Create your own telegram bot with python and deta.sh
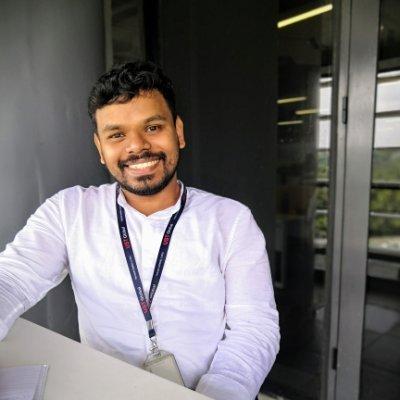
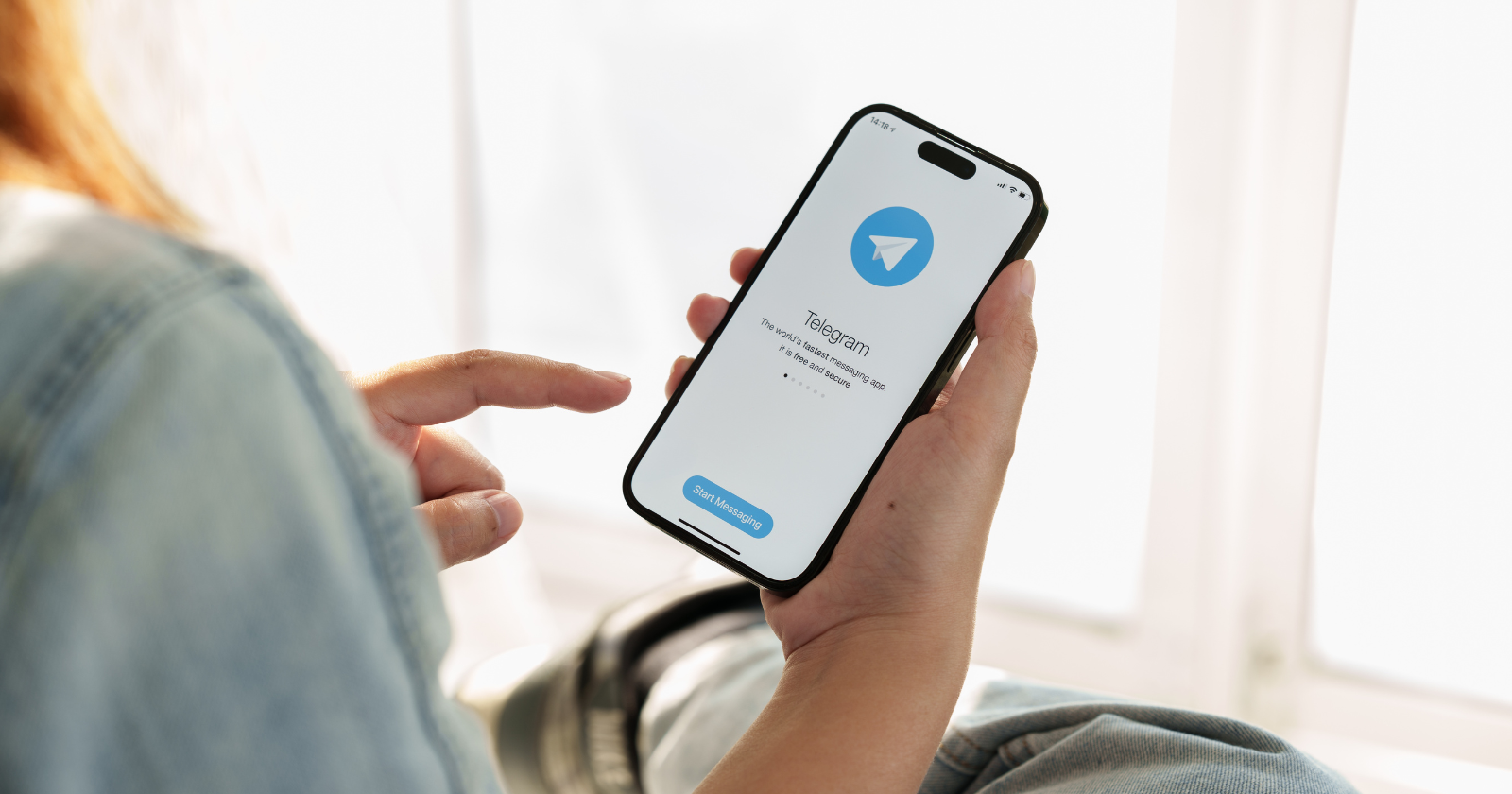
Creating your own telegram bot and doing simple things with the telegram is easy and fun tasks and with deta free web app, you don’t have to have any paid cloud service for deploying a simple app. It is simple to create and customize and deploy your telegram bot with minimal python coding knowledge and that’s all you need.
We will start off by introducing you to what is telegram bot, bots and small third-party applications which runs inside the bigger app that is telegram. By the way, if you don’t know what is telegram, then it is a encrypted cloud based messaging app which is available in Platforms like android, iOS, windows, Linux, Mac, etc. By creating telegram bot, you can integrate your favourite services, get notification and news, build games etc.
Without further ado, let’s get started to creating telegram bot.
This tutorial requires accounts in telegram, and deta.sh.
Telegram
If you doesn’t already have go ahead download and install the telegram app in your mobile or computer by searching on the application store and sign-in by your mobile number, telegram will sent an OTP to your mobile number which will be used for identifying it is your number, add your name and profile photo which is optional and voilà, your account in created.
Deta (https://www.deta.sh/)
Deta is a cloud based micro service solution which let's you deploy your python or node based app in a matter of seconds. Deta has the below listed services and some of the services in beta stage and some are coming soon.
- Deta micros, which stands for micro services
- Deta base, which is a no sql database service
- Deta drive, cloud drive services
- Deta access, permission management
Go ahead and create an account in deta and we need to download deta cli tools to work with deta, download it for your computer (whichever your operating system is, mine in Linux), you will find the instructions in the deta website and login with deta cli.
With all the downloading and installing done we can go ahead with the fun part of creating the bot.
The telegram bot creation is split into four parts,
Creating your telegram bot in telegram
Go ahead into your telegram and press the search icon in the top left corner and put “botFather” in the search box and find the one with the blue tick to it’s right of the name.
Bot father search result
The first one is the one we want. Go ahead and click on it and click the start button which is in the welcome page of the “botFather”.
Now we need to create a bot using the commend “/newbot” command it will ask for your bot name(which is not unique, you can give anything you want) and a username(which has to be unique and must end with 'bot’). In the screenshot below you can see I have choosen 'Telegram bot tutorial' as the name and 'Teletutorialbot' as the name(since you have to have unique username, it may take multiple tries).
Here we got a link to the bot that we have created, and a unique token, which is used to communicate with our bot, never reveal your bot token since that is used for complete control of your bot. Now your bot is ready you can set photo, about text and description of your bot at this stage. Please click the link which is created for your link which is in the format 't.me/' for accessing your bot, at this time your bot won’t do anything, we will get to that part later.
Creating a fastapi server in deta
We will create a fastapi server next for the telegram bot to communicate back and forth, this server will be the one which is receiving message from the user and sending response back to the user. Creating a fastapi server in deta is simple and you can refer their document for more details.
We will be doing it the same way as the documents suggests.
First of all we will create a new folder or directory and open that folder in your favourite code editor, I am using vscode with black theme (because I am not a geek).
for Linux users you can use the commands.
mkdir telegram-bot-tutorial && code telegram-bot-tutorial
Create a main.py file and put the below Contents in it
Now create a requirements.txt file and put 'fastapi' without the quote in it.
And finally run
deta new
command in your terminal for creating a new deta micros. This will create a new micro project, install the dependency from the requirements.txt file.
At this stage your deta project is created in your account, go ahead and deploy the service with 'deta deploy’. You will also need the endpoint that is generated from the deta new command going forward. In my case it’s
https://6sfsh0.deta.dev
Don’t copy my link, create your own you morons 😏
You can go ahead and open the link in your favourite browser and get the hello world in there.
Initializing webhook in telegram bot
Initializing webhook in telegram bot requires the url of the server which we have deployed in the last stage and the token that we have generated when creating bot. You can also head over to the telegram bot api documentation for advanced usage of the api, for starters we will need to initialize the webhook and tell the telegram server which server we are using for sending and receiving messages.
The telegram api url follows the following pattern
http://api.telegram.org/bot/method
For our purpose we will need the method 'setWebhook' and token which we obtained from first step. And it needs a parameter url which will be our server url.
So in our case the url will be
http://api.telegram.org/bot1836606926:AAEoxnNXWMLvsIlij9ENJomlLZM7qg6V6Zk/setWebhook?url=https://6sfsh0.deta.dev/
Where 1836606926:AAEoxnNXWMLvsIlij9ENJomlLZM7qg6V6Zk is the token generated for the bot.
And the url of our fastapi server is https://6sfsh0.deta.dev/
When we paste the url in the browser, we will get a message saying our webhook is set.
Congrats you are 90% done.
Sending/receiving message telegram server
Since we don’t want anyone else to know our token for telegram bot we will input that as an environment variable, which will keep it away from the code and prying eyes.
Go back to your vscode or the code editor you were using and create a file named '.env' and put the below content in it
We will go to the terminal and tell deta that we want the environmental variable to be added to your code by running the command
deta update --env .env
Where '.env' is the location of the environmental variable file, if your location is different please change accordingly.
Now we will update the main.py file to get message from the telegram server and sent back appropriate response back to the user. For this tutorial we will sent back the exact text the user sent to the bot, looking forward to create more tutorials in the future which will incorporate more features of telegram.
The above picture is the entire code required for it, you can see we have barely 24 lines of codes for the entire bot. We will go line by line and explain what each line does.
In the first two lines we are importing the os module in the python which will help getting the key variable which we have put into '.env' file. We save the variable with name my_secret.
We will give the base_url the starting url of the telegram server which we needed to use several places in the code.
In the lines 6-8 we will import the fastapi, requests and pydantic module, the fast api module we were importing for the server before. Requests module is used to sent and recieve http connections from telegram server. And lastly pydantic is used for defining dataclass which will enforce the data in specific format.
After Initializing the fastapi app in line 10, we will go ahead and create a data class using the base model from the pydantic module, which is the data receiving from the telegram server. It is fully optional to use the base model, but it will make the fastapi documentation easier which we can access for our server in the endpoint '/docs’.
Functon 'get_response' is used to echo the user input back to the user, we can further improve this function to give whatever response we like. For now it will return the same message which is recieved from the user.
We have changed the fastapi server get response that we initially created to respond with 'chatbot is up' instead of hello world for understanding.
Going into line 23 is the real magic happens.
whenever a user message is received in telegram server it pushes the message to our server in a post method. We will use the data which is sent by the telegram server in this function to respond to the user when some input or event occurs. We extract the message data from the incoming message from telegram server which is in the below format
We need the 'id' and message 'text' content from this format for processing. We get that in line 26 and 28 respectively.
Using the function 'get_response' we will get the appropriate response, which in this case is the user text itself. If that doesn’t make any sense now, your get_response function can return what ever you want.
With the id and bot response in hand we can send the message back to the telegram server in the method 'sendMessage’. The url follows the same format as before like base_url then token and then the method. Creating the url is in line 29 using f-string functionality of python and we will use the request module to sent back a post request to the telegram server itself with parameters 'chat_id' which is the ID we obtained from the request and 'text' which is the bot response we have created.
Congarts now, you have created your first telegram bot with webhook. Now go ahead and deploy the changes in you fastapi application using the command
deta deploy
And go to your telegram bot(using your username or the link proveded while creating your bot in botfather) in the application and send any message, the bot will respond back with the same message.
This is my first try in creating a blog of what I have done in telegram, with your support I am hoping to create more articles like this and help others as well. If you have any suggestions and feedback ping me in telegram by clicking here.
Thank you.
Subscribe to my newsletter
Read articles from Noufal Salim directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
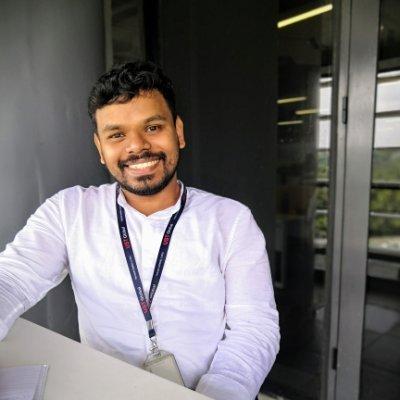