Understanding the Basic Syntax of Go
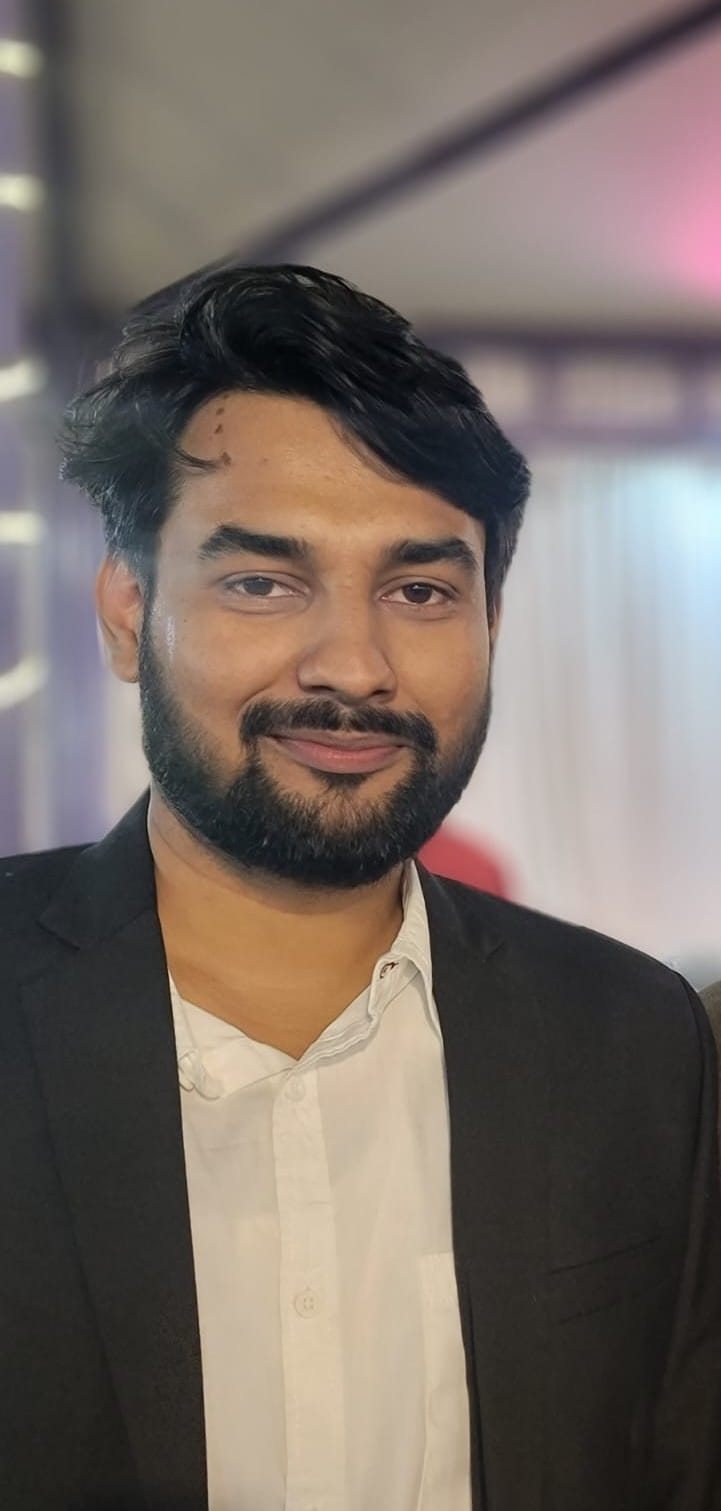
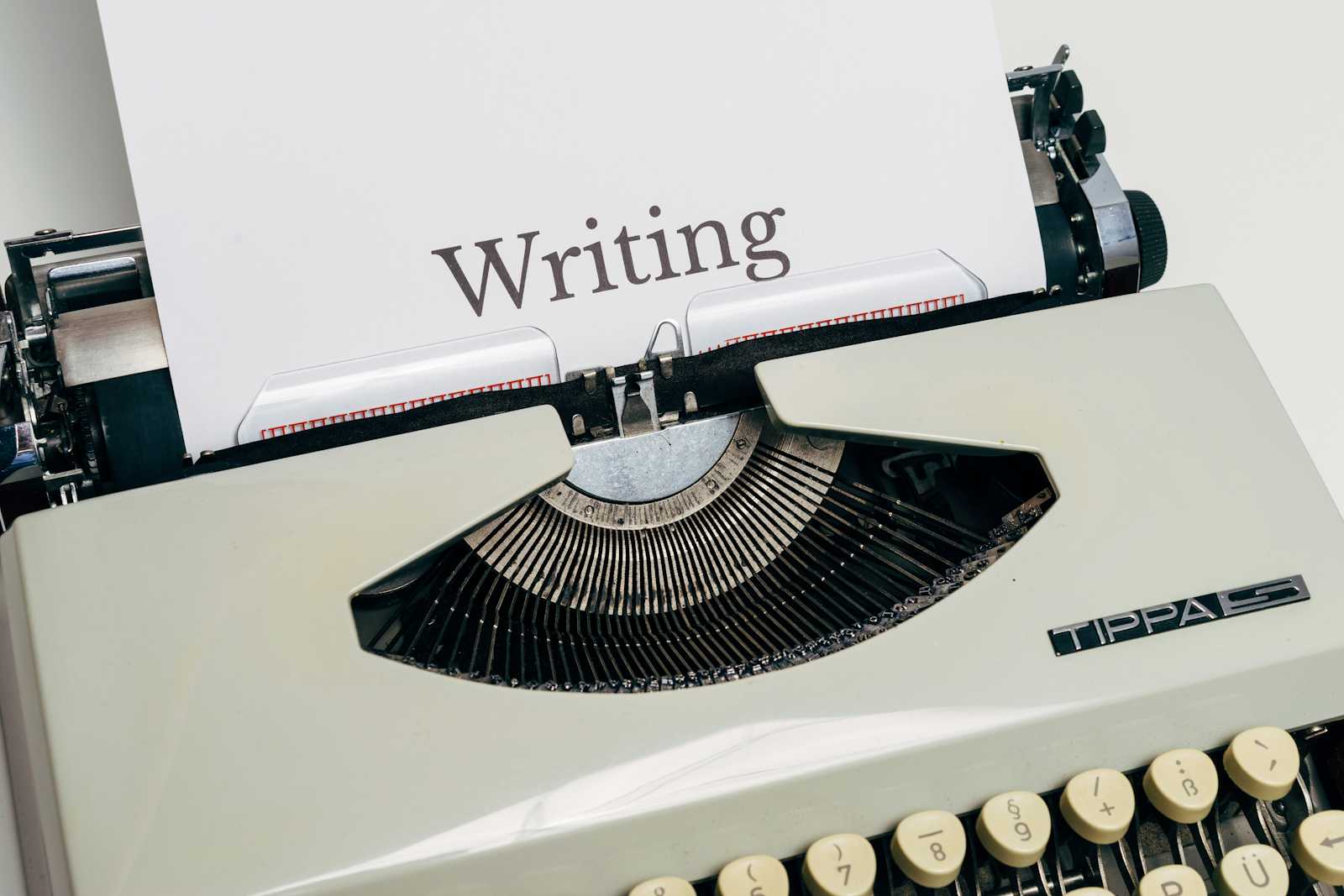
Go (Golang) is known for its simplicity and clarity, which makes it an excellent choice for both new and experienced programmers. In this article, we will explore the basic syntax of Go, focusing on variables and constants, data types and type inference, and basic operators and expressions.
Variables and Constants
In Go, variables are used to store data that can change during the execution of a program, while constants are used for values that remain unchanged.
Variables:
To declare a variable in Go, you use the var
keyword, followed by the variable name and type. For example:
var name string
var age int
You can also initialize a variable at the time of declaration:
var name string = "Alice"
var age int = 25
Go provides a shorthand for variable declaration and initialization using the :=
syntax, which allows you to omit the var
keyword and the type:
name := "Alice"
age := 25
Constants:
Constants are declared using the const
keyword and must be initialized at the time of declaration:
const pi float64 = 3.14
const greeting = "Hello, World!"
Constants, unlike variables, cannot be reassigned after they are defined.
Data Types and Type Inference
Go is a statically typed language, meaning that the type of a variable is known at compile time. It supports several basic data types:
Integer Types:
int
,int8
,int16
,int32
,int64
Unsigned Integer Types:
uint
,uint8
,uint16
,uint32
,uint64
Floating-Point Types:
float32
,float64
Complex Types:
complex64
,complex128
Boolean Type:
bool
String Type:
string
Type Inference:
Go can automatically infer the type of a variable based on the assigned value, which is particularly useful for concise code. For example:
name := "Alice" // string
age := 25 // int
height := 5.9 // float64
isStudent := true // bool
In these cases, Go infers the type from the initial value assigned to the variable.
Basic Operators and Expressions
Operators in Go are used to perform operations on variables and values. They can be categorized as follows:
Arithmetic Operators:
+
(Addition)-
(Subtraction)*
(Multiplication)/
(Division)%
(Modulus)
Example:
a := 10
b := 3
sum := a + b // 13
difference := a - b // 7
product := a * b // 30
quotient := a / b // 3
remainder := a % b // 1
Relational Operators:
==
(Equal to)!=
(Not equal to)<
(Less than)>
(Greater than)<=
(Less than or equal to)>=
(Greater than or equal to)
Example:
a := 10
b := 3
isEqual := (a == b) // false
isNotEqual := (a != b) // true
isLess := (a < b) // false
isGreater := (a > b) // true
Logical Operators:
&&
(Logical AND)||
(Logical OR)!
(Logical NOT)
Example:
isTrue := true
isFalse := false
andResult := isTrue && isFalse // false
orResult := isTrue || isFalse // true
notResult := !isTrue // false
Assignment Operators:
=
(Assign)+=
(Add and assign)-=
(Subtract and assign)*=
(Multiply and assign)/=
(Divide and assign)%=
(Modulus and assign)
Example:
a := 10
a += 5 // a = a + 5 => a = 15
a -= 3 // a = a - 3 => a = 12
a *= 2 // a = a * 2 => a = 24
a /= 4 // a = a / 4 => a = 6
a %= 3 // a = a % 3 => a = 0
Putting It All Together
Let's create a simple Go program that utilizes variables, constants, data types, type inference, and basic operators.
package main
import "fmt"
func main() {
// Constants
const pi = 3.14
// Variables
var radius float64 = 5.0
// Type inference
circumference := 2 * pi * radius
// Arithmetic operations
var diameter = radius * 2
var area = pi * radius * radius
// Print results
fmt.Println("Radius:", radius)
fmt.Println("Diameter:", diameter)
fmt.Println("Circumference:", circumference)
fmt.Println("Area:", area)
// Logical and relational operations
isLargeCircle := area > 50
fmt.Println("Is it a large circle?", isLargeCircle)
}
In this program, we declare constants and variables, use type inference, perform arithmetic operations, and print the results. We also demonstrate the use of relational and logical operators.
Conclusion
Understanding the basic syntax of Go is essential for writing efficient and clear code. By mastering variables and constants, data types and type inference, and basic operators and expressions, you can build a strong foundation in Go programming. Continue exploring these concepts and practice writing your own Go programs to enhance your skills.
Subscribe to my newsletter
Read articles from Lokesh Jha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
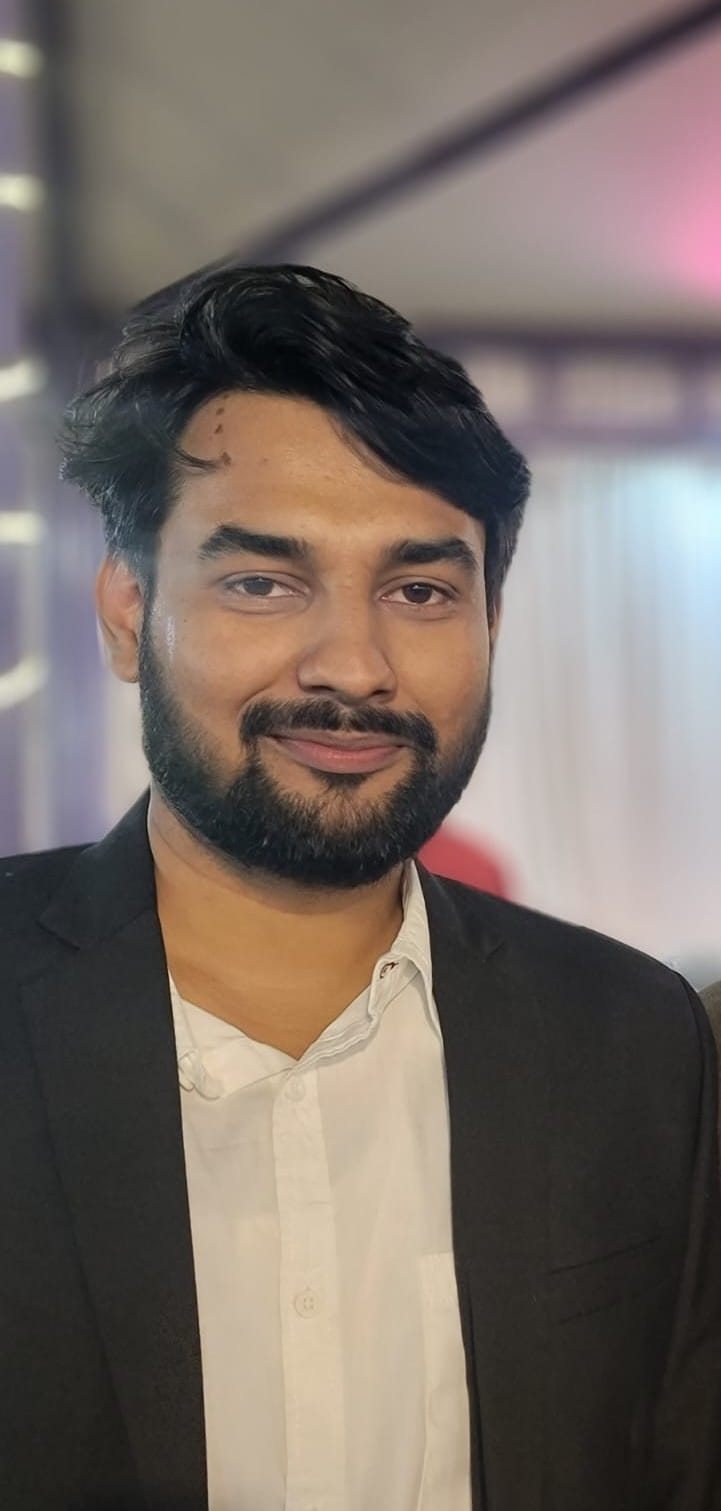
Lokesh Jha
Lokesh Jha
I am a senior software developer and technical writer who loves to learn new things. I recently started writing articles about what I've learned so that others in the community can gain the same knowledge. I primarily work with Node.js, TypeScript, and JavaScript, but I also have past experience with Java and C++. Currently, I'm learning Go and may explore Web3 in the future.