I am able to set cookie in Postman, but not able to set it in browser
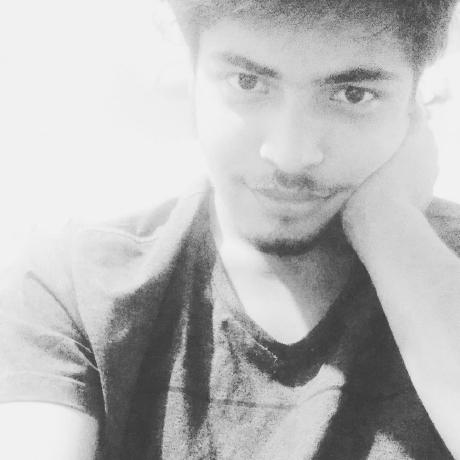
2 min read
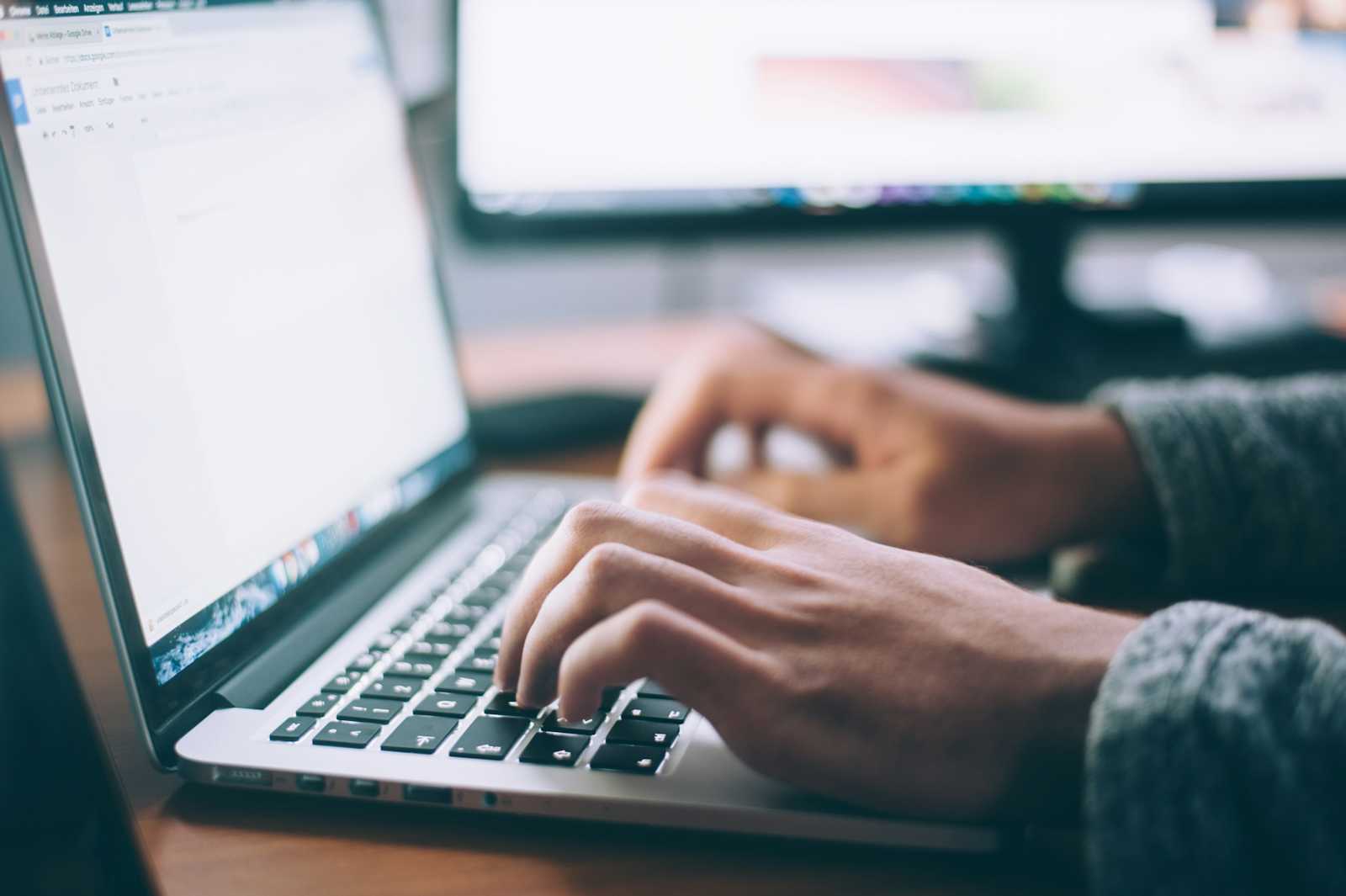
I faced the same issue, but then I tried this solution. My backend is built in Node.js, and for the frontend, we just need to add this line: withCredentials: true
.
Here's what I did:
// my backend code
// just do this simple thing in your cors
app.use(cors({
origin: 'http://localhost:3000',
credentials: true,
}));
// route for login
app.post('/login', async (req, res) => {
try {
const { email, password } = req.body;
if (!email || !password) {
return res.status(400).json({ message: 'All fields are required' });
}
const user = await userModel.findOne({ email });
if (!user) {
return res.status(404).json({ message: 'User not found' });
}
const passOk = await bcrypt.compare(password, user.password);
if (passOk) {
const jwtSecret = process.env.JWT_SECRET;
const data = { userId: user._id, email };
const token = jwt.sign(data, jwtSecret, { expiresIn: '10h' });
res.cookie('token', token, { httpOnly: true }).status(200).json({
id: user._id,
message: 'Login successful',
});
return res;
} else {
return res.status(400).json({ message: 'Wrong Password' });
}
} catch (error) {
console.log(error);
return res.status(500).json({ message: 'Something went wrong' });
}
});
And here is my frontend code
const loginFunction = async () => {
try {
// Here just adding this simple thing....
// and it worked for me...
const config = {
headers: {
"Content-Type": "application/json"
},
withCredentials: true
}
const data = { email: email, password: password };
const res = await axios.post('/login', data, config);
console.log(res);
if (res.status === 200) {
alert('login done');
navigate('/home');
} else {
alert('login failed');
}
} catch (err) {
console.log(err);
}
}
I am excited to share this solution with you! ๐ I hope it's just what you needed. Happy coding ahead! ๐
0
Subscribe to my newsletter
Read articles from Abhinav Jha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
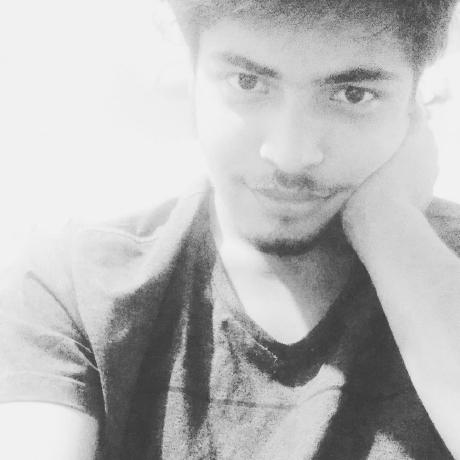
Abhinav Jha
Abhinav Jha
Software Engineer