arrays. These functions can accept arrays as arguments, return arrays, and perform various tasks such as initialization, summation, searching, sorting, etc. Here's an overview of how to work with functions and arrays in C++.
NOTE: Pointer in C++ is a very important topic. We will further discuss pointers in detail in the later part. As of now just know pointer is a variable that holds the memory address of another variable.
Key Points:
Passing Arrays to functions:
Pass arrays to functions using pointers. The function parameter should be a pointer to the type of the array elements. When we pass an array using a pointer it is going by reference in the function parameter which means the original value is passing to the function. By default array[] always works as Pass by reference. If we don't use a pointer then also it works on original values.
For dynamic arrays. 'std::vector' from the C++ standard library is often used because it handles memory management automatically and can be returned from functions.
While passing an array to functions, we must also pass the array size as a function parameter.
Linear Search in Arrays using C++:
Linear search is a straightforward searching algorithm that checks each element in an array sequentially until it finds the target value or reaches the end of the array.
Code Analysis:
In the above code, an Array is declared of size 5. The target is set to 40.
We have to search an element 40 in an array using a linear search.
Declared a function 'findElement' and passed array, size of array, and target element.
In the 'findElement' function there is a logic for searching an element is written.
Using a for loop we will iterate over each element and check if that element is the same as the target element. If it matches it will return the index of the element if not we will continue searching elements in the array till the size of the array.
Output:
Let's explore some fundamental questions regarding one-dimensional arrays:
Find Max Element:
Code Analysis:
In the above code, an array of size 5 is given. We need to find the maximum element in the given array of elements.
In the function, we initialized the max variable with INT_MIN. It's a good practice to use INT_MIN && INT_MAX as max and min elements.
We Linearly search each element one by one and check whether it is greater than the max element if yes we will initialize it with max.
Output:
Count 0s & 1s:
Code Analysis:
In the above code, we need to find how many 0 and 1 are present in the given array.
The size of the array is passed in the function parameter.
In function, we declare two variables which will count as 0 & 1. It will increment by 1 if any of the values is present.
Output:
Shift 2 elements in an array by 2 places in a clockwise direction:
Code Analysis:
We need to shift the last element by two places which means it will come to the 0th and 1st index.
Pass an array with its size. Inside the function, first, we will check how many shifts are possible. If all elements need to be shifted, it returns the original array.
Then we will declare a temp array which will store the last 2 elements.
After this, we will shift the rest all the elements to their last place. Then we copy the temp elements in the original array.
Output:
Two-Dimensional Array || 2D Array
In C++, a 2D array is an array of arrays. It's essentially a grid of elements arranged in rows and columns. Each element in a 2D array is identified by two indices: the row index and the column index.
In the above figure, an array is declared as rows =3, columns = 3.
The first box shows rows and the second box shows columns.
Code Analysis:
In the above figure, an array is declared as having 3 rows and 2 columns.
Same as a 1D array rows and columns start from 0 and 0.
In a 2D array, we will make use of nested loops same as patterns. For each row column will run from 0 to its size.
Output:
1 2 3 4 5 6
Horizontal Wave printing - 2D Array:
Code Analysis:
The print wave function prints the elements of the 2D array in a wave pattern according to the specified direction(horizontal or vertical).
In horizontal Wave printing, it iterates through each row and alternates between left-to-right and right-to-left directions on each row.
In vertical wave printing, it iterates through each column and alternates between the top-to-bottom and bottom-to-top directions on each column.
Output:
Remember, the journey to mastery is ongoing, and each step you take brings you closer to becoming a proficient programmer. Embrace the challenges, celebrate the victories, and never stop learning.
Happy coding!
Subscribe to my newsletter
Read articles from Rishabh Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
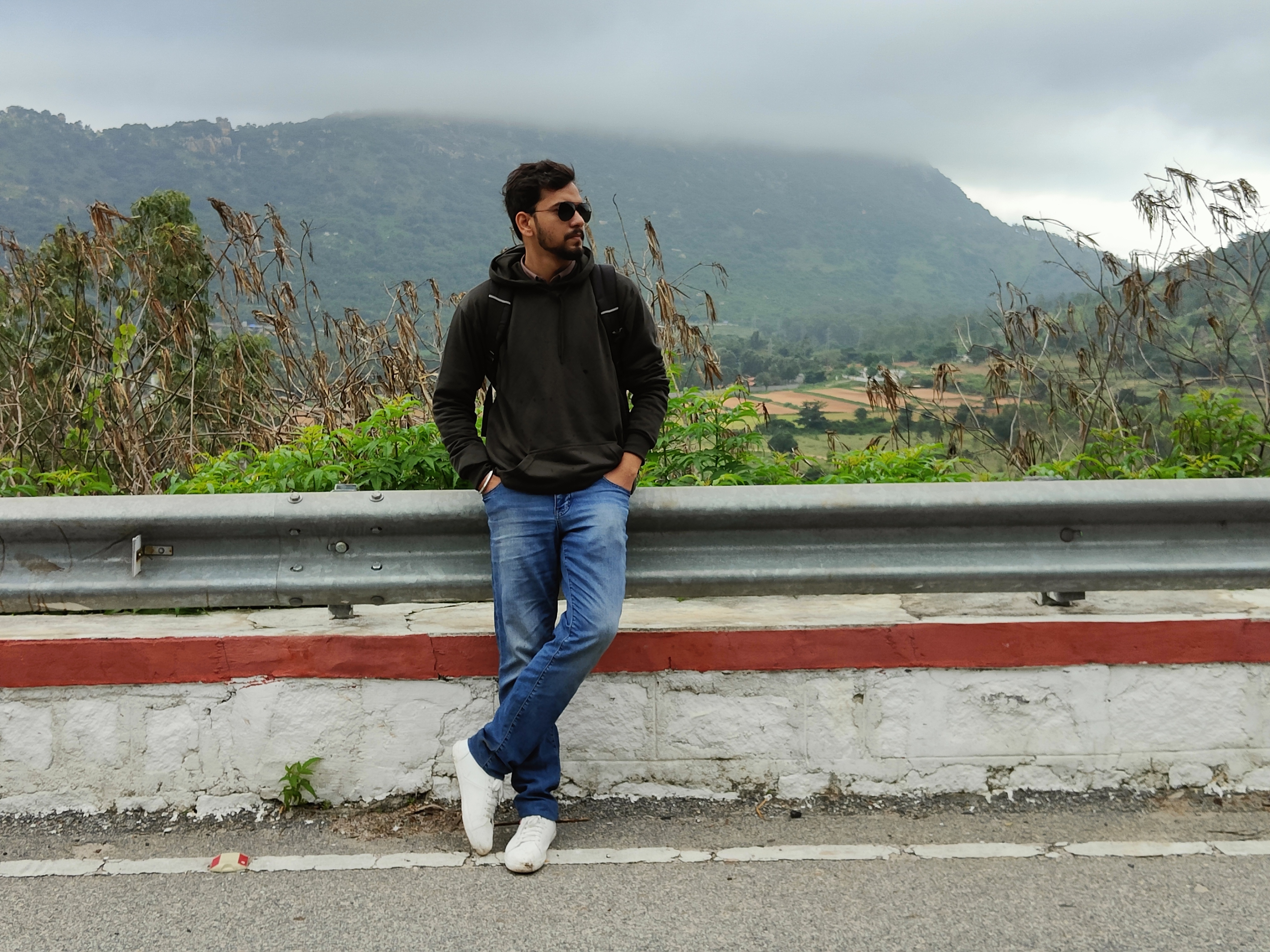