What are webhooks?
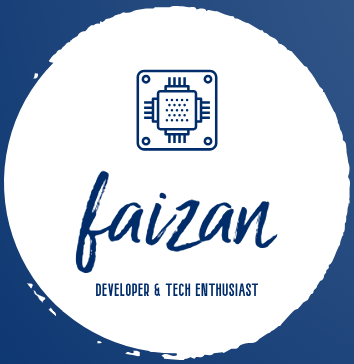
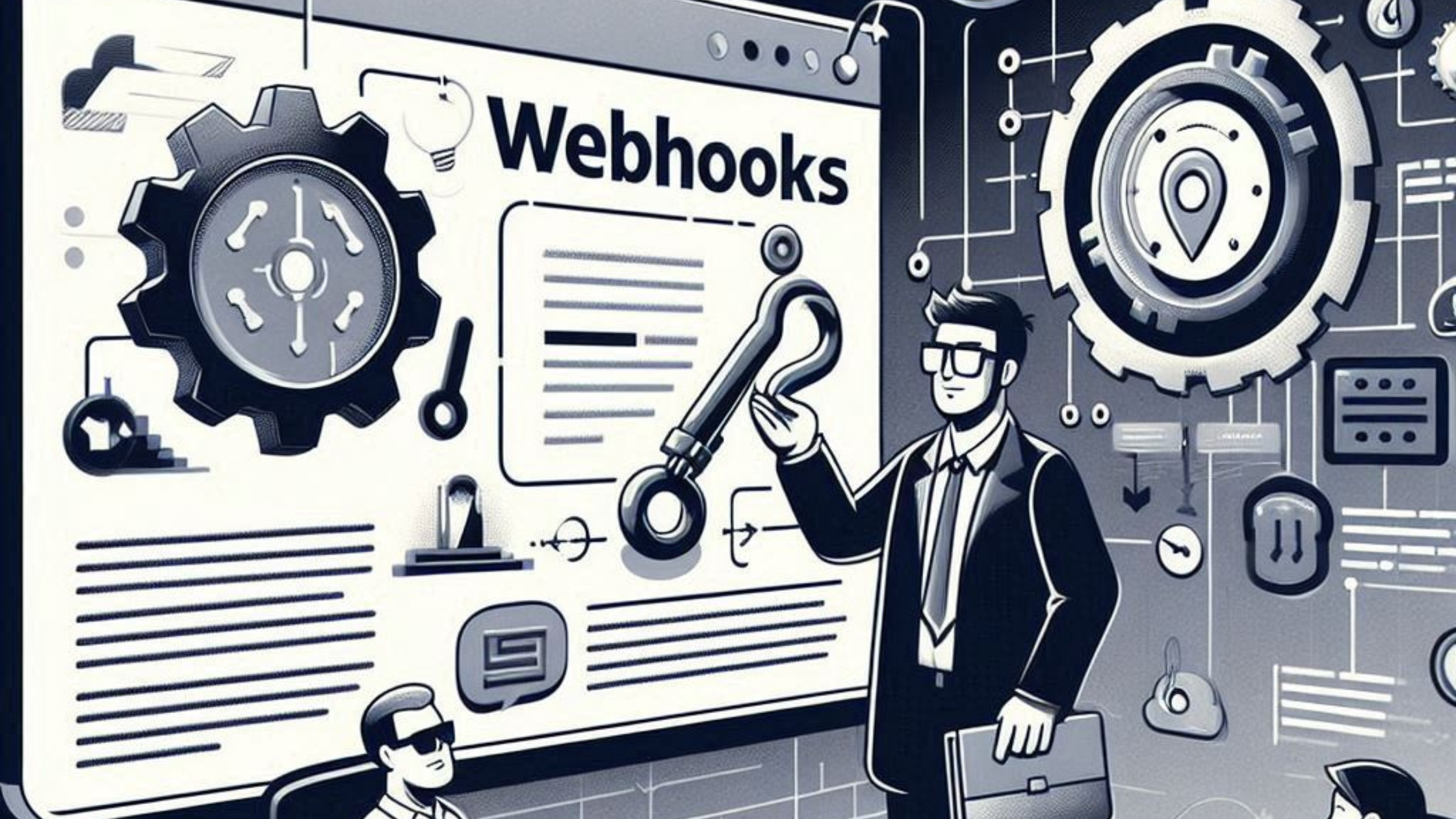
In modern technology, everything is connected to each other and every App works seamlessly through the combination and coordination of many services. This coordination is achieved by webhooks.
Webhooks are HTTP-based callback functions in which one service uses APIs to instantly notify another service of an event. That’s the simple version. Technically, webhooks are “user-defined callbacks made with HTTP” according to Jeff Lindsay, one of the first people to conceptualize webhooks.
The Basics of Webhooks
Here’s a breakdown of the basics of webhooks:
Event-driven chat: Instead of constantly checking, apps can use webhooks to chat with each other whenever something specific happens, like a new customer order or a friend’s birthday.
Push notification, not waiting: Forget about refreshing a page to see updates. Webhooks are like getting a notification — the app instantly tells another app when something important happens. This is quicker and saves time.
Web messages with details: Webhooks use web calls to send messages. These messages include a short report, like a news update, about what happened, often in a format like JSON.
Updates in real-time: Because webhooks deliver information right away, apps can stay up-to-date with each other instantly.
How Webhooks Work?
working of webhooks by the Author
Event Occurs: An event triggers the webhook process. This event can be anything from a new customer signing up on a website to a change in inventory levels in a store.
HTTP POST: Once the event is triggered, an HTTP POST request is sent to a designated URL, which is the webhook URL. This URL points to a web server that’s designed to receive and handle these webhook notifications.
Webhook Triggered: Upon receiving the HTTP POST request, the webhook is triggered on the web server.
Notification: The webhook server then parses the data included in the HTTP POST request. This data typically contains details about the event that triggered the webhook.
Request Received & Processed: The webhook server then verifies the authenticity of the request and processes the data accordingly.
Action Taken: Finally, based on the data received from the event, the webhook server performs a specific action. This action can vary depending on the specific application but could involve updating a database, sending an email notification, or triggering another workflow.
In simpler terms, webhooks act as messengers between applications. They notify applications about specific events and provide them with relevant data so they can take appropriate actions.
Implementing Webhooks with GitHub
Now that we have seen the basics and understood the workings of webhooks, let us try to implement it in one of the most popular Dev Apps — Github.
Step 1: Navigate to Your Repository Settings
Log in to your GitHub account.
Go to the repository where you want to set up the webhook.
Step 2: Add a New Webhook
In the Payload URL field, enter the URL of the server where you want to receive the webhook payloads.
Step 3: Configure Payload URL and Content-Type
Choose the Content type. For JSON payloads, select
application/json
.Optionally, set a Secret for security, which will be used to validate received payloads.
Step 4: Select Events to Trigger the Webhook
- Decide which events should trigger the webhook. You can select individual events or choose to receive them all.
Step 5: Activate the Webhook
Example Code Snippet for a Webhook Receiver in Node.js:
const express = require('express');
const crypto = require('crypto');
const bodyParser = require('body-parser');
const app = express();
const port = 3000;
const secret = 'mySecret'; // Replace with your GitHub webhook secret
app.use(bodyParser.json());
app.post('/webhook', (req, res) => {
const signature = `sha1=${crypto.createHmac('sha1', secret)
.update(JSON.stringify(req.body))
.digest('hex')}`;
if (req.headers['x-hub-signature'] === signature) {
console.log('Webhook received:', req.body);
// Handle the webhook event
res.status(200).send('Webhook received!');
} else {
res.status(401).send('Invalid signature');
}
});
app.listen(port, () => {
console.log(`Server is listening at http://localhost:${port}`);
});
This code creates a simple server that listens for POST requests at /webhook
. It verifies the request signature using the secret you configured in GitHub. When a webhook event occurs, GitHub sends a POST request to the specified Payload URL with the event’s data. Your server can then process this data accordingly.
Remember to replace 'mySecret'
with the actual secret you set in GitHub. This ensures that the payloads you receive are indeed from GitHub and not an imposter.
Some real-world examples of webhooks include the following:
Instagram photos that automatically upload to Twitter accounts.
A connected doorbell is configured to flash certain lights inside a home as it rings.
Sending GitHub update notifications to Slack or Discord channels as messages.
Creating a Microsoft Teams channel that relays messages when people buy or sell certain stocks.
Benefits and Best Practices
Advantages of Using Webhooks:
Real-Time Data Transfer: Webhooks provide immediate communication between systems, allowing for instant data exchange as events occur.
Efficiency: They eliminate the need for constant polling, reducing server load and improving performance.
User Experience: Immediate updates enhance user interfaces, making them more responsive and dynamic.
Flexibility: Webhooks can be tailored to trigger specific actions for different events, offering customization in how applications interact.
Best Practices for Effective Use of Webhooks:
Secure Connections: Use HTTPS to ensure that the data transmitted by webhooks is encrypted and secure.
Verify Webhooks: Implement verification methods, such as validating digital signatures, to confirm that incoming data is from a trusted source.
Error Handling: Design your system to handle failures gracefully, including retries and alerts for unsuccessful webhook deliveries.
Logging: Maintain detailed logs of webhook activity to facilitate debugging and provide an audit trail for the data flow.
By leveraging the advantages of webhooks and adhering to these best practices, developers can create efficient and reliable integrations for their applications.
Conclusion
Webhooks, with their real-time, event-driven capabilities, are a transformative tool in today’s digital economy. Webhooks expedite workflows, improve responsiveness, and promote efficiency across several domains by allowing apps to communicate fluidly and automatically with one another. Webhooks, whether used for continuous integration, automated notifications, or dynamic data synchronization, minimize the need for manual involvement, decreasing errors and speeding up procedures.
References:
Webhooks Explained: What Are Webhooks and How Do They Work?
Introduction to webhooksmedium.com
Thank you for reading! If you have any feedback or notice any mistakes, please feel free to leave a comment below. I’m always looking to improve my writing and value any suggestions you may have. If you’re interested in working together or have any further questions, please don’t hesitate to reach out to me at fa1319673@gmail.com.
Subscribe to my newsletter
Read articles from Md Faizan Alam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
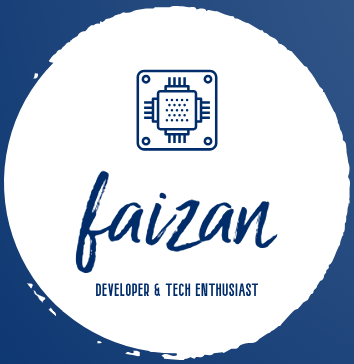
Md Faizan Alam
Md Faizan Alam
I am a Fullstack Developer from India and a Tech Geek. I try to learn excting new technologies and document my journey in this Blog of mine. I try to spread awareness about new and great technologies I come across or learn.